STARTING OUT WITH C++ REVEL >IA<
9th Edition
ISBN: 9780135853115
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 9PC
Program Plan Intro
Rainfall Statistics Modification
Program Plan:
IntList.h:
- Include the required specifications into the program.
- Define a class template named “IntList”.
- Declare the member variables “value” and “*next” in structure named “ListNode”.
- Declare the constructor, copy constructor, destructor, and member functions in the class.
- Declare a class template and define a function named “appendNode()” to insert the node at end of the list.
- Declare the structure pointer variables “newNode” and “dataPtr” for the structure named “ListNode”.
- Assign the value “num” to the variable “newNode” and assign null to the variable “newNode”.
- Using “if…else” condition check whether the list is empty or not, if the “head” is empty then make a new node into “head” pointer. Otherwise, make a loop to find last node in the loop.
- Assign the value of “newNode” into the variable “dataPtr”.
- Declare a class template and define a function named “print()” to print the values in the list.
- Declare the structure pointer “dataPtr” for the structure named “ListNode”.
- Initialize the variable “dataPtr” with the “head” pointer.
- Check whether the list is empty or not; if the list is empty then display the values of the list.
- Declare a class template and define a function named “insertNode()” used to insert a value into the list.
- Declare the structure pointer variables “newNode”, “dataPtr”, and “prev” for the structure named “ListNode”.
- Make a “newNode” value into the received variable value “num”.
- Using “if…else” condition to check whether the list is empty or not.
- If the list is empty then initialize “head” pointer with the value of “newNode” variable.
- Otherwise, make a “while” loop to test whether the “num” value is less than the list values or not.
- Use “if…else” condition to initialize the value into list.
- Declare a class template and define a function named “deleteNode()” to delete a value from the list.
- Declare the pointer variables “dataPtr”, and “prev” for the structure named “ListNode”.
- Using “if…else” condition to check whether the “head” value is equal to “num” or not.
- Initialize the variable “dataPtr” with the value of the variable “head”.
- Remove the value using “delete” operator and reassign the “head” value into the “dataPtr”.
- If the “num” value not equal to the “head” value, then define the “while” loop to assign the “dataPtr” into “prev”.
- Use “if” condition to delete the “prev” pointer.
- Declare a class template and define a function named “getTotal()” to calculate total value in a list.
- Define a variable named “total” and initialize it to “0” in type of template.
- Define a pointer variable “nodePtr” for the structure “ListNode” and initialize it to be “NULL”.
- Assign the value of “head” pointer into “nodePtr”.
- Define a “while” loop to calculate “total” value of the list.
- Return a value of “total” to the called function.
- Declare a class template and define a function named “numNodes()” to find the number of values that are presented in the list.
- Declare a variable named “count” in type of “integer”.
- Define a pointer variable “nodePtr” and initialize it to be “NULL”.
- Assign a pointer variable “head” to the “nodePtr”.
- Define a “while” loop to traverse and count the number of elements in the list.
- Declare a class template and define a function named “getAverage()”to find an average value of elements that are presented in list.
- Declare a class template and define a function named “getLargest()”to find largest element in the list.
- Declare a template variable “largest” and pointer variable “nodePtr” for the structure.
- Using “if” condition, assign the value of “head” into “largest” variable.
- Using “while” loop, traverse the list until list will be empty.
- Using “if” condition, check whether the value of “nodePtr” is greater than the value of “largest” or not.
- Assign address of “nodePtr” into “nodePtr”.
- Return a value of “largest” variable to the called function.
- Declare a class template and define a function named “getSmallest()” to find largest element in the list.
- Declare a template variable “smallest” and pointer variable “nodePtr” for the structure.
- Using “if” condition, assign the value of “head” into “smallest” variable.
- Using “while” loop, traverse the list until list will be empty.
- Using “if” condition, check the value of “nodePtr” is smaller than the value of “smallest”.
- Assign address of “nodePtr” into “nodePtr”.
- Return a value of “smallest” variable to the called function.
- Declare a class template and define a function named “getSmallestPosition()” to find the position of smallest value in the list.
- Declare a template variable “smallest” and pointer variable “nodePtr” for the structure.
