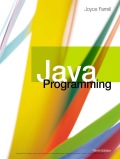
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 5, Problem 3GZ
Program Plan Intro
Card game
Program plan:
- In a file “Card.java”, create a class “Card”,
- Declare and initialize the necessary variables.
- Define the method “get_Suit()” to return the suit value.
- Define the method “get_Value()” to return the value.
- Define the method “set_Suit()” to set the suit value.
- Define the method “set_Value()”,
- Check whether the value is greater than or equal to low and less than or equal to high value,
- If it is true, set the given integer as the value.
- Otherwise,
- Set the lowest value.
- Check whether the value is greater than or equal to low and less than or equal to high value,
- In a file “War.java”, create a class “War”,
- Define the method “main ()”,
- Declare and initialize the necessary variables.
- Create two objects for “Card” class.
- Generate the random value for the player.
- Generate the random value for the computer.
- Set the value for the player and the computer.
- Generate the random suit for the player and the computer.
- Check whether the random value and the suite value of the player is same as that of computer,
- If it is true, increment the computer’s suit value by “1”.
- Check whether the computer’s suit value is greater than the highest value,
- Set the computer’s suit value to “1”.
- Check whether the player’s suit is “1”,
- If it is true, call the method “set_Suit()” with “s” as the parameter.
- Otherwise, check whether the player’s suit is “2”,
- If it is true, call the method “set_Suit()” with “h” as the parameter.
- Otherwise, check whether the player’s suit is “3”,
- If it is true, call the method “set_Suit()” with “d” as the parameter.
- Otherwise,
- Call the method “set_Suit()” with “c” as the parameter.
- Check whether the computer’s suit value is “1”,
- If it is true, call the method “set_Suit()” with “s” as the parameter.
- Otherwise, check whether the computer’s suit is “2”,
- If it is true, call the method “set_Suit()” with “h” as the parameter.
- Otherwise, check whether the computer’s suit is “3”,
- If it is true, call the method “set_Suit()” with “d” as the parameter.
- Otherwise,
- Call the method “set_Suit()” with “c” as the parameter.
- Print the messages in the console.
- Check whether the player’s value is same as the computer’s value,
- If it is true, print the string “It’s a tie”.
- Otherwise, check whether the player’s value is greater than the computer’s value,
- If it is true, print the string “I win”.
- Otherwise, print the string “You win”.
- Define the method “main ()”,
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
This is the question -
Create a class named Apartment that holds an apartment number, number of bedrooms, number of baths, and rent amount. Create a constructor that accepts values for each data field. Also create a get method for each field.
Write an application that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or No apartments met your criteria if no such apartments are available.
This is the code it has given and I have done some on-
public class Apartment {
int aptNumber;
int bedrooms;
double baths;
double rent;
public Apartment(int num, int bdrms, double bths, double rent) {
}
public int getAptNumber() {
return aptNumber;
}
public int getBedrooms() {
retrun bedrooms;
}
public double getBaths() {…
This is the question -
Create a class named Apartment that holds an apartment number, number of bedrooms, number of baths, and rent amount. Create a constructor that accepts values for each data field. Also create a get method for each field.
Write an application that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or No apartments met your criteria if no such apartments are available.
This is the code I already have. It doesn't seem to like under TestApartments my apt. variable. I am unsure what to do to fix this cause it doesn't like any of my code -
public class Apartment {
int aptNumber;
int bedrooms;
double baths;
double rent;
public Apartment(int num, int bdrms, double bths, double rent) {
//write code here
aptNumber = num;…
Read the instructions below and write your code using Microsoft Visual Studio (Community version).
Build your own console application with C#.
Write a Rectangle class, the private data members are the length (len) and width (wid) of the rectangle, the parameterless constructor sets len and wid to 0, and the parameterized constructor sets the value of length (len) and width (wid). In addition, the class also includes common methods such as calculating the perimeter of the rectangle, calculating the area of the rectangle, obtaining the length value of the rectangle, obtaining the width value of the rectangle, and modifying the length and width values of the rectangle to the corresponding formal parameter values.
Please upload your executable C# file as attachment in the system.
Chapter 5 Solutions
EBK JAVA PROGRAMMING
Ch. 5 - Prob. 1RQCh. 5 - Prob. 2RQCh. 5 - Prob. 3RQCh. 5 - Prob. 4RQCh. 5 - Prob. 5RQCh. 5 - Prob. 6RQCh. 5 - Prob. 7RQCh. 5 - Prob. 8RQCh. 5 - Prob. 9RQCh. 5 - Prob. 10RQ
Ch. 5 - Prob. 11RQCh. 5 - Prob. 12RQCh. 5 - Prob. 13RQCh. 5 - Prob. 14RQCh. 5 - Prob. 16RQCh. 5 - Prob. 17RQCh. 5 - Prob. 18RQCh. 5 - Prob. 19RQCh. 5 - Prob. 20RQCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 3PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 1GZCh. 5 - Prob. 2GZCh. 5 - Prob. 3GZCh. 5 - Prob. 4GZCh. 5 - Prob. 5GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The button1_Click() method that is generated by the IDE ___________. has a private access specifier is nonstatic contains parameters between its parentheses all of the abovearrow_forwardIn this exercise, you modify the Grade Calculator application from this chapter’s Apply lesson. Use Windows to make a copy of the Grade Solution folder. Rename the copy Grade Solution-Intermediate. Open the Grade Solution.sln file contained in the Grade Solution-Intermediate folder. Open the CourseGrade.vb file. The DetermineGrade method should accept an integer that represents the total number of points that can be earned in the course. (Currently, the total number of points is 200: 100 points per test.) For an A grade, the student must earn at least 90% of the total points. For a B, C, and D grade, the student must earn at least 80%, 70%, and 60%, respectively. If the student earns less than 60% of the total points, the grade is F. Make the appropriate modifications to the DetermineGrade method and then save the solution. Unlock the controls on the form. Add a label control and a text box to the form. Change the label control’s Text property to “&Maximum points:” (without the quotation marks). Change the text box’s name to txtMax. Lock the controls and then reset the tab order. Open the form’s Code Editor window. The txtMax control should accept only numbers and the Backspace key. Code the appropriate procedure. The grade should be cleared when the user makes a change to the contents of the txtMax control. Code the appropriate procedure. Modify the frmMain_Load procedure so that each list box displays numbers from 0 through 200. Locate the btnDisplay_Click procedure. If the txtMax control does not contain a value, display an appropriate message. The maximum number allowed in the txtMax control should be 400; if the control contains a number that is more than 400, display an appropriate message. The statement that calculates the grade should pass the maximum number of points to the studentGrade object’s DetermineGrade method. Make the necessary modifications to the procedure. Save the solution and then start and test the application.arrow_forwardQ2:Assume that we have a Button object named btnPrint, and a method named print(). Write a statement that will cause the button to trigger a call to the print method.arrow_forward
- Create a class named Apartment that holds an apartment number, number of bedrooms, number of baths, and rent amount. Create a constructor that accepts values for each data field. Also create a get method for each field. Write an application that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent that the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or an appropriate message if no such apartments are available.arrow_forwardUsing the Circle2D class you defined in Exercise 8.18, write a program that enables the user to specify the location and size of two circles and displays whether the circles intersect, as shown in Figure 12.20. Enable the user to point the mouse inside a circle and drag it. As a circle is being dragged, the program updates the circle’s center coordinates and its radius in the text fields.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
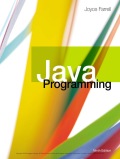
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
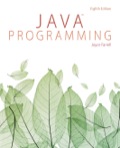
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
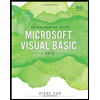
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
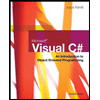
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY