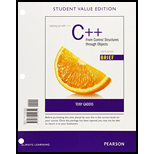
Starting Out with C++ from Control Structures through Objects Brief, Student Value Edition (8th Edition)
8th Edition
ISBN: 9780134014852
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 11PC
Program Plan Intro
File Compare
Program Plan:
Main.cpp:
- Include required header files
- Inside “main ()” function,
- Create an object “ifile” for input file stream
- Create an object “ofile” for output file stream.
- Create two class templates “q1” and “q2” to hold characters
- Declare variables “char1” and “char2”
- Till the end of file,
- Enqueue a character from file1.
- Till the end of file,
- Enqueue a character from file2.
- Close both the files.
- Assign “true” to a Boolean variable “status”.
- Do until both the queue becomes empty,
- Dequeue a character from 1st queue “q1”.
- Dequeue a character from 2nd queue “q2”.
- Check if both the characters are not equal.
- If the condition is true then, assign “false” to the Boolean variable.
- Check if the status is true,
- If the condition is true then, print “The files are identical”.
- If the condition is not true then, print “The files are not identical”.
Dynqueue.h:
- Include required header files.
- Create template class
- Declare a class named “Dynqueue”. Inside the class,
- Inside the “private” access specifier,
- Create a structure named “QueueNode”.
- Create an object for the template
- Create a pointer named “next”.
- Create two pointers named “front” and “rear”.
- Declare a variable.
- Create a structure named “QueueNode”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue ()”, “dequeue ()”, “isEmpty ()”, “isFull ()”, and “clear ()”.
- Inside the “private” access specifier,
- Declare template class.
- Give definition for the constructor.
- Assign the values.
- Declare template class.
- Give definition for the destructor.
- Call the function “clear ()”.
- Declare template class.
- Give function definition for “enqueue ()”.
- Make the pointer “newNode” as null.
- Assign “num” to newNode->value.
- Make newNode->next as null.
- Check whether the queue is empty using “isEmpty ()” function.
- If the condition is true then, assign newNode to “front” and “rear”.
- If the condition is not true then,
- Assign newNode to rear->next
- Assign newNode to “rear”.
- Increment the variable “numItems”.
- Declare template class.
- Give function definition for “dequeue ()”.
- Assign temp pointer as null.
- Check if the queue is empty using “isEmpty ()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Assign the value of front to the variable “num”.
- Make front->next as “temp”.
- Delete the front value
- Make temp as front.
- Decrement the variable “numItems”.
- Declare template class.
- Give function definition for “isEmpty ()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “clear ()”.
- Create an object for template.
- Dequeue values from queue till the queue becomes empty using “while” condition.
- Create an object for template.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
A queue and a deque data structure are related concepts. Deque is an acronym meaning "double-ended queue." With a deque, you may insert, remove, or view from either end of the queue, which distinguishes it from the other two. Use arrays to implement a deque
Elections
Problem Description
Elections are going on, and there are two candidates A and B, contesting with each other. There is a queue of voters and in this queue some of them are supporters of A and some of them are supporters of B. Many of them are neutral. The fate of the election will be decided on which side the neutral voters vote. Supporters of A and supporters of B make attempt to win the votes of neutral voters.
The way this can be done is explained below:
1. The voter queue is denoted by three characters, viz {-, A, B}. The - denotes neutral candidate, A denotes supporter of candidate A and B denotes supporter of candidate B.
2. Supporters of A can only move towards the left side of the queue.
3. Supporters of B can only move towards the right side of the queue.
4. Since time is critical, supporters of both A and B will move simultaneously.
5. They both will try and influence the neutral voters by moving in their direction in the queue. If supporter of A reaches the…
LINKED LIST IN PYTHON
Create a program using Python for the different operations of a Linked
List.
Your program will ask the user to choose an operation.
1. Create a List
-Ask the user how many nodes he/she wants.
-Enter the element/s
-Display the list
-Back to menu
2. Add at beginning
-Ask for the element to be inserted.
-Display the list
-Back to menu
3. Add after
-Ask for the element to be inserted.
-Ask for the position AFTER which the element is to be inserted
-Display the list
-Back to menu
4. Delete
-Ask for the element (data) to be deleted
-If found, delete the node with that data.
-If multiple values, delete only the first element found
-If not found, display that the element is not found
-back to menu
5. Display
-Display the list
6. Count
-Display the number of elements
7. Reverse
-Reverse the list and display it
8. Search
-Ask the user for the element (data) to be searched
-Display a message if the element is found or not
9. Quit
-Exits the program
Chapter 18 Solutions
Starting Out with C++ from Control Structures through Objects Brief, Student Value Edition (8th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - The STL stack is considered a container adapter....
Ch. 18 - What types may the STL stack be based on? By...Ch. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - The STL stack container is an adapter for the...Ch. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - Prob. 26RQECh. 18 - Write two different code segments that may be used...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Dynamic String Stack Design a class that stores...Ch. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Inventory Bin Stack Design an inventory class that...Ch. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- void do(queue<int>&q){ stack<int>temp; while(!q.empty()){temp.push(q.front()); q.pop();} while(!temp.empty(){q.push(temp.top()); temp.pop();}} After this function the queue q is a. doesn't changed b. has rubbish data c. destroyed (empty) d. reversedarrow_forwardUsing circular arrays concept Implement Queue using circular arrays (must use functions and arrays only) Write a C++ program to perform the following operations on the Queue Implement a menu to give the user options to choose from a) create Q b) enq (add an item) c) deq (remove an item) d) front (what item is in the front) e) empty check f) full check g) purge destroy the q I need help with how to write the program i C++! Thank you so much in advance.arrow_forwardCreate a pseudocode for the Queue Operations. The pseudocode should accept N values, checks if the queue is full or empty and display messages indicating if the Queue is empty or full. It should also print the final contents.arrow_forward
- #include <iostream> using namespace std; #define SIZE 5 //creating the queue using array int A[SIZE]; int front = -1; int rear = -1; //function to check if the queue is empty bool isempty() { if(front == -1 && rear == -1) return true; else return false; } //function to enter elements in queue void enqueue ( int value ) { //if queue is full if ((rear + 1)%SIZE == front) cout<<"Queue is full \n"; else { //now the first element is inserted if( front == -1) front = 0; //inserting element at rear end rear = (rear+1)%SIZE; A[rear] = value; } } //function to remove elements from queue void dequeue ( ) { if( isempty() ) cout<<"Queue is empty\n"; else //only one element if( front == rear ) front = rear = -1; else front = ( front + 1)%SIZE; } //function to show the element at front void showfront() { if( isempty()) cout<<"Queue is empty\n"; else cout<<"element at front is:"<<A[front]; } //function to display the queue void…arrow_forwardA deque data structure and a queue are related ideas. The abbreviation "deque" stands for "double-ended queue." A deque differs from the other two in that you may insert, remove, or view from either end of the queue. Implement a deque using arrays.arrow_forwardA data structure called a deque is closely related to a queue. The name deque stands for “double-ended queue.” The difference between the two is that with a deque, you can insert, remove, or view from either end of the queue. Implement a deque using arraysarrow_forward
- please use DEQUE #include <iostream>#include <string>#include <deque> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","TUL"}; int main(){// define stack (or queue ) herestring origin;string dest;string citypair;cout << "Loading the CONTAINER ..." << endl;// LOAD THE STACK ( or queue) HERE// Create all the possible Airport combinations that could exist from the list provided.// i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ...// DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached shell program (AirportCombos.cpp), create a list of strings to process and place on a STL DEQUE container. Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible…arrow_forwardQueue is referred to be as First-In-First-Out (FIFO) list. True or Falsearrow_forwardjava data structure Queue: Q4: A program performs the following operations on an empty queue Q: Q.enqueue(24) Q.enqueue(74) Q.enqueue(34) Q.first() Q.dequeue() Q.enqueue(12) Q.dequeue() Please show the queue contents at the end of these operations. Clearly show the front of the queue.arrow_forward
- IN C LANGUAGE True or False: You can not store multiple linked lists in a contiguous block of memory, even if there is space available for new nodes.arrow_forwardIN C LANGUAGE When inserting a node into a linked list, where (in memory) will that node be placed if there is no overflow? A) The location of the AVAIL pointer B) The next contiguous spot of memory from the last node C) The very end of the contiguous memory block D) A randomized memory locationarrow_forward20 T OR F An application programmer can prevent accessing an empty queue by using the isEmpty method in an if-statement to prevent such access.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
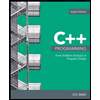
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning