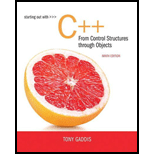
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 16, Problem 2PC
Program Plan Intro
Time Format
Program plan:
source.cpp:
- Include the required header files to the program.
- Define the “main()” function.
- Call the function “setTime()” and pass the “hours” and “sec” with valid and invald data.
- Define the “setTime()” function.
- Create an object for “MilTime” and pass the “hours” and “sec”.
- Display the military hours using “getHour()” function.
- Display the military hours using “getStandHr()” function.
- Display the military hours using “getMin()” function.
- Display the military hours using “getSec()” function.
Time.h:
- Define the “Time.h”.
- In protected, declare the required variable.
- In private, create the constructor by passing hours, minutes and seconds.
- Make a call to the function “getHour()”, “getMin()” and “getSec()” to get hours, minutes and seconds.
MilTime.h:
- Define the “MilTime.h”.
- In private, declare the required variable.
- In public, create the constructor by passing two integer arguments.
- Declare a function “convert()” which convert the hours to military format.
- Create a mutator function for time.
- Create an accessor function for military hours and standard format.
MilTime.cpp:
- Define the “MilTime.h”.
- Create a constructor “MilTime” which is derived from “Time” class.
- Check the hours and seconds value.
- If it is in out of range, then call the “BadHour” and “BadSeconds” exceptions.
- Else set “hrs” to “milHours” and set “sec” to milSeconds;
- Call the function “Convert()”.
- Function definition for function “Convert()”
- Declare a required variable “val”.
- Check whether the “milHours” is greater than “1200”.
- Subtract “milHours” by “1200”.
- Divide “hours” by 100 and assign to variable “hour”.
- Subtract “milHours” by “1200”and divide by 100.0.
- Type cast the variable “val” to “int”.
- Function definition for function “setTime()”
- Assign “hrs” to “milHours”.
- Assign “sec” to “milSeconds”.
- Call the function “convert()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ D.S. Malik Exercise 14-4
Write a program that prompts the user to enter time in 12-hour notation. The program then outputs the time in 24-hour notation. Your program must contain three exception classes: invalidHr, invalidMin, and invalidSec. If the user enters an invalid value for hours, then the program should throw and catch an invalidHr object. Follow similar conventions for the invalid values of minutes and seconds.
Challenge 1: Division.java
You have to implement a Division class that divides two numbers given by the user. The program should handle exceptions that could be thrown during operation:
If the second number is 0
If any of the numbers is not a valid number, such as a letter.
Instructions:
Exception handing
Add exception handling to this game (try, catch, throw). You are expected to use the standard exception classes to try functions, throw objects, and catch those objects when problems occur.
At a minimum, you should have your code throw an invalid_argument object when data from the user is wrong.
File GamePurse.h:
class GamePurse
{
// data
int purseAmount;
public:
// public functions
GamePurse(int);
void Win(int);
void Loose(int);
int GetAmount();
};
File GamePurse.cpp:
#include "GamePurse.h"
// constructor initilaizes the purseAmount variable
GamePurse::GamePurse(int amount){
purseAmount = amount;
}
// function definations
// add a winning amount to the purseAmount
void GamePurse:: Win(int amount){
purseAmount+= amount;
}
// deduct an amount from the purseAmount.
void GamePurse:: Loose(int amount){
purseAmount-= amount;
}
// return the value of purseAmount.
int GamePurse::GetAmount(){
return purseAmount;
}
File…
Chapter 16 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 16.1 - Prob. 16.1CPCh. 16.1 - Prob. 16.2CPCh. 16.1 - Prob. 16.3CPCh. 16.1 - Prob. 16.4CPCh. 16.1 - Prob. 16.5CPCh. 16.3 - Prob. 16.6CPCh. 16.3 - The following function accepts an i nt argument...Ch. 16.3 - Prob. 16.8CPCh. 16.3 - Prob. 16.9CPCh. 16.4 - Prob. 16.10CP
Ch. 16.4 - Prob. 16.11CPCh. 16 - Prob. 1RQECh. 16 - Prob. 2RQECh. 16 - Prob. 3RQECh. 16 - Prob. 4RQECh. 16 - What is unwinding the stack?Ch. 16 - What happens if an exception is thrown by a classs...Ch. 16 - How do you prevent a program from halting when the...Ch. 16 - Why is it more convenient to write a function...Ch. 16 - Why must you be careful when writing a function...Ch. 16 - The line containing a throw statement is known as...Ch. 16 - Prob. 11RQECh. 16 - Prob. 12RQECh. 16 - Prob. 13RQECh. 16 - The beginning of a template is marked by a(n)...Ch. 16 - Prob. 15RQECh. 16 - Prob. 16RQECh. 16 - Write a function that searches a numeric array for...Ch. 16 - Write a function that dynamically allocates a...Ch. 16 - Make the function you wrote in Question 17 a...Ch. 16 - Write a template for a function that displays the...Ch. 16 - Prob. 21RQECh. 16 - Prob. 22RQECh. 16 - Prob. 23RQECh. 16 - Prob. 24RQECh. 16 - T F All type parameters defined in a function...Ch. 16 - Prob. 26RQECh. 16 - T F A class object passed to a function template...Ch. 16 - Prob. 28RQECh. 16 - Prob. 29RQECh. 16 - Prob. 30RQECh. 16 - Prob. 31RQECh. 16 - T F A class template may not be derived from...Ch. 16 - T F A class template may not be used as a base...Ch. 16 - Prob. 34RQECh. 16 - Prob. 35RQECh. 16 - try { quotient = divide(num1, num2); } cout The...Ch. 16 - template class T T square(T number) { return T T;...Ch. 16 - template class T int square(int number) { return...Ch. 16 - Prob. 39RQECh. 16 - Assume the following definition appears in a...Ch. 16 - Assume the following statement appears in a...Ch. 16 - Prob. 1PCCh. 16 - Prob. 2PCCh. 16 - Prob. 3PCCh. 16 - Prob. 4PCCh. 16 - Prob. 5PCCh. 16 - IntArray Class Exception Chapter 14 presented an...Ch. 16 - TestScores Class Write a class named TestScores....Ch. 16 - Prob. 8PCCh. 16 - Prob. 9PCCh. 16 - SortableVector Class Template Write a class...Ch. 16 - Inheritance Modification Assuming you have...Ch. 16 - Prob. 12PCCh. 16 - Prob. 13PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a Month class that holds information about the month. Write exception classes for the following error conditions:• A number less than 1 or greater than 12 is given for the month number.• An invalid string is given for the name of the month.Modify the Month class so that it throws the appropriate exception when either of these errors occurs. Demonstrate the classes in a program.arrow_forward(Constructors Throwing Exceptions) Write a program that shows a constructor passing information about constructor failure to an exception handler after a try blockarrow_forward10.8 LAB: Step counter - exceptions A pedometer treats walking 2,000 steps as walking 1 mile. Write a steps_to_miles() function that takes the number of steps as a parameter and returns the miles walked. The steps_to_miles() function throws a ValueError object with the message "Exception: Negative step count entered." when the number of steps is negative. Complete the main() program that reads the number of steps from a user, calls the steps_to_miles() function, and outputs the returned value from the steps_to_miles() function. Use a try-except block to catch any ValueError object thrown by the steps_to_miles() function and output the exception message. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print(f'{your_value:.2f}') The code I have so far(Python Code): import sysdef steps_to_miles(steps): try: if steps<0: raise ValueError('Exception: Negative step count entered.') return…arrow_forward
- C++ Visual Studio 2019 Complete #13. Dependent #1 Employee and ProductionWorker classes showing below. Modify the Employee and ProductionWorker classes so they throw exceptions when the following errors occur: The Employee class should throw an exception named InvalidEmployeeNumber when it receives an employee number that is less than 0 or greater than 9999. The ProductionWorker class should throw an exception named InvalidShift when it receives an invalid shift. The ProductionWorker class should throw an exception named InvalidPayRate when it receives a negative number for the hourly pay rate. Write a driver program that demonstrates how each of these exception conditions works. #1 Employee and ProductionWorker classes #include <string>#include <iostream>#include <iomanip>using namespace std; class Employee{private: string name; // Employee name string number; // Employee number string hireDate; // Hire date public: // Default…arrow_forwardExceptions are caught and handled by usingarrow_forwardC++ programming D.S.Malik 14-5 Write a program that prompts the user to enter a person’s date of birth in numeric form such as 8-27-1980. The program then outputs the date of birth in the form: August 27, 1980. Your program must contain at least two exception classes: invalidDay and invalidMonth. If the user enters an invalid value for day, then the program should throw and catch an invalidDay object. Follow similar conventions for the invalid values of month and year. (Note that your program must handle a leap year.)arrow_forward
- C++ part1: Define a class named “Variable” that manages a variable name (string) and a description (string). It should not provide the default constructor. And the “VariableString” class that must inherit from the "Variable" class. This class manages a variable name (string), description (string), and value (string). Define a new exception class “VarNameException” that must inherit from the C++ runtime_error class. This class will capture the error case (generate an exception and capture reason and error value) for a variable name when it does not start with a letter (lower case or upper case) or contains at least one blank (for instance: "9name", "*name" or "first name"). Update the class named “Variable” to generate a "VarNameException" whenever the caller tries to create the Variable object with a bad name. part2: Write a function “createVariableObject” that will read the variable name, description, and a value (string) from the user and create a dynamic Variable or VariableString…arrow_forward-Modify your parameter constructors to call your set methods. This will cause validation of the parameter.-Create an exception class named TractorException.-Modify your setters to throw TractorException if invalid values are passed in. You may also need to add a throws clause to your parameter constructors. This way we will not have any print methods in our Tractor object defining class-Modify your main method to try and catch exceptions. It should catch TractorExceptions and general Exceptions and display an appropriate message import java.util.*; class TestException { public static void main(String s[]) { Scanner scanner = new Scanner(System.in); boolean valid=false; // validation loop while (!valid) { try { System.out.println("Enter integer:"); int number = scanner.nextInt(); // may throw an exception System.out.println("You entered "+ number); valid=true;…arrow_forwardCalculator.java, is a simple command-line calculator. Note the program terminates if any operand is nonnumeric.Write a program with an exception handler that deals with nonnumeric operands;then write another program without using an exception handler to achieve thesame objective. Your program should display a message that informs the user ofthe wrong operand type before exitingarrow_forward
- part1: Define a class named “Variable” that manages a variable name (string) and a description (string). It should not provide the default constructor. And the “VariableString” class that must inherit from the "Variable" class. This class manages a variable name (string), description (string), and value (string). Define a new exception class “VarNameException” that must inherit from the C++ runtime_error class. This class will capture the error case (generate an exception and capture reason and error value) for a variable name when it does not start with a letter (lower case or upper case) or contains at least one blank (for instance: "9name", "*name" or "first name"). part2: Write a function “createVariableObject” that will read the variable name, description, and a value (string) from the user and create a dynamic Variable or VariableString depending on the value: if the user provides an empty string value, it will create a Variable object, otherwise it will create a VariableString…arrow_forwardDefine an exception class called CoreBreachException. The class should have a default constructor. If an exception is thrown using zero-argument constructor, getMessage should return "Cor Breach Evacuation Ship!" The class should also define a constructor having a single parameter of type String. If an exception is thrown using constructor, getMessage should return the value that was used as an argument to constructor.arrow_forwardC++ PROGRAM EXCEPTION HANDLING Write a program with a function that accepts a string from user. The function prints “Valid String” only when the string contains at least one capital letter, one vowel, one integer and one ‘@’ character. An example of a valid string is @Developer123. If the user enters any operators like +,*,= then your program must throw a character exception. If the string contains any brackets like {[(}]), then your program must throw an integer exception. Write appropriate catch blocks of these exceptions.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
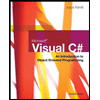
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
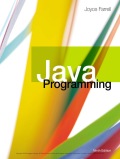
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT