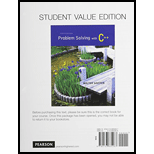
Write a
The user is asked how many cards she or he has, and the user responds with one of the integers 2, 3, 4, or 5. The user is then asked for the card values. Card values are 2 through 10, jack, queen, king, and ace. A good way to handle input is to use the type char so that the card input 2, for example, is read as the character '2', rather than as the number 2. Input the values 2 through 9 as the characters '2' through '9'. Input the values 10, jack, queen, king, and ace as the characters 't', 'j', 'q', 'k', and 'a'. (Of course, the user does not type in the single quotes.) Be sure to allow upper- as well as lowercase letters as input.
After reading in the values, the program should convert them from character values to numeric card scores, taking special care for aces. The output is either a number between 2 and 21 (inclusive) or the word Busted. You are likely to have one or more long multiway branches that use a switch statement or nested if-else statement. Your program should include a loop that lets the user repeat this calculation until the user says she or he is done.

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
Additional Engineering Textbook Solutions
Experiencing MIS
Starting Out with Java: From Control Structures through Objects (6th Edition)
Starting out with Visual C# (4th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Modern Database Management (12th Edition)
Introduction to Programming Using Visual Basic (10th Edition)
- Write a program that plays a guessing game with the user. The user should pick a letter, and the computer should try to guess the letter. After each guess, the user should tell the computer whether its guess was too high or too low. With this information, the computer should be able to guess the letter within five tries. The user should be able to give whole words or single upper or lower case letters as responses. c++arrow_forwardWrite a program that plays rock paper scissors against the user. Ask the user for what they want to throw (rock, paper, or scissors) and then have the computer randomly pick their throw. Then check to see who wins (or if it’s a tie). You can interpret 1 as rock, 2 as paper, and 3 as scissors if you’d like (or any other combination of numbers). 2. Craps is a dice game played at many casinos. A player rolls a pair of normal six-sided dice. If the initial roll is 2, 3, or 12, the player loses. If the roll is 7 or 11, the player wins. Any other initial roll causes the player to roll for point. That is, the player keeps rolling the dice until either rolling a 7 or re-rolling the value of the initial roll. If the player re-rolls the initial value before rolling a 7, it’s a win. Rolling a 7 first is a loss. Write a program to simulate multiple games of craps and estimate the probability that the player wins. For example, if the player wins 249 out of 500 games, then the estimated probability…arrow_forwardWrite a program that reads two integers as input, and outputs the first integer and subsequent increments of 10 as long as the value is less than or equal to the second integer. Answer in Coral Language Ex: If the input is: -15 30 the output is: -15 -5 5 15 25 Ex: If the second integer is less than the first as in: 20 5 the output is: Second integer can't be less than the first. For coding simplicity, output a space after every integer, including the last.arrow_forward
- Write a program that displays the classic BINGO game, displays a BINGO card (5x5 square), and tests the bingo card for a winner via 2 usersarrow_forwardWrite a program that simulates a snail trying to crawl up a building of height 6steps. The snail starts on the ground, at height 0. In each iteration, the snaileither crawls up one step, or slips off one step and falls all the way back to theground. In each iteration you would take a number as input from user and if the number is less 50 than the snail will slip otherwise it will crawl up. The program should keep going until the snail gets to the top of the building. It should then print out the number of falls that the snail took before it finally reached the top.Here is a sample execution:Iteration 1: Please enter a number between [1,100]: 60 Snail Moved: UpIteration 2: Please enter a number between [1,100]: 60 Snail Moved: UpIteration 2: Please enter a number between [1,100]: 40 Snail Moved: DownNumber of falls: 8arrow_forwardWrite a Java program for a Play-House Manager, who would like to calculate the total profit from selling tickets of the Drama. There are 3 types of tickets: Balcony (120 AED), VIP (70 AED) and Basic (40 AED). The manager has bills and salaries to pay. Therefore, the profit is equal to (incomes – expenses). last time it was wrong codearrow_forward
- Write a program that reads an integer age of kids, finds the maximum of them, and counts its occurances, finds the minimum of them, and counts its occurances. And Find the average ages. Assum that the input ends with number -1.arrow_forward"Write a program that reads true positives and false positives for each model and prints \"Good model\" and the value of the precision if the precision is above 0.75 or \"Bad model\" and the value of the precision if the precision is below 75. Teste the two models with your program and determine which one (or both) are good or bad models."arrow_forwardWrite a program with total change amount in pennies as an integer input, and output the change using the fewest coins, one coin type per line. The coin types are Dollars, Quarters, Dimes, Nickels, and Pennies. Use singular and plural coin names as appropriate, like 1 Penny vs. 2 Pennies. Ex: If the input is: 0 the output is: No change Ex: If the input is: 45 the output is: 1 Quarter 2 Dimesarrow_forward
- Write a program which reads two integers and prints out the sum, the differenceand the product. Divide them too, printing your answer to two decimal places.Also print the remainder after the two numbers are divided. Introduce a test to ensure that when dividing the numbers, the second number isnot zeroarrow_forwardWrite a program that implements a tip calculator. Your program should ask the user for the bill amount and the percentage of the tip, and then output the dollar amount of the tip and the final bill in three different formats. (see sample below) Example: Enter the bill amount: 27.95 Enter tip percentage: 17 A 17.0% tip on $27.95 equals $4.7515. The total bill is: 32.701499999999996 The total bill is $32.7. The total bill is $32.70.arrow_forwardWrite a program that prompts the capacity, in gallons, of an automobile fuel tank and the miles per gallon theautomobile can be driven without refueling.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
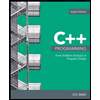