STARTING OUT WITH C++ REVEL >IA<
9th Edition
ISBN: 9780135853115
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 7PC
Program Plan Intro
Dynamic MathStack
Program Plan:
MathStack.h:
- Include required header files
- Declare a class named “MathStack”. Inside the class,
- Inside “public” access specifier,
- Declare functions “add ()”, “sub ()”, “mult ()”, “div ()”, “addAll ()”, and “multAll ()”.
- Inside “public” access specifier,
MathStack.cpp:
- Include required header files.
- Give function definition to add elements “add()”.
- Declare required variables “number”, and “sum_Value”.
- Call the function “pop()”.
- Add the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to subtract elements “sub()”.
- Declare required variables “number”, and “diff_Value”.
- Call the function “pop()”.
- Subtract the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to multiply elements “mult()”.
- Declare required variables “number”, and “prod_Value”.
- Call the function “pop()”.
- Multiply the elements.
- Push the value into the stack using the function “push ()”.
- Give function definition to divide elements “div()”.
- Declare required variables “number”, and “quo_Value”.
- Call the function “pop()”.
- Divide the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to add all the elements “addAll()”.
- Declare required variables “number”, and “sum_Value”.
- Call the function “pop()”.
- Add all the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to multiply all the elements “multAll()”.
- Declare required variables “number”, and “prod_Value”.
- Call the function “pop()”.
- Multiply all the elements.
- Push the value into the stack using the function “push()”.
IntStack.h:
- Include required files.
- Declare a class named “IntStack”. Inside the class,
- Inside “protected” access specifier,
- Declare a pointer named “stackArray”.
- Declare variables “stackSize” and “top”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Give function declarations.
- Inside “protected” access specifier,
IntStack.cpp:
- Declare required header files.
- Give definition for constructor,
- Create a stack array and assign the size
- Give definition for destructor,
- Delete the array and assign it to null
- Give function definition to push elements “push()”
- Check if the stack is full using the function “isFull()”,
- If the condition is true then, print “The stack is full”.
- If the condition is not true then,
- Increment the variable.
- Assign “num” to the top position.
- Check if the stack is full using the function “isFull()”,
- Give function definition to pop elements “pop ()”,
- Check if the stack is empty using the function “isEmpty()”.
- If the condition is true then, print “The stack is empty”.
- Assign top element to the variable.
- Decrement the variable.
- If the condition is true then, print “The stack is empty”.
- Check if the stack is empty using the function “isEmpty()”.
- Give function definition to check if the stack is full “isFull()”.
- Assign “false” to the Boolean variable “status”.
- Check if the stack size if full.
- Assign true
- Return the Boolean variable “status”.
- Give function definition to check if the stack is empty “isEmpty()”.
- Assign “false” to the Boolean variable
- Check if top is empty
- Assign true.
- Return the variable
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Declare constant variables “STACKSIZE”, “ADDSIZE”, and “MULTSIZE”.
- Create three stacks “stack”, “addAllStack”, and “multAllStack”.
- Declare a variable “popVar”.
- Push two elements to perform add operation.
- Call the function “add()”.
- Display the element.
- Push two elements to perform multiplication operation.
- Call the function “mult()”.
- Display the element.
- Push two elements to perform division operation.
- Call the function “div()”.
- Display the element.
- Push two elements to perform subtraction operation.
- Call the function “sub()”.
- Display the element.
- Push four elements to perform addAll operation.
- Call the function “addAll()”.
- Display the element.
- Push six elements to perform multAll operation.
- Call the function “multAll()”.
- Display the element.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
7. Dynamic MathStack The MathStack class shown in this chapter has only two member functions: add and sub. Write the following additional member functions: Function Description mult Pops the top two values off the stack, multiplies them, and pushes their product onto the stack. div Pops the top two values off the stack, divides the second value by the first, and pushes the quotient onto the stack. addAll Pops all values off the stack, adds them, and pushes their sum onto the stack. multA11 Pops all values off the stack, multiplies them, and pushes their prod- uct onto the stack. Demonstrate the class with a driver program.
here is the extention file please use it.
// Specification file for the IntStack class
#ifndef INTSTACK_H
#define INTSTACK_H
class IntStack
{
private:
int *stackArray; // Pointer to the stack array
int stackSize; // The stack size
int top; // Indicates the top of the stack
public:
// Constructor
IntStack(int);
// Copy constructor…
C#
Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function.
This would occur in the NumberStack class, not the main class. These are the provided variables:
private int [] stack;private int size;
Create a method that will reverse the stack when put into the main class.
Use Python for this question. Also please comment what each line of code means for this question as well:
Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!)
Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top.
push() – take an item as input and push it on the top of the stack
pop() – remove and return the item at the top of the stack
isEmpty() – returns True if the stack is empty,…
Chapter 19 Solutions
STARTING OUT WITH C++ REVEL >IA<
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java All classes must be named as shown in the above UML diagram except the concrete child classes. The ItemList is a singly-linked list with the following methods: • insert(index: int, item: Item) : boolean o Inserts the given item at the given index if an equal item is not already in the list. If the method is successful it returns true. If an equal item already exists in the list or the index is out of range, this method returns false. • add(item: Item): boolean o Adds the given item to the front of the list. Returns true if successful and false if an equal item is already in the list. • set(index: int, item: Item) : Item o Replaces the object in the list at the given index with this object. If the index is out of range or the given item is already in the list, the method returns null. If the operation is successful, the method returns the object that was replaced by this one. • delete(index: int) : Item o Deletes the object at the given index from the list. If the index is out of…arrow_forwardWrite JAVA code General Problem Description: It is desired to develop a directory application based on the use of a double-linked list data structure, in which students are kept in order according to their student number. In this context, write the Java codes that will meet the requirements given in detail below. Requirements: Create a class named Student to represent students. In the Student class; student number, name and surname and phone numbers for communication are kept. Student's multiple phones number (multiple mobile phones, home phones, etc.) so phone numbers information will be stored in an “ArrayList”. In the Student class; parameterless, taking all parameters and It is sufficient to have 3 constructor methods, including a copy constructor, get/set methods and toString.arrow_forwardC++ ProgrammingTopic: stacks queues and dequesBelow is the initial program of the main file, only modify the main file, sllstack file also provided for the reference of the main. See attached photo for instructions. main.cpp #include <iostream> #include <cstring> #include "sllstack.h" using namespace std; int main(int argc, char** argv) { SLLStack* stack = new SLLStack(); int test; string str; cin >> test; switch (test) { case 0: getline(cin, str); // PERFORM SOLUTION TO BRACKETS PROBLEM HERE // FYI: Place your variable declarations, if any, before switch. break; case 1: stack->push('a'); stack->push('b'); stack->push('c'); cout << stack->pop() << endl; cout << stack->pop() << endl; cout << stack->pop() << endl; cout << stack->isEmpty()…arrow_forward
- Part 2. Library Class Implement a class, Library, as described in the class diagram below. Library must implement the Comparable interface. The compareTo() method must compare the branch names and only the branch names. The comparison must be case insensitive. The equals() method must compare the branch names and only the branch names. The comparison must be case insensitive. Be sure to test the equals() and compareTo() methods before proceeding. Library - state: String - branch: String - city: String - zip: String - county: String - int squareFeet: int - int hoursOpen: int - int weeksOpen: int + Library(state: String, branch: String, city: String, zip: String, county: String, squareFeet: int, hoursOpen: int, weeksOpen: int) + getState(): String + getBranch(): String + getCity(): String + getZip(): String + getCounty(): String + getSquareFeet(): int + getHoursOpen(): int + getWeeksOpen(): int + setState(state: String): void + setBranch(branch: String):void +…arrow_forwardCompulsory Task Follow these steps: ● For this task, you are required to refactor the badly written program RPN.java. This program is a Reverse-Polish Notation calculator which uses a stack. A Reverse-Polish Notation calculator is a calculator that will calculate equations where the operator follows the