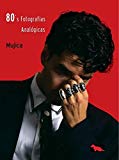
REVEL for Gaddis C++ -- Access Card (What's New in Computer Science)
1st Edition
ISBN: 9780134403922
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 21, Problem 4PC
Program Plan Intro
Height of the Binary Tree
Program Plan:
- Create a template prefix and define the template class BinaryTree to perform the following functions:
- Declare the required variables.
- Declare the function prototypes.
- Define the no-argument generic constructor BinaryTree() to initialize the root value as null.
- Call the functions insertNode(), remove(), displayInOrder(), and treeHeight().
- Define the generic function insert() to insert the node in position pointed by the tree node pointer in a tree.
- Define the generic function insertNode() to create a new node and it should be passed inside the insert() function to insert a new node into the tree.
- Define the generic function remove()which calls deleteNode() to delete the node.
- Define the generic function deleteNode() which deletes the node stored in the variable num and it calls the makeDeletion() function to delete a particular node passed inside the argument.
- Define the generic function makeDeletion()which takes the reference to a pointer to delete the node and the brances of the tree corresponding below the node are reattached.
- Define the generic function displayInOrder()to display the values in the subtree pointed by the node pointer.
- Define the generic function getTreeHeight() to count the height of the tree.
- Define the generic function TreeHeight()which calls getTreeHeight() to display the height of the tree.
- In main() function,
- Create a tree with integer data type to hold the integer values.
- Call the function treeHeight() to print the initial height of the tree.
- Call the function insertNode() to insert the node in a tree.
- Call the function displayInOrder() to display the nodes inserted in the order.
- Call the function remove() to remove the nodes from tree.
- Call the function treeHeight() to print the height of the tree after deleting the nodes.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ Data Structures (Binary Trees)- Write the definition of the function nodeCount that returns the number of nodes in the binary tree. Add this function to the class binaryTreeType and create a program to test this function. Here are the headers:
binaryTree.h
//Header File Binary Search Tree
#ifndef H_binaryTree
#define H_binaryTree
#include <iostream>
using namespace std;
//Definition of the Node
template <class elemType>
struct nodeType
{
elemType info;
nodeType<elemType> *lLink;
nodeType<elemType> *rLink;
};
//Definition of the class
template <class elemType>
class binaryTreeType
{
public:
const binaryTreeType<elemType>& operator=
(const binaryTreeType<elemType>&);
//Overload the assignment operator.
bool isEmpty() const;
//Function to determine whether the binary tree is empty.
//Postcondition: Returns true if the binary tree is empty;
// otherwise, returns false.
void inorderTraversal() const;
//Function to do an inorder…
In this chapter, the class to implement the nodes of a linked list is defined as a struct. The following rewrites the definition of the struct nodeType so that it is declared as a class and the member variables are private.
template <class Type>class nodeType{public:const nodeType<Type>& operator=(const nodeType<Type>&);//Overload the assignment operator.void setInfo(const Type& elem);//Function to set the info of the node.//Postcondition: info = elem;Type getInfo() const;//Function to return the info of the node.//Postcondition: The value of info is returned.void setLink(nodeType<Type> *ptr);//Function to set the link of the node.//Postcondition: link = ptr;nodeType<Type>* getLink() const;//Function to return the link of the node.//Postcondition: The value of link is returned.nodeType();//Default constructor//Postcondition: link = NULL;nodeType(const Type& elem, nodeType<Type> *ptr);//Constructor with parameters//Sets info point to…
In this chapter, the class to implement the nodes of a linked list is defined as a struct .
The following rewrites the definition of the struct nodeType so that it is declared as a class and the member variables are private.
template <class Type> class nodeType{ public: const nodeType<Type>& operator=(const nodeType<Type>&); //Overload the assignment operator.
void setInfo(const Type& elem); //Function to set the info of the node. //Postcondition: info = elem;
Type getInfo() const; //Function to return the info of the node. //Postcondition: The value of info is returned.
void setLink(nodeType<Type> *ptr); //Function to set the link of the node. //Postcondition: link = ptr;
nodeType<Type>* getLink() const; //Function to return the link of the node. //Postcondition: The value of link is returned.
nodeType(); //Default constructor //Postcondition: link = nullptr;…
Chapter 21 Solutions
REVEL for Gaddis C++ -- Access Card (What's New in Computer Science)
Ch. 21.1 - Prob. 21.1CPCh. 21.1 - Prob. 21.2CPCh. 21.1 - Prob. 21.3CPCh. 21.1 - Prob. 21.4CPCh. 21.1 - Prob. 21.5CPCh. 21.1 - Prob. 21.6CPCh. 21.2 - Prob. 21.7CPCh. 21.2 - Prob. 21.8CPCh. 21.2 - Prob. 21.9CPCh. 21.2 - Prob. 21.10CP
Ch. 21.2 - Prob. 21.11CPCh. 21.2 - Prob. 21.12CPCh. 21 - Prob. 1RQECh. 21 - Prob. 2RQECh. 21 - Prob. 3RQECh. 21 - Prob. 4RQECh. 21 - Prob. 5RQECh. 21 - Prob. 6RQECh. 21 - Prob. 7RQECh. 21 - Prob. 8RQECh. 21 - Prob. 9RQECh. 21 - Prob. 10RQECh. 21 - Prob. 11RQECh. 21 - Prob. 12RQECh. 21 - Prob. 13RQECh. 21 - Prob. 14RQECh. 21 - Prob. 15RQECh. 21 - Prob. 16RQECh. 21 - Prob. 17RQECh. 21 - Prob. 18RQECh. 21 - Prob. 19RQECh. 21 - Prob. 20RQECh. 21 - Prob. 21RQECh. 21 - Prob. 22RQECh. 21 - Prob. 23RQECh. 21 - Prob. 24RQECh. 21 - Prob. 25RQECh. 21 - Prob. 1PCCh. 21 - Prob. 2PCCh. 21 - Prob. 3PCCh. 21 - Prob. 4PCCh. 21 - Prob. 5PCCh. 21 - Prob. 6PCCh. 21 - Prob. 7PCCh. 21 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Hi everyone, can somone help me with this Programming Assignment from Data Structures. Thank you.In this assignment, you will use a modified Node structure to create a “Double Ended Doubly Linked List” data structure with extended functionality. The Node structure is defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; public Node previous; } The Double Ended Doubly Linked List (DEDLL) class definition is as follows: public class DEDLL { public int currentSize; public Node head; public Node tail; } This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The…arrow_forwardTree Define a class called TreeNode containing three data fields: element, left and right. The element is a generic type. Create constructors, setters and getters as appropriate. Define a class called BinaryTree containing two data fields: root and numberElement. Create constructors, setters and getters as appropriate. Define a method balanceCheck to check if a tree is balanced. A tree being balanced means that the balance factor is -1, 0, or 1.arrow_forwardHelp with codes and using C++ I will appreciate it and thumbs up // Templated binary search tree template<typename Type> class BST { friend class DSA_TestSuite_Lab7; // Giving access to test code struct Node { Type data; // The value being stored Node* left, * right; // The left and right nodes Node* parent; // The parent node // Constructor // Always creates a leaf node // // In: _data The value to store in this node // _parent The parent pointer (optional) Node(const Type& _data, Node* _parent = nullptr) { // TODO: Implement this method } }; // Data members Node* mRoot; // The top-most Node in the tree public: // Default constructor // Always creates an empty tree BST() { // TODO: Implement this method } // Destructor // Clear all dynamic memory ~BST() { // TODO: Implement this method } // Copy constructor // Used to initialize one object to another // // In: _copy The object to copy from BST(const BST<Type>& _copy) { // TODO: Implement this…arrow_forward
- Given the following definition of a node and binary search tree, write the member functions described below. You may declare and implement more functions if you need but you need to fully code any function you call. Any function you call will calculate towards runtime of your function. Please ensure that the run time requirement is met to get full marks class BST{ struct Node{ int data_; Node* left_; Node* right_; Node(int dat){ data_=dat; left_=right_=nullptr; } }; Node* root_; ...}; Write the following member functions of BST: bool BST::removeSmallest(int v); Runtime requirement O(log n) (assuming tree is balanced) This function removes the smallest value from subtree with root value of varrow_forwardBinary search tree. Write a function named totalSum that takes as parameter the root of the binary search tree(with the following type) and returns the total sum of the numbers in the tree. struct tree{ int data; struct tree *left, *right; };arrow_forwardHELP Write C code that implements a soccer team as a linked list. 1. Each node in the linkedlist should be a member of the team and should contain the following information: What position they play whether they are the captain or not Their pay 2. Write a function that adds a new members to this linkedlist at the end of the list.arrow_forward
- 12.7 The BinaryTree class is a recursive data structure, unlike the List class.Describe how the List class would be different if it were implemented as arecursive data structure.12.8 The parent reference in a BinaryTree is declared protected and isaccessed through the accessor methods parent and setParent. Why is this anydifferent than declaring parent to be public.arrow_forwardTwo stacks of the same kind are the same if they contain the same number of elements and have the same items at the same places. Overload the relational operator == for the class stackType, which returns true if two stacks of the same type are identical; otherwise, it returns false. Also, write the function template definition to overload this operator. Create a programme to test the overloaded operators and functions of the stackType class.arrow_forwardJava Your Java project has a class named Tree with the current class header: public class Tree { Now you want to make a collection of Tree objects and be able to sort them. Rewrite the entire class header to make this possible. b. In a UML class diagram for a class named Computer that has a private String field named model, you want to represent a standard getter. Write the line you would enter to do this. (To make the spacing work, use the general rule to put a space between any symbol and the words to either side of it.)arrow_forward
- write in c++ Define the 3 bolded functions for the following DynIntStack (linked list): class DynIntStack {private: struct Node { int value; // Value in the node Node *next; // Pointer to the next node }; Node *top; // Pointer to the stack toppublic: DynIntStack() { head = nullptr; } void push(int); //assume this is already defined void removeTop(); // removes the top element without returning it int topValue(); // returns the top element without removing it bool isEmpty() { return head == nullptr; } bool isFull() { return false; } void pushMany(int values[], int n); //add n values from the array}; Hints: void removeTop() (hint 3 lines of code) int topValue() (hint 1 line of code) void pushMany(int values[], int n) (hint 2 lines of code, use a for loop, call another function)arrow_forwardCalculator Assignment Modify a calculator program that currently displays the Abstract Syntax Tree (AST) structure after parsing an input expression. Instead of printing the AST, enhance the program to evaluate the expression and display the result. Add support for the binary "%" remainder (modulo) operator and introduce variables with the assignment operator "=". Key steps to implement the assignment operator and variables: Introduce a new static attribute in the AstNode class to represent variables: Add a method isIdentifier() to the TokenStream class to detect variables: Modify the primaryRule() in AstNode to handle variables and variable assignment: Handle new node types in the AstNode evaluate() method for variables and assignment: These changes allow the calculator to evaluate expressions, support variables, and handle variable assignments. Users can create and use variables in subsequent expressions.EmployeeJava Type: package week3;import static week3.MainApp.*; public enum…arrow_forwardtree algorithm The program must be in Java or Python under the object-oriented methodology They will have to develop a tree algorithmThe tree will be programmed using linked listsThe program must have classes, objects, public attributes and at least one private attribute, methods, constructor, inheritance, polymorphism, exception handling, ToString method usage, constructor overloadingarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
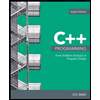
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning