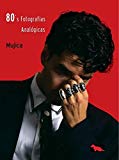
REVEL for Gaddis C++ -- Access Card (What's New in Computer Science)
1st Edition
ISBN: 9780134403922
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21, Problem 3PC
Program Plan Intro
Leaf Counter
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Display the number of leaf nodes by calling the function “num_LeafNodes ()”.
- Insert nodes into the binary tree by using the function “insert_Node ()”.
- Display those nodes by using the function “display_InOrder ()”.
- Now, display the number of leaf nodes by calling the function “num_LeafNodes ()”.
- Delete two nodes from the binary tree by using the function “remove ()”.
- Display remaining nodes by using the function “display_InOrder ()”.
- Finally, display the number of leaf nodes by calling the function “num_LeafNodes ()”.
BinaryTree.h:
- Include required header files.
- Create a class template.
- Declare a class named “BinaryTree”. Inside the class,
- Inside the “private” access specifier,
- Give the structure declaration for the creation of node.
- Create an object for the template.
- Create two pointers named “left_Node” and “right_Node” to access the value left and right nodes respectively.
- Declare a variable “leafCount”.
- Create a pointer named “root” to access the value of root node.
- Give function declaration for “insert ()”, “destroy_SubTree ()”, “delete_Node ()”, “make_Deletion ()”, “display_InOrder ()”, “display_PreOrder ()”, “display_PostOrder ()”, “count_Nodes ()”, “count_Leaves ()”.
- Give the structure declaration for the creation of node.
- Inside “public” access specifier,
- Give the definition for constructor and destructor.
- Give function declaration.
- Inside the “private” access specifier,
- Declare template class.
- Give function definition for “insert ()”.
- Check if “nodePtr” is null.
- If the condition is true then, insert node.
- Check if value of new node is less than the value of node pointer
- If the condition is true then, Insert node to the left branch by calling the function “insert ()” recursively.
- Else
- Insert node to the right branch by calling the function “insert ()” recursively.
- Check if “nodePtr” is null.
- Declare template class.
- Give function definition for “insert_Node ()”.
- Create a pointer for new node.
- Assign the value to the new node.
- Make left and right node as null
- Call the function “insert ()” by passing parameters “root” and “newNode”.
- Declare template class.
- Give function definition for “destroy_SubTree ()”.
- Check if the node pointer points to left node
- Call the function recursively to delete the left sub tree.
- Check if the node pointer points to the right node
- Call the function recursively to delete the right sub tree.
- Delete the node pointer.
- Check if the node pointer points to left node
- Declare template class.
- Give function definition for “search_Node ()”.
- Assign false to the Boolean variable “status”.
- Assign root pointer to the “nodePtr”.
- Do until “nodePtr” exists.
- Check if the value of node pointer is equal to “num”.
- Assign true to the Boolean variable “status”
- Check if the number is less than the value of node pointer.
- Assign left node pointer to the node pointer.
- Else
- Assign right node pointer to the node pointer.
- Check if the value of node pointer is equal to “num”.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “remove ()”.
- Call the function “delete_Node ()”
- Declare template class.
- Give function definition for “delete_Node ()”
- Check if the number is less than the node pointer value.
- Call the function “delete_Node ()” recursively.
- Check if the number is greater than the node pointer value.
- Call the function “delete_Node ()” recursively.
- Else,
- Call the function “make_Deletion ()”.
- Check if the number is less than the node pointer value.
- Declare template class.
- Give function definition for “make_Deletion ()”
- Create pointer named “tempPtr”.
- Check if the nodePtr is null.
- If the condition is true then, print “Cannot delete empty node.”
- Check if right node pointer is null.
- If the condition is true then,
- Make the node pointer as the temporary pointer.
- Reattach the left node child.
- Delete temporary pointer.
- If the condition is true then,
- Check is left node pointer is null
- If the condition is true then,
- Make the node pointer as the temporary pointer.
- Reattach the right node child.
- Delete temporary pointer.
- If the condition is true then,
- Else,
- Move right node to temporary pointer
- Reach to the end of left-Node using “while” condition.
- Assign left node pointer to temporary pointer.
- Reattach left node sub tree.
- Make node pointer as the temporary pointer.
- Reattach right node sub tree
- Delete temporary pointer.
- Declare template class.
- Give function definition for “display_InOrder ()”.
- Check if the node pointer exists.
- Call the function “display_InOrder ()” recursively.
- Print the value
- Call the function “display_InOrder ()” recursively.
- Check if the node pointer exists.
- Declare template class.
- Give function definition for “display_PreOrder ()”.
- Print the value.
- Call the function “display_PreOrder ()” recursively.
- Call the function “display_PreOrder ()” recursively.
- Declare template class.
- Give function definition for “display_PostOrder ()”.
- Call the function “display_PostOrder ()” recursively.
- Call the function “display_PostOrder ()” recursively.
- Print value
- Declare template class.
- Give function definition for “numNodes ()”.
- Call the function “count_Nodes ()”.
- Declare template class.
- Give function definition for “count_Nodes ()”.
- Declare a variable named “count”.
- Check if the node pointer is null
- Assign 0 to count.
- Else,
- Call the function “count_Nodes ()” recursively.
- Return the variable “count”.
- Declare template class.
- Give function definition for “num_LeafNodes()”.
- Assign 0 to “leafCount”
- Call the function “count_Leaves ()”
- Return the variable.
- Declare template class.
- Give function definition for “count_Leaves()”.
- Call the function “count_Leaves ()” recursively by passing left node pointer as the parameter.
- Call the function “count_Leaves ()” recursively by passing right node pointer as the parameter.
- Check if left and right node pointers are null.
- Increment the variable “leafCount”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a code to delete a node from a Binary search tree.
The format of the function is void deleteNode(int key).
Note - Do not use any other return form for the function. Do not return any node pointers
Computer Science
QUESTION:
Expression tree is an application of binary tree to represent arithmetic expression.
Write a program to implement an ExpressionTree class that can represent an arithmetic expression with binary operators and integer operands including floating-point. Limit the operators to addition, subtraction, multiplication and division. Operands can be constants or variables. Your class should be able to build a tree from its signature, and evaluate it on a set of values for the variable operands.
Add to the BinarySearchTree class a member function string smallest() const that returns the smallest element of the tree. Provide a test program to test your function.
#include <iostream>#include <string>using namespace std;class TreeNode{public:void insert_node(TreeNode* new_node);void print_nodes() const;bool find(string value) const;private:string data;TreeNode* left;TreeNode* right;friend class BinarySearchTree;};class BinarySearchTree{public:BinarySearchTree();void insert(string data);void erase(string data);int count(string data) const;void print() const;private:TreeNode* root;};BinarySearchTree::BinarySearchTree(){root = NULL;}void BinarySearchTree::print() const{if (root != NULL)root->print_nodes();}void BinarySearchTree::insert(string data){TreeNode* new_node = new TreeNode;new_node->data = data;new_node->left = NULL;new_node->right = NULL;if (root == NULL) root = new_node;else root->insert_node(new_node);}void TreeNode::insert_node(TreeNode*…
Chapter 21 Solutions
REVEL for Gaddis C++ -- Access Card (What's New in Computer Science)
Ch. 21.1 - Prob. 21.1CPCh. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21.1 - Prob. 21.4CPCh. 21.1 - Prob. 21.5CPCh. 21.1 - Prob. 21.6CPCh. 21.2 - Prob. 21.7CPCh. 21.2 - Prob. 21.8CPCh. 21.2 - Prob. 21.9CPCh. 21.2 - Prob. 21.10CP
Ch. 21.2 - Prob. 21.11CPCh. 21.2 - Prob. 21.12CPCh. 21 - Prob. 1RQECh. 21 - Prob. 2RQECh. 21 - Prob. 3RQECh. 21 - Prob. 4RQECh. 21 - Prob. 5RQECh. 21 - Prob. 6RQECh. 21 - Prob. 7RQECh. 21 - Prob. 8RQECh. 21 - Prob. 9RQECh. 21 - Prob. 10RQECh. 21 - Prob. 11RQECh. 21 - Prob. 12RQECh. 21 - Prob. 13RQECh. 21 - Prob. 14RQECh. 21 - Prob. 15RQECh. 21 - Prob. 16RQECh. 21 - Prob. 17RQECh. 21 - Prob. 18RQECh. 21 - Prob. 19RQECh. 21 - Prob. 20RQECh. 21 - Prob. 21RQECh. 21 - Prob. 22RQECh. 21 - Prob. 23RQECh. 21 - Prob. 24RQECh. 21 - Prob. 25RQECh. 21 - Prob. 1PCCh. 21 - Prob. 2PCCh. 21 - Prob. 3PCCh. 21 - Prob. 4PCCh. 21 - Prob. 5PCCh. 21 - Prob. 6PCCh. 21 - Prob. 7PCCh. 21 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Text concordance using BST 1 Project description Write a cross-reference program that constructs a binary search tree (any type, including splay tree) with all words included from a text file and records the positions (word or line numbers) on which these words were used. These line numbers should be stored on linked lists associated with the nodes of the tree. After the input file has been processed, print in alphabetical order all words of the text file along with the corresponding list of numbers of the lines in which the words occur. 2 What to turn in You will turn in a short written report containing: A description of the significant choices/issues in the design of your code. The source-code of your program. 3 Coding standards A percentage of your grade will be based on the quality of your code, so pay attention to it. Discuss changes (if any) you made to programs presented in class. Take extra care in documenting the code you are implementing on your…arrow_forwardTo copy a tree, traverse the tree using an ___ traversal and add each data item visited to the new tree. inorder postorder preorder reorderarrow_forwardBuild a Node class. It is should have attributes for the data it stores as well as its left and right children. As a bonus, try including the Comparable module and make nodes compare using their data attribute. Build a Tree class that accepts an array when initialized. The Tree class should have a root attribute that uses the return value of #build_tree which you'll write next. Write a #build_tree method that takes an array of data (e.g. [1, 7, 4, 23, 8, 9, 4, 3, 5, 7, 9, 67, 6345, 324]) and turns it into a balanced binary tree full of Node objects appropriately placed (don't forget to sort and remove duplicates!). The #build_tree method should return the level-1 root node. Write an #insert and #delete method which accepts a value to insert/delete. Compile and submit your source code and screenshots of the application executing the application and the results based in python. Your paper should be 2-3 pages in length (not including title and references pages)arrow_forward
- JAVA PROGRAMMING LANGUAGE: 1. Create a class that accepts id numbers ranging from 1 to 29. 2. The id numbers are nodes of a binary tree. 3. Traverse the tree in inorder, preorder, and postorder and display the traversed values.arrow_forward1. Insert operation on AVL Trees2. Delete operation on AVL TreesQuestion 1: For this lab, use your BST code implemented in the previous lab.In this lab, you haveto create a height-balanced tree class named “AVL”. Inherit the BST class publicly in your new“AVL” class. You can add “height” variable in your existing TNode struct implementation.Implement the following methods for AVL class:a. A default Constructor which calls the default constructor of base class (BST class).b. Override the insert method of base class (BST class) in your AVL class, so that the AVL treeremains height-balanced after insertion of a new node.c. Override the delete method of base class (BST class) in your AVL class, so that the AVL treeremains height-balanced after deletion of a node.d. A function “height” which returns the height of the tree. int height()conste. A function “search” which returns a pointer to the value of the node containing the required keybQuestion 2: Now run the following main program.int…arrow_forwardJava - Write an insert module for a Binary Tree data structure. The module will take two parameters: a root pointer/reference to a TreeNode, and a character as a data element (each TreeNode has a character data element called "data", and 2 references to a TreeNode; called "left" and "right"). This insert module is not a member method. Higher values go to the left. Do NOT assume the existence of any other methodsarrow_forward
- Programming questions:typedef struct node { int data; struct node *left, *right;}BT;The node structure of the binary tree (BT) is shown above. There is a binary tree T, please complete the function: int degreeone(BT *T) to compute how many degree 1 node in the BT. The T is the root pointer, and the function shoule return the total number of degree 1 node.arrow_forward(Python)- Implement functions successor and predecessor. These functions will take two arguments: the root of a tree and an integer. The successor function returns the smallest item in the tree that is greater than the given item. The predecessorfunction returns the largest item in the tree that is smaller than the given item. Both functions will return -1 if not found. Note that the predecessor or successor may exist even if the given item is not present in the tree. Use this template: # Node definition provided, please don't modify it.class TreeNode:def __init__(self, val=None):self.val = valself.left = Noneself.right = None# TODO: Please implement the following functions that return an integer# Return the largest value in the tree that is smaller than given value. Return -1 if not found.def predecessor(root, value):pass# Return the smallest value in the tree that is bigger than given value. Return -1 if not found.def successor(root, value):passif __name__ == "__main__":# TODO:…arrow_forwardC++ Build a templated version a binary search tree using a linked implementation along with functions for insertion and deletion. You must use the textbook's BinarySearchTree ADT as the base for your code. (See attached file.) Of course, you will need to modify the code a bit. Build a function that searches for a given item in the BST in the tree. It must return the number of compares that it used before returning as well as an indicator telling whether the sought item was or was not in the tree. Build a driver than inserts 10K unique random ints into the BST. Then, use the search function to seek the 1st, 500th, 5,000th, and 10,000th integers that were inserted in the tree, reporting the number of compares for each item. Also seek at least 2 integers that are not in the tree, one integer must be larger than the largest value in the tree and one integer must be within the range of values that are stored within the tree. Report the number of compares for these searches as well.arrow_forward
- Java Programming Language: 1. Make a class that accepts id numbers ranging from 1 to 29. 2. The id numbers are nodes of a binary tree. 3. Traverse the tree in inorder, preorder, and postorder and display the traversed values.arrow_forwardSubject: Information Technology/Computer Science Java Programming Language: 1. Create a class that accepts id numbers ranging from 1 to 29. 2. The id numbers are nodes of a binary tree. 3. Traverse the tree in inorder, preorder, and postorder and display the traversed values.arrow_forwardTo insert an element into a binary search tree (BST), you need to locate where to insert it in the tree. The key idea is to locate the parent for the new node. True Falsearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
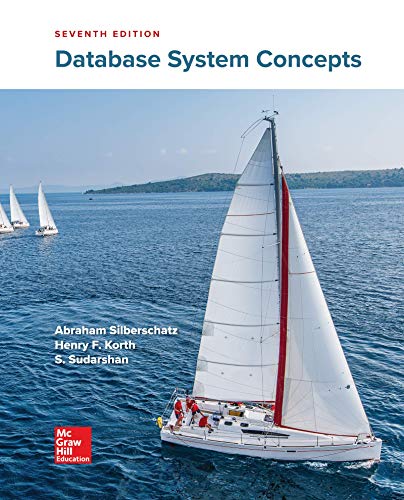
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
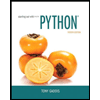
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
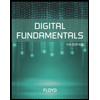
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
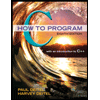
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
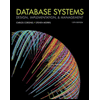
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
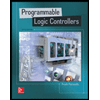
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education