operands. Therefore, instead of inputting an equation as “1 + 2”, a Reverse-Polish Notation calculator would take the following input “ 1 2 +”. A stack is a data structure in which items are added to the top of the stack and removed from the top of the stack. It is therefore known as a last-in, first-out (LIFO) data structure. Stack terminology: ○ Push — is an operation that adds an item to the top of a stack. ○ Pop — is an operation that removes an item from the top of a stack. The pseudocode for this program is: ○ Get an equation (e.g. 2 3 +) from the user as input. ○ Loop through the string value input by the user. ■ When you encounter a number (remember that numbers can include…arrow_forwardDesign and implement an application that reads a sentence fromthe user and prints the sentence with the characters of each wordbackwards. Use a stack to reverse the characters of each word.8. Pop the infix expression off the stack and print it.arrow_forward
- 44 Computer Science In JAVA (not javascript) Build a class to encapsulate and implement the ADT of the problem below. Include instance variables, if it has public methods, and other classes if needed. Incude a test driver The ADT in this case is a Stack. Write the code for this stack. You are hired to help design software to help with a key train operation: processing food orders on a trip. Once the manager says that it is ok, train staff to walk from the back of the plane to the front taking everyone’s order. When they reach the front, they begin preparing those orders. Food orders are prepared in groups of 10, that being the number of cups that can fit onto a carrying tray. The first group whose food orders are prepared are those in the front of the plane (first class). The second group is the next 10 people further back in the plane and so on. You are in charge of developing a new application that will let train staff take food orders and then display those orders 10 at a…arrow_forwardC# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. private int [] stack; private int size; public void ReverseStack(){ }arrow_forwardC++ ProgrammingTopic: Stack, Ques and DequesBelow is the initial program of the main file, only modify the main file, sllstack file also provided. See attached photo for instructions. main.cpp #include <iostream> #include <cstring> #include "sllstack.h" using namespace std; int main(int argc, char** argv) { SLLStack* stack = new SLLStack(); int test; string str; cin >> test; switch (test) { case 0: getline(cin, str); // PERFORM SOLUTION TO BRACKETS PROBLEM HERE // FYI: Place your variable declarations, if any, before switch. break; case 1: stack->push('a'); stack->push('b'); stack->push('c'); cout << stack->pop() << endl; cout << stack->pop() << endl; cout << stack->pop() << endl; cout << stack->isEmpty() << endl; break;…arrow_forward
- C# Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function. public void ReverseStack(){ }arrow_forward(Card Shuffling and Dealing) Create a program to shuffle and deal a deck of cards. Theprogram should consist of class Card, class DeckOfCards and a driver program. Class Card shouldprovide:a) Data members face and suit of type int.b) A constructor that receives two ints representing the face and suit and uses them to initialize the data members.c) Two static arrays of strings representing the faces and suits.d) A toString function that returns the Card as a string in the form “face of suit.” Youcan use the + operator to concatenate strings.Class DeckOfCards should contain:a) An array of Cards named deck to store the Cards.b) An integer currentCard representing the next card to deal.c) A default constructor that initializes the Cards in the deck.d) A shuffle function that shuffles the Cards in the deck. The shuffle algorithm shoulditerate through the array of Cards. For each Card, randomly select another Card in thedeck and swap the two Cards.e) A dealCard function that returns the next…arrow_forwardC++ Language C++ Code not working, what is the missing piece in this code? #include <iostream>#include <string>#include <vector>#include <stack>using namespace std; int main(){string x;stack < char, vector<char> > iStack;boolean status=true; cout << "Enter series of parentheses: ";getline(cin, x);for (int i=0; i<x.length(); i++)//measure the length of the string inputif (x[i] == '(' || x[i] == '{' || x[i] == '[')iStack.push(x[i]);else{ //if not opening grouping symbolif(x[i] == ']') {if(iStack.isEmpty() || iStack.top() != '[') {return false;}} else if (x[i] == ')') {if(iStack.isEmpty() || iStack.top() != '(') {return false;}} else if (x[i] == '}') {if(iStack.isEmpty() || iStack.top() != '{') {return false;} }iStack.pop();} if (iStack.empty())cout << "Balance!" << endl;elsecout << "Not Balance!" << endl;}arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
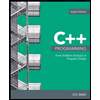
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage