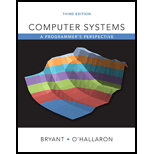
You are given the task of writing a function that will copy an integer val into a buffer buf, but it should do so only if enough space is available in the buffer.
Here is the code you write:
/* Copy integer into buffer if space is available */
/* WARNING: The following code is buggy */
void copy_int(int val; void *buf, int maxbytes) {
if (maxbytes-sizeof (val) >= 0)
memcpy(buf, (void *) &val, sizeof(val));
}
This code makes use of the library function memcpy. Although its use is a bit artificial here, where we simply want to copy an int, it illustrates an approach commonly used to copy larger data structures.
You carefully test the code and discover that it always copies the value to the buffer, even when maxbytes is too small.
- A. Explain why the conditional test in the code always succeeds. Hint: The sizeof operator returns a value of type size_t.
- B. Show how you can rewrite the conditional test to make it work properly.

Trending nowThis is a popular solution!
Learn your wayIncludes step-by-step video

Chapter 2 Solutions
Computer Systems: A Programmer's Perspective Plus Mastering Engineering With Pearson Etext -- Access Card Package (3rd Edition)
Additional Engineering Textbook Solutions
Digital Fundamentals (11th Edition)
Concepts Of Programming Languages
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Modern Database Management
Modern Database Management (12th Edition)
Absolute Java (6th Edition)
- You will write a function that can be used to decrypt Dan’s encrypted text. You will be given an open file that contains an encrypted passage from one of the books, and the name of a file containing a wordlist of English words. You need to discover the shift value to use (0-25) in order to decrypt the text. A correct shift value is one that leads to the maximum number of words being found in the English list of words. To find the words in the encrypted text, you must call split(). For a given shift value, convert all letters to lowercase, then try to find each word in the English wordlist. Do not remove any punctuation or symbols from the word: for example, if the word is hello!, then that is the exact string, including exclamation mark, that you should try to find in the English wordlist. Your function should return a string where all letters are in lowercase, and all other characters (newlines, spaces, punctuation, etc.) are retained. Input In each test case, we will call your…arrow_forwardTrace the following Code and then print the output. msg db 'hello world$' newline db 10,13,'$' buffer db 10 dup mov ah,9 mov dx,offset msg int 21h ;new line mov ah,9 mov dx, offset newline int 21h ;enter a string from the keyboard lea dx,buffer mov ah,0ah int 21h ;new line mov ah,9 mov dx,offset newline int 21h ;adding the $ symbol at the end of the string mov bx,0 mov bl,buffer[1] mov buffer[bx+2],'$' mov ah,9 mov dx,offset buffer+2 int 21harrow_forwardThe ".text" section of an ELF file contains the machine code of the compiled program. Question 1 options: True False Both the ".symtab" and the ".debug" sections will be loaded into the memory when the loader loads this ELF file into the memory for execution. Question 2 options: True False For a function of "int f(){static int x = 0; return x;}", the compiler (say, gcc) will allocate space for this static variable in the ".data" section. Question 3 options: True Falsearrow_forward
- USE PYTHON Before there were emoji, there were emoticonsLinks to an external site., whereby text like :) was a happy face and text like :( was a sad face. Nowadays, programs tend to convert emoticons to emoji automatically! In a file called faces.py, implement a function called convert that accepts a str as input and returns that same input with any :) converted to ? (otherwise known as a slightly smiling faceLinks to an external site.) and any :( converted to ? (otherwise known as a slightly frowning faceLinks to an external site.). All other text should be returned unchanged. Then, in that same file, implement a function called main that prompts the user for input, calls convert on that input, and prints the result. You’re welcome, but not required, to prompt the user explicitly, as by passing a str of your own as an argument to input. Be sure to call main at the bottom of your file. ( Please see attached file for Demo) use PYTHONarrow_forwardImplement a function wordCount, which reads a text file, and returns thenumber of words in the file. You can assume the file only contains thealphabet characters and the space character. However there may be morethan one space character between two words, so “abc abc” and “abc abc”,for example, are both two words. int wordCount ( const char * filename ) {}arrow_forwardIn C, write a function that gets 3 pointers int* a, int* b, int* c, and rotates the values in their addresses to the left. That is, a gets the value of b, b gets the value of c, and c gets the value of a. void rotate3 (int* a, int* b, int* c); For example, if we have int x=1, y=2, z=3, then after calling rotate3 (&x, &y, &z) we should have x==2, y==3, and z==1. if we have int x=7, y=1, z=6, then after calling rotate3 (&x, &y, &z) we should have x==1, y==6, and z==7.arrow_forward
- Write a program that receives a coded message file(Lab3ExtraCreditCT.txt) from your local espionage agent and decodes it into a file using standard English. The problem is your agent forgot to tell you the key used to decode the message. Fortunately, this is a simple substitution code consistently using 1 alphanumeric character to represent another, this is case sensitive. All other characters are not substituted, so a space will always be a space, a – will always be a –, a @ will always be a @, etcetera. You may use the following table to help you, it contains the most common letters used in the English language in descending order. E A R I O T N S L C U D P M H G B F Y W K V X Z J Q 0 5 3 2 4 6 8 1 9 7 Using the following key to convert plaintext to coded text: Plaintext = Now is the time for all good men to come to the aid of their country. Key = THEQUICKBROWNFXJMPSVLAZYDG The file your program outputs should look like this: Coded Text = Fxz bs vku vbnu ixp tww…arrow_forwardIn this challenge, the task is to debug the existing code to successfully execute all provided test files. You are required to extend the existing code so that it handles std::invalid_argument exception properly. More specifically, you have to extend the implementation of process_input function. It takes integer as an argument and has to work as follows: It calls function largest_proper_divisor(n). If this call returns a value without raising an exception, it should print in a single line result=d where is the returned value. Otherwise, if the call raises a std::invalid_argument exception, it has to print in a single line the string representation of the raised exception, i.e. its message. Finally, no matter if the exception is raised or not, it should print in a single line returning control flow to caller after any other previously printed output. To keep the code quality high, you are advised to have exactly one line printing returning control flow to caller in the body of…arrow_forwardBy using Linux (Centos 7 or 8) develop a C program to apply the memory times and times, run with this shellprogram, then show the MemToal,MemFree,Active with excel file. Here are the codes for reference. Code no.1: #include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> main(int argc, char *argv[]) { void * p[100]; int i,times,msize; if(argc!=3) { times=20; msize=1024; } else { sscanf(argv[1],"%d",×); sscanf(argv[2],"%d",&msize); if(msize>10240) msize=10240; msize=1024*msize; if(times>100) times=100; } for( i=0; i<times; i++) {…arrow_forward
- Write a function-based C++ program that reads an integer array from a file (“input.txt”) and finds all integers which start and end with the same digit. Write these integers into an output file (“output.txt”). You may assume the length of each integer to be a minimum of 2 digits. Example: Input File 12 131 54 7541 9849 6456 Output File 131 9849 6456arrow_forwardC Programming Task Write a programme called pgmReduce which takes 3 arguments:1. an input file (either ASCII or binary)2. an integer factor n, and3. an output fileand reduces the input file by that factor in each dimension. For example, if the invocation is:pgmReduce inputFile 5 outputFileand inputFile is a 13x17 image, then outputFile should be a 2x3 image in which only thepixels with row and column of 0 modulo 5 in the inputFile existarrow_forwardSuppose a library is processing an input file containing the titles of books in order to identify duplicates. Write a program that reads all of the titles from an input file called bookTitles.inp and writes them to an output file called duplicateTitles.out. When complete, the output file should contain all titles that are duplicated in the input file. Note that the duplicate titles should be written once, even though the input file may contain same titles multiple times. If there are not duplicate titles in the input file, the output file should be empty. Create the input file using Notepad or another text editor, with one title per line. Make sure you have a number of duplicates, including some with three or more copies.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
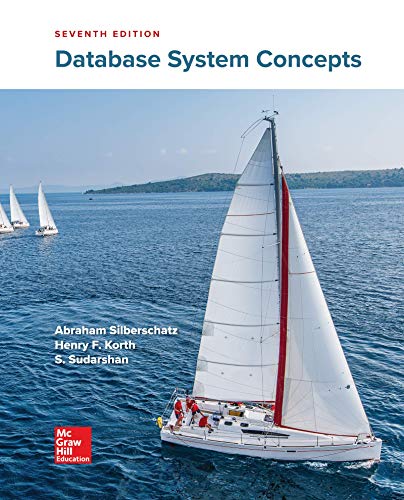
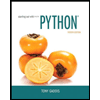
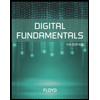
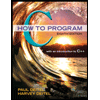
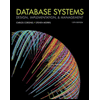
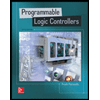