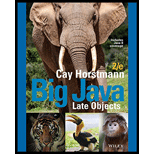
Big Java Late Objects
2nd Edition
ISBN: 9781119330455
Author: Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 16, Problem 15PP
Program Plan Intro
To implement a hash table with quadratic probing
Program plan:
- In a file “HashSet.java”, import necessary package, and create a class “HashSet”,
- Declare the array of “Object” type.
- Declare the necessary variable.
- Define the constructor to create a hash table.
- Create an array and set the current size to “0”.
- Define the method “toString()” that returns the string representation of array.
- Define the method “contains()”,
- Assign the hash code.
- Check the condition,
- If it is true, returns true.
- Create a loop,
-
- Compute the probe.
- Check the condition,
- If it is true, returns true.
- Check whether the bucket value contains null,
- If it is true, returns false.
- Returns false.
- If it is true, returns true.
- Define the method “getHashCode()”,
- Assign the hash code.
- Check whether the value is less than “0”,
- If it is true, assign the negative value.
- Update the hash value.
- Return the hash code.
- If it is true, assign the negative value.
- Define the method “add()”
- Check whether there is a room before probing,
- If it is true, call the method “resizeTable()()”.
- Assign the hash code returned from the method “getHashCode()”.
- Check whether the has value is a null,
-
- If it is true, assign the object properties.
- Otherwise, execute a loop,
-
- Compute the quadratic probe.
- Check whether the bucket contains null value,
- If it is true, assign the object properties.
- Use break statement.
- Otherwise, Check the condition,
- Returns false.
- Increment the current size.
- Returns true.
- If it is true, call the method “resizeTable()()”.
- Check whether there is a room before probing,
- Define the method “remove()” to remove the object from the set,
- Get the hash code.
- Set the position to “-1”.
- Check the condition,
- If it is true, assign the hash code to position.
- Create a loop,
-
- Compute the probe.
- Check the condition, set the new position.
- Check whether the position value is “-1”,
-
- If it is true, returns false.
- If the item found, find the last item in the rest of the probing sequence.
- Create a loop,
-
- Compute the probe.
- Check whether the current bucket value contains null,
- If it is true, use break.
- Otherwise, check the condition,
- If it is true, assign the current value to as last index.
- Check whether the last sequence is “-1”,
-
- If it is true, assign the null value and decrement the size.
- Otherwise, assign the last index value to the current position.
- Assign null value to last index.
- Create a loop to rehash the table,
-
- Check the condition,
- Continue to skip nulls.
- Assign the current bucket value to object.
- Assign null value to bucket.
- Decrement current size.
- Call the method “add()”.
- Check the condition,
- Returns true.
- If it is true, assign the hash code to position.
- Create a class “HashSetIterator”,
- Declare the variable to denote bucket index.
- Define the constructor that create a hash set iterator that points to the first element of the hash set.
- Define the method “hasNext ()”,
- Create a loop,
- Check the condition,
-
- Returns true.
- Returns false.
- Create a loop,
- Define the method “size()” to return the size.
- Define the method “next ()”,
- Execute the statement without checking the condition,
- Increment the index.
- Check the condition,
- If it is true, create an object for “NoSuchElementException”.
- Check whether the current bucket value contains null,
- Return the value.
- Execute the statement without checking the condition,
- Define the method “remove()”,
- Throw an exception “UnsupportedOperationException”.
- In a file “HashSetTest.java”, create a class “HashSetTest”,
- Define the method “main()”,
- Create an object for “HashSet”.
- Add the name “James” into the set.
- Add the name “David” into the set.
- Add the name “John” into the set.
- Add the name “Kean” into the set.
- Add the name “Juliet” into the set.
- Add the name “Lilly” into the set.
- Print the object properties.
- Print the expected output.
- Initialize the array.
- Iterate over the names,
- Print the actual and expected output.
- Remove the name “John” from the set.
- Print the set and expected output.
- Define the method “main()”,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
Big Java Late Objects
Ch. 16.1 - Prob. 1SCCh. 16.1 - Prob. 2SCCh. 16.1 - Prob. 3SCCh. 16.1 - Prob. 4SCCh. 16.1 - Prob. 5SCCh. 16.1 - Prob. 6SCCh. 16.1 - Prob. 7SCCh. 16.2 - Prob. 8SCCh. 16.2 - Prob. 9SCCh. 16.2 - Prob. 10SC
Ch. 16.2 - Prob. 11SCCh. 16.2 - Prob. 12SCCh. 16.3 - Prob. 13SCCh. 16.3 - Prob. 14SCCh. 16.3 - Prob. 15SCCh. 16.3 - Prob. 16SCCh. 16.3 - Prob. 17SCCh. 16.3 - Prob. 18SCCh. 16.4 - Prob. 19SCCh. 16.4 - Prob. 20SCCh. 16.4 - Prob. 21SCCh. 16.4 - Prob. 22SCCh. 16.4 - Prob. 23SCCh. 16.4 - Prob. 24SCCh. 16 - Prob. 1RECh. 16 - Prob. 2RECh. 16 - Prob. 3RECh. 16 - Prob. 4RECh. 16 - Prob. 5RECh. 16 - Prob. 6RECh. 16 - Prob. 7RECh. 16 - Prob. 8RECh. 16 - Prob. 9RECh. 16 - Prob. 10RECh. 16 - Prob. 11RECh. 16 - Prob. 12RECh. 16 - Prob. 13RECh. 16 - Prob. 14RECh. 16 - Prob. 15RECh. 16 - Prob. 16RECh. 16 - Prob. 17RECh. 16 - Prob. 18RECh. 16 - Prob. 19RECh. 16 - Prob. 20RECh. 16 - Prob. 21RECh. 16 - Prob. 22RECh. 16 - Prob. 23RECh. 16 - Prob. 24RECh. 16 - Prob. 25RECh. 16 - Prob. 26RECh. 16 - Prob. 1PECh. 16 - Prob. 2PECh. 16 - Prob. 3PECh. 16 - Prob. 4PECh. 16 - Prob. 5PECh. 16 - Prob. 6PECh. 16 - Prob. 7PECh. 16 - Prob. 8PECh. 16 - Prob. 9PECh. 16 - Prob. 10PECh. 16 - Prob. 11PECh. 16 - Prob. 12PECh. 16 - Prob. 13PECh. 16 - Prob. 14PECh. 16 - Prob. 15PECh. 16 - Prob. 16PECh. 16 - Prob. 17PECh. 16 - Prob. 18PECh. 16 - Prob. 19PECh. 16 - Prob. 20PECh. 16 - Prob. 21PECh. 16 - Prob. 1PPCh. 16 - Prob. 2PPCh. 16 - Prob. 3PPCh. 16 - Prob. 4PPCh. 16 - Prob. 5PPCh. 16 - Prob. 6PPCh. 16 - Prob. 7PPCh. 16 - Prob. 8PPCh. 16 - Prob. 9PPCh. 16 - Prob. 10PPCh. 16 - Prob. 11PPCh. 16 - Prob. 12PPCh. 16 - Prob. 13PPCh. 16 - Prob. 14PPCh. 16 - Prob. 15PPCh. 16 - Prob. 16PPCh. 16 - Prob. 17PP
Knowledge Booster
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
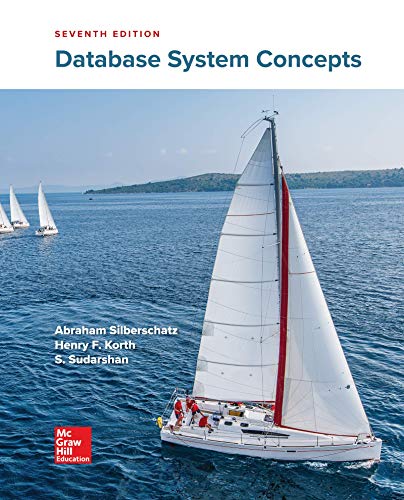
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
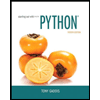
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
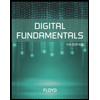
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
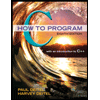
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
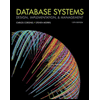
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
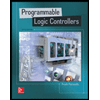
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education