STARTING OUT WITH C++ REVEL >IA<
9th Edition
ISBN: 9780135853115
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 19, Problem 1PC
Program Plan Intro
Static Stack Template
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object named “stck” for stack.
- Declare a variable named “popElem”.
- Push 5 elements inside the stack using the function “push_Elem ()”.
- Pop 5 elements from the stack using the function “pop_Elem ()”.
Stack.h:
- Include required header files.
- Create a template.
- Declare a class named “Stack”. Inside the class
- Inside the “private” access specifier,
- Create an object for the template
- Declare the variables “stackSize” and “top_Elem”.
- Inside the “public” access specifier,
- Give a declaration for an overloaded constructor.
- Give function declaration for “push_Elem ()”, “pop_Elem ()”, “is_Full ()”, and “is_Empty ()”.
- Inside the “private” access specifier,
- Give function definition for the overloaded constructor.
- Create stack size
- Assign the value to the “stackSize”.
- Assign -1 to the variable “top_Elem”
- Give function definition for “push_Elem ()”.
- Check if the stack is full using the function “is_Full ()”
- If the condition is true then print “The stack is full”.
- If the condition is not true then,
- Increment the variable “top_Elem”.
- Assign the element to the top position.
- Check if the stack is full using the function “is_Full ()”
- Give function definition for “pop_Elem ()”.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign the element to the variable “num”.
- Decrement the variable “top_Elem”.
- Check if the stack is empty using the function “is_Empty ()”
- Give function definition for “is_Full ()”.
- Assign Boolean value to the variable
- Check if the top and the stack size is same
- Assign true to “status”.
- Return the status
- Give function definition for “is_Empty ()”.
- Assign Boolean value to the variable
- Check if the top is equal to -1.
- Assign true to “status”.
- Return the status
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
static binding,a) Allocate stack memoryb) allocate static memoryWhat is the advantage of a) compared to b)?
Apply the Stack Applications algorithms in c++. You have to implement the stack using the static array or the linked list.
You have to create a project for each algorithm.
Add a screenshot of the output for each project.
1. Assume the existence of a class Stack whose implementation is unknown (array versus nodes), withoperationspushandpop. Write a Stack methodvoid reverseStack()that reverses the order of theelements contained in the Stack. You can assume the existence of any other ADT data structures andtheir operations.
Chapter 19 Solutions
STARTING OUT WITH C++ REVEL >IA<
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Similar questions
- Simple stack implementation please help (looks like a lot but actually isnt, everything is already set up for you below to make it even easier) help in any part you can, please be clear, thank you Given an interface for Stack- Without using the java collections interface (ie do not import java.util.List,LinkedList, Stack, Queue...)- Create an implementation of Stack interface provided- For the implementation create a tester to verify the implementation of thatdata structure performs as expected Get on the bus – Stack (lifo)- Implement the provided Stack interface ( fill out the implementation shell)- Put your implementation through its paces by exercising each of the methods ina test harness- Add to your ‘BusClient’ the following functionality using your Stack-o Create (push) 6 riders by name§ Iterate over the stack, print all riderso Peek at the stack / print the resulto Remove (pop) the top of the stack§ Iterate over the stack, print all riderso Peek at the stack / print the resulto…arrow_forwardCompulsory Task Follow these steps: ● For this task, you are required to refactor the badly written program RPN.java. This program is a Reverse-Polish Notation calculator which uses a stack. A Reverse-Polish Notation calculator is a calculator that will calculate equations where the operator follows the operands. Therefore, instead of inputting an equation as “1 + 2”, a Reverse-Polish Notation calculator would take the following input “ 1 2 +”. A stack is a data structure in which items are added to the top of the stack and removed from the top of the stack. It is therefore known as a last-in, first-out (LIFO) data structure. Stack terminology: ○ Push — is an operation that adds an item to the top of a stack. ○ Pop — is an operation that removes an item from the top of a stack. The pseudocode for this program is: ○ Get an equation (e.g. 2 3 +) from the user as input. ○ Loop through the string value input by the user. ■ When you encounter a number (remember that numbers can include…arrow_forward7. Dynamic MathStack The MathStack class shown in this chapter has only two member functions: add and sub. Write the following additional member functions: Function Description mult Pops the top two values off the stack, multiplies them, and pushes their product onto the stack. div Pops the top two values off the stack, divides the second value by the first, and pushes the quotient onto the stack. addAll Pops all values off the stack, adds them, and pushes their sum onto the stack. multA11 Pops all values off the stack, multiplies them, and pushes their prod- uct onto the stack. Demonstrate the class with a driver program. here is the extention file please use it. // Specification file for the IntStack class #ifndef INTSTACK_H #define INTSTACK_H class IntStack { private: int *stackArray; // Pointer to the stack array int stackSize; // The stack size int top; // Indicates the top of the stack public: // Constructor IntStack(int); // Copy constructor…arrow_forward
- C# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. private int [] stack; private int size; public void ReverseStack(){ }arrow_forwardc++ code We know that stack is used to manage function calls. Whenever a function is called, the local variables,and return address is pushed on to the stack. Once the function completes its execution the returnaddress and local variables are popped from the stack. Your task is to provide an array-based stackimplementation to manage the function calls.Data Members and Methods:string * arr (This array should be used to store the return address against each function call)int size (size of array).int count (count of elements pushed on stack).parameterized constructor, it should receive the size and creates an array of that fixed size.isFull function, it should return 1 if the stack is full otherwise 0.push function, receive and inserts the element into the stack. If the stack is full call the reSize function.isEmpty function, it should return 1 if the stack is empty otherwise 0.pop function, removes the element from stack. if the stack is empty than return "-1".reSize…arrow_forward3.Write a generic class called GenericStack<T> that represents a stack structure. A stack structure follow the strategy last-in-first-out, which means that the last element added to the stack, is the first to be taken out. The GenericStack class has the following attributes and methods: --An attribute ArrayList<T> elements which represents the elements of the stack.(All of you refer collection framework for ArrayList. or you can use an array to hold the elements of Stack.)[Refer the following links to have intro on ArrayList: https://www.w3schools.com/java/java_arraylist.asp, https://www.geeksforgeeks.org/arraylist-in-java/] --A constructor that creates the ArrayList or an Array --A method push(T e) which adds the element to the ArrayList<T> or array. --A method pop() which removes the last element of the ArrayList<T> (last element added), if the list is not already empty and returns it. --A method print() which prints the elements of the stack starting from the…arrow_forward
- Working with Stacks 1. Create a method on the Stack class that determines whether a given value occurs consecutively in the stack. bool Stack::isConsecutive( const T & data ) const; Example: isConsecutive(…) function Result Stack isConsecutive (5) true top->(1) (1) (5) (3) (5) (5) (2) isConsecutive (3) false top->(1) (1) (5) (3) (5) (5) (5) (2) 2. Create a method on the Stack class that reverses the values on the stack. void Stack::reverse(); Example: reverse() function Stack original Result: Stack reversed reverse () top->(1) (1) (5) (3) (5) (5) (2) top->(2) (5) (5) (3) (5) (1) (1) reverse () top->(1) (1) (5) (3) (5) (5) (5) (2) top->(2) (5) (5) (5) (3) (5) (1) (1)arrow_forward3.4 Queue via Stacks: Implement a MyQueue class which implements a queue using two stacks.arrow_forwardStack Implementation in C++make code for an application that uses the StackX class to create a stack.includes a brief main() code to test this class.arrow_forward
- C++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow #include "stack.h" #include "linkedlist.h" // SLLStack means Singly Linked List (SLL) Stack class SLLStack : public Stack { LinkedList* list; public: SLLStack() { list = new LinkedList(); } void push(char e) { list->add(e); return; } char pop() { char elem; elem = list->removeTail(); return elem; } char top() { char elem; elem = list->get(size()); return elem; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } };arrow_forwardJava - For 7 and 8 use the following definition: class node { boolean data; node link; } 7. Consider a structure using a linked list in which you added and removed from the back of the list. Using the node definition above, write the pseudocode for a member method “add” to this structure. Assume the existence of a “front” and “back” reference. 8. Assume you are working with a stack implementation of the linked list definition above (above question #7), write a member method “pop”. The method should return a value (in the “popped” node). Assume the existence of the node references called “TheStack” and “Top”. These references point to the start (or bottom) and top of the stack (or back of the list).arrow_forwardC++ The List class represents a linked list of dynamically allocated elements. The list has only one member variable head which is a pointer that leads to the first element. See the following code for the destructor to List. ~ List () { for (int i = 0; i <size (); i ++) { pop_back (); } } What problems does the destructor have? Select one or more options: 1. There are no parameters for the destructor. 2. The return value from pop_back (if any) is nerver handled. 3. The destructor will create a stack overflow. 4. The destructor will create dangling pointers. 5.The destructor will create memory leaks. 6.The destructor will create undefined behavior (equivalent to zero pointer exception). 7.The condition must be: i <size () - 1 8. There is at least one problem with the destructor, but none of the above.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
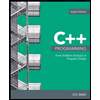
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning