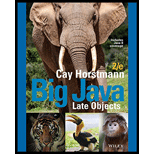
Big Java Late Objects
2nd Edition
ISBN: 9781119330455
Author: Horstmann
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Question
Chapter 16, Problem 4PE
Program Plan Intro
A method “size()”
Program plan:
- In a file “ListIterator.java”, create an interface “ListIterator”,
- Declare the method “next()” that moves the iterator past the next element.
- Declare the method “hasNext()” that check if there is an element after the iterator position.
- Declare the method “add()” that adds an element before the position of the iterator and moves the iterator past the added element.
- Declare the method “remove()” that removes the last traversed element.
- Declare the method “set()” to set the last traversed element to a previous value.
- In a file “LinkedList.java”, import the package and create a class “LinkedList”,
- Declare the object variable.
- Define the constructor to create an empty linked list.
- Define the method “size()” to compute the linked list size,
- Initialize the variable that holds size.
- Assign the first reference to the current node.
- Execute a loop,
- Increment the size.
- Assign the next of the current node to the current node.
- Return the linked list size.
-
- Define the method “getFirst()” that returns the first element in the linked list.
- Define the method “removeFirst()” to remove the first element in the linked list.
- Define the method “addFirst()” that adds an element to the front of the linked list,
-
- Create a new node.
- Assign the value to the new node.
- Assign the first element to the next pointer of new node.
- Assign new node to the first position.
- Define the method “listIterator()” of type “ListIterator”, that returns an iterator for iterating through the list.
- Create a class “Node”,
-
- Declare the object variable for “Object”, and “Node”.
- Create a class “LinkedListIterator”,
-
- Declare the necessary object variables.
- Define the constructor to create an iterator that points to the front of the linked list.
- Define the method “next()”,
- If there is no next element then throws an exception.
- Assign the position to the front of the linked list.
- Check if position is null, set the first element to the position.
- Otherwise, set the next position to the current position.
- Returns the value.
- Define the method “hasNext()” that check whether there is next element,
- Check if position is null,
- Returns true if first reference is not null.
- Otherwise,
- Returns true, if the next reference of the position is not null.
- Check if position is null,
- Define the method “add()”,
- If position is null, call the method “addFirst()”.
- Set the first to the position.
- Otherwise, create a new node.
- Assign the element to the new node.
- Set the next position to the next pointer of the new node.
- Set the new node value to the next position.
- Set the new node as the position.
- Set the Boolean value as false.
- If position is null, call the method “addFirst()”.
- Define the method “remove()”,
- Check if there is no next element, throws an exception.
- If position is same as the first, calls the method “removeFirst()”.
- Otherwise, set the next position to the previous position.
- Set the previous to the positions.
- St the Boolean value to false.
- Define the method “set()”,
- If there is no next element, throw an exception.
- Set the value to the position.
- Define the method “hasNext()” that check whether there is next element,
-
- In a file “SizeTest.java”, create a class “SizeTest”,
- Define the “main()” method.
- Create the “LinkedList” object.
- Call the method “addFirst()” to add “Tommy” to the front of the linked list.
- Call the method “addFirst()” to add “Roma” to the font of the linked list.
- Call the method “addFirst()” to add “Harris” to the font of the linked list.
- Call the method “addFirst()” to add “David” to the font of the linked list.
- Assign the value returned from the method “listIterator()” calling by LinkedList object.
- Call the method “next()”.
- Call the method “next()”.
- Call the method “remove()”.
- Call the method “next()”.
- Call the method “remove()”.
- Call the method “add()” to add “John” to the linked list.
- Call the method “next()”.
- Call the method “set()”.
- Call the method “removeFirst()”.
- Assign the value returned from the method “listIterator()”.
- Execute a loop,
- Print the actual output.
-
-
-
- Print the new line.
- Print the expected output.
- Print the actual size of the linked list.
- Print the expected size of the linked list.
-
-
-
- Print the actual output.
