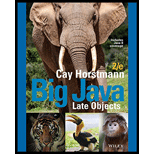
Big Java Late Objects
2nd Edition
ISBN: 9781119330455
Author: Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 16, Problem 21PE
Program Plan Intro
Implementation of hash set
Program plan:
- In a file “HashSet.java”, import necessary packages, and create a class “HashSet”,
- Declare the array of “Node” type.
- Declare the necessary variables.
- Define the constructor to create a hash table.
- Create an array and set the current size to “0”.
- Compute the value for “p”.
- Execute a loop,
- Increment the value of “p”.
- Set the value for “a”, and “b”.
- Define the method “isPrime()” to test whether the value passed is prime or not.,
- Define the method “contains()”,
- Assign the hash code.
- Check whether the value is less than “0”,
- If it is true, compute the absolute value of hash code.
- Update the hash value.
- Assign the bucket value to the current node.
- Execute loop till array becomes null,
- Check the condition,
- Return true.
-
- Assign the current value.
- Check the condition,
- Returns false.
- Define the method “add()” to add the element to the set,
- Get the hash code.
- Check whether the value is less than “0”,
- If it is true, assign the negative value.
- Update the hash code.
- Assign the bucket value at the hash code to the current node.
- Check whether the current node is not null value,
- If it is true, set the collision value to “1”
- Execute a loop,
- Check the condition,
- Returns “0”.
-
- Assign the next value to the current node.
- Check the condition,
- Create a new node.
- Assign the value to new node value.
- Assign the value to the next node.
- Assign the new node value to the bucket.
- Increment the size.
- Return collision value.
- Define the method “remove()” to remove the object from the set,
- Get the hash code.
- Check whether the value is less than “0”,
- If it is true, assign the negative value.
- Update the hash code.
- Assign the hash code value to the current node.
- Set null to the previous node.
- Execute a loop,
- Check the condition,
- Check whether the previous node contains null value,
-
- If it is true, assign the next node value to the bucket.
- Otherwise,
-
- Assign the next of the current node value to the next of the previous node value.
- Decrement the size.
- Return true.
-
-
- Assign the current node value to the previous node value.
- Assign the next of the current node value to the current node.
-
- Check the condition,
- Return false.
- Define the method “iterator ()” to return an iterator that traverse the set elements,
- Return the object of “HashSetIterator”.
- Define the method “size ()” to return the size of the set.
- Create a class “Node”,
- Declare the object for “Object”.
- Declare the object of “Node”.
- Create a class “HashSetIterator” that implements an interface “Iterator”,
- Declare the necessary variables.
- Define the constructor to create a hash set that point to the first element of the hash set.
- Define the method “hasNext()”,
- Check whether the current node and the next of the current node is not null,
- If it is true, returns true.
-
-
- Execute a loop,
- Check whether the bucket contains not null value,
- If it is true, returns true.
- Check whether the bucket contains not null value,
- Returns false.
- Execute a loop,
-
- Check whether the current node and the next of the current node is not null,
- Define the method “next()”,
- Check the condition,
- Assign the next node value to the current node value.
- Otherwise,
- Execute the following statement,
- Increment the index.
- Check the condition,
- Throws an exception “NoSuchElementException”.
- Assign the value.
- Check the condition at the end of the loop.
- Execute the following statement,
- Return the current node value.
- Check the condition,
- Define the method “remove()”,
- Throw an exception “UnsupportedOperationException”.
- In a file “HashSetFromBook.java”, import necessary packages, and create a class “HashSetFromBook”,
- Declare the array of “Node” type.
- Declare the necessary variables.
- Define the constructor to create a hash table.
- Create an array and set the current size to “0”.
- Define the method “isPrime()” to test whether the value passed is prime or not.,
- Define the method “contains()”,
- Assign the hash code.
- Check whether the value is less than “0”,
- If it is true, assign the negative value.
- Update the hash value.
- Assign the bucket value to the current node.
- Execute loop till array becomes null,
- Check the condition,
- Return true.
-
-
- Assign the current value.
-
- Check the condition,
- Returns false.
- Define the method “add()” to add the element to the set,
- Declare and initialize the variable.
- Get the hash code.
- Check whether the value is less than “0”,
- If it is true, compute the absolute value of hash code.
- Update the hash code.
- Assign the bucket value at the hash code to the current node.
- Check whether the current node is not null value,
- If it is true, set the collision value to “1”
- Execute a loop,
- Check the condition whether the element is already in the set,
- Returns “0”.
-
-
- Assign the next value to the current node.
-
- Check the condition whether the element is already in the set,
- Create a new node.
- Assign the value to new node value.
- Assign the value to the next node.
- Assign the new node value to the bucket.
- Increment the size.
- Return collision value.
- Define the method “remove()” to remove the object from the set,
- Get the hash code.
- Check whether the value is less than “0”,
- If it is true, assign the negative value.
- Update the hash code.
- Assign the hash code value to the current node.
- Set null to the previous node.
- Execute a loop,
- Check the condition,
- Check whether the previous node contains null value,
-
- If it is true, assign the next node value to the bucket.
-
- Otherwise,
- Assign the next of the current node value to the next of the previous node value.
- Decrement the size.
- Return true.
- Otherwise,
- Assign the current node value to the previous node value.
- Assign the next of the current node value to the current node.
-
- If it is true, assign the next node value to the bucket.
- Check the condition,
- Return false.
- Define the method “iterator ()” to return an iterator that traverse the set elements,
- Return the object of “HashSetIterator”.
- Define the method “size ()” to return the size of the set.
- Create a class “Node”,
- Declare the object for “Object”.
- Declare the object of “Node”.
- Create a class “HashSetIterator” that implements an interface “Iterator”,
- Declare the necessary variables.
- Define the constructor to create a hash set that point to the first element of the hash set.
- Define the method “hasNext()”,
- Check whether the current node and the next of the current node is not null,
- If it is true, returns true.
-
-
- Execute a loop,
- Check whether the bucket contains not null value,
- If it is true, returns true.
- Check whether the bucket contains not null value,
- Returns false.
- Execute a loop,
-
- Check whether the current node and the next of the current node is not null,
- Define the method “next()”,
- Check the condition,
- Assign the next node value to the current node value.
- Otherwise,
- Execute the following statement,
- Increment the index.
- Check the condition,
- Throws an exception “NoSuchElementException”.
- Assign the value.
- Check the condition at the end of the loop.
- Execute the following statement,
- Return the current node value.
- Check the condition,
- Define the method “remove()”,
- Throw an exception “UnsupportedOperationException”.
- In a file “HashSetTest.java”, import necessary packages, and create a class “HashSetTest”,
- Define the “main()” method,
- Declare and initialize the necessary variables.
- Create an object for “HashSet”.
- Create an object for “HashSetFromBook”.
- Execute “try” statement,
- Execute a loop,
- Get the string.
- Update the necessary values.
- Execute a loop,
- Catch if the exception occurs,
- Print the message.
- Print the output.
- Define the “main()” method,
Explanation of Solution
“Yes”, the multiply-add-divide (MAD) method decreases the collisions.
Explanation:
- The hash function is defined as follows,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
Big Java Late Objects
Ch. 16.1 - Prob. 1SCCh. 16.1 - Prob. 2SCCh. 16.1 - Prob. 3SCCh. 16.1 - Prob. 4SCCh. 16.1 - Prob. 5SCCh. 16.1 - Prob. 6SCCh. 16.1 - Prob. 7SCCh. 16.2 - Prob. 8SCCh. 16.2 - Prob. 9SCCh. 16.2 - Prob. 10SC
Ch. 16.2 - Prob. 11SCCh. 16.2 - Prob. 12SCCh. 16.3 - Prob. 13SCCh. 16.3 - Prob. 14SCCh. 16.3 - Prob. 15SCCh. 16.3 - Prob. 16SCCh. 16.3 - Prob. 17SCCh. 16.3 - Prob. 18SCCh. 16.4 - Prob. 19SCCh. 16.4 - Prob. 20SCCh. 16.4 - Prob. 21SCCh. 16.4 - Prob. 22SCCh. 16.4 - Prob. 23SCCh. 16.4 - Prob. 24SCCh. 16 - Prob. 1RECh. 16 - Prob. 2RECh. 16 - Prob. 3RECh. 16 - Prob. 4RECh. 16 - Prob. 5RECh. 16 - Prob. 6RECh. 16 - Prob. 7RECh. 16 - Prob. 8RECh. 16 - Prob. 9RECh. 16 - Prob. 10RECh. 16 - Prob. 11RECh. 16 - Prob. 12RECh. 16 - Prob. 13RECh. 16 - Prob. 14RECh. 16 - Prob. 15RECh. 16 - Prob. 16RECh. 16 - Prob. 17RECh. 16 - Prob. 18RECh. 16 - Prob. 19RECh. 16 - Prob. 20RECh. 16 - Prob. 21RECh. 16 - Prob. 22RECh. 16 - Prob. 23RECh. 16 - Prob. 24RECh. 16 - Prob. 25RECh. 16 - Prob. 26RECh. 16 - Prob. 1PECh. 16 - Prob. 2PECh. 16 - Prob. 3PECh. 16 - Prob. 4PECh. 16 - Prob. 5PECh. 16 - Prob. 6PECh. 16 - Prob. 7PECh. 16 - Prob. 8PECh. 16 - Prob. 9PECh. 16 - Prob. 10PECh. 16 - Prob. 11PECh. 16 - Prob. 12PECh. 16 - Prob. 13PECh. 16 - Prob. 14PECh. 16 - Prob. 15PECh. 16 - Prob. 16PECh. 16 - Prob. 17PECh. 16 - Prob. 18PECh. 16 - Prob. 19PECh. 16 - Prob. 20PECh. 16 - Prob. 21PECh. 16 - Prob. 1PPCh. 16 - Prob. 2PPCh. 16 - Prob. 3PPCh. 16 - Prob. 4PPCh. 16 - Prob. 5PPCh. 16 - Prob. 6PPCh. 16 - Prob. 7PPCh. 16 - Prob. 8PPCh. 16 - Prob. 9PPCh. 16 - Prob. 10PPCh. 16 - Prob. 11PPCh. 16 - Prob. 12PPCh. 16 - Prob. 13PPCh. 16 - Prob. 14PPCh. 16 - Prob. 15PPCh. 16 - Prob. 16PPCh. 16 - Prob. 17PP
Knowledge Booster
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
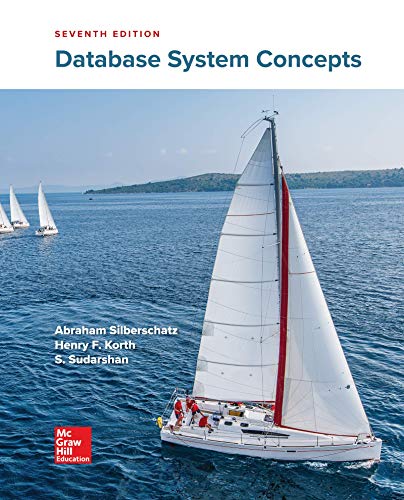
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
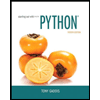
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
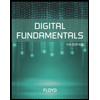
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
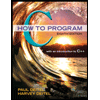
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
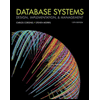
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
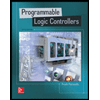
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education