STARTING OUT WITH C++ REVEL >IA<
9th Edition
ISBN: 9780135853115
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 11PC
Program Plan Intro
List Search using template
Program Plan:
“IntList.h”:
- Include the required specifications into the program.
- Define a class template named “IntList”.
- Declare the member variables “value” and “*next” in structure named “ListNode”.
- Declare the constructor, copy constructor, destructor, and member functions in the class.
- Define a copy constructor named “IntList()” as a template which takes an address of object for the “IntList” class as “const”.
- Declare a structure pointer variable “nodePtr” and initialize it to be “nullptr”.
- Assign “obj.head” value into the received variable “nodePtr”.
- Make a “while” loop to copy the received values into “nodePtr”.
- Make a call to “appendNode()” to insert values to “nodePtr” and initialize address of “next” into “nodePtr”.
- Define a function named “appendNode()”as a template to insert the node at end of the list.
- Declare the structure pointer variables “newNode” and “dataPtr” for the structure named “ListNode”.
- Assign the value “num” to the variable “newNode” and assign null to the variable “newNode”.
- Using “if…else” condition check whether the list is empty or not, if the “head” is empty then make a new node into “head” pointer. Otherwise, make a loop to find last node in the loop.
- Assign the value of “dataPtr” into the variable “newNode”.
- Define a function named “print()”as a template to print the values in the list.
- Declare the structure pointer “dataPtr” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Make a loop “while” to display the values of the list.
- Define a function named “insertNode()”as a template to insert a value into the list.
- Declare the structure pointer variables “newNode”, “dataPtr”, and “prev” for the structure named “ListNode”.
- Make a “newNode” value into the received variable value “num”.
- Use “if…else” condition to check whether the list is empty or not.
- If the list is empty then initialize “head” pointer with the value of “newNode” variable.
- Otherwise, make a “while” loop to test whether the “num” value is less than the list values or not.
- Use “if…else” condition to initialize the value into list.
- Define a function named “deleteNode()” as a template to delete a value from the list.
- Declare the structure pointer variables “dataPtr”, and “prev” for the structure named “ListNode”.
- Use “if…else” condition to check whether the “head” value is equal to “num” or not.
- Initialize the variable “dataPtr” with the value of the variable “head”.
- Remove the value using “delete” operator and reassign the “head” value into the “dataPtr”.
- If the “num” value not equal to the “head” value, then define the “while” loop to assign the “dataPtr” into “prev”.
- Use “if” condition to delete the “prev” pointer.
- Define a function named “reverse()”as a template to reverse the values in a list.
- Declare the pointer variables “newNode”, “newHead”, “nodePtr”, and “tempPtr” for the structure named “ListNode”.
- Initialize the variable “nodePtr” with the value of the variable “head”.
- Define a “while” loop to allocate “newNode” variable.
- Create a “newNode” for the structure “ListNode”.
- Store the value of “nodePtr” into “newNode” and assign address as null to the “newNode” pointer.
- Using “if…else” condition swap the values of “newHead” and “newNode”.
- Assign the address of “next” node into “nodePtr”.
- Initialize the variable “head” with the value of the variable “newHead”.
- Define a function named “destroy()”as a template to destroy the list values from the memory.
- Declare the structure pointer variables “dataPtr”, and “nextNode” for the structure named “ListNode”.
- Initialize the “head” value into the “dataPtr”.
- Define a “while” loop to make the links of node into “nextNode” and remove the node using “delete” operator.
- Define a function named “insert()” as a template with the arguments of “value” and “pos” to insert a value at specified location.
- Declare a pointer variable “newNode” for the structure “ListNode”.
- Assign the value of received variable “value” into “newNode” value and make address of “newNode” into “nullptr”.
- Using “if” condition to check whether the list is empty or not.
- If list is empty, initialize the variable “head” with the value of the variable “newNode”.
- Using “if” condition to insert the value of received variable “pos” into the list.
- Assign the “head” node into address of “newNode” .
- Initialize the variable “head” with the value of the variable “newNode”.
