STARTING OUT WITH C++ REVEL >IA<
9th Edition
ISBN: 9780135853115
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 9PC
Program Plan Intro
File Reverser
Program Plan:
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Create an object “file1” for input file stream.
- Create an object “file2” for output file stream.
- Declare a class template
- Declare character variables named “popChar”, and “ch”.
- Till the end of file,
- Push each character into the stack using the function “push ()”.
- Close “file1”.
- Until the stack gets empty,
- Pop the character stack and write it on “file2” using the function “pop ()”.
- Close “file2”.
- Open “file2”.
- Till the end of file,
- Get a character and print it on the console screen.
DynStack.h:
- Include required header files.
- Create a template.
- Declare a class named “DynStack”. Inside the class
- Inside the “private” access specifier,
- Give structure declaration for the stack
- Create an object for the template
- Create a stack pointer name “next”.
- Create a stack pointer name “top”
- Give structure declaration for the stack
- Inside the “public” access specifier,
- Give a declaration for a constructor.
- Assign null to the top node.
- Give function declaration for “push ()”, “pop ()”,and “isEmpty ()”.
- Give a declaration for a constructor.
- Inside the “private” access specifier,
- Give the class template.
- Give function definition for “push ()”.
- Assign null to the new node.
- Dynamically allocate memory for new node
- Assign “num” to the value of new node.
- Check if the stack is empty using the function “isEmpty ()”
- If the condition is true then assign new node as the top and make the next node as null.
- If the condition is not true then, assign top node to the next of new node and assign new node as the top.
- Give the class template.
- Give function definition for “pop ()”.
- Assign null to the temp node.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign top value to the variable “num”.
- Link top of next node to temp node.
- Delete the top node and make temp as the top node.
- Give function definition for “isEmpty ()”.
- Assign Boolean value to the variable
- Check if the top node is null
- Assign true to “status”.
- Return the status
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In this assignment you are going to create an application that reverses a text file and saves it. This application is a single file application so you do not need to think about additional classes; you will simply have a main.cpp file.
Your application should accomplish:
In main, create a stack. Then, open a text file and read its contents into a stack of characters..
The program should then pop the characters from the stack and save them in another text file.
As this is a stack, (LIFO, so last item you put into the stack gets the first one to come out), the new file will be the reverse of the other. So if the text file at first said KHAS, the new file must have SAHK.
File Encryption and DecryptionWrite a program that uses a dictionary to assign “codes” to each letter of the alphabet. Forexample:codes = { ‘A’ : ‘%’, ‘a’ : ‘9’, ‘B’ : ‘@’, ‘b’ : ‘#’, etc . . .}Using this example, the letter A would be assigned the symbol %, the letter a would beassigned the number 9, the letter B would be assigned the symbol @, and so forth.The program should open a specified text file, read its contents, then use the dictionaryto write an encrypted version of the file’s contents to a second file. Each character in thesecond file should contain the code for the corresponding character in the first file.Write a second program that opens an encrypted file and displays its decrypted contentson the screen.
in phyton
Create class Test in a file named Test.java. This class contains a main program that
performs the following actions:
Instantiate a doubly linked list.
Insert strings “a”, “b”, and “c” at the head of the list using three Insert() operations. The state of the list is now [“c”, “b”, “a”].
Set the current element to the second-to-last element with a call to Tail() followed by a call to Previous()Then insert string “d”. The state of the list is now [“c”, “d”, “b”, “a”].
Set the current element to past-the-end with a call to Tail() followed by a call to Next(). Then insert string “e”. The state of the list is now [“c”, “d”, “b”, “a”, “e”] .
Print the list with a call to Print() and verify that the state of the list is correct.
Chapter 19 Solutions
STARTING OUT WITH C++ REVEL >IA<
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. public void ReverseStack(){ }arrow_forwardLINKED LIST IN PYTHON Create a program using Python for the different operations of a Linked List. Your program will ask the user to choose an operation. 1. Create a List -Ask the user how many nodes he/she wants. -Enter the element/s -Display the list -Back to menu 2. Add at beginning -Ask for the element to be inserted. -Display the list -Back to menu 3. Add after -Ask for the element to be inserted. -Ask for the position AFTER which the element is to be inserted -Display the list -Back to menu 4. Delete -Ask for the element (data) to be deleted -If found, delete the node with that data. -If multiple values, delete only the first element found -If not found, display that the element is not found -back to menu 5. Display -Display the list 6. Count -Display the number of elements 7. Reverse -Reverse the list and display it 8. Search -Ask the user for the element (data) to be searched -Display a message if the element is found or not 9. Quit -Exits the programarrow_forwardlinked_list_stack_shopping_list_manager.py This is a file that includes the class of linked list based shopping list manager. This class contains such methods as init, insert_item, is_list_empty, print_item_recursive_from_top, print_items_from_top, print_item_recursive_from_bottom, print_items_from_bottom, getLastItem, removeLastItem. In addition, this class requires the inner class to hold onto data as a linked list based on Stack. DO NOT use standard python library, such as deque from the collections module. Please keep in mind the following notes for each method during implementation: Init(): initializes linked list based on Stack object to be used throughout object life. insert_item(item): inserts item at the front of the linked list based on Stack. Parameters: item name. is_list_empty (): checks if the current Stack object is empty (ex. check if head is None). print_item_recursive_from_top(currentNode): a helper method to print linked list based on Stack item recursively. Note:…arrow_forward
- 1. In a loop, fill an array for up to 50 even numbers and a set for up to 50 odd numbers. Use the merge() algorithm to merge these containers into a vector. Display the vector contents to show that all went well. (In C++ language) 2. Write a program that copies a source file of integers to a destination file, using stream iterators. The user should supply both source and destination filenames to the program. You can use a while loop approach and a list container with integer data type. Within the loop, read each integer value from the input iterator and add the value to the list container by using push_back() function, then increment the input iterator.Finally, use an output iterator to write the list to the destination file. (In C++ language)arrow_forwardContact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe 123-5432 Linda…arrow_forwardOCaml Code: I need help with writing the push and pop statements in order to create an interpreter for OCaml. I need help with writing the print statement as well. The println command pops a string off the top of the stack and writes it, followed by a newline, to the output file that is specified as the second argument to the interpreter function. In the case that the top element on the stack is not a string, it should be returned to the stack and an :error: pushed. If the stack is empty, an :error: shall be pushed. Below is the unfinished code. Make sure to use the test cases as well and show the screenshots of the code passing the test cases. Attached is info. for push and pop. interpreter.ml type stackValue = BOOL of bool | INT of int | ERROR | STRING of string | NAME of string | UNIT type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP of stackValue | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT let interpreter (input, output) = let ic = open_in input in…arrow_forward
- Which variable is appropriate to track the insertion position to a stack?arrow_forwardC# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. private int [] stack; private int size; public void ReverseStack(){ }arrow_forwardStacks 1- Write a Python function that takes a user input of a word and returns True if it is a Palindrome and returns False otherwise (Your function should use a Stack data structure). A palindrome is a word that can be read the same backward as forward. Some examples of palindromic words are noon, civic, radar, level, rotor, kayak, reviver, racecar, redder, madam, and refer. 2- Write a Python function that takes a stack of integer numbers and returns the maximum value of the numbers in the stack. The stack should have the same numbers before and after calling the function. 3- Write a main function that tests the functions you wrote in 1 and 2 above and make sure that your code is well documented.arrow_forward
- (C Language) A photographer is organizing a photo collection about the national parks in the US and would like to annotate the information about each of the photos into a separate set of files. Write a program that reads the name of a text file containing a list of photo file names. The program then reads the photo file names from the text file, replaces the "_photo.jpg" portion of the file names with "_info.txt", and outputs the modified file names. Assume the unchanged portion of the photo file names contains only letters and numbers, and the text file stores one photo file name per line. If the text file is empty, the program produces no output. Assume also the maximum number of characters of all file names is 100.arrow_forwardC++ Write a program that checks the spelling of all words in a file. It should read each word of a file and check whether it is contained in a word list. A word list is available on most UNIX systems (including Linux and Mac OS X) in the file /usr/share/dict/words. (If you don’t have access to a UNIX system, you can find a copy of the file on the Internet by searching for /usr/share/dict/words.) The program should print out all words that it cannot find in the word list. Follow this pseudocode: Open the dictionary file. Define a vector of strings called words. For each word in the dictionary file Append the word to the words vector. Open the file to be checked. For each word in that file If the word is not contained in the words vector Print the word.arrow_forwardCreate an application that reads a statement from the user and outputs it with the characters of each word reversed. To reverse the characters in each word, use a stack.Create an application that reads a statement from the user and outputs it with the characters of each word reversed. To reverse the characters in each word, use a stack.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
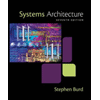
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning