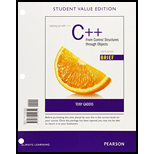
Concept explainers
Inventory Bin Stack
Design an inventory class that stores the following members:
serialNum: An integer that holds a part’s serial number.
manufactDate: A member that holds the date the part was manufactured.
lotNum: An integer that holds the part’s lot number.
The class should have appropriate member functions for storing data into, and retrieving data from, these members.
Next, design a stack class that can hold objects of the class described above. If you wish, you may use the template you designed in
Last, design a program that uses the stack class described above. The program should have a loop that asks the user if he or she wishes to add a part to inventory, or take a part from inventory. The loop should repeat until the user is finished.
If the user wishes to add a part to inventory, the program should ask for the serial number, date of manufacture, and lot number. The data should be stored in an inventory object, and pushed onto the stack.
If the user wishes to take a part from inventory, the program should pop the top-most part from the stack and display the contents of its member variables.
When the user finishes the program, it should display the contents of the member values of all the objects that remain on the stack.

Want to see the full answer?
Check out a sample textbook solution
Chapter 18 Solutions
Starting Out with C++ from Control Structures through Objects Brief, Student Value Edition (8th Edition)
Additional Engineering Textbook Solutions
C Programming Language
Starting Out with Programming Logic and Design (4th Edition)
Database Concepts (8th Edition)
Computer Systems: A Programmer's Perspective (3rd Edition)
Starting Out With Visual Basic (8th Edition)
Problem Solving with C++ (10th Edition)
- Coding Language C++ Note: Do not make use of coding techniques that are too advanced. Techniques such as linked lists, recursive functions are not permitted. Must be done using classes Private Member Functions The member functions declared private, isLeap, daysPerMonth, name, number, are helper functions - member functions that will never be needed by a user of the class, and so do not belong to the public interface (which is why they are "private"). They are, however, needed by the interface functions (public member functions), which use them to test the validity of arguments and construct valid dates. For example, the constructor that passes in the month as a string will call the number function to assign a value to the unsigned member variable month. isLeap: The rule for whether a year is a leap year is: (year % 4 == 0) implies leap year except (year % 100 == 0) implies NOT leap year except (year % 400 == 0) implies leap year So, for instance, year 2000 is a leap year, but 1900…arrow_forwardCoding Language C++ Note: Do not make use of coding techniques that are too advanced such as linked lists or recursive functions Must be done using classes Private Member Functions The member functions declared private, isLeap, daysPerMonth, name, number, are helperfunctions - member functions that will never be needed by a user of the class, and so do not belong to the public interface (which is why they are "private"). They are, however, needed by the interface functions (public member functions), which use them to test the validity of arguments and construct valid dates. For example, the constructor that passes in the month as a string will call the number function to assign a value to the unsigned member variable month. isLeap: The rule for whether a year is a leap year is: (year % 4 == 0) implies leap year except (year % 100 == 0) implies NOT leap year except (year % 400 == 0) implies leap year So, for instance, year 2000 is a leap year, but 1900 is NOT a leap year. Years…arrow_forwardPointer and classImplement a class called Team as specified:data members:name - the name of the Team (defined as a dynamic variable)members - a dynamic array of stringsize - number of members in the team.functions:Course(): default constructor set name to “TBD”, and size to 0, members toempty listCourse(string): one argument constructor set name, and size to 0, members toempty listaccessor - an accessor for the name and size variablemutator - an mutator for the name variableUse following main() to test your class.int main() {Team a,b("Mets");cout<<a.getName()<<endl; // print TBDa.setName("Yankee");cout<<a.getName()<<endl; // print Yankeecout<<b.getName()<<endl; // print Metscout<<a.getSize()<<endl; // print 0return 0;}arrow_forward
- (Polynomial Class) Develop class Polynomial. The internal representation of a Polynomialis an array of terms. Each term contains a coefficient and an exponent, e.g., the term2x4has the coefficient 2 and the exponent 4. Develop a complete class containing proper constructorand destructor functions as well as set and get functions. The class should also provide the followingoverloaded operator capabilities:a) Overload the addition operator (+) to add two Polynomials.b) Overload the subtraction operator (-) to subtract two Polynomials.c) Overload the assignment operator to assign one Polynomial to another.d) Overload the multiplication operator (*) to multiply two Polynomials.e) Overload the addition assignment operator (+=), subtraction assignment operator (-=),and multiplication assignment operator (*=).arrow_forwardC++ Write a class template that uses a vector to implement a stack data structure with these member functions: 1. (constructor) : Construct stack (public member function ) 2. empty : Test whether container is empty (public member function ) 3. size : Return size (public member function ) 4. top : Access next element (public member function ) 5. push : Insert element (public member function ) 6. pop : Remove top element (public member function ) Put function definitions outside the definition of the class (i.e. inline functions are not allowed).arrow_forwardJava - For 7 and 8 use the following definition: class node { boolean data; node link; } 7. Consider a structure using a linked list in which you added and removed from the back of the list. Using the node definition above, write the pseudocode for a member method “add” to this structure. Assume the existence of a “front” and “back” reference. 8. Assume you are working with a stack implementation of the linked list definition above (above question #7), write a member method “pop”. The method should return a value (in the “popped” node). Assume the existence of the node references called “TheStack” and “Top”. These references point to the start (or bottom) and top of the stack (or back of the list).arrow_forward
- Using C++ to Creates a class called Member with two integer x and y. a. Add a constructor able to create a Member object with tow integers and the default values 0,0; b. Add the methods setX and setY to modify the attributes x and y; c. Add the method display able to display the attributes, - A Queue is a special array where the insertion and deletion will be via a specific index called "head". A Queue is characterized by 3 attributes: a. capacity (int): the maximum number element Member that can be contained into the Queue; b. head: presents the index where we can add/remove element to the Queue. The head value presents also the current number of elements into the Queue. When a Queue is created the initial value of head is 0; c. Member content[]: an array of elements of type Member; Creates the class Queue with the following methods: a. a Constructor able to create a Queue with maximum 10 Members; b. bool empty (): this method returns true if no element exists in the Queue c. bool…arrow_forward// CLASS PROVIDED: p_queue (priority queue ADT)// TYPEDEFS and MEMBER CONSTANTS for the p_queue class:// typedef _____ value_type// p_queue::value_type is the data type of the items in// the p_queue. It may be any of the C++ built-in types// (int, char, etc.), or a class with a default constructor, a// copy constructor, an assignment operator, and a less-than operator forming a strict weak ordering.// typedef _____ size_type// p_queue::size_type is the data type considered best-suited// for any variable meant for counting and sizing (as well as// array-indexing) purposes; e.g.: it is the data type for a// variable representing how many items are in the p_queue.// It is also the data type of the priority associated with// each item in the p_queue// static const size_type DEFAULT_CAPACITY = _____// p_queue::DEFAULT_CAPACITY is the default initial capacity of a// p_queue that is created by the default constructor.// CONSTRUCTOR for the…arrow_forwardUse Python for this question. Also please comment what each line of code means for this question as well: Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!) Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. push() – take an item as input and push it on the top of the stack pop() – remove and return the item at the top of the stack isEmpty() – returns True if the stack is empty,…arrow_forward
- In C++ Syntax for arrays of objects classGrades allCS[15]; //the default constructor is applied to //all elements of this array //to set the number of students in each class to 25 for (i = 0; i < 15; i++) { allCS[i].setNumStudents(25); } //Assume values in all classes. Write the code to print all class averages?arrow_forwardCPU Scheduling Algorithm Simulator in C++Instructions:Create a .zip file consisting of code, output files, readme file .The readme file should explain briefly your code. The simulator will consists of following classes:(i) Process: The data members of this class should store process id, arrival time in the ready queue,CPU burst time, completion time, turn around time, waiting time, and response time. The memberfunctions of this class should assign values to the data members and print them. A constructorshould also be used.(ii) Process_Creator: This class will create an array of processes and assign a random arrival timeand burst time to each process. Data members, constructor and member functions can be writtenaccordingly.(iii) Scheduler: This class will implement the scheduling algorithm. The class will maintain a readyqueue of infinite capacity (i.e., any number of processes can be accommodated in the queue). Theready queue should be implemented using the min-Heap data structure…arrow_forward// This program reads floating point data from a data file and places those // values into the private data member called values (a floating point array) // of the FloatList class. Those values are then printed to the screen.// The input is done by a member function called GetList. The output// is done by a member function called PrintList. The amount of data read in // is stored in the private data member called length. The member function// GetList is called first so that length can be initialized to zero.#include <iostream>#include <fstream>#include <iomanip> using namespace std;const int MAX_LENGTH = 50; // MAX_LENGTH contains the maximum length of our list class FloatList // Declares a class that contains an array of// floating point numbers{ public:void getList(ifstream&); // Member function that gets data from a file void printList() const;// Member function that prints data from that // file to the screen.FloatList();// constructor that sets length to…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
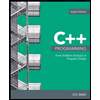