STARTING OUT WITH C++ REVEL >IA<
9th Edition
ISBN: 9780135853115
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 3PC
Program Plan Intro
Static Queue Template
Program Plan:
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Declare a constant variable
- Declare a template
- Manipulate and insert elements into the queue using “enqueue ()” function.
- Delete the queue elements using “dequeue ()” function.
Queue.h:
- Include required header files.
- Create template class
- Declare a class named “Queue”. Inside the class,
- Inside the “private” access specifier,
- Create an object for the template
- Declare required variables.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue ()”, “dequeue ()”, “isEmpty ()”, “isFull ()”, and “clear ()”.
- Inside the “private” access specifier,
- Declare template class.
- Give definition for the constructor.
- Allocate memory dynamically for the template.
- Assign the values.
- Declare template class.
- Give definition for the destructor.
- Delete queue array and make it as null.
- Declare template class.
- Give function definition for “enqueue ()”.
- Check whether the queue is full using “isFull ()” function.
- If the condition is true then, print “The queue is full”.
- If the condition is not true then,
- Calculate the “rear” position.
- Assign “num” to the “queue_Array [rear]”.
- Increment the variable “numItems”.
- Check whether the queue is full using “isFull ()” function.
- Declare template class.
- Give function definition for “dequeue ()”.
- Check if the queue is empty using “isEmpty ()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Calculate “front” position.
- Assign the value of “queue_Array [front]” to the variable “num”.
- Decrement the variable “numItems”.
- Check if the queue is empty using “isEmpty ()” function.
- Declare template class.
- Give function definition for “isEmpty ()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “isFull ()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is less than “size”
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “clear ()”.
- Decrement queue size and assign it to “front”.
- Decrement queue size and assign it to “rear”.
- Assign 0 to the variable “numItems”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Answer the following by True or False:
NOTE: When the question has been underlined, it requires two (2) answers.
The ADT makes use of static array for its queue implementation.
The class includes a constructor and destructor.
A value must be provided when you initiate a dequeue operation.
True or False?
___________ The ADT makes use of static array for its queue implementation.
___________ ___________ The class includes a constructor and destructor.
___________ A value must be provided when you initiate a dequeue operation.
Add the operation queueCount to the class Queue, which returns the number of elements in thequeue. Write the definition of the function template to implement this operation.
Note: Code in C++
Chapter 19 Solutions
STARTING OUT WITH C++ REVEL >IA<
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- c++ Write a client function that returns the back of a queue while leaving the queue unchanged. This function can call any of the methods of the ADT queue. It can also create new queues. The return type is ITemType, and it accepts a queue as a parameter.arrow_forwardA double-ended queue or deque is a generalization of a stack and a queue that supports adding and removing items from either the front or the back of the data structure. This assignment has two parts: Part-1 Create a doubly linked list based DeQueDLL class that implements the DequeInterface. The class skeleton and interface are provided to you. Implement a String toString () method that creates and returns a string that correctly represents the current deque. Such a method could prove useful for testing and debugging the class and for testing and debugging applications that use the class. Assume each queued element already provides its own reasonable toString method. Part-2 Create an application program that gives a user the following three options to choose from – insert, delete, and quit. If the user selects ‘insert’, the program should accept the integer input from the user and insert it into the deque in a sorted manner. If the user selects ‘delete’, the program should…arrow_forwardCreate a Circular Queue Class and write a main() program to instantiate and use this class. You will use Arrays and Java PL for this implementation. Ensure that your class has the following attributes and methods: Attributes: rear, front and any others which you deem necessary Methods: queue(), enqueue(), dequeue(), isFull(), isEmpty(), peek()arrow_forward
- Part 3: Building a Point of Sales (POS) linked list data structure. In a POS system, a transaction is based on items purchased by the customer. The following is an example of a customer transaction receipt, where the prices shown in the receipt are GST inclusive. Write a linked list classes (one class for Node and another class for List), which store the items in the transaction. Test the classes by printing the items in the linked list and show the total price of the transaction. The following listing is the sample output for your reference: ============================== BC Items Price ============================== 10 Pagoda Gnut 110g 3.49 11 Hup Seng Cream Cracker 4.19 12 Yit Poh 2n1 Kopi-o 7.28 13 Zoelife SN & Seed 5.24 14 Gatsby S/FO Wet&Hard 16.99 15 GB W/G U/Hold 150g 6.49 ============================== Total (GST Incl.) 43.68…arrow_forwardC++ ProgrammingActivity: Queue Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. SEE ATTACHED PHOTO FOR THE PROBLEM #include "queue.h" #include "linkedlist.h" class SLLQueue : public Queue { LinkedList* list; public: SLLQueue() { list = new LinkedList(); } void enqueue(int e) { list->addTail(e); return; } int dequeue() { int elem; elem = list->removeHead(); return elem; } int first() { int elem; elem = list->get(1); return elem;; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } int collect(int max) { int sum = 0; while(first() != 0) { if(sum + first() <= max) { sum += first();…arrow_forwardC++ ProgrammingActivity: Queue Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. #include "queue.h" #include "linkedlist.h" class SLLQueue : public Queue { LinkedList* list; public: SLLQueue() { list = new LinkedList(); } void enqueue(int e) { list->addTail(e); return; } int dequeue() { int elem; elem = list->removeHead(); return elem; } int first() { int elem; elem = list->get(1); return elem;; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } int collect(int max) { int sum = 0; while(first() != 0) { if(sum + first() <= max) { sum += first(); dequeue(); } else {…arrow_forward
- Simple queue implementation please help (looks like a lot but actually isnt, everything is already set up for you to make it even easier) help in any part you can, please be clear, thank you Given an interface for Queue- Without using the java collections interface (ie do not import java.util.List,LinkedList, Stack, Queue...)- Create an implementation of Queue interface provided- For the implementation create a tester to verify the implementation of thatdata structure performs as expected Wait in line – Queue (fifo)- Implement the provided Queue interface ( fill out the implementation shell)- Put your implementation through its paces by exercising each of the methods ina test harness- Add to your ‘BusClient’ the following functionality using your Queue-o Create (enqueue) 6 riders by name§ Iterate over the queue, print all riderso Peek at the queue / print the resulto Remove (dequeue) the head of the queue§ Iterate over the queue, print all riderso Add two more riders to the queueo Peek…arrow_forward17 T OR F The QueueInterface interface represents a contract between the implementer of a Queue ADT and the programmer who uses the ADT.arrow_forwardQ. Difference between Stack and Queue Data Structures. Write down key differences. Write some code snippets. Draw images with some examples. Show differences for operations like push/pop, add/remove, etc.arrow_forward
- static binding,a) Allocate stack memoryb) allocate static memoryWhat is the advantage of a) compared to b)?arrow_forwardWrite a C++ program to perform the Queue operation. Note:Use the following class template for Queue creation. template <class T>class Queue{ private: int front,rear; T *queue; int maxsize;}; Define the following function in the class Queue class Method name Description int isFull() The method is used to check whether the queue is full or not. void insert(T) The method is used to display the rear element in the queue (if the queue is stored with the element(s)). void deletion() The method is used to delete the front element from the queue. void atFront() The method is used to display the front element in the queue (if the queue is stored with the element(s)). void atRear() The method is used to add data to the rear end of the queue. void display() The method is used to display all the data in the queue. int isEmpty() The method is used to check whether the queue is empty or not. Input and Output Format:The first line of input…arrow_forwardWhat is the relationship between a queue and its underlying array?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
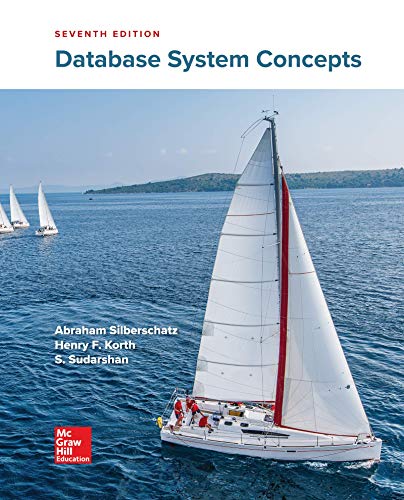
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
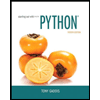
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
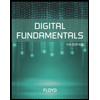
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
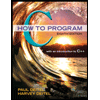
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
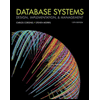
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
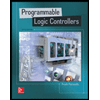
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education