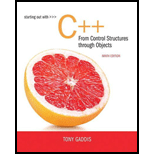
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 13PC
Program Plan Intro
Employee and ProductionWorker class
Program plan:
source.cpp:
- Include the required header files to the program.
- Declare the required function prototype.
- Define the “main()” function.
- Create the pointer and call the “create_Production_Worker” function with employee data.
- If the pointer is not null, then Call the function “display()” to display the employee details.
- Function definition for function “create_Production_Worker”.
- Create an object for “ProductionWorker” class and pass the employee data.
- Construct catch blocks for handling “InvalidEmployeeNumber”, “InvalidShift” and “InvalidPayRate”.
- Function definition for function “display()”.
- Display the name, number, hire date, shift name, shift number and pay rate using the respective function.
Employee.h:
- Define the “Employee.h”.
- Define an empty “InvalidEmployeeNumber” exception class.
- In private, declare the required variable.
- In public, create the constructor and return the name, number and hire date.
- Create a mutator function for name, number and hire date.
- Create an accessor function for name, number and hire date.
ProductionWorker.h:
- Define the “ProductionWorkder.h”.
- Define an empty “InvalidShift” exception class.
- Define an empty “InvalidPayRate” exception class.
- Create a “ProductionWorker” which is derived from “Employee” class.
- In private, declare the required variable.
- In public, create a default constructor which initializes the “shift” and “rate” as “0” and “0.0” respectively.
- Invoke the constructor “productionWorker” which also calls the “Employee” constructor.
- Assign the “shift” and “rate” as “empShift” and “empPayRate” respectively.
- Create a mutator function for shift name, rate and shift number.
- Create an accessor function for shift name, rate and shift number.
- Create a bool() function to check the employee shift number and pay rate. If it is not valid, then call its relevant exception.
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
Define an exception class called CoreBreachException. The class should have a default constructor. If an exception is thrown using this zero- argument constructor, getMessage should return "Core Breach! Evacuate Ship!" The class should also define a constructor having a single parameter of type String. If an exception is thrown using this constructor, getMessage should return the value that was used as an argument to the constructor.
Define an exception class called CoreBreachException. The class should have a default
constructor. If an exception is thrown using zero-argument constructor, getMessage should
return "Cor Breach Evacuation Ship!" The class should also define a constructor having a single
parameter of type String. If an exception is thrown using constructor, getMessage should return
the value that was used as an argument to constructor.
The following class maintains an account balance and returns aspecial error code.public class Account{private double balance;// returns new balance or -1 if errorpublic double deposit(double amount){if (amount > 0)balance += amount;elsereturn -1; // Code indicating errorreturn balance;}}Rewrite the class so that it throws appropriate exception instead ofreturning -1 as an error code. Write test code that attempts to depositinvalid amounts and catches the exceptions that are thrown
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - Prob. 13RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 21RQECh. 16 - Prob. 22RQECh. 16 - Prob. 23RQECh. 16 - Prob. 24RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 26RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 34RQECh. 16 - Prob. 35RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 39RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 8PCCh. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Create a tornadoException exception class. The class should have two constructors, one of which should be the default function Object() { [native code] }. If the default function Object() { [native code] } throws an error, the function should return "Tornado: Take cover immediately!" The other function Object() { [native code] } takes a single int argument, say m. If this function Object() { [native code] } throws an error, the function should return "Tornado: m miles distant; and approaching!"arrow_forwardModify the attached code: Create a new class called CalculatorWithMod. This class should be a sub class of the base class Calculator. This class should also have an additional method for calculating the modulo. The modulo method should only be seen at the sub class, and not the base class! Include exception handling for instances when dividing by 0 or calculating the modulo with 0. You would need to use throw, try, and catch. The modulo (or "modulus" or "mod") is the remainder after dividing one number by another.Example: 20 mod 3 equals 2Because 20/3 = 6 with a remainder of 2 Refer also to the attached sample outputs:arrow_forwardC++ Visual Studio 2019 Complete #13. Dependent #1 Employee and ProductionWorker classes showing below. Modify the Employee and ProductionWorker classes so they throw exceptions when the following errors occur: The Employee class should throw an exception named InvalidEmployeeNumber when it receives an employee number that is less than 0 or greater than 9999. The ProductionWorker class should throw an exception named InvalidShift when it receives an invalid shift. The ProductionWorker class should throw an exception named InvalidPayRate when it receives a negative number for the hourly pay rate. Write a driver program that demonstrates how each of these exception conditions works. #1 Employee and ProductionWorker classes #include <string>#include <iostream>#include <iomanip>using namespace std; class Employee{private: string name; // Employee name string number; // Employee number string hireDate; // Hire date public: // Default…arrow_forward
- Java Problem:Create a Class named Bishop. Make sure the class cannot be inherited. If there have already been 5 objects initiated for this class, trying to initiate the 6th object, the constructor will throw a user-defined exception BishopCreationLimitExceeded. This class has an instance method named printObjectNumber which will print the object creation sequence number for the object for which you are calling the method as follows: This Bishop Object number is 3. Here, 3 is the object creation sequence number that is the third call to new Bishop() in your code that initiated the object. The BishopCreationLimitExceeded class sets the exception message (using super call in the constructor) as follows: The maximum number of bishop objects can be 5. You can define instance variables in the Bishop class if you need them.arrow_forwardWrite a Month class that holds information about the month. Write exception classes for the following error conditions:• A number less than 1 or greater than 12 is given for the month number.• An invalid string is given for the name of the month.Modify the Month class so that it throws the appropriate exception when either of these errors occurs. Demonstrate the classes in a program.arrow_forwardInstructions: Exception handing Add exception handling to this game (try, catch, throw). You are expected to use the standard exception classes to try functions, throw objects, and catch those objects when problems occur. At a minimum, you should have your code throw an invalid_argument object when data from the user is wrong. File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File…arrow_forward
- Develop an exception class named InvalidMonthException that extends the Exception class. Instances of the class will be thrown based on the following conditions: The value for a month number is not between 1 and 12 The value for a month name is not January, February, March, … December Develop a class named Month. The class should define an integer field named monthNumber that holds the number of a month. For example, January would be 1, February would be 2, and so forth. In addition, provide the following methods: A no-argument constructor that sets the monthNumberto 1. An overloaded constructor that accepts the number of the month as an argument. The constructor should set the monthNumberfield to the parameter value if the parameter contains the value 1 – 12. Otherwise, throw an InvalidMonthException exception back to the caller. The exception should note that the month number was incorrect. An overloaded constructor that accepts a string containing the name of the month,…arrow_forwardDefine the class HotelRoom. The class has the following private data members: the room number (an integer) and daily rate (a double). Include a default constructor as well as a constructor with two parameters to initialize the room number and the room’s daily rate. The class should have get/set functions for all its private data members. The constructors and the set functions must throw an invalid_argument exception if either one of the parameter values are negative. The exception handler should display the message “Negative Parameter”. Include a toString() function that nicely formats and returns a string that displays the information ( room number and rate) about the HotelRoom object. Write a main function to test the class HotelRoom, create a HotelRoom Try to set the room rate to an invalid value to generate an exception. Invoke the toSting() function to display the HotelRoom object. Derive the classes GuestRoom from the base class HotelRoom. The GuestRoom has private data fields…arrow_forwardJAVA Problem – CycleThrowTryCatch Revisit the Cycle class. Modify your application such that the properties, numberOfWheels and weightare entered as double values interactively (at the keyboard). Exception handling will be used to determine whether a type mismatch occurs. Edit your application such that, in addition to [A], the values for numberOfWheels and weight, entered interactively, will throw a new exception “Values cannot be less than or equal to zero” only If the values are less than or equal to zero. Add or use the appropriate try and/or catch blocks. Directions Examine your application for the class called Cycle. Add Try and Catch blocks appropriately. Add the throw statement for the new exception. Display an appropriate message if an exception occurs. Display the properties of the object Program public class Cycle { // Declear integer instance variable private int numberOfWheels; private int weight; // Constructer declear class public Cycle(int numberOfWheels,…arrow_forward
- ArgumentException is an existing class that derives from Exception; you use it when one or more of a method’s arguments do not fall within an expected range. Write a C# application SwimmingWaterTemperature containing a variable that can hold a temperature expressed in degrees Fahrenheit. Within the class, create a method that accepts a parameter for a water temperature and returns true or false, indicating whether the water temperature is between 70 and 85 degrees and thus comfortable for swimming. If the temperature is not between 32 and 212 (the freezing and boiling points of water), it is invalid, and the method should throw an ArgumentException. In the Main() method, continuously prompt the user for data temperature, pass it to the method, and then display the following messages indicating whether the temperature is comfortable, not comfortable, or invalid: X degrees is comfortable for swimming. X degrees is not comfortable for swimming. Value does not fall within the expected…arrow_forwardWrite a class named TestScores. The class have an integer array to store test scores, provide getters, setters, constructor should accept an array of test scores as its argument. The class should have a member function that returns the average of the test scores. If any test score in the array is negative or greater than 100, the class should throw an exception. Also if number of values increases the array size it should throw an exception, Demonstrate the class in a program. Use exceptions Programming language : Javaarrow_forwardC++ D.S. Malik Exercise 14-4 Write a program that prompts the user to enter time in 12-hour notation. The program then outputs the time in 24-hour notation. Your program must contain three exception classes: invalidHr, invalidMin, and invalidSec. If the user enters an invalid value for hours, then the program should throw and catch an invalidHr object. Follow similar conventions for the invalid values of minutes and seconds.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
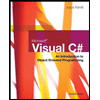
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,