Output of the Program:
The given program has been added with comments and line number.
/*Include required variables*/
#include <iostream> //Line 1
using namespace std; //Line 2
/*Function prototype*/
void myFunc();//Line 3
/*Main function*/
int main()//Line 4
{//Line 5
/*Variable "var" is declared as "int" data type and it is assigned with the value "100"*/
int var = 100; //Line 6
/*Print the value*/
cout << var << endl; //Line 7
/*Call the function "myFunc()"*/
myFunc();//Line 8
/*Display the value of variable "val" after calling the function*/
cout << var << endl; //Line 9
/*Return the value 0*/
return 0; //Line 10
}//Line 11
/* Function definition */
void myFunc()//Line 12
{//Line 13
/*Variable "var" is declared as "int" data type and it is assigned with the value "50"*/
int var = 50; //Line 14
/* Print the value of the variable "var" */
cout << var << endl; //Line 15
}//Line 16

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
STARTING OUT WITH C++ REVEL >IA<
- Please help me explain the following void function c++ code, which I did not understand. Especially for the first void function and int main(), I do not understand why the first void function enter the number and the int main function print out the final answer. #include <iostream>using namespace std; void getNumber(int *input); void doubleValue(int *val); int main(){ int number; getNumber(&number); doubleValue(&number); cout<<"That value doubled is"<<number<<endl; return 0;}void getNumber(int *input){ cout<<"Enter an integer number:"; cin>>*input; }void doubleValue(int *val){ *val *=2;}arrow_forwardIn C programming, if the return type of a function is Void how can you implement assert statements? For example, Void ceasar(int n, char*x); //if char = c and n = 3 output == f how do i use assert statements as this does not work assert (caesar(3,c)==f);arrow_forward1. Write a program in python that contains a main function and a custom, void function named show_larger that takes two random integers as parameters. This function should display which integer is larger and by how much. The difference must be expressed as a positive number if the random integers differ. If the random integers are the same, show_larger should handle that, too. See example outputs. In the main function, generate two random integers both in the range from 1 to 5 inclusive, and call show_larger with the integers as arguments.EXAMPLE OUTPUT 13 is larger than 1 by 2EXAMPLE OUTPUT 2The integers are equal, both are 3arrow_forward
- I need help in creating 5 user defined function in C to solve the following problem as shown in the screenshot. Below is the skeleton code for the problem. The 5 user defined functions need to be created in accordance to the screenshot and should be tested in the int main function. The Skeleton Code #include <stdio.h>#include <string.h> typedef char String30[31];typedef char String60[61]; /* TO DO: Implement the functions as specified in screenshot above RESTRICTIONS: 1. Do NOT call printf() or scanf() in any of the required function definition EXCEPT in the main() function. */ /* Step 1: Define the function that will achieve this step right after this comment. Do NOT call print(), scanf() and any other library functions not indicated above. User defined function has to be made in accordance to step 1 in the screenshot */ /* Step 2: Define the function that will achieve this step right after this comment. Do…arrow_forward#include<stdio.h> //Standard library math.h for Mathematics functions #include<math.h> //function declaration void function1(void); void function2(void); int main(void) { //calling both function function1(); function2(); printf("\nMain function for Ceil value: %f",ceil(4.5)); return0; } // Defining the first function void function1(void) { printf("\nFirst function for Square Root: %f",sqrt(91)); } //Defining the second function void function2(void) { printf("\nSecond function for Power: %f",pow(2,4)); } I need to do what the picture is asking for.arrow_forwardplease typed Let the function fun be defined as: int fun(int* k) { *k += 6; return 4 * (*k); } Suppose fun is used in a program as follows: void main() { int i = 10, j = 20, sum1, sum2; sum1 = (i / 2) + fun(&i); sum2 = fun(&j) + (j / 2); } Question: What are the values of sum1 and sum2 if a) operands in the expressions are evaluated left to right? b) operands in the expressions are evaluated right to left?arrow_forward
- I could really use help with void-user defined, value-returning functions. here's my Instructions for my C++ program: The function printGrade in Example 6-13 is written as a void function to compute and output the course grade. The course score is passed as a parameter to the function printGrade. Rewrite the function printGrade as a value-returning function so that it computes and returns the course grade. (The course grade must be output in the function main.) Also, change the name of the function to calculateGrade.The function printGrade has been posted below for your convenience. void printGrade(int cScore){cout << "The course grade is: "; if (cScore >= 90) {cout << "A." << endl; } else if (cScore >= 80) {cout << "B." << endl;} else if(cScore >= 70) {cout << "C." << endl;} else if (cScore >= 60) {cout << "D." << endl; } else {cout << "F." << endl; } I don't understand how to program a void function. It…arrow_forwardWhen is the body of a function executed? A. When the function is defined B. When the program begins to run C. When the function is called D. Shortly after it is convicted Which selection below best answers the question, "How many parameters can a function have?" A. zero or more B. one or more C. none D. up to five How is the body of a function defined? A. The body of a function must be registered at python.org B. By placing the code between the 'def' and 'end' statements C. By surrounding the code immediately following the 'def' statement with curly braces '{}' D. By indenting the lines of code immediately following the 'def' statementarrow_forwardUse Clojure to complete these questions: Please note that this is the beginning of the course so please use simple methods possible, thanks a. The built-in function type is useful for checking what kind of object an expression evaluates to. Write a function both-same-type? that takes two arguments, and returns true when they both have the same type, and false otherwise. b. Write a function list-longer-than? which takes two arguments: an integer n, and a list lst and returns true if lst has more than n elements, and false otherwise. For example, (list-longer-than? 3 '(1 2 3)) should return false, and (list-longer-than? 2 '(1 2 3)) should return true. c. See attached picture.arrow_forward
- Please send me answer within 10 min!! I will rate you good for sure!! Please provide screenshot of code and output with explaination!! Write a C program that implements and tests a function that simulates strcmp function. You should not use functions from <string.h> library. You may use array and/or pointer notation to define the functions. More precisely, you should implement this function: Hint: int myStrcmp (const char *s1, const char *s2) This function compares the string pointed to by s1 to the string pointed to by s2. If the string pointed to by s1 comes before the string pointed to by s2 in dictionary ordering, then -1 is returned. If the string pointed to by s1 is the same as the string pointed to by s2, then 0 is returned (the compare function is case sensitive). Otherwise, 1 is returned. C programarrow_forwardWrite a function to divide variable ‘a’ with ‘b’. Store the result in a separate variable andreturn it from the function. Practice default parameters by calling your function with 1 argumentand with 2 arguments. Use the concept of function prototyping for this question. The signature offunction is as follows: int divide (int a, int b=2)arrow_forwardQuestion 11Given the code segment below, what should be the data type of a in the function prototype of func(), given the call from main()? Use pointer notation. void func( ______ a); int main() { double aData[15]; func(aData); return 0; }arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
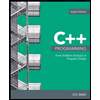