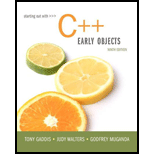
Palindromic Numbers
A palindromic number is a positive integer that reads the same forward as backward. For example, the numbers 1, 11, and 121 are palindromic. Moreover, 1 and 11 are very special palindromic numbers: their squares are also palindromic. How many positive integers less than 10,000 have this property? Write a
The beginning part of your output should look like this:
1 has square 1
2 has square 4
3 has square 9
11 has square 121
22 has square 484
Hint: If str is a string object, the reverse () function (declared in <
reverse(str.begin(), str.end());

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Starting Out with C++: Early Objects (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: Early Objects (6th Edition)
Database Concepts (8th Edition)
Absolute Java (6th Edition)
Computer Science: An Overview (12th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Computer Systems: A Programmer's Perspective (3rd Edition)
- A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes in word pairs that consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Ex: If the input is: Joe 123-5432 Linda 983-4123 Frank 867-5309 Frank the output is: 867-5309 please make it simple im a starter at pythonarrow_forwardMake Camel Case. Create a program that reads a multi-word phrase, and prints the Camel Case equivalent. For example, for input “total account balance” the program shall output “totalAccountBalance”.Notice that the first word is in lowercase (even if it contains uppercase letters). The rest of the words, only their initials are capitalized.arrow_forwardA string is a palindrome if it is identical forward and backward. For example “anna”,“civic”, “level” and “hannah” are all examples of palindromic words.Write a programthat reads a string from the user and uses a loop to determines whether or not it is apalindrome. In javaarrow_forward
- java: Run length coding is a method to represent a string in a more compact manner. Each character that occurs more than 2 times in a row is represented by the character and a number following it. Two examples are: "abba" → "abba""abcccbbbba" → "abc3b4a"Write a function that calculates how many characters the encoded string is shorter than the original.arrow_forwardpython Write a Pig Latin converter. We will use a simple version of Pig Latin. To convert a word to Pig Latin: If the first letter of a word is a consonant: remove the first letter and place that letter at the end of the word. Then, append the string "ay" to the end of the word. If the first letter of the word is a vowel (a, e, i, o, u): Simply append "way" to the end of the word. Treat the letter "y" as a consonant. Make sure all letters in the Pig Latin word are lowercase. Ex: If the input is: Sleep the output is: leepsay Ex: If the input is: apple the output is: applewayarrow_forwardA contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Ex: If the input is: 3 Joe 123-5432 Linda 983-4123 Frank 867-5309 Frank the output is: 867-5309 Your program must define and call the following function. The return value of GetPhoneNumber is the phone number associated with the specific contact name.string GetPhoneNumber(vector<string> nameVec, vector<string> phoneNumberVec, string contactName) #include <iostream>#include <vector>using namespace std; string GetPhoneNumber(vector<string> nameVec, vector<string> phoneNumberVec, string contactName) {} int main()…arrow_forward
- Q12,. A phrase is a palindrome if, after converting all uppercase letters into lowercase letters and removing all non-alphanumeric characters, it reads the same forward and backward. Alphanumeric characters include letters and numbers. Given a string s, return true if it is a palindrome, or false otherwise. Example 1: Input: s = "A man, a plan, a canal: Panama" Output: true Explanation: "amanaplanacanalpanama" is a palindrome. Example 2: Input: s = "race a car" Output: false Explanation: "raceacar" is not a palindrome..arrow_forwardEncryption is commonly used to disguise messages on the internet. A Caesar cipher performs a shift of all of the characters in a string (based on their ASCII values, see Table 2.1), e.g. h e l l o → m j q q tThe example shows a shift with a distance of 5characters, i.e. h(ASCII:104) → m(ASCII:109)Write a C/C++ program that asks the user to input a line of plaintext and the distance value and outputs an encrypted text using a Caesar cipher, with the ASCII values range from 0 through 127. Use underscores (ASCII: 95) to represent space characters.Underscore characters should not be encrypted, and any character that is encrypted may not become an underscore. In this case, the character should be changed to the next character in the ASCII table.The program should work for any printable characters.NB: No strings (datatype) or library functions may be used.See Figure 2.1 for example output.arrow_forwardRead the input one line at a time and output the current line if and only if it is largerthan any other line read so far or strictly smaller than the previously outputted line. (Here,smaller is with respect to the usual order on Strings, as defined by String.compareTo()). you have to use arrylist and cant modify any code under public static void main(string[] args). example: input 138456792 output: 138492 import java.util.*; import java.io.BufferedReader; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; import java.io.InputStreamReader; import java.io.PrintWriter; import java.util.Stack; public class Part2 { /** * Your code goes here - see Part0 for an example * @param r the reader to read from * @param w the writer to write to * @throws IOException */ public static void doIt(BufferedReader r, PrintWriter w) throws IOException { /*ArrayList<String> arr = new ArrayList<>(); int count…arrow_forward
- A palindromic prime is a prime number and also palindromic. For example, 131 is a prime and also a palindromic prime, as are 313 and 757. Write a program that displays the first 120 palindromic prime numbers. Display 10 numbers per line, separated by exactly one space, as follows: 3 5 7 11 101 131 151 181 191 313 353 373 383 727 757 787 797 919 929arrow_forwardA palindrome is a number or a text phrase that reads the same backwardsas forwards. For example, each of the following five-digit integers is apalindrome: 12321, 55555, 45554 and 11611. Write a program that reads ina five-digit integer and determines whether it is a palindromearrow_forwardWrite a program that reads a word and prints whether it is short (fewer than 5 letters).it is long (at least 10 letters).it ends with the letter y.starts and ends with the same letter.words.cpp 12345678910111213141516171819202122232425262728293031323334#include <iostream>#include <iomanip>#include <string>using namespace std;int main(){ cout << "Enter a word: " << endl; string word; cin >> word; if (. . .) { . . . } if (. . .) { . . . } if (. . .) { . . . } if (. . .) { . . . } return 0;}CodeCheckResetarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
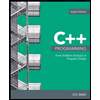