- Define the “main()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Chapter 16 Solutions
Big Java Late Objects
Ch. 16.1 - Prob. 1SCCh. 16.1 - Prob. 2SCCh. 16.1 - Prob. 3SCCh. 16.1 - Prob. 4SCCh. 16.1 - Prob. 5SCCh. 16.1 - Prob. 6SCCh. 16.1 - Prob. 7SCCh. 16.2 - Prob. 8SCCh. 16.2 - Prob. 9SCCh. 16.2 - Prob. 10SC
Ch. 16.2 - Prob. 11SCCh. 16.2 - Prob. 12SCCh. 16.3 - Prob. 13SCCh. 16.3 - Prob. 14SCCh. 16.3 - Prob. 15SCCh. 16.3 - Prob. 16SCCh. 16.3 - Prob. 17SCCh. 16.3 - Prob. 18SCCh. 16.4 - Prob. 19SCCh. 16.4 - Prob. 20SCCh. 16.4 - Prob. 21SCCh. 16.4 - Prob. 22SCCh. 16.4 - Prob. 23SCCh. 16.4 - Prob. 24SCCh. 16 - Prob. 1RECh. 16 - Prob. 2RECh. 16 - Prob. 3RECh. 16 - Prob. 4RECh. 16 - Prob. 5RECh. 16 - Prob. 6RECh. 16 - Prob. 7RECh. 16 - Prob. 8RECh. 16 - Prob. 9RECh. 16 - Prob. 10RECh. 16 - Prob. 11RECh. 16 - Prob. 12RECh. 16 - Prob. 13RECh. 16 - Prob. 14RECh. 16 - Prob. 15RECh. 16 - Prob. 16RECh. 16 - Prob. 17RECh. 16 - Prob. 18RECh. 16 - Prob. 19RECh. 16 - Prob. 20RECh. 16 - Prob. 21RECh. 16 - Prob. 22RECh. 16 - Prob. 23RECh. 16 - Prob. 24RECh. 16 - Prob. 25RECh. 16 - Prob. 26RECh. 16 - Prob. 1PECh. 16 - Prob. 2PECh. 16 - Prob. 3PECh. 16 - Prob. 4PECh. 16 - Prob. 5PECh. 16 - Prob. 6PECh. 16 - Prob. 7PECh. 16 - Prob. 8PECh. 16 - Prob. 9PECh. 16 - Prob. 10PECh. 16 - Prob. 11PECh. 16 - Prob. 12PECh. 16 - Prob. 13PECh. 16 - Prob. 14PECh. 16 - Prob. 15PECh. 16 - Prob. 16PECh. 16 - Prob. 17PECh. 16 - Prob. 18PECh. 16 - Prob. 19PECh. 16 - Prob. 20PECh. 16 - Prob. 21PECh. 16 - Prob. 1PPCh. 16 - Prob. 2PPCh. 16 - Prob. 3PPCh. 16 - Prob. 4PPCh. 16 - Prob. 5PPCh. 16 - Prob. 6PPCh. 16 - Prob. 7PPCh. 16 - Prob. 8PPCh. 16 - Prob. 9PPCh. 16 - Prob. 10PPCh. 16 - Prob. 11PPCh. 16 - Prob. 12PPCh. 16 - Prob. 13PPCh. 16 - Prob. 14PPCh. 16 - Prob. 15PPCh. 16 - Prob. 16PPCh. 16 - Prob. 17PP
Knowledge Booster
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
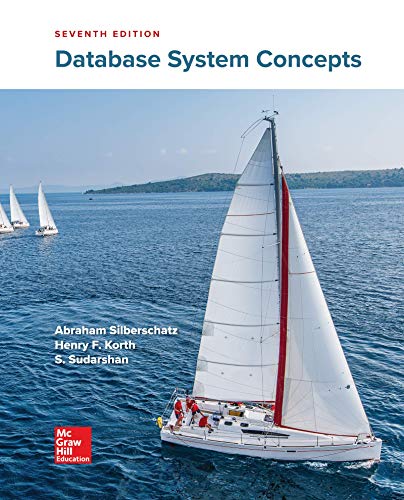
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
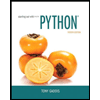
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
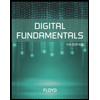
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
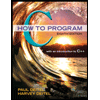
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
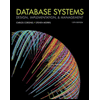
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
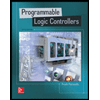
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education