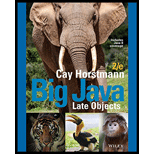
Big Java Late Objects
2nd Edition
ISBN: 9781119330455
Author: Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 16, Problem 9PE
Program Plan Intro
Simplified implementation of “ArrayList” class
Program plan:
- In a file “ArrayList.java”, import necessary packages and create a class “ArrayList” that implements “Iterable” interface,
- Declare the object variable.
- Declare the necessary variable.
- Define the constructor to create an empty array list.
- Define the method “size()” to return the array list size.
- Define the method “checkBounds()” that throws an exception if the index that has been checked is out of bounds.
- Define the method “get()” that returns an element at a given position.
- Define the method “set()” that locates the element at a given position.
- Define the method “remove()” that removes an element at a given location.
- Call the method “checkBounds()”.
- Assign the element.
- Create a loop,
- Assign the current element to the previous location.
- Decrement the size.
- Return the element.
- Define the method “add()” to insert the element next to the given position.
- Define the method “addHere()”,
- Call the method “add()”.
- Define the method “addLast()” that insert the element next to the end of the array list.
- Define the method “growBufferIfNecessary()” that grow the buffer the buffer if the current size is same as the capacity of the buffer.
- Define the method “iterator()” of type “ArrayListIterator”,
- Return the object of “ArrayListIterator”.
- Create a class “ArrayListIterator”,
- Declare the necessary variables.
- Define the constructor to create an iterator.
- Define the method “add()” that inserts an element before the position of the iterator.
- Define the method “hasNext()” that checks whether there is an element next to the iterator position.
- Define the method “hasPrevious()” that checks whether there is an element before the iterator position.
- Define the method “next()” that moves the iterator past the succeeding element.
- Define the method “nextIndex()”,
- Check whether the next position is greater than the current size,
- If it is true, returns the position.
-
- Returns the next position.
- Check whether the next position is greater than the current size,
- Define the method “previous()”,
- Check whether there is not a previous element,
- If it is true, throws an exception.
-
- Decrement the position.
- Returns the function call.
- Check whether there is not a previous element,
- Define the method “previousIndex()”,
- Check whether there is the previous position id greater than “0”,
- If it is true, returns the position.
-
- Returns the previous position.
- Check whether there is the previous position id greater than “0”,
- Define the method “remove()” that removes the last traversed element.
- Define the method “set()” that assign last traversed element to the different value.
- In a file “ListTest.java”, create a class “ListTest”,
- Define the “main()” method.
- Create an “ArrayList” object.
- Call the method “addLast()” to add the element “David”.
- Call the method “addLast()” to add the element “Harris”.
- Call the method “addLast()” to add the element “Roman”.
- Call the method “addLast()” to add the element “Tommy”.
- Create a loop,
- Print the actual output.
- Print new line.
- Print the expected output.
- Define the “main()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
Big Java Late Objects
Ch. 16.1 - Prob. 1SCCh. 16.1 - Prob. 2SCCh. 16.1 - Prob. 3SCCh. 16.1 - Prob. 4SCCh. 16.1 - Prob. 5SCCh. 16.1 - Prob. 6SCCh. 16.1 - Prob. 7SCCh. 16.2 - Prob. 8SCCh. 16.2 - Prob. 9SCCh. 16.2 - Prob. 10SC
Ch. 16.2 - Prob. 11SCCh. 16.2 - Prob. 12SCCh. 16.3 - Prob. 13SCCh. 16.3 - Prob. 14SCCh. 16.3 - Prob. 15SCCh. 16.3 - Prob. 16SCCh. 16.3 - Prob. 17SCCh. 16.3 - Prob. 18SCCh. 16.4 - Prob. 19SCCh. 16.4 - Prob. 20SCCh. 16.4 - Prob. 21SCCh. 16.4 - Prob. 22SCCh. 16.4 - Prob. 23SCCh. 16.4 - Prob. 24SCCh. 16 - Prob. 1RECh. 16 - Prob. 2RECh. 16 - Prob. 3RECh. 16 - Prob. 4RECh. 16 - Prob. 5RECh. 16 - Prob. 6RECh. 16 - Prob. 7RECh. 16 - Prob. 8RECh. 16 - Prob. 9RECh. 16 - Prob. 10RECh. 16 - Prob. 11RECh. 16 - Prob. 12RECh. 16 - Prob. 13RECh. 16 - Prob. 14RECh. 16 - Prob. 15RECh. 16 - Prob. 16RECh. 16 - Prob. 17RECh. 16 - Prob. 18RECh. 16 - Prob. 19RECh. 16 - Prob. 20RECh. 16 - Prob. 21RECh. 16 - Prob. 22RECh. 16 - Prob. 23RECh. 16 - Prob. 24RECh. 16 - Prob. 25RECh. 16 - Prob. 26RECh. 16 - Prob. 1PECh. 16 - Prob. 2PECh. 16 - Prob. 3PECh. 16 - Prob. 4PECh. 16 - Prob. 5PECh. 16 - Prob. 6PECh. 16 - Prob. 7PECh. 16 - Prob. 8PECh. 16 - Prob. 9PECh. 16 - Prob. 10PECh. 16 - Prob. 11PECh. 16 - Prob. 12PECh. 16 - Prob. 13PECh. 16 - Prob. 14PECh. 16 - Prob. 15PECh. 16 - Prob. 16PECh. 16 - Prob. 17PECh. 16 - Prob. 18PECh. 16 - Prob. 19PECh. 16 - Prob. 20PECh. 16 - Prob. 21PECh. 16 - Prob. 1PPCh. 16 - Prob. 2PPCh. 16 - Prob. 3PPCh. 16 - Prob. 4PPCh. 16 - Prob. 5PPCh. 16 - Prob. 6PPCh. 16 - Prob. 7PPCh. 16 - Prob. 8PPCh. 16 - Prob. 9PPCh. 16 - Prob. 10PPCh. 16 - Prob. 11PPCh. 16 - Prob. 12PPCh. 16 - Prob. 13PPCh. 16 - Prob. 14PPCh. 16 - Prob. 15PPCh. 16 - Prob. 16PPCh. 16 - Prob. 17PP
Knowledge Booster
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
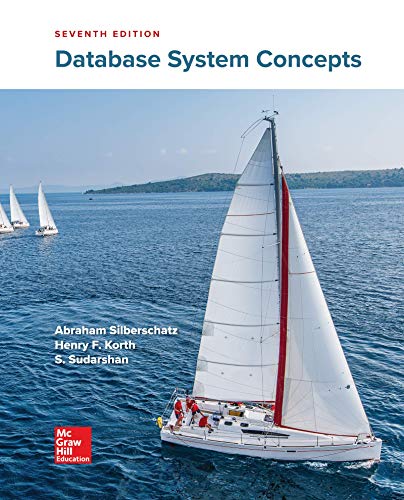
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
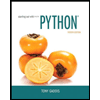
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
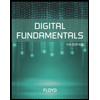
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
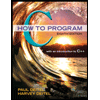
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
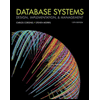
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
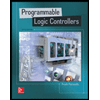
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education