- Using “while” loop traverses the list until the list will be empty.
- Using “if” condition, find the position of “smallest” value in the list.
- Return the value of “position” to the called function.
- Declare a class template and define a function named “getLargestPosition()” to find the position of largest value in the list.
- Declare a template variable “largest” and pointer variable “nodePtr” for the structure.
- Using “while” loop traverses the list until the list will be empty.
- Using “if” condition, find the position of “largest” value in the list.
- Return the value of “position” to the called function.
- Define the destructor to destroy the values in the list.
- Declare the structure pointer variables “dataPtr”, and “nextNode” for the structure named “ListNode”.
- Initialize the “head” value into the “dataPtr”.
- Define a “while” loop to make the links of node into “nextNode” and remove the node using “delete” operator.
main.cpp:
- Include the required header files into the program.
- Declare a variable “months” in type of integer.
- Read the value of “months” from user and using “while” loop to validate the data entered by user.
- Declare an object named “rainfall” for the class “IntList”.
- Using “for” loop, read an input for every month from user.
- Append the value entered from user into the list.
- Make a call to “getTotal()”, “getAverage()”, “getLargest()”, “getSmallest()”, “getLargestPosition()”, and “getSmallestPosition()” function and display the values on the screen.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What advantages does a List have over an array?
Sets store their elements in an unordered fashion.True or False
Using C Sharp, Declare an array animals with 5 animals in it such as “dog”, “cat”, etc. After the declaration write the code to add another animal to the array. Write the code to add each animal to a list box called lstAnimals.
Chapter 18 Solutions
STARTING OUT WITH C++ REVEL >IA<
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - Prob. 18.2CPCh. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.2 - Prob. 18.5CPCh. 18.2 - Prob. 18.6CPCh. 18.2 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - Prob. 3RQECh. 18 - Prob. 4RQECh. 18 - Prob. 5RQECh. 18 - Prob. 6RQECh. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 19RQECh. 18 - Prob. 20RQECh. 18 - Prob. 21RQECh. 18 - Prob. 22RQECh. 18 - Prob. 23RQECh. 18 - Prob. 24RQECh. 18 - Prob. 25RQECh. 18 - T F The programmer must know in advance how many...Ch. 18 - T F It is not necessary for each node in a linked...Ch. 18 - Prob. 28RQECh. 18 - Prob. 29RQECh. 18 - Prob. 30RQECh. 18 - Prob. 31RQECh. 18 - Prob. 32RQECh. 18 - Prob. 33RQECh. 18 - Prob. 34RQECh. 18 - Prob. 35RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - List Template Create a list class template based...Ch. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- Assignment: Change the styles of ordered list and unordered listarrow_forwardQuestion: struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad. Question: struct node { char *name; int marks; node *next; }; By using the above declaration for the Linked List, write a program in C++ to display the summary report regarding the pass/fail ratio of the subject Data Structure for the class BSI-3 that have only 15 students. The required Report Summary for the exam will be like wise: The No. of A grades........??? The No. of B grades........??? And so on by applying the grading criteria of COMSATS-University Islamabad.arrow_forwardCapital QuizWrite a program that creates a dictionary containing the U.S. states as keys, and their capitals as values. (Use the Internet to get a list of the states and their capitals.) The programshould then randomly quiz the user by displaying the name of a state and asking the userto enter that state’s capital. The program should keep a count of the number of correct andincorrect responses. (As an alternative to the U.S. states, the program can use the names ofcountries and their capitals.) in phytonarrow_forward
- The last part of the code is not completed. public static void displayArray(int[] list) { System.out.println("The sum resulting array is" + Arrays.toString(list)); } }arrow_forwardQuestion1: define two lists of numbers (arrays) and ask the user to give the size of each, and then ask the user to fill the list(according to a size that he gave), then compare two arrays and determine whether they are equal? Note: two arrays are equal if all elements are equal. (Array not vector )arrow_forwardDefine the function (siftNum lst). This function should resolve to all the numbers in the list, lst. For example: (siftNum '(a 8 2 1 m l 90 p 2)) resolves to '(8 2 1 1 90 2).arrow_forward
