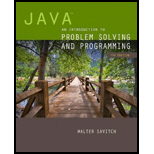
Explanation of Solution
a.
Method heading for each method:
- • Method heading for access method of each attribute:
- ○ Access method for person first name is “public String getPersonFirstName()”.
- ○ Access method for person last name is “public String getPersonLastName()”.
- ○ Access method for person email address name is “public String getPersonEmailAddress()”...
Explanation of Solution
b.
Preconditions and postconditions of each method:
- • Precondition and postcondition of access method for each attribute.
- ○ For method “getPersonFirstName()”:
- ■ Precondition: None
- ■ Postcondition: Returns the first name of given person.
- ○ For method “getPersonLastName()”:
- ■ Precondition: None
- ■ Postcondition: Returns the last name of given person.
- ○ For method “getPersonEmailAddress()”:
- ■ Precondition: None
- ■ Postcondition: Returns the email address of given person.
- ○ For method “getPersonTelephoneNumber()”:
- ■ Precondition: None
- ■ Postcondition: Returns the telephone number of given person.
- ○ For method “getPersonFirstName()”:
- • Precondition and postcondition of “changeEmailAddress(String nEmail)” method...
Explanation of Solution
c.
Test class for some Java statement:
//Create object "p1" for "PersonAddress" class
PersonAddress p1 = new PersonAddress();
//Create object "p2" for "PersonAddress" class
PersonAddress p2 = new PersonAddress();
//Create object "p3" for "PersonAddress" class
PersonAddress p3 = new PersonAddress();
//Set values for "p1" by calling method "setPersonAddress"
p1.setPersonAddress("John", "Merry", "john123@aaa.com", "123-4567");
//Set values for "p2" by calling method "setPersonAddress"
p2.setPersonAddress("John", "Merry", "jr@ccc.com", "123-7654");
//Set values for "p3" by calling method "setPersonAddress"
p3.setPersonAddress("Jansi", "Rose", "htr@aaa.com", "333-4444");
//Display the statement
System.out.println("Values of attributes for person 1.");
//Display person "p1" first name by calling method "getPersonFirstName"
System.out.println("First Name: " + p1.getPersonFirstName());
//Display person "p1" last name by calling method "getPersonLastName"
System.out.println("Last Name: " + p1.getPersonLastName());
//Display person "p1" email address by calling method "getPersonEmailAddress"
System.out.println("Email Address: " + p1.getPersonEmailAddress());
//Display person "p1" telephone number by calling method "getPersonTelephoneNumber"
System.out.println("Telephone Number: " + p1.getPersonTelephoneNumber());
System.out.println();
//Display the given statement
System...
Explanation of Solution
d.
Implementation of class:
PersonAddress.java:
//Import package
import java.util.Scanner;
//Define class "PersonAddress"
public class PersonAddress
{
//Declare required variables in "private" access specifier
private String first_Name;
private String last_Name;
private String email_addr;
private String telephone_number;
//Set value for "PersonAddress" class
public void setPersonAddress(String f, String l, String e, String p)
{
//Assign value to given instance variables
first_Name = f;
last_Name = l;
email_addr = e;
telephone_number = p;
}
//Access method for person first name
public String getPersonFirstName()
{
//Returns person first name
return first_Name;
}
//Access method for person last name
public String getPersonLastName()
{
//Returns person last name
return last_Name;
}
//Access method for person email address
public String getPersonEmailAddress()
{
//Returns person email address
return email_addr;
}
//Access method for person telephone number
public String getPersonTelephoneNumber()
{
//Returns person telephone number
return telephone_number;
}
//Method for change person Email Address
public void changeEmailAddress(String nEmail)
{
//Assign "email_addr" to "nEmail"
email_addr = nEmail;
}
//Method for change person PhoneNumber
public void changePhoneNumber(String nPhone)
{
//Assign "telephone_number" to "nPhone"
telephone_number = nPhone;
}
//Method for check two persons are equal
public boolean equalMethod(PersonAddress otherPerson)
{
//Returns true if persons are equal
return last_Name.equals(otherPerson.last_Name) && first_Name.equals(otherPerson.first_Name);
}
//Define main function
public static void main(String[] args)
{
//Create object "p1" for "PersonAddress" class
PersonAddress p1 = new PersonAddress();
//Create object "p2" for "PersonAddress" class
PersonAddress p2 = new PersonAddress();
//Create object "p3" for "PersonAddress" class
PersonAddress p3 = new PersonAddress();
//Set values for "p1" by calling method "setPersonAddress"
p1.setPersonAddress("John", "Merry", "john123@aaa.com", "123-4567");
//Set values for "p2" by calling method "setPersonAddress"
p2.setPersonAddress("John", "Merry", "jr@ccc.com", "123-7654");
//Set values for "p3" by calling method "setPersonAddress"
p3.setPersonAddress("Jansi", "Rose", "htr@aaa.com", "333-4444");
//Display the statement
System.out.println("Values of attributes for person 1.");
//Display person "p1" first name by calling method "getPersonFirstName"
System.out.println("First Name: " + p1.getPersonFirstName());
//Display person "p1" last name by calling method "getPersonLastName"
System.out.println("Last Name: " + p1.getPersonLastName());
//Display person "p1" email addressby calling method "getPersonEmailAddress"
System...

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Java: An Introduction to Problem Solving and Programming plus MyProgrammingLab with Pearson eText -- Access Card Package (7th Edition)
- Write a java program Write a class named Employee with name and salary as private data fields. Add a constructor with both parameters. Write a class named Manager which unherits the Employee class. Add an instance variable, named department, of type String and 15 private. Add a constructor in the subclass class and a toString () method that prints the manager's name, department, and salary [Hint: Add a tastanel) method in the super class as well]. Create objects of Manager and Employee dassarrow_forwardWrite a full class that represents a Phone, described by a name, price, and then whether the phone comes enabled with Bluetooth. The default value is that most phones come enabled. Include instance data, one or more constructors, getters and setters, a toString, and an equals method. Required validation for the variables Implement the Comparable interface. Order phones based on name and then price. Show make the driver in a different class to test the methods using user-inputarrow_forwardWrite a complete program for the description given below. Consider a Billing class that implements an interface Payable having a method getTotalPayment Amount(). Besides this, you have a Doctor class with private instance variables (docID, docName, and docFee) and a public getDoc() method, Patient class with private instance variables (pName, pID. pDisease), Medicine class with private instance variables (medID, medName, medQty, medPrice), and Medical Test class with private instance variables (testID, testName, testPrice). Each of these classes has the toString() method to display the information of its object. The Billing class is having "Has A" relationship with the other four classes (Doctor, Patient, Medicine, and MedicalTest) mentioned above. The getPayment Amount() method of Billing class returns the total billing amount that includes doc fee, medicine cost, and medical test fee that a patient has to pay. After implementing these classes, you are required to do the following in…arrow_forward
- 1/ Write a BankAccountTester class whose main method constructs a bank account, deposits $1,000, withdraws $500, withdraws another $400, and then prints the remaining balance. Also print the expected result 2/ Implement a class Employee. An employee has a name (a string) and a salary (a double). Provide a constructor with two arguments public Employee(String employeeName, double currentSalary) and methods public String getName() public double getSalary() public void raiseSalary(double byPercent) These methods return the name and salary, and raise the employee's salary by a certain percentage. Sample usage: Employee harry = new Employee("Hacker, Harry", 50000); harry.raiseSalary(10); // Harry gets a 10 percent raise Supply an EmployeeTester class that tests all methods. 3/Implement a class Car with the following properties. A car has a certain fuel efficiency (measured in miles/gallon or liters/km—pick one) and a certain amount of fuel in the gas tank. The efficiency is specified in…arrow_forwardWrite a class to model a television set. A TV has a constructor that takes the brand as a String. It also takes double , size, which is the screen size. Call the instance variables brand and size. The size is measured on the diagonal. TV has methods getSize() and getBrand(). TV implements the Comparable interface from the Java library. Do not write your own. TVs are ordered by size. If two TVs have the same size, then the TV with the brand that comes first in the lexicographical (alphabetical) order is the smaller. For Example, "LG" comes before "Sharp" alphabetically and so is the smaller. A toString method is provided for you. This is to help in testing your code. Provide Javadoc and remember that the compareTo method takes an Object as its parameter, not a TV (That means you will have to cast).arrow_forwardWrite a class to model a television set. A TV has a constructor that takes the brand as a String. It also takes double , size, which is the screen size. Call the instance variables brand and size. The size is measured on the diagonal. TV has methods getSize() and getBrand(). TV implements the Comparable interface from the Java library. Do not write your own. TVs are ordered by size. If two TVs have the same size, then the TV with the brand that comes first in the lexicographical (alphabetical) order is the smaller. For Example, "LG" comes before "Sharp" alphabetically and so is the smaller. A toString method is provided for you. This is to help in testing your code.arrow_forward
- Consider a class that could be used to play a game of hangman. The classhas the following attributes:- the secret word- the disguised word, in which each unknown letter is replaced with a question mark (?). For example, if the secret word isabracadabra and the letters a, b, and e have been guessed, the disguisedword would be ab?a?a?ab?a.- the number of guesses made- the number of incorrect guessesIt will have the following methods:- makeGuess(c) guesses that character c is in the word.- getDisguisedWord returns a string containing correctly guessed letters in their correct positions and unknown letters replaced with ?.- getSecretWord returns the secret word.- getGuessCount returns the number of guesses made.- isFound returns true if the hidden word has been discovered.a. write method heading for eeach methodb. write preconditions and postconditions for each methodc. write some java statements that test the classd. Implement the class.2. Write an application that plays the game of…arrow_forwardWrite a Java program with at least two classes. Use a circle object to represent it. The class must offer at least one (non-static) setter method and one (non-static) getter method. Implement at least one test that invokes the setter method and the corresponding getter method so that the value out == the value in. Also use assert.Equal(60, circle1.getCircumference(), 10); and assert.Equals(10, circle1.get Radius(), 0.1); for radiusarrow_forwardWrite a Java program for a class called MyPoint, which models a 2D point with x and y coordinates, is designed as shown in the class diagram. It contains: Two instance variables x (int) and y (int). A default (or "no-argument" or "no-arg") constructor that construct a point at the default location of (0, 0). A overloaded constructor that constructs a point with the given x and y coordinates. Getter and setter for the instance variables x and y. A method setXY() to set both x and y. A method getXY() which returns the x and y in a 2-element int array. A toString() method that returns a string description of the instance in the format "(x, y)". A method called distance(int x, int y) that returns the distance from this point to another point at the given (x, y) coordinates, e.g.,MyPoint p1 = new MyPoint(3, 4); System.out.println(p1.distance(5, 6)); An overloaded distance(MyPoint another) that returns the distance from this point to the given MyPoint instance (called another), e.g.,MyPoint…arrow_forward
- In Java Programming: Example, 8-8, defined the class Person to store the name of a person. The methods that we included merely set the name and print the name of a person. Redefine the class Person so that, in addition to what the existing class does, ypu can: 1. Set the last name only. 2. Set the first name only. 3 Set the middle name. 4. Check whether a given last name is the same as the last name of this person. 5. Check wether a given first name is the same as the first name of this person. 6. Check whether a given middle name is the same as the middle name of thi person. 7. Add the method equals that returns true if two objects contain the same first, middle, and last name. 8. Add the method makeCopy that copies the instance variables of a Person object into another Person object. 9. Add the method getCopy that creates and returns the address of the object, which is a copy of another Person object. 10. Add a copy constructor. 11. Write the definitions of the methods of the class…arrow_forwardWe have a parking office class for an object-oriented parking management system using java Add (implement) a method to the Parking Office class to return a collection of permit ids (getPermitIds) I have attached two class diagrams with definitions of all related classes in our system (i.e car, customer, .....). N.B. Parking office methods in the class definition like register, getcustomer and addcharge have already been implemented, we just need an additional getPermitIds method as mentioned abovearrow_forwardWe have a parking office class for an object-oriented parking management system using java Add(implement) a method to the Parking Office class to return the collection of permit ids for a specific customer (getPermitIds(Customer)) using java I have attached two class diagrams with definitions of all related classes in our system (i.e car, customer, .....). N.B. Parking office methods in the class definition like register, getcustomer and addcharge have already been implemented, we just need an additional getPermitIds(Customer) method as mentioned above Explain your code in a few words Important- We have a method to return a collection of permit ids (getPermitIds), what we need is a method to return the collection of permit ids for a specific customer (getPermitIds(Customer))arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
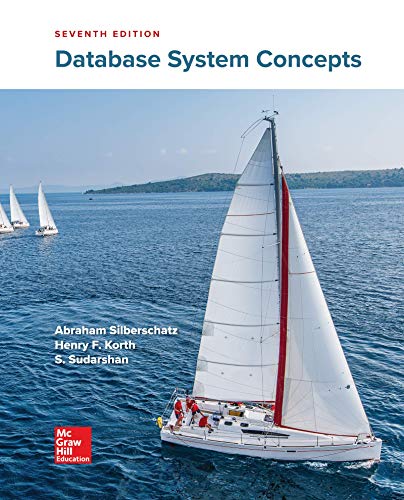
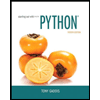
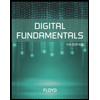
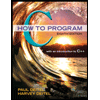
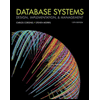
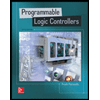