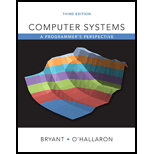
Explanation of Solution
Function definition for “float_le()” function:
The implementation for “float_le()” and “f2u()”function is given below:
//Header file
#include <stdio.h>
#include <assert.h>
//Function definition for returns the result as an unsigned
unsigned f2u(float xvl)
{
/* Returns the unsigned value by using "*(unsigned*)&x" */
return *(unsigned*)&xvl;
}
//Function definition for float_le function
int float_le(float x, float y)
{
//Compute the unsigned value for "x"
unsigned ux = f2u(x);
//Compute the unsigned value for "y"
unsigned uy = f2u(y);
//Get the sign bits for "x"
unsigned sx = ux >> 31;
//Get the sign bits for "y"
unsigned sy = uy >> 31;
/* Returns the result for float_le() function */
return (ux << 1 == 0 && uy << 1 == 0) ||
(sx && !sy) ||
(!sx && !sy && ux <= uy) ||
(sx && sy && ux >= uy);
}
//Main function
int main(int argc, char* argv[])
{
/* Call function "float_le" with checking value using "assert" function */
assert(float_le(-0, +0));
assert(float_le(+0, -0));
assert(float_le(0, 6));
assert(float_le(-8, 8));
return 0;
}
The given code is used to check whether its first argument in given function is less than or equal to its second argument...

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: A Programmer's Perspective Plus Mastering Engineering With Pearson Etext -- Access Card Package (3rd Edition)
- 1. Write a C++ program that takes two binary numbers of 8 bits eachrepresented with the letters Z and O (for zero or one) and displays thesum in binary with Zs and Os. Do this by converting the numbers to ints,adding the its using +, displaying the 3 ints, then converting the sum toZ and Os and displaying the result. 1 a.) Redo number 1, by doing a bit-by-bit addition, not forgetting the carry. 1b.) Write a program to take two numbers represented with romannumerals and display the sum. Assume the sum will be less than 20.Hints: Ask the user to terminate each number with a semicolon and usechars to hold the roman ‘digits’.The numbers are I, II, III IV, V, VI, VII, VIII, IX, X, XI, XII, XIII, XIV, XV…isfive, Change the program to allow the sum to go up to 49 See how large a number you can handle. L is 50, C is 100, M is 1000. Using basic C++ int, char, if- else statements, and switch statements, please! no functions, or strings, or Cmath etc.arrow_forwardWrite a C-function with two arguments (n and r) that has prototype: char clearbit(char k, char bits) The function clears (sets to 0) the bit number k (in the range of 0 to 7) in bits and returns the resulting value. For example, if k is 0x02 and bits is 0x07, the function would return bits with its k’th bit cleared, resulting in 0x03. It must not change other bits in bits. Hint: You may use any number of C-statements, but this task can be accomplished in as few as one!arrow_forwardWrite a function setbits(x,p,n,y) that returns x with the n bits that begin at position p set to the rightmost n bits of y, leaving the other bits unchanged.arrow_forward
- I want to write C or C++ functions that evaluate to ONE when the given conditions are true, and to ZERO when they are false. The following are the fourt conditions: int a(int x); //Any bit of x equals 0. int b(int x); // Any bit of x equals 1. int c(int x); //Any bit in the LSB of x equals 0. int d(int x); //Any bit in the MSB of x equals 1. The code should follow the bit-level integer coding rules, with the additional restriction that you may not use equality (==) or inequality (!=) tests.arrow_forwardplease write in ARM UAL and add comment for explanation.. Thank you..; Write a program that swaps 5th~11th bits in data_a with 25th~31th bits in data_b; Your program must work for any data given, not just the example below; In this question, we assmue that the positions of bits count from right to left.; That is, the first bit is the least significant bit.data_a DCD 0x77FFD1D1data_b DCD 0x12345678arrow_forwardSuppose A = -14 and B = +6 (both in base 10) C=A*B What is the resulting product of your previous multiplication in FP decimal representation (simply use a decimal point)? Take the result you just produce and shift it by three digits to the right of the decimal point (e.g., if your number was 12.0 it now becomes 0.012). Now, express your shifted result as a single precision FP number using the IEEE 754 standard for single precision (of course you need to convert the number from decimal to binary). Please do not calculate more than the first 8 fraction bits.arrow_forward
- Write a function int bitset(int x, int n) to set nth bit of x to 1, if its (n-1)th bit is 1. if n <1 or n >15, the function will return -1 to thecalling functionarrow_forwardIn C, write a function int setbit(int n, int i) to set the i^ᵗʰ bit of n if i^ᵗʰ bit is 0.arrow_forwardBELOW MCQ GIVEN ANSWER CAN BE MORE THAN ONE OPTION. PLEASE PROVIDE CORRECT ANSWERS. ------------------- Q3 :- The ANSI C function below causes the program in which it runs to malfunction . Which of the following connection will help to perform function successfully ? /* Return a count of all the bits set in bytes */ int bitcount (unsigned char c) { unsigned char x , b , count =0; for (b = 0 , x= 1 ; !(x & 0x100); ++b, x <<=1) { if (x | c ) ++count; } return count; } A) change the type of x to a type larger than unsigned char. B) remove the extraneous variable b. C) change the return type to unsigned char . D) change if ( x | c) to if ( x & c) inside the for loop. E) change ! (c & 0x100) to ( x < 0x100) inside the for loop.arrow_forward
- Suppose we number the bytes in a w-bit word from 0 (least significant) to w/8 – 1 (most significant). Write code for the following C function, which will return an unsigned value in which byte i of argument x has been replaced by byte b: unsigned replace_byte (unsigned x, int i, unsigned char b); Here are some examples showing how the function should work: replace_byte(0x12345678, 2, 0xAB) --> 0x12AB5678 replace_byte(0x12345678, 0, 0xAB) --> 0x123456AB Bit-Level Integer Coding Rules In several of the following problems, we will artificially restrict what programming constructs you can use to help you gain a better understanding of the bit-level, logic, and arithmetic operations of C. In answering these problems, your code must follow these rules: Allowed operations All bit-level and logic operations. Left and right shifts, but only with shift amounts between 0 and w – 1. Addition and subtraction. Equality (==) and inequality (!=) tests. (Some of the problems do not allow…arrow_forwardQ2/ Write a program to add the followig five data bytes stored in data segment offset starting from [0500H], if the sum generates a carry, stop the adding and store (01) in data segment offset [0600H], otherwise, continue adding and store the sum in data segment offset [0600H],arrow_forwardplease help me this simpe operation of paython 1. stu = [1, 2, 3, [‘Bob’, 22], [‘Peter’, 21],’Hello world’]stu[1]: stu[3][0]: len(stu):arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
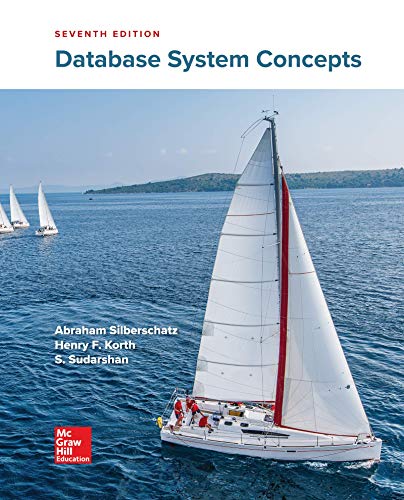
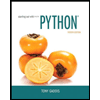
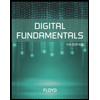
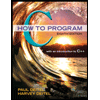
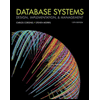
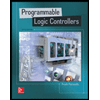