- Max Absolute In List Function Lab Description Implement function max_abs_val(lst), which returns the maximum absolutevalue of the elements in list.For example, given a list lst: [-19, -3, 20, -1, 0, -25], the functionshould return 25. The name of the method should be max_abs_val and the method should take one parameter which is the list of values to test. Here is an example call to the function print(max_abs_val([-19, -3, 20, -1, 0, -25])) File Name maxabsinlst.py Score There are three tests each worth 2 points Note: You do not need any other code including the main method or any print statements. ONLY the max_abs_val method is required. Otherwise, the autograder will fail and be unable to grade your code. (I.e., do not include the above example in your code.) The above example should be used be test your code but deleted or comment out upon submission. PYTHON LABarrow_forwardData Structure and algorithms ( in Java ) Please solve it urgent basis: Make a programe in Java with complete comments detail and attach outputs image: Question is inside the image also: a). Write a function to insert elements in the sorted manner in the linked list. This means that the elements of the list will always be in ascending order, whenever you insert the data. For example, After calling insert method with the given data your list should be as follows: Insert 50 List:- 50 Insert 40 List:- 40 50 Insert 25 List:- 25 40 50 Insert 35 List:- 25 35 40 50 Insert 40 List:- 25 35 40 40 50 Insert 70 List:- 25 35 40 50 70 b). Write a program…arrow_forwardstats.py: We can create a numpy array from separate lists by specifying a format for each field:arr = np.zeros(4, dtype={'names': ('name', 'age', 'major', 'gpa'), 'formats': ('U50', 'i4', 'U4', 'f8')})arr['name'] = ['Alice', 'Bob', 'Carol', 'Dennis']arr['age'] = [21, 25, 18, 29]arr['major'] = ['CS', 'Math', 'Chem', 'Phys']arr['gpa'] = [3.8, 3.2, 4.0, 3.5] where U50 is a string of max length 50, i4 is a 4-byte integer, U4 is a string of max length 4, and f8 is an 8-byte floating-point number. Then arr has value array([('Alice', 21, 'CS', 3.8), ('Bob', 25, 'CS', 3.2),('Carol', 18, 'Chem', 4. ), ('Dennis', 29, 'Phys', 3.5)],dtype=[('name', '<U50'), ('age', '<i4'), ('major', '<U4'), ('gpa', '<f8')]) Then, for example, arr[’name’] will give an array of just the student names.Write a program that reads the file roster2.dat that has this format: name,age,major,gpa Convert this to a numpy array as shown above. Then use that array to compute…arrow_forward
- Linked list is more suitable data structure than array for a program that requires a lot of insert/erase operations.True or False.arrow_forwardRemove Char This function will be given a list of strings and a character. You must remove all occurrences of the character from each string in the list. The function should return the list of strings with the character removed. Signature: public static ArrayList<String> removeChar(String pattern, ArrayList<String> list) Example:list: ['adndj', 'adjdlaa', 'aa', 'djoe']pattern: a Output: ['dndj', 'djdl', '', 'djoe']arrow_forwardLab Goal : This lab was designed to teach you more about sorting data with the built-in java sorts. Lab Description : Take a list of words and output the list in ascending order. Use Arrays.sort().Sample Data : abc ABC 12321 fred alexandera zebra friendly acrobatics 435 TONER PRinTeRb x 4 r s y $123 ABC abc 034 dog cat sally sue bob 2a2Sample Output : word 0 :: 12321word 1 :: ABCword 2 :: abcword 3 :: alexanderword 4 :: fred word 0 :: 435word 1 :: PRinTeRword 2 :: TONERword 3 :: aword 4 :: acrobaticsword 5 :: friendlyword 6 :: zebra word 0 :: $word 1 :: 4word 2 :: bword 3 :: rword 4 :: sword 5 :: xword 6 :: y word 0 :: 034word 1 :: 123word 2 :: 2a2word 3 :: ABCword 4 :: abcword 5 :: bobword 6 :: catword 7 :: dogword 8 :: sallyword 9 :: sue I need a code class and a runner classarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
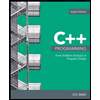
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
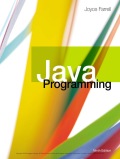
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
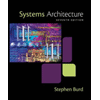
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning