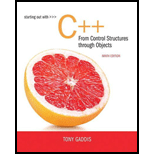
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 8PC
Program Plan Intro
Modification of “SimpleVector” class:
Program plan:
SimpleVector.h:
- Include the required header files in the program.
- Define the class “SimpleVector”:
- Declare the required class data members and exception function under “private” access specifier.
- Declare the required class member functions and class constructor under “public” access specifier.
- Define the “SimpleVector” function:
- Get the array size and allocate the memory for corresponding array size
- If allocation gets fail, then call the “memError()” function.
- Else initialize the array elements.
- Define the copy constructor of the “SimpleVector” function:
- Copy the array size and allocate the memory for corresponding array size
- If allocation gets fail, then call the “memError()” function.
- Else copy the array elements.
- Define the “~SimpleVector” class destructor:
- Delete the allocated memory for the array.
- Define the “getElement” function:
- Get the array subscript and check it is in the array range.
- If not then call the exception function.
- Return the subscript.
- Define the operator overload for “[]” operator
- Get the array subscript and check it is in the array range.
- If not then call the exception function.
- Return the subscript.
- Define the “push_back()” function:
- Get the element which is going to insert.
- Create a new array and allocate the memory for the array which is greater than 1 from an old array.
- Copy the elements from old array to new array.
- Insert the new element at the last of the array.
- Delete the old array and make the pointer points the new array
- Finally adjust the array size.
- Define the “pop_back()” function:
- Save the last element which is going to delete.
- Create a new array and allocate the memory for the array which is lesser than 1 from an old array.
- Copy the elements from old array to new array.
- Delete the old array and make the pointer points the new array
- Finally adjust the array size.
- Return the deleted value.
Main.cpp:
- Include the required header files in the program.
- Create an object for the class “SimpleVector” for integer type.
- Create an object for the class “SimpleVector” for double type.
- Use for loop to iterate array elements
- Insert the integer value on integer array.
- Insert the double value on double array.
- Using for loop, display an integer array on the output screen.
- Using for loop, display the double array on the output screen.
- Insert a new integer element by calling the “push_back()” function.
- Insert a new double element by calling the “push_back()” function.
- Using for loop, display an integer array on the output screen.
- Using for loop, display the double array on the output screen.
- construct the “try” block
- Call the “pop_back()” function for deleting the last integer element from the array.
- Call the “pop_back()” function for deleting the last double element from the array.
- Construct the “catch” block
- Display the exception message on the output screen.
- Using for loop, display an integer array on the output screen.
- Using for loop, display the double array on the output screen.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
please just help with parts afterr //
3) Write friend function for the following class to overload the << and >> operators. The friend function, input operator (operator >>), should read 5 elements into the array attribute called “grade” for the objet and output (operator <<) should print the content of the array “grade” of the object on the screen.
class Math
{ // put the prototype of the friend functions here
public:
intgrade[5]:
math( ) {for (int i=0, i<10, i++) grade[i] = 0;};
// implement the overloaded input operator function
// implement the overloaded output operator function
void main ()
{
Math ObjA;
//your friend function should be able to take care of the following code
cin >> ObjA;
cout << ObjA;
Please just use main.cpp,sequence.cpp and sequence.h. It has provided the sample insert()function.
No documentation (commenting) is required for this assignment.
This assignment is based on an assignment from "Data Structures and Other Objects Using C++" by Michael Main and Walter Savitch.
Use linked lists to implement a Sequence class that stores int values.
Specification
The specification of the class is below. There are 9 member functions (not counting the big 3) and 2 types to define. The idea behind this class is that there will be an internal iterator, i.e., an iterator that the client cannot access, but that the class itself manages. For example, if we have a Sequence object named s, we would use s.start() to set the iterator to the beginning of the list, and s.advance() to move the iterator to the next node in the list.
(I'm making the analogy to iterators as a way to help you understand the point of what we are doing. If trying to think in terms of iterators is confusing,…
(Polynomial Class) Develop class Polynomial. The internal representation of a Polynomialis an array of terms. Each term contains a coefficient and an exponent, e.g., the term2x4has the coefficient 2 and the exponent 4. Develop a complete class containing proper constructorand destructor functions as well as set and get functions. The class should also provide the followingoverloaded operator capabilities:a) Overload the addition operator (+) to add two Polynomials.b) Overload the subtraction operator (-) to subtract two Polynomials.c) Overload the assignment operator to assign one Polynomial to another.d) Overload the multiplication operator (*) to multiply two Polynomials.e) Overload the addition assignment operator (+=), subtraction assignment operator (-=),and multiplication assignment operator (*=).
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - Prob. 13RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 21RQECh. 16 - Prob. 22RQECh. 16 - Prob. 23RQECh. 16 - Prob. 24RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 26RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 34RQECh. 16 - Prob. 35RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 39RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 8PCCh. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- //3. toggleCommentSection//a. Receives a postId as the parameter//b. Selects the section element with the data-post-id attribute equal to the postId//received as a parameter//c. Use code to verify the section exists before attempting to access the classList//property//d. At this point in your code, the section will not exist. You can create one to test if//desired.//e. Toggles the class 'hide' on the section element//f. Return the section element function code so far passes a.b,c fails rest function toggleCommentSection(postId) { // If Post Id Is Passed, Return Undefined if (!postId) { return undefined; } else { // Else, Get All Comment Sections const commentSections = document.querySelectorAll('[data-post-id]'); // Loop Through Each Comment Section for (let i = 0; i < commentSections.length; i++) { const commentSection = commentSections[i]; // If Post Id Attribut Of Comment Section Is Equal To Post Id Passed Arg…arrow_forwardC++ Programming D.S.Malik12-10: Add the function max as an abstract function to the class arrayListType to return the largest element of the list. Also, write the definition of the function max in the class unorderedArrayListType and write a program to test this function.arrow_forwardPART 2: Pets Examine carefully the UML class diagram below: NOTE: Class Reptile was missing a toString() method. It has now been added. class Pet has an attribute of type java.util.Date. no specific dates are required for this attribute. compareTo(Dog) compares Dogs by weight. Create executable class TestPet as follows: create at least one Reptile pet and display it create an array of at least four Dog pets sort the array of Dogs by weight use a foreach loop to fully display all data for all dogs sorted by weight (see sample output)arrow_forward
- 3) Write friend function for the following class to overload the << and >> operators. The friend function, input operator (operator >>), should read 5 elements into the array attribute called “grade” for the objet and output (operator <<) should print the content of the array “grade” of the object on the screen. class Math { // put the prototype of the friend functions here public: intgrade[5]: math( ) {for (int i=0, i<10, i++) grade[i] = 0;}; // implement the overloaded input operator function // implement the overloaded output operator function void main () { Math ObjA; //your friend function should be able to take care of the following code cin >> ObjA; cout << ObjA;arrow_forwardClass student contains roll number, name and course as data members and Input_studentand display_student as member functions. Create a class exam and publicly inherit it fromstudent class. The derived class contains an array of marks and no_of_subjects as datamembers, and input_marks and display_result as member functions. Overload “<=”, “()” and“+=” operators and use the overloaded operators in the main function. Create an array ofobjects of the exam class and display the result of 5 studentarrow_forwardSubject Object oriented programming DO task in C++ Start with the safearay class from the ARROVER3 program in Chapter 8 of Object Orient programming by Rober Lafore. Make this class into a template, so the safe array can store any kind of data. Include following member functions in Safe array class. The minimum function finds the minimum value in array. The maximum function find the maximum value in array. The average function find average of all the elements of an array. The total function finds the running total of all elements of an array. In main(), create safe arrays of at least two different types int and double and store some data in them. Then display minimum, maximum, average and total of array elements. Note: use subscript ([]) operator in sasfearay class.arrow_forward
- Tic-Tac-Toe) Write a program that allows two players to play the tic-tac-toe game. Your program must contain the class ticTacToe to implement a ticTacToe object. Include a 3-by-3 two-dimensional array, as a private member variable, to create the board. If needed, include additional member variables. Some of the operations on a ticTacToe object are printing the current board, getting a move, checking if a move is valid, and determining the winner after each move. Add additional operations as needed. (programming language C#)arrow_forward(Tic-Tac-Toe) Write a program that allows two players to play the tic-tac-toe game. Your program must contain the class ticTacToe to implement a ticTacToe object. Include a 3-by-3 two-dimensional array, as a private member variable, to create the board. If needed, include additional member variables. Some of the operations on a ticTacToe object are printing the current board, getting a move, checking if a move is valid, and determining the winner after each move. Add additional operations as needed.arrow_forwardPointer and classImplement a class called Team as specified:data members:name - the name of the Team (defined as a dynamic variable)members - a dynamic array of stringsize - number of members in the team.functions:Course(): default constructor set name to “TBD”, and size to 0, members toempty listCourse(string): one argument constructor set name, and size to 0, members toempty listaccessor - an accessor for the name and size variablemutator - an mutator for the name variableUse following main() to test your class.int main() {Team a,b("Mets");cout<<a.getName()<<endl; // print TBDa.setName("Yankee");cout<<a.getName()<<endl; // print Yankeecout<<b.getName()<<endl; // print Metscout<<a.getSize()<<endl; // print 0return 0;}arrow_forward
- PROBLEM - Design a template class “myArray” to represent an array. This class should allow the user to define the start and end index that can be positive OR negative. The constructor should calculate the size and dynamically allocate the required memory. First index must be less than the second index. • Define the class Example: myArray object1(2,9) Creates an array of type int and size 8. myArray object2(-2,4) Creates an array of type int and size 7 • Define the class .• Define the constructor. The default values of start and end index should be 0 and 5 • Define a member function TakeInput that asks the user to input values in this array from console • Define a member function DisplayAtIndex that takes a single parameter x for index. It first check whether x is in bound or not, and prints the value at that index. Example code myArray obj1(-5, 0); obj1.TakeInput(); obj1.DisplayAtIndex(7); obj1.DisplayAtIndex(-1); Output: Object created with indices -5 -4, -3, -2, -1, 0 Values entered…arrow_forwardEach member-function definition outside its corresponding class template definitionmust begin with template and the same template parameters as its class template T/Farrow_forwardC++ (Tic-Tac-Toe) Write a program that allows two players to play the tic- tac-toe game. Your program must contain the class ticTacToe to implement a ticTacToe object. Include a 3-by-3 two-dimensional array, as a private member variable, to create the board. If needed, include additional member variables. Some of the operations on a ticTacToe object are printing the current board, getting a move, checking if a move is valid, and determining the winner after each move. Add additional operations as needed.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
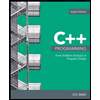
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning