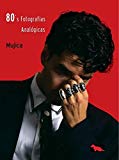
REVEL for Gaddis C++ -- Access Card (What's New in Computer Science)
1st Edition
ISBN: 9780134403922
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21, Problem 7PC
Program Plan Intro
Queue Converter
Program Plan:
DynIntQueue.h:
- Include required header files.
- Declare a class named “DynIntQueue”; inside the class,
- Inside the “private” access specifier,
- Create a structure named “QueueNode”.
- Declare a variable “value”.
- Create a pointer named “next”.
- Create two pointers named “front” and “rear”.
- Declare a variable “numItems”.
- Create a structure named “QueueNode”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue()”, “dequeue()”, “isEmpty()”, “isFull()”, and “clear()”.
- Inside the “private” access specifier,
DynIntQueue.cpp:
- Include required header files.
- Give definition for the constructor.
- Assign the values.
- Give definition for the destructor.
- Call the function “clear()”.
- Give function definition for “enqueue()”.
- Make the pointer “newNode” as null.
- Assign “num” to “newNode->value”.
- Make “newNode->next” as null.
- Check whether the queue is empty using “isEmpty()” function.
- If the condition is true then, assign “newNode” to “front” and “rear”.
- If the condition is not true then,
- Assign “newNode” to “rear->next”.
- Assign “newNode” to “rear”.
- Increment the variable “numItems”.
- Give function definition for “dequeue()”.
- Assign “temp” pointer as null.
- Check if the queue is empty using “isEmpty()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Assign the value of front to the variable “num”.
- Make “front->next” as “temp”.
- Delete the front value.
- Make temp as front.
- Decrement the variable “numItems”.
- Give function definition for “isEmpty()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Give function definition for “clear()”.
- Declare a variable.
- Dequeue values from queue till the queue becomes empty using “while” condition.
- Declare a variable.
IntBinaryTree.h:
- Include required header files.
- Declare a class named “IntBinaryTree”. Inside the class,
- Inside the “private” access specifier,
- Give the structure declaration for the creation of node.
- Declare a variable
- Create two pointers named “left” and “right” to access the value left and right nodes respectively.
- Create a pointer named “root” to access the value of root node.
- Give function declaration for “insert ()”, “destroy_SubTree ()”, “delete_Node ()”, “make_Deletion ()”, “display_InOrder ()”, “display_PreOrder ()”, “display_PostOrder ()”, “copyTree ()”, and “setQueue ()”.
- Give the structure declaration for the creation of node.
- Inside “public” access specifier,
- Give the definition for constructor and destructor.
- Give function declaration for binary tree operations.
- Inside the “private” access specifier,
IntBinaryTree.cpp:
- Include required header files.
- Give definition for copy constructor.
- Give function definition for “insert()”.
- Check if “nodePtr” is null.
- If the condition is true then, insert node.
- Check if value of new node is less than the value of node pointer
- If the condition is true then, Insert node to the left branch by calling the function “insert()” recursively.
- Else,
- Insert node to the right branch by calling the function “insert()” recursively.
- Check if “nodePtr” is null.
- Give function definition for “insert_Node ()”.
- Create a pointer for new node.
- Assign the value to the new node.
- Make left and right node as null.
- Call the function “insert()” by passing parameters “root” and “newNode”.
- Give function definition for “destroy_SubTree()”.
- Check if the node pointer points to left node
- Call the function recursively to delete the left sub tree.
- Check if the node pointer points to the right node
- Call the function recursively to delete the right sub tree.
- Delete the node pointer.
- Check if the node pointer points to left node
- Give function definition for “search_Node()”.
- Assign false to the Boolean variable “status”.
- Assign root pointer to the “nodePtr”.
- Do until “nodePtr” exists.
- Check if the value of node pointer is equal to “num”.
- Assign true to the Boolean variable “status”
- Check if the number is less than the value of node pointer.
- Assign left node pointer to the node pointer.
- Else,
- Assign right node pointer to the node pointer.
- Check if the value of node pointer is equal to “num”.
- Return the Boolean variable.
- Give function definition for “remove()”.
- Call the function “delete_Node()”
- Give function definition for “delete_Node()”
- Check if the number is less than the node pointer value.
- Call the function “delete_Node()” recursively.
- Check if the number is greater than the node pointer value.
- Call the function “delete_Node()” recursively.
- Else,
- Call the function “make_Deletion()”.
- Check if the number is less than the node pointer value.
- Give function definition for “make_Deletion()”
- Create pointer named “tempPtr”.
- Check if the “nodePtr” is null.
- If the condition is true then, print “Cannot delete empty node.”
- Check if right node pointer is null.
- If the condition is true then,
- Make the node pointer as the temporary pointer.
- Reattach the left node child.
- Delete temporary pointer.
- If the condition is true then,
- Check is left node pointer is null
- If the condition is true then,
- Make the node pointer as the temporary pointer.
- Reattach the right node child.
- Delete temporary pointer.
- If the condition is true then,
- Else,
- Move right node to temporary pointer
- Reach to the end of left-Node using “while” condition.
- Assign left node pointer to temporary pointer.
- Reattach left node sub tree.
- Make node pointer as the temporary pointer.
- Reattach right node sub tree
- Delete temporary pointer.
- Give function definition for “display_InOrder()”.
- Check if the node pointer exists.
- Call the function “display_InOrder()” recursively.
- Print the value
- Call the function “display_InOrder()” recursively.
- Check if the node pointer exists.
- Give function definition for “display_PreOrder()”.
- Print the value.
- Call the function “display_PreOrder()” recursively.
- Call the function “display_PreOrder()” recursively.
- Give function definition for “display_PostOrder()”.
- Call the function “display_PostOrder()” recursively.
- Call the function “display_PostOrder()” recursively.
- Print value.
- Give function definition for assignment operator.
- Call the function “destroy_SubTree()”
- Call the copy constructor.
- Return the pointer.
- Copy tree function is called by copy constructor and assignment operator function
- Create a pointer named “newNode”.
- Check if “nPtr” is not equal to null
- Allocate memory dynamically.
- Assign pointer value to the new node.
- Call the function “copyTree()” by passing “nPtr” of left.
- Call the function “copyTree()” by passing “nPtr” of right
- Return the new node.
- Function definition for “setQueue()”.
- Check if the pointer “nodePtr” exists.
- Call the function “setQueue()” recursively by passing the left node.
- Call the function “setQueue()” recursively by passing the right node.
- Call the function “enqueue()” recursively by passing the left node.
Main.cpp:
- Include required header files.
- Inside “main()” function,
- Declare a variable “value” and assign it to 0.
- Create an object “intBT” for “IntBinaryTree” class.
- Insert 5 values using “insert_Node()” function.
- Display all the values by using the function “display_InOrder()”.
- Create an object “iqueue” for “DynIntQueue” class.
- Load the address to the pointer “qPtr”.
- Pass this pointer to the function “treeToQueue ()”.
- Do until the queue is not empty.
- Declare a variable.
- Call the function “dequeue()”.
- Display the value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Programming questions:typedef struct node
{ int data; struct node *left, *right;}BT;The node structure of the binary tree (BT) is shown above. There is a binary tree T, please complete the function: int degreeone(BT *T) to compute how many degree 1 node in the BT. The T is the root pointer, and the function shoule return the total number of degree 1 node.
C PROGRAMMING
•Write a program that counts how many lotto tickets each person has bought
.•Create a sorted binary tree
.•In the tree node you have to store first name, last name, number of tickets and references to two nodes.
•Read the file lotto300k.txt into a binary tree.
•Traverse the completed tree and print those customers that bought more than one ticket.
Subject: Information Technology/Computer Science Java Programming Language:
1. Create a class that accepts id numbers ranging from 1 to 29.
2. The id numbers are nodes of a binary tree.
3. Traverse the tree in inorder, preorder, and postorder and display the traversed values.
Chapter 21 Solutions
REVEL for Gaddis C++ -- Access Card (What's New in Computer Science)
Ch. 21.1 - Prob. 21.1CPCh. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21.1 - Prob. 21.4CPCh. 21.1 - Prob. 21.5CPCh. 21.1 - Prob. 21.6CPCh. 21.2 - Prob. 21.7CPCh. 21.2 - Prob. 21.8CPCh. 21.2 - Prob. 21.9CPCh. 21.2 - Prob. 21.10CP
Ch. 21.2 - Prob. 21.11CPCh. 21.2 - Prob. 21.12CPCh. 21 - Prob. 1RQECh. 21 - Prob. 2RQECh. 21 - Prob. 3RQECh. 21 - Prob. 4RQECh. 21 - Prob. 5RQECh. 21 - Prob. 6RQECh. 21 - Prob. 7RQECh. 21 - Prob. 8RQECh. 21 - Prob. 9RQECh. 21 - Prob. 10RQECh. 21 - Prob. 11RQECh. 21 - Prob. 12RQECh. 21 - Prob. 13RQECh. 21 - Prob. 14RQECh. 21 - Prob. 15RQECh. 21 - Prob. 16RQECh. 21 - Prob. 17RQECh. 21 - Prob. 18RQECh. 21 - Prob. 19RQECh. 21 - Prob. 20RQECh. 21 - Prob. 21RQECh. 21 - Prob. 22RQECh. 21 - Prob. 23RQECh. 21 - Prob. 24RQECh. 21 - Prob. 25RQECh. 21 - Prob. 1PCCh. 21 - Prob. 2PCCh. 21 - Prob. 3PCCh. 21 - Prob. 4PCCh. 21 - Prob. 5PCCh. 21 - Prob. 6PCCh. 21 - Prob. 7PCCh. 21 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- AVL Tree Implementation program Important note: You must separate your program into three files: header file (*.h), implementation file (*.cpp) and main file (*.cpp). Write a program to build an AVL tree by accepting the integers input from users. For each input, balance the tree and display it on the screen; you then calculate the indorder traversals as well. There should be a menu to drive the program. It should be similar as follows: AVL Tree Implementation A: Insert an integer to tree and show the balanced tree at each insertion. B: Display the balanced tree and show inorder traversal. C: Exit To be sure, your program is correctly working, use the following data to test AVL tree: 15, 18, 10, 7, 57, 6, 13, 12, 9, 65, 19, 16, 23 You should perform more test with different data sets.arrow_forwardJAVA PROGRAMMING LANGUAGE: 1. Create a class that accepts id numbers ranging from 1 to 29. 2. The id numbers are nodes of a binary tree. 3. Traverse the tree in inorder, preorder, and postorder and display the traversed values.arrow_forwardPROLOG PROGRAMMING LANGUAUGE PLEASE (* First argument is a BST and the second argument is a file name. Visits the tree nodes in preorder recursively and writes its data to the file separated by spaces. *) preOrderWrite/2arrow_forward
- Binary Search Tree (BST)A. Draw the binary search tree after you insert the following values40,20,10,25,30,22,50 Hint: BST has property that right child node is greater than root whileThe left child node is smaller than the root.B. Run then traverse the tree using inorder, preorder, and postorderHint: ( inorder: left root right , preorder: root left right, postorder: left right root ) Write the pseudo code to delete ..... then draw the tree.D. Write the pseudo code to delete ....then draw the tree.E. Write the pseudo code to delete ..... then draw the tree.F. What is the search running time for trees that are not balanced?G. What is the search running time for a tree that is balanced?arrow_forwardPython binary search tree: a function that takes in a root, p, and checks whether the tree rooted in p is a binary search tree or not. What is the time complexity of your function? def is_bst(self, p: Node):arrow_forwardIn c++ 127, 125, 129,122, 128,126,130,131,124,123 Implement Binary tree using above values. Write a function to implement Depth First Search. Write code to implement Preorder, Inorder, Postorder traversal A function to display the values.arrow_forward
- Java Programming Language: 1. Make a class that accepts id numbers ranging from 1 to 29. 2. The id numbers are nodes of a binary tree. 3. Traverse the tree in inorder, preorder, and postorder and display the traversed values.arrow_forwardC++ DATA STRUCTURES Implement the TNode and Tree classes. The TNode class will include a data item name of type string,which will represent a person’s name. Yes, you got it right, we are going to implement a family tree!Please note that this is not a Binary Tree. Write the methods for inserting nodes into the tree,searching for a node in the tree, and performing pre-order and post-order traversals.The insert method should take two strings as input. The second string will be added as a child node tothe parent node represented by the first string. Hint: The TNode class will need to have two TNode pointers in addition to the name data member:TNode *sibling will point to the next sibling of this node, and TNode *child will represent the first child ofthis node. You see two linked lists here??? Yes! You’ll need to use the linked listsarrow_forwardComputer Science QUESTION: Expression tree is an application of binary tree to represent arithmetic expression. Write a program to implement an ExpressionTree class that can represent an arithmetic expression with binary operators and integer operands including floating-point. Limit the operators to addition, subtraction, multiplication and division. Operands can be constants or variables. Your class should be able to build a tree from its signature, and evaluate it on a set of values for the variable operands.arrow_forward
- To insert an element into a binary search tree (BST), you need to locate where to insert it in the tree. The key idea is to locate the parent for the new node. True Falsearrow_forward45 300 24 65 70 10 55 200 500 0 A. Convert it into a Binary Search Tree ( values are sorted in increasing order ), write the new values in the array. B. Traverse the tree in INORDER and print the values on the screen.arrow_forward127, 125, 129,122, 128,126,130,131,124,123• Implement Binary tree using above values. o Write a function to implement Depth First Search.o Write code to implement Preorder, Inorder, Postorder traversalo A function to display the values.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
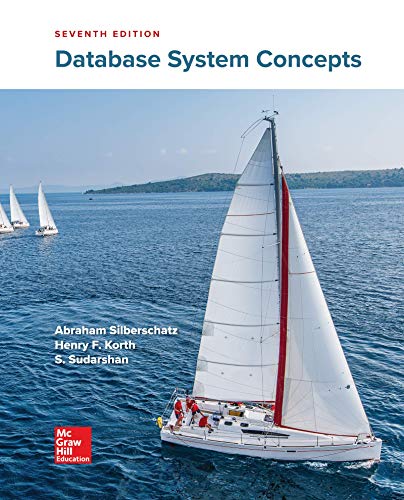
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
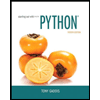
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
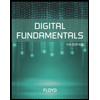
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
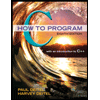
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
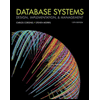
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
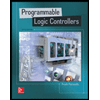
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education