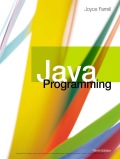
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Question
Chapter 5, Problem 7PE
Program Plan Intro
Job application
Program plan:
- In a file “JobApplicant.java”, create a class “JobApplicant”,
- Declare the necessary variables.
- Define the constructor,
- Assign the initial values for the job applicant.
- Define the method “get_Name()”,
- Return the name.
- Define the method “get_Phone()”,
- Return the phone number.
- Define the method “get_HasWordSkill()”,
- Return the Boolean value.
- Define the method “get_HasSpreadsheetSkill()”,
- Return the Boolean value.
- Define the method “get_HasDatabaseSkill()”,
- Return the Boolean value.
- Define the method “get_HasGraphicsSkill()”,
- Return the Boolean value.
- In a file “TestJobApplicants.java”, create a class “TestJobApplicants”,
- Define the method “main ()”,
- Call the constructor “JobApplicant()” that instantiates several job applicant objects.
- Assign the text messages.
- Execute for “true” statement for the first applicant.
- Call the method “display1()” with qualified message.
- Otherwise, call the method “display1()” with non-qualified message.
- Execute for “true” statement for the second applicant.
-
- Call the method “display1()” with qualified message.
- Otherwise, call the method “display1()” with non-qualified message.
- Execute for “true” statement for the third applicant.
-
- Call the method “display1()” with qualified message.
- Otherwise, call the method “display1()” with non-qualified message.
- Execute for “true” statement for the fourth applicant.
-
- Call the method “display1()” with qualified message.
- Otherwise, call the method “display1()” with non-qualified message.
- Call the method “display1()” with qualified message.
- Define the method “is_Qualified()” that accepts each job applicant properties,
- Set the count to “0”.
- Declare Boolean variable.
- Declare the final variable.
- Execute for the Boolean value at “word”,
- Increment the count by “1”.
- Execute for the Boolean value at “sp”,
-
- Increment the count by “1”.
- Execute for the Boolean value at “db”,
-
- Increment the count by “1”.
- Execute for the Boolean value at “gr”,
-
- Increment the count by “1”.
- Check whether the count is greater than or equal to minimum number of skills,
-
- If it is true, set the Boolean value to “true”.
- Otherwise, set the Boolean value to “false”.
- Return the Boolean value.
- Increment the count by “1”.
- Define the method “display1()”,
- Print the output.
- Define the method “main ()”,
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
This is java question:
Create a class named Bottle, which contains the following attributes: isFull (boolean), drinkType (ex: water), fillLevel (max: 100). Then, create a method called drink, which takes an argument of how much liquid the user drinks. When the user calls drink, subtract the amount drank from the fillLevel and make sure that isFull is set to false. Lastly, create a constructor that initializes the object as a full bottle containing water.
Can you write it in JAVA programming language
Add a toString method to your Account class. For the purposes of this assignment, please use the toString display the following:
This account contains $x. You have earned $y in the last month.
where x is the account balance (please don't format the decimals) and y is the monthly interest earned, obtained from the getMonthlyInterest method.
Compile and test in a driver by creating and printing an Account object.
Add an equals method to your Account class. Two Account objects are equal if their balance and annualInterestRates are equal.
Compile and test in your driver by creating 2 Account objects to see if they are equal.
Create a class called Complex for performing arithmetic with complex numbers. Complex numbers have the form
realPart + imaginaryPart * i where i is
-1
Write a program to test your class. Use floating-point variables to represent the private data of the class. Provide a constructor that enables an object of this class to be initialized when it is declared. Provide a no-argument constructor with default values in case no initializers are provided. Provide public methods that perform the following operations:
a) Add two Complex numbers: The real parts are added together and the imaginary parts are added together.
b) Subtract two Complex numbers: The real part of the right operand is subtracted from the real part of the left operand, and the imaginary part of the right operand is sub- tracted from the imaginary part of the left operand.
c) Print Complex numbers in the form (a, b), where a is the real part and b is the imaginary part.
Chapter 5 Solutions
EBK JAVA PROGRAMMING
Ch. 5 - Prob. 1RQCh. 5 - Prob. 2RQCh. 5 - Prob. 3RQCh. 5 - Prob. 4RQCh. 5 - Prob. 5RQCh. 5 - Prob. 6RQCh. 5 - Prob. 7RQCh. 5 - Prob. 8RQCh. 5 - Prob. 9RQCh. 5 - Prob. 10RQ
Ch. 5 - Prob. 11RQCh. 5 - Prob. 12RQCh. 5 - Prob. 13RQCh. 5 - Prob. 14RQCh. 5 - Prob. 16RQCh. 5 - Prob. 17RQCh. 5 - Prob. 18RQCh. 5 - Prob. 19RQCh. 5 - Prob. 20RQCh. 5 - Prob. 1PECh. 5 - Prob. 2PECh. 5 - Prob. 3PECh. 5 - Prob. 4PECh. 5 - Prob. 5PECh. 5 - Prob. 6PECh. 5 - Prob. 7PECh. 5 - Prob. 8PECh. 5 - Prob. 9PECh. 5 - Prob. 10PECh. 5 - Prob. 1GZCh. 5 - Prob. 2GZCh. 5 - Prob. 3GZCh. 5 - Prob. 4GZCh. 5 - Prob. 5GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a program named DemoJobs for Harolds Home Services. The program should instantiate several Job objects and demonstrate their methods. The Job class contains four data fields—description (for example, wash windows), time in hours to complete (for example, 3.5), per-hour rate charged (for example, $25.00), and total fee (hourly rate times hours). Include properties to get and set each field except the total fee—that field will be read-only, and its value is calculated each time either the hourly fee or the number of hours is set. Overload the + operator so that two Jobs can be added. The sum of two Jobs is a new Job containing the descriptions of both original Jobs (joined by and), the sum of the time in hours for the original Jobs, and the average of the hourly rate for the original Jobs. Harold has realized that his method for computing the fee for combined jobs is not fair. For example, consider the following: His fee for painting a house is $100 per hour. If a job takes 10 hours, he earns $1000. His fee for dog walking is $10 per hour. If a job takes 1 hour, he earns $10. If he combines the two jobs and works a total of 11 hours, he earns only the average rate of $55 per hour, or $605. Devise an improved, weighted method for calculating Harolds fees for combined Jobs and include it in the overloaded operator+() method. Write a program named DemoJobs2 that demonstrates all the methods in the class work correctly.arrow_forwardWrite a program named DemoJobs for Harold’s Home Services. The program should instantiate several Job objects and demonstrate their methods. The Job class contains four data fields—description (for example, “wash windows”), time in hours to complete (for example, 3.5), per-hour rate charged (for example, $25.00), and total fee (hourly rate times hours). Include properties to get and set each field except the total fee—that field will be read-only, and its value is calculated each time either the hourly fee or the number of hours is set. Overload the + operator so that two Jobs can be added. The sum of two Jobs is a new Job containing the descriptions of both original Jobs (joined by and), the sum of the time in hours for the original Jobs, and the average of the hourly rate for the original Jobs.arrow_forwardCreate a class called Invoice that a hardware store might use to represent an invoice for an item sold at the store. An Invoice should include four information as instance variables: a part number (type String), a part description (type String), a quantity of the item being purchased (type int) and a price per item (double). Your class should have a constructor that initializes the four instance variables. Provide a set and a get method for each instance variable. In addition, provide a method named getInvoiceAmount that calculates the invoice amount (i.e., multiplies the quantity by the price per item), then returns the amount as a double value. If the quantity is not positive, it should be set to 0. If the price per item is not positive, it should be set to 0.0. Write a tester class named InvoiceTest that demonstrates class Invoice’s capabilities by creating two invoices then changing and printing their characteristics.arrow_forward
- Write a class called Alien. The Alien class must have one field of type String called name, one of type String called planet and one field of type boolean called humanoid. Write one constructor that takes three parameters to initialise all of these fields. The parameters must be passed in the order name, planet and humanoid status. IntelliJ hint (this won't be in the real test): put your cursor after the last field; right-click, choose Generate, choose Constructor, select the fields that are set via parameters and click Ok. Check that the constructor is correct. Write a getter method for each field. IntelliJ hint (this won't be in the real test): put your cursor after the closing curly bracket of the constructor; right click, choose Generate, choose Getter, select all of the fields; click Ok. Check that the you have three getters. Do not write setters methods. Write a method called getDetails that will return the values of the fields in one of the following two formats. For example,…arrow_forwardWrite Rectangle class to represent a rectangle. The class contains:a. Two double data fields named width and height that specify the width and height ofthe rectangle. The default values are 1 for both width and height.b. A no-arg constructor that creates a default rectangle.c. A constructor that creates a rectangle with the specified width and height.d. A method named getArea() that returns the area of this rectangle.e. A method named getPerimeter() that returns the perimeter. in Javaarrow_forwardPart A Create a class named Apartment that holds an apartment number as aptNumber, number of bedrooms as bedrooms, number of baths as baths, and rent amount as rent. Create a default constructor that accepts no arguments and an overloaded constructor that accepts values for each data field. Also create a get method for each field. Part B Write an application called TestApartments that creates at least five Apartment objects. Then prompt a user to enter a minimum number of bedrooms required, a minimum number of baths required, and a maximum rent the user is willing to pay. Display data for all the Apartment objects that meet the user’s criteria or an appropriate message if no such apartments are available. An example of the program is shown below: Enter minimum number of bedrooms needed >> 2 Enter minimum number of bathrooms needed >> 1.5 Enter maximum rent willing to pay >> 1200 Apartments meeting criteria of at least 2 bedrooms, at least 1.5 baths, and no more than…arrow_forward
- Create a class named Pay that includes five double variables that hold hours worked, rate of pay per hour, withholding rate, gross pay, and net pay. Create three overloaded computeNetPay() methods. When computeNetPay() receives values for hours, pay rate, and withholding rate, it computes the gross pay and reduces it by the appropriate withholding amount to produce the net pay. (Gross pay is computed as hours worked multiplied by pay per hour.) When computeNetPay() receives two parameters, they represent the hours and pay rate, and the withholding rate is assumed to be 15%. When computeNetPay() receives one parameter, it represents the number of hours worked, the withholding rate is assumed to be 15%, and the hourly rate is assumed to be 5.85. Write a main() method that tests all three overloaded methods. Save the application as Pay.java.arrow_forwardCreate a class named Checkup with fields that hold a patient number, two blood pressure figures (systolic and diastolic), and two cholesterol figures (LDL and HDL). Include methods to get and set each of the fields. Include a method named computeRatio() that divides LDL cholesterol by HDL cholesterol and displays the result. Include an additional method named explainRatio() that explains that HDL is known as “good cholesterol” and that a ratio of 3.5 or lower is considered optimum. Save the class as CheckupType.cpp. Create a tester program named TestCheckup whose main() method declares four Checkup objects. Call a getData() method four times. Within the method, prompt a user for values for each field for a Checkup, and return a Checkup object to the main() method where it is assigned to one of main()’s Checkup objects. Then, in main(), pass each Checkup object in turn to a showValues()method that displays the data. Blood pressure values are usually displayed with a slash between the…arrow_forward1. Write an app that computes student average2. Create a class called Student3. Use the init () function to collect the student information: name, math, science, and English grade4. Create a method (class function) that computes the average5. Create another method that outputs (similarly to) the ff when calledName: John Math: 90Science: 90English: 90Average: 90 (Passed)6. Instantiate a Student object and utilize the defined methods***Let the user input that student infoarrow_forward
- My professor has given me additional information for this question: Create a class named CollegeCourse that includes data fields that hold thedepartment (for example, ENG), the course number (for example, 101), thecredits (for example, 3), and the fee for the course (for example, $360). All of thefields are required as arguments to the constructor, except for the fee, which iscalculated at $120 per credit hour. Include a display() method that displaysthe course data. Create a subclass named LabCourse that adds $50 to the coursefee. Override the parent class display() method to indicate that the course isa lab course and to display all the data. Write an application named UseCoursethat prompts the user for course information. If the user enters a class in any ofthe following departments, create a LabCourse: BIO, CHM, CIS, or PHY. If theuser enters any other department, create a CollegeCourse that does not includethe lab fee. Then display the course data. Save the files as…arrow_forwardAdd a toString method to your Account class. For the purposes of this assignment, please use the toString display the following: This account contains $x. You have earned $y in the last month. where x is the account balance (please don't format the decimals) and y is the monthly interest earned, obtained from the getMonthlyInterest method. Compile and test in a driver by creating and printing an Account object. Add an equals method to your Account class. Two Account objects are equal if their balance and annualInterestRates are equal. Compile and test in your driver by creating 2 Account objects to see if they are equal.arrow_forwardWrite Rectangle class to represent a rectangle. The class contains:a. Two double data fields named width and height that specify the width and height ofthe rectangle. The default values are 1 for both width and height.b. A no-arg constructor that creates a default rectangle.c. A constructor that creates a rectangle with the specified width and height.d. A method named getArea() that returns the area of this rectangle.e. A method named getPerimeter() that returns the perimeter.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
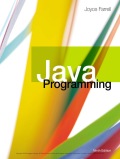
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
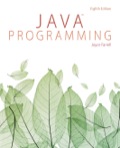
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
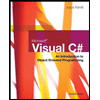
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Memory Management Tutorial in Java | Java Stack vs Heap | Java Training | Edureka; Author: edureka!;https://www.youtube.com/watch?v=fM8yj93X80s;License: Standard YouTube License, CC-BY