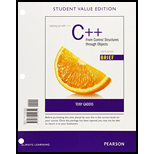
Starting Out with C++ from Control Structures through Objects Brief, Student Value Edition (8th Edition)
8th Edition
ISBN: 9780134014852
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 10PC
Program Plan Intro
File Filter
Program Plan:
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Create an object “file1” for input file stream.
- Create an object “ofile” for output file stream.
- Declare a class template
- Declare character variables named “popChar”, and “ch”.
- Till the end of file,
- Convert each character and Enqueue the character using the function “enqueue ()”.
- Close “file1”.
- Until the stack gets empty,
- Pop the character stack and write it on output file “ofile” using the function “dequeue ()”.
- Close the output file“ofile”.
- Open the output file “ofile”.
- Till the end of file,
- Get a character from queue and print it on the console screen.
DynQueue.h:
- Include required header files.
- Create template class
- Declare a class named “DynQueue”. Inside the class,
- Inside the “private” access specifier,
- Create a structure named “QueueNode”.
- Create an object for the template
- Create a pointer named “next”.
- Create two pointers named “front” and “rear”.
- Declare a variable.
- Create a structure named “QueueNode”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue ()”, “dequeue ()”, “isEmpty ()”, “isFull ()”, and “clear ()”.
- Inside the “private” access specifier,
- Declare template class.
- Give definition for the constructor.
- Assign the values.
- Declare template class.
- Give definition for the destructor.
- Call the function “clear ()”.
- Declare template class.
- Give function definition for “enqueue ()”.
- Make the pointer “newNode” as null.
- Assign “num” to newNode->value.
- Make newNode->next as null.
- Check whether the queue is empty using “isEmpty ()” function.
- If the condition is true then, assign newNode to “front” and “rear”.
- If the condition is not true then,
- Assign newNode to rear->next
- Assign newNode to “rear”.
- Increment the variable “numItems”.
- Declare template class.
- Give function definition for “dequeue ()”.
- Assign temp pointer as null.
- Check if the queue is empty using “isEmpty ()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Assign the value of front to the variable “num”.
- Make front->next as “temp”.
- Delete the front value
- Make temp as front.
- Decrement the variable “numItems”.
- Declare template class.
- Give function definition for “isEmpty ()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “clear ()”.
- Create an object for template.
- Dequeue values from queue till the queue becomes empty using “while” condition.
- Create an object for template.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
File Encryption and DecryptionWrite a program that uses a dictionary to assign “codes” to each letter of the alphabet. Forexample:codes = { ‘A’ : ‘%’, ‘a’ : ‘9’, ‘B’ : ‘@’, ‘b’ : ‘#’, etc . . .}Using this example, the letter A would be assigned the symbol %, the letter a would beassigned the number 9, the letter B would be assigned the symbol @, and so forth.The program should open a specified text file, read its contents, then use the dictionaryto write an encrypted version of the file’s contents to a second file. Each character in thesecond file should contain the code for the corresponding character in the first file.Write a second program that opens an encrypted file and displays its decrypted contentson the screen.
in phyton
Word FrequencyWrite a python program that reads the contents of a text file. The program should create a dictio-nary inwhich the keys are the individual words found in the file and the values are the number of timeseach word appears. For example, if the word “the” appears 128 times, the dictionary wouldcontain an element with 'the' as the key and 128 as the value. The program should eitherdisplay the frequency of each word or create a second file containing a list of each word and itsfrequency.
Unique WordsWrite a python program that opens a specified text file then displays a list of all the unique words foundin the file.Hint: Store each word as an element of a set.
Chapter 18 Solutions
Starting Out with C++ from Control Structures through Objects Brief, Student Value Edition (8th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - The STL stack is considered a container adapter....
Ch. 18 - What types may the STL stack be based on? By...Ch. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - The STL stack container is an adapter for the...Ch. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - Prob. 26RQECh. 18 - Write two different code segments that may be used...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Dynamic String Stack Design a class that stores...Ch. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Inventory Bin Stack Design an inventory class that...Ch. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- LINKED LIST IN PYTHON Create a program using Python for the different operations of a Linked List. Your program will ask the user to choose an operation. 1. Create a List -Ask the user how many nodes he/she wants. -Enter the element/s -Display the list -Back to menu 2. Add at beginning -Ask for the element to be inserted. -Display the list -Back to menu 3. Add after -Ask for the element to be inserted. -Ask for the position AFTER which the element is to be inserted -Display the list -Back to menu 4. Delete -Ask for the element (data) to be deleted -If found, delete the node with that data. -If multiple values, delete only the first element found -If not found, display that the element is not found -back to menu 5. Display -Display the list 6. Count -Display the number of elements 7. Reverse -Reverse the list and display it 8. Search -Ask the user for the element (data) to be searched -Display a message if the element is found or not 9. Quit -Exits the programarrow_forwardLinear list directory structures have the disadvantage that you must search an entire linked list to ensure that a new file doesn't match a name already in the directory. Question 2 options: True Falsearrow_forwardWord IndexWrite a python program that reads the contents of a text file. The program should create a dictionary inwhich the key-value pairs are described as follows:Key. The keys are the individual words found in the file.576Values. Each value is a list that contains the line numbers in the file where the word (thekey) is found.For example, suppose the word “robot” is found in lines 7, 18, 94, and 138. The dictionarywould contain an element in which the key was the string “robot”, and the value was a listcontaining the numbers 7, 18, 94, and 138.Once the dictionary is built, the program should create another text file, known as a word index,listing the contents of the dictionary. The word index file should contain an alphabetical listingof the words that are stored as keys in the dictionary, along with the line numbers where thewords appear in the original file. Figure 9-1 shows an example of an original text file(Kennedy.txt) and its index file (index.txtarrow_forward
- Exercise 6. Create a dictionary from a Text File: When a program incorporates a large dictionary, the dictionary is usually created from a file, such as a text file by using dict function to convert a list into a dictionary. Whenever a word in the original sentence is not a key in the dictionary, the get method places the word itself into the translated sentence. The file “Textese.txt” contains a word and its translation into textese. Write a program to create a dictionary with the file, ask the user to enter a simple sentence then translate it into textese. Define two function createDictionary (filename) and translate (sentence, dictionary). Then define a main function to test your functionsarrow_forwardThis file object method returns a list containing the file’s contents.a. to_listb. getlistc. readlined. readlinesarrow_forwardThe program, word_frequency, counts the number of occurrences of words in a document. The program takes a filename as a parameter and performs the task. Get the program to run using the attached text file called document.txt. The file contains a piece of text. After you run the program and understand it's logic, modify word_frequency.py to use a binary search tree instead of a dictionary. Tasks: 1. Import a binary search tree 2. Change the filename in the program to reflect the change(s) made Python Only and no other coding languages. Import the module first before running it. Please show output when done. (build off of this file and try not to change too much) word_frequency.py: import sysfilename = sys.argv[1] freq = {}for piece in open(filename).read().lower().split():# only consider alphabetic characters within this pieceword = ''.join(c for c in piece if c.isalpha())if word: # require at least one alphabetic character freq[word] = 1 +…arrow_forward
- Phyton: Program 6: Write a program that allows the user to add data from files or by hand. The user may add more data whenever they want. The print also allows the user to display the data and to print the statistics. The data is store in a list. It’s easier if you make the list global so that other functions can access it. You should have the following functions: · add_from_file() – prompts the user for a file and add the data from it. · add_by_hand() – prompts the user to enter data until user type a negative number. · print_stats() – print the stats (min, max, mean, sum). You may use the built-in functions. Handling errors such as whether the input file exist is extra credit. To print a 10-column table, you can use the code below: for i in range(len(data)): print(f"{data[i]:3}", end="") if (i+1)%10==0: print() print() Sample run: Choose an action 1) add data from a file 2) add data by hand 3) print stats 4)…arrow_forwardlinked_list_stack_shopping_list_manager.py This is a file that includes the class of linked list based shopping list manager. This class contains such methods as init, insert_item, is_list_empty, print_item_recursive_from_top, print_items_from_top, print_item_recursive_from_bottom, print_items_from_bottom, getLastItem, removeLastItem. In addition, this class requires the inner class to hold onto data as a linked list based on Stack. DO NOT use standard python library, such as deque from the collections module. Please keep in mind the following notes for each method during implementation: Init(): initializes linked list based on Stack object to be used throughout object life. insert_item(item): inserts item at the front of the linked list based on Stack. Parameters: item name. is_list_empty (): checks if the current Stack object is empty (ex. check if head is None). print_item_recursive_from_top(currentNode): a helper method to print linked list based on Stack item recursively. Note:…arrow_forward(C Language) A photographer is organizing a photo collection about the national parks in the US and would like to annotate the information about each of the photos into a separate set of files. Write a program that reads the name of a text file containing a list of photo file names. The program then reads the photo file names from the text file, replaces the "_photo.jpg" portion of the file names with "_info.txt", and outputs the modified file names. Assume the unchanged portion of the photo file names contains only letters and numbers, and the text file stores one photo file name per line. If the text file is empty, the program produces no output. Assume also the maximum number of characters of all file names is 100.arrow_forward
- 1. In a loop, fill an array for up to 50 even numbers and a set for up to 50 odd numbers. Use the merge() algorithm to merge these containers into a vector. Display the vector contents to show that all went well. (In C++ language) 2. Write a program that copies a source file of integers to a destination file, using stream iterators. The user should supply both source and destination filenames to the program. You can use a while loop approach and a list container with integer data type. Within the loop, read each integer value from the input iterator and add the value to the list container by using push_back() function, then increment the input iterator.Finally, use an output iterator to write the list to the destination file. (In C++ language)arrow_forwardFile Analysis USING PYTHON- DICTIONARY NOTE FIND ATTACHED BELOW 2 FILES THA SHOULD BE USED FOR THIS ASSIGNMENT Using python, Write a program that reads the contents of two text files and compares them in the followingways:It should display a list of all the unique words contained in both files.It should display a list of the words that appear in both files.It should display a list of the words that appear in the first file but not the second.It should display a list of the words that appear in the second file but not the first.It should display a list of the words that appear in either the first or second file, but notboth.Hint: Use set operations to perform these analyses. ATTACHED FILES first FILENAME: first_file.txt The quick brown fox jumps over the lazy dog. Second FILENAME: second_file.txt Jack be nimble, Jack be quick, Jack jump over the candlestick. . For the program, you need to write: Comments for all the values, constants, and functions IPO Variables Pseudocodearrow_forwardContact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe 123-5432 Linda…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
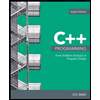
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning