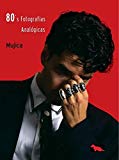
REVEL for Gaddis C++ -- Access Card (What's New in Computer Science)
1st Edition
ISBN: 9780134403922
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21, Problem 1PC
Program Plan Intro
Binary Tree Template
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Insert nodes into the binary tree by using the function “insert_Node ()”.
- Display those nodes by using the function “display_InOrder ()”.
- Display those nodes by using the function “display_PreOrder ()”.
- Display those nodes by using the function “display_PostOrder ()”.
- Delete two nodes from the binary tree by using the function “remove ()”.
- Display remaining nodes by using the function “display_InOrder ()”.
BinaryTree.h:
- Include required header files.
- Create a class template.
- Declare a class named “BinaryTree”. Inside the class,
- Inside the “private” access specifier,
- Give the structure declaration for the creation of node.
- Create an object for the template.
- Create two pointers named “left_Node” and “right_Node” to access the value left and right nodes respectively.
- Declare a variable “leafCount”.
- Create a pointer named “root” to access the value of root node.
- Give function declaration for “insert ()”, “destroy_SubTree ()”, “delete_Node ()”, “make_Deletion ()”, “display_InOrder ()”, “display_PreOrder ()”, and “display_PostOrder ()”.
- Give the structure declaration for the creation of node.
- Inside “public” access specifier,
- Give the definition for constructor and destructor.
- Give function declaration.
- Inside the “private” access specifier,
- Declare template class.
- Give function definition for “insert ()”.
- Check if “nodePtr” is null.
- If the condition is true then, insert node.
- Check if value of new node is less than the value of node pointer
- If the condition is true then, Insert node to the left branch by calling the function “insert ()” recursively.
- Else
- Insert node to the right branch by calling the function “insert ()” recursively.
- Check if “nodePtr” is null.
- Declare template class.
- Give function definition for “insert_Node ()”.
- Create a pointer for new node.
- Assign the value to the new node.
- Make left and right node as null
- Call the function “insert ()” by passing parameters “root” and “newNode”.
- Declare template class.
- Give function definition for “destroy_SubTree ()”.
- Check if the node pointer points to left node
- Call the function recursively to delete the left sub tree.
- Check if the node pointer points to the right node
- Call the function recursively to delete the right sub tree.
- Delete the node pointer.
- Check if the node pointer points to left node
- Declare template class.
- Give function definition for “search_Node ()”.
- Assign false to the Boolean variable “status”.
- Assign root pointer to the “nodePtr”.
- Do until “nodePtr” exists.
- Check if the value of node pointer is equal to “num”.
- Assign true to the Boolean variable “status”
- Check if the number is less than the value of node pointer.
- Assign left node pointer to the node pointer.
- Else
- Assign right node pointer to the node pointer.
- Check if the value of node pointer is equal to “num”.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “remove ()”.
- Call the function “delete_Node ()”
- Declare template class.
- Give function definition for “delete_Node ()”
- Check if the number is less than the node pointer value.
- Call the function “delete_Node ()” recursively.
- Check if the number is greater than the node pointer value.
- Call the function “delete_Node ()” recursively.
- Else,
- Call the function “make_Deletion ()”.
- Check if the number is less than the node pointer value.
- Declare template class.
- Give function definition for “make_Deletion ()”
- Create pointer named “tempPtr”.
- Check if the nodePtr is null.
- If the condition is true then, print “Cannot delete empty node.”
- Check if right node pointer is null.
- If the condition is true then,
- Make the node pointer as the temporary pointer.
- Reattach the left node child.
- Delete temporary pointer.
- If the condition is true then,
- Check is left node pointer is null
- If the condition is true then,
- Make the node pointer as the temporary pointer.
- Reattach the right node child.
- Delete temporary pointer.
- If the condition is true then,
- Else,
- Move right node to temporary pointer
- Reach to the end of left-Node using “while” condition.
- Assign left node pointer to temporary pointer.
- Reattach left node sub tree.
- Make node pointer as the temporary pointer.
- Reattach right node sub tree
- Delete temporary pointer.
- Declare template class.
- Give function definition for “display_InOrder ()”.
- Check if the node pointer exists.
- Call the function “display_InOrder ()” recursively.
- Print the value
- Call the function “display_InOrder ()” recursively.
- Check if the node pointer exists.
- Declare template class.
- Give function definition for “display_PreOrder ()”.
- Print the value.
- Call the function “display_PreOrder ()” recursively.
- Call the function “display_PreOrder ()” recursively.
- Declare template class.
- Give function definition for “display_PostOrder ()”.
- Call the function “display_PostOrder ()” recursively.
- Call the function “display_PostOrder ()” recursively.
- Print value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ DATA STRUCTURES
Implement the TNode and Tree classes. The TNode class will include a data item name of type string,which will represent a person’s name. Yes, you got it right, we are going to implement a family tree!Please note that this is not a Binary Tree.
Write the methods for inserting nodes into the tree,searching for a node in the tree, and performing pre-order and post-order traversals.The insert method should take two strings as input. The second string will be added as a child node tothe parent node represented by the first string.
Hint:
The TNode class will need to have two TNode pointers in addition to the name data member:TNode *sibling will point to the next sibling of this node, and TNode *child will represent the first child ofthis node. You see two linked lists here??? Yes! You’ll need to use the linked lists
Text concordance using BST
1 Project description
Write a cross-reference program that constructs a binary search tree (any type, including splay tree) with all words included from a text file and records the positions (word or line numbers) on which these words were used. These line numbers should be stored on linked lists associated with the nodes of the tree. After the input file has been processed, print in alphabetical order all words of the text file along with the corresponding list of numbers of the lines in which the words occur.
2 What to turn in
You will turn in
a short written report containing: A description of the significant choices/issues in the design of your code.
The source-code of your program.
3 Coding standards
A percentage of your grade will be based on the quality of your code, so pay attention to it. Discuss changes (if any) you made to programs presented in class. Take extra care in documenting the code you are implementing on your…
(binary search tree c++)i am having issues with my code i am able to insert names into into the tree but they do not save(it says tree is empty). i will show you a picture of my output(black background) and a picture of the desired output(white background), i will also include my source main and .h class.
//bt.cpp - file contains member functions#include "bt.h"#include <iostream>using namespace std;//ConstructorBT::BT(){ root = NULL;}
//Insert new item in BSTvoid BT::insert(string d){ node* t = new node; node* parent;
t->data = d; t->left = NULL; t->right = NULL; parent = NULL;
if (isEmpty()) root = t; else { //Note: ALL insertions are as leaf nodes node* curr; curr = root; // Find the Node's parent while (curr) { parent = curr; if (t->data > curr->data) curr = curr->right; else curr = curr->left; }…
Chapter 21 Solutions
REVEL for Gaddis C++ -- Access Card (What's New in Computer Science)
Ch. 21.1 - Prob. 21.1CPCh. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21.1 - Prob. 21.4CPCh. 21.1 - Prob. 21.5CPCh. 21.1 - Prob. 21.6CPCh. 21.2 - Prob. 21.7CPCh. 21.2 - Prob. 21.8CPCh. 21.2 - Prob. 21.9CPCh. 21.2 - Prob. 21.10CP
Ch. 21.2 - Prob. 21.11CPCh. 21.2 - Prob. 21.12CPCh. 21 - Prob. 1RQECh. 21 - Prob. 2RQECh. 21 - Prob. 3RQECh. 21 - Prob. 4RQECh. 21 - Prob. 5RQECh. 21 - Prob. 6RQECh. 21 - Prob. 7RQECh. 21 - Prob. 8RQECh. 21 - Prob. 9RQECh. 21 - Prob. 10RQECh. 21 - Prob. 11RQECh. 21 - Prob. 12RQECh. 21 - Prob. 13RQECh. 21 - Prob. 14RQECh. 21 - Prob. 15RQECh. 21 - Prob. 16RQECh. 21 - Prob. 17RQECh. 21 - Prob. 18RQECh. 21 - Prob. 19RQECh. 21 - Prob. 20RQECh. 21 - Prob. 21RQECh. 21 - Prob. 22RQECh. 21 - Prob. 23RQECh. 21 - Prob. 24RQECh. 21 - Prob. 25RQECh. 21 - Prob. 1PCCh. 21 - Prob. 2PCCh. 21 - Prob. 3PCCh. 21 - Prob. 4PCCh. 21 - Prob. 5PCCh. 21 - Prob. 6PCCh. 21 - Prob. 7PCCh. 21 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- AVL Tree Implementation program Important note: You must separate your program into three files: header file (*.h), implementation file (*.cpp) and main file (*.cpp). Write a program to build an AVL tree by accepting the integers input from users. For each input, balance the tree and display it on the screen; you then calculate the indorder traversals as well. There should be a menu to drive the program. It should be similar as follows: AVL Tree Implementation A: Insert an integer to tree and show the balanced tree at each insertion. B: Display the balanced tree and show inorder traversal. C: Exit To be sure, your program is correctly working, use the following data to test AVL tree: 15, 18, 10, 7, 57, 6, 13, 12, 9, 65, 19, 16, 23 You should perform more test with different data sets.arrow_forwardComputer Science I need help in coding a method in c# called union that combines a tree so that the tree is returned to having at most one instance of each level.arrow_forwardProgramming questions:typedef struct node { int data; struct node *left, *right;}BT;The node structure of the binary tree (BT) is shown above. There is a binary tree T, please complete the function: int degreeone(BT *T) to compute how many degree 1 node in the BT. The T is the root pointer, and the function shoule return the total number of degree 1 node.arrow_forward
- Build a tree identification code. please Fast thank youarrow_forwardC++ please add comments so I understand Write a program that takes n elements as input and inserts them into a binary tree. Include a function to print out the elements in the tree. Please do not use header files - just one simple program that I can understand.arrow_forwardAdd to the BinarySearchTree class a member function string smallest() const that returns the smallest element of the tree. Provide a test program to test your function. #include <iostream>#include <string>using namespace std;class TreeNode{public:void insert_node(TreeNode* new_node);void print_nodes() const;bool find(string value) const;private:string data;TreeNode* left;TreeNode* right;friend class BinarySearchTree;};class BinarySearchTree{public:BinarySearchTree();void insert(string data);void erase(string data);int count(string data) const;void print() const;private:TreeNode* root;};BinarySearchTree::BinarySearchTree(){root = NULL;}void BinarySearchTree::print() const{if (root != NULL)root->print_nodes();}void BinarySearchTree::insert(string data){TreeNode* new_node = new TreeNode;new_node->data = data;new_node->left = NULL;new_node->right = NULL;if (root == NULL) root = new_node;else root->insert_node(new_node);}void TreeNode::insert_node(TreeNode*…arrow_forward
- DATA STRUCTURE C++: Binary trees What is the definition of the public method setRootData for binary tree? Implement it using a recursive helper in a way that the resulting binary tree stayed balanced.arrow_forwardp-8.65in Java Write a program that takes as input a fully parenthesized ,arithmetic expression and convert it to a binary expression tree.Your program should display the tree in some way and also print the valueassociated with the root. For an additional challenge , allow the leaves tostore variables of X1, X2 , X3 and so on, which are initially 0 and whichcan be updated interactively by your program, with the corresponding updatein the printed value of the root of the expression tree.arrow_forwardPython binary search tree: a function that takes in a root, p, and checks whether the tree rooted in p is a binary search tree or not. What is the time complexity of your function? def is_bst(self, p: Node):arrow_forward
- Assume the above tree is a binary search tree, a) Show the tree after removing node h; b) Show the tree after removing node f; c) Show the tree after removing node a; Please do part a b and c (Note, each small question is independent.)arrow_forwardC programming I need help writing a code that uses a struct pointer into a binary tree and using the same pointer into an arrayarrow_forwardPYTHON PROGRAMMING ONLY (BINARY SEARCH TREE) IF POSSIBLE USE PYCHARM AND INSTALL BINARYTREE IN PYTHON PACKAGES. Create a program that does the following:1. Generate a random binary tree with 12 elements. You can leave the perfect option asFalse.2. Print the tree.3. Test the tree properties. The documentation uses the assert statement.4. Create a binary search tree manually (not using the bst function, but instead buildingit by hand. Put at least 8 elements in it.5. Print this tree.6. Print the values in the tree in several orders (inorder, postorder, preorder, etc.)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
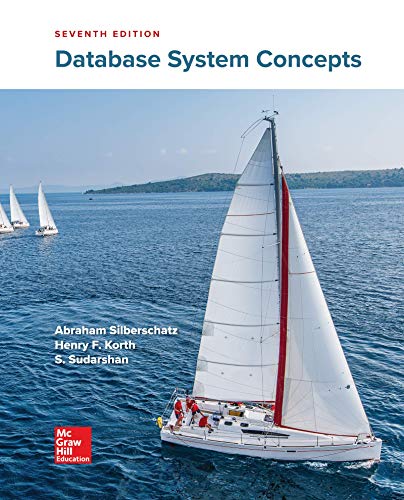
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
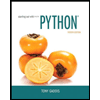
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
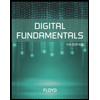
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
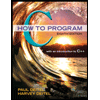
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
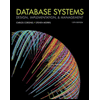
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
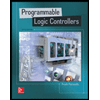
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education