- Using “while” loop to insert the value at specified position in the list.
- Define a function named “removeByPos()” as a template with an argument “pos” to remove a value at specified position in list.
- Declare a pointer variable “temp” for the structure “ListNode”.
- Using “if” condition, check whether the list is “empty” or not. If the list is empty, return “null” to “main()” function.
- Otherwise, using “while” loop to traverse the list to find the “pos” in list.
- Using “if…else” condition, check whether the received value of “pos” is value of “head” or not.
- If the condition is true, delete “head” node from the list.
- Otherwise, assign pointers to the next node of removable value then delete the node using “delete” operator.
- Define the destructor to call the member function “destroy()” in the list.
- Define a function named “search()” as a template to find the value of “num” in the list.
- Declare a variable “count” in type of “int”.
- Declare a structure pointer variable “*dataPtr” for the structure named “ListNode”.
- Define a “while” loop to search the value in the list.
- Using “if…else” statement, check the value of “dataPtr” in the list.
- If the condition is “true”, return the value “count” variable.
- Otherwise, point the “next” value of “dataPtr” and then increment the value of “count” variable.
- Using “if…else” statement, check the value of “dataPtr” in the list.
- Return a value “-1” to the function call.
“Main.cpp”:
- Include the required header files into the program.
- Declare an object named “obj” for the class “IntList”.
- Make a call to functions for insert and append operations.
- Make a call to “print()” function to display the list on the screen.
- Make a call to “search()” function to find the value in the list.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ function Linked list
Write a function, to be included in an unsorted linked list class, called replaceItem, that will receive two parameters, one called olditem, the other called new item. The function will replace all occurrences of old item with new item (if old item exists !!) and it will return the number of replacements done.
HELP
Write C code that implements a soccer team as a linked list.
1. Each node in the linkedlist should be a member of the team and should contain the following information:
What position they play
whether they are the captain or not
Their pay
2. Write a function that adds a new members to this linkedlist at the end of the list.
Write C code that implements a soccer team as a linked list.
1. Each node in the linkedlist should be a member of the team and should contain the following information:
What position they play
whether they are the captain or not
Their pay
2. Write a function that adds a new members to this linkedlist at the end of the list.
Chapter 18 Solutions
STARTING OUT WITH C++ REVEL >IA<
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - Prob. 18.2CPCh. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.2 - Prob. 18.5CPCh. 18.2 - Prob. 18.6CPCh. 18.2 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - Prob. 3RQECh. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - Prob. 6RQECh. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - T F The programmer must know in advance how many...Ch. 18 - T F It is not necessary for each node in a linked...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 33RQECh. 18 - Prob. 34RQECh. 18 - Prob. 35RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - List Template Create a list class template based...Ch. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a struct ‘Student’ that has member variables: (string) first name, (int) age and (double) fee. Create a sorted (in ascending order according to the age) linked list of three instances of the struct Student. The age of the students must be 21, 24 and 27. Write a function to insert the new instances (elements of the linked list), and insert three instance whose member variable have age (i) 20, (ii) 23 and (iii) 29. Write a function to remove the instances from the linked list, and remove the instances whose member variables have age (i) 21 and (ii) 29. Write a function to count the elements of the linked list. Write a function to search the elements from the linked list, and implement the function to search an element whose age is 23. Write a function to print the linked list on the console. Consider the Student struct as defined above. Create a stack of 5objects of the class. Implement the following (i) push an element to the stack (ii) pop an element from the stack (iii) get the…arrow_forward(Circular linked lists) This chapter defined and identified various operations on a circular linked list.a. Write the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.)b. Write a program to test various operations of the class defined in (a).arrow_forwardTopic: Singly Linked ListImplement the following functions in C++ program. Read the question carefully. (See attached photo for reference) void isEmpty() This method will return true if the linked list is empty, otherwise return false. void clear() This method will empty your linked list. Effectively, this should and already has been called in your destructor (i.e., the ~LinkedList() method) so that it will deallocate the nodes created first before deallocating the linked list itself.arrow_forward
- Max Absolute In List Function Lab Description Implement function max_abs_val(lst), which returns the maximum absolutevalue of the elements in list.For example, given a list lst: [-19, -3, 20, -1, 0, -25], the functionshould return 25. The name of the method should be max_abs_val and the method should take one parameter which is the list of values to test. Here is an example call to the function print(max_abs_val([-19, -3, 20, -1, 0, -25])) File Name maxabsinlst.py Score There are three tests each worth 2 points Note: You do not need any other code including the main method or any print statements. ONLY the max_abs_val method is required. Otherwise, the autograder will fail and be unable to grade your code. (I.e., do not include the above example in your code.) The above example should be used be test your code but deleted or comment out upon submission. PYTHON LABarrow_forward8.16 LAB: Mileage tracker for a runner C++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the…arrow_forward8.16 LAB: Mileage tracker for a runner C++ Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 I need the //TODO completed Main.cpp #include "MileageTrackerNode.h" #include <string> #include <iostream> using namespace std; int main (int argc, char* argv[]) { // References for MileageTrackerNode objects MileageTrackerNode* headNode; MileageTrackerNode* currNode; MileageTrackerNode* lastNode; double miles; string date; int i; // Front of nodes list headNode = new MileageTrackerNode(); lastNode = headNode; // TODO: Read in the number of nodes cin >> i int p = i; currNode…arrow_forward
- Concatenate Map This function will be given a single parameter known as the Map List. The Map List is a list of maps. Your job is to combine all the maps found in the map list into a single map and return it. There are two rules for addingvalues to the map. You must add key-value pairs to the map in the same order they are found in the Map List. If the key already exists, it cannot be overwritten. In other words, if two or more maps have the same key, the key to be added cannot be overwritten by the subsequent maps. Signature: public static HashMap<String, Integer> concatenateMap(ArrayList<HashMap<String, Integer>> mapList) Example: INPUT: [{b=55, t=20, f=26, n=87, o=93}, {s=95, f=9, n=11, o=71}, {f=89, n=82, o=29}]OUTPUT: {b=55, s=95, t=20, f=26, n=87, o=93} INPUT: [{v=2, f=80, z=43, k=90, n=43}, {d=41, f=98, y=39, n=83}, {d=12, v=61, y=44, n=30}]OUTPUT: {d=41, v=2, f=80, y=39, z=43, k=90, n=43} INPUT: [{p=79, b=10, g=28, h=21, z=62}, {p=5, g=87, h=38}, {p=29,…arrow_forwardC Language In a linear linked list, write a function named changeFirstAndLast that swaps the node at the end of the list and the node at the beginning of the list. The function will take a list as a parameter and return the updated list.arrow_forwardQuestion: struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad. Question: struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad.arrow_forward
- 8.14 LAB: Mileage tracker for a runner Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp: #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData()…arrow_forward8.14 LAB: Mileage tracker for a runner Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp: #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData()…arrow_forward8.14 LAB: Mileage tracker for a runner Given the MileageTrackerNode class, complete main() to insert nodes into a linked list (using the InsertAfter() function). The first user-input value is the number of nodes in the linked list. Use the PrintNodeData() function to print the entire linked list. DO NOT print the dummy head node. Ex. If the input is: 3 2.2 7/2/18 3.2 7/7/18 4.5 7/16/18 the output is: 2.2, 7/2/18 3.2, 7/7/18 4.5, 7/16/18 Main.cpp #include "MileageTrackerNode.h"#include <string>#include <iostream>using namespace std; int main (int argc, char* argv[]) {// References for MileageTrackerNode objectsMileageTrackerNode* headNode;MileageTrackerNode* currNode;MileageTrackerNode* lastNode; double miles;string date;int i; // Front of nodes listheadNode = new MileageTrackerNode();lastNode = headNode; // TODO: Read in the number of nodes // TODO: For the read in number of nodes, read// in data and insert into the linked list // TODO: Call the PrintNodeData()…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
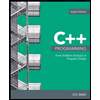
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning