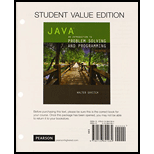
Java: Intro. to Prob. Solv... (Looseleaf)
7th Edition
ISBN: 9780133841084
Author: SAVITCH
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 2PP
Program Plan Intro
Displaying right arrow and left arrow
Program Plan:
Filename: Test.java
- • Include the required header files.
- • Define the class “Test”.
- • Define main function.
- ○ Create an object for scanner.
- ○ Declare the character variable.
- ○ The “do-while” condition is used to get the user response to do the operation once again.
- ■ Create the objects “a1” and “b1” for “LeftArrow” and “RightArrow” classes.
- ■ Call the method “writeOutput” using both the objects.
- ■ Create the objects “a2” and “b2” for “LeftArrow” and “RightArrow” classes.
- ■ Call the method “writeOutput” using both the objects.
- ■ Create the objects “a3” and “b3” for “LeftArrow” and “RightArrow” classes.
- ■ Call the method “writeOutput” using both the objects.
- ■ Change the value of offset from 0 to 1 and call the method “writeOutput” using both the objects.
- ■ Call the method “setOffset” using both the objects.
- ■ Change the value of offset from 0 to 2 and call the method “writeOutput” using both the objects.
- ■ Call the method “setTail” using both the objects.
- ■ Change the value of offset from 3 to 10 and call the method “writeOutput” using both the objects.
- ■ Call the method “setBase” using both the objects.
- ■ Test the write offset values for both arrows by calling “writeOffset” method.
- ■ Test the return offset for both arrows by calling “getOffset” method.
- ■ Test the write tail for both arrows by calling “writeTail” method.
- ■ Test the return tail for both arrows by calling “getTail” method.
- ■ Test the write base for both arrows by calling “writeBase” method.
- ■ Test the return tail for both arrows by calling “getBase” method.
- ■ Reset the values and call “set” method.
- ■ Call the method “writeOutput” using both the objects.
- ■ Call the “drawHere” method using both the objects.
- ■ Reset the values and call “set” method.
- ■ Call the method “writeOutput” using both the objects.
- ■ Call the “drawHere” method using both the objects.
- ■ Reset the values and call “set” method.
- ■ Call the method “writeOutput” using both the objects.
- ■ Call the “drawHere” method using both the objects.
- ■ Finally display the two arrows on the output screen.
Filename: LeftArrow.java
- • Define the class “LeftArrow” extends from the “ShapeBase” class.
- ○ Declare the required variables.
- ○ Define the default constructor.
- ■ Call the “super” method.
- ■ Set the values.
- ○ Define the constructor with the arguments “theTail”, “theBase”.
- ■ Call the method “super” with an offset value.
- ■ Set the value.
- ■ Check the “theBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “theBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “theBase”.
- • Assign “base” is equal to 3.
- ○ Define another constructor with the arguments “theTail”, “theBase”.
- ■ Call the method “super”.
- ■ Set the value.
- ■ Check the “theBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “theBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “theBase”.
- ■ Define another constructor with the parameters “theTail”, “theBase”.
- • Assign “base” is equal to 3.
- ○ Define the “set” method with the arguments “newOffset”, newTail”, and “newBase”.
- ■ Call the method “super” with an offset value.
- ■ Set the value.
- ■ Check the “newBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “newBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “newBase”.
- • Assign “base” is equal to 3.
- ○ Define the “setTail” method with an argument “newTail”.
- ■ Set the value.
- ○ Define the method “setBase” with an argument “newBase”.
- ■ Check the “newBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “newBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “newBase”.
- • Assign “base” is equal to 3.
- ■ Check the “newBase” value is less than 3.
- ○ Define the “writeOutput()” method.
- ■ Call the “writeOffset()”, “writeTail ()”, and “writeBase()” methods.
- ○ Define the “writeOffset()” method.
- ■ Display the offset by calling the “getOffset()” method.
- ○ Define the “writeTail()” method.
- ■ Display the offset by calling the “getTail()” method.
- ○ Define the “writeBase()” method.
- ■ Display the offset by calling the “getBase()” method.
- ○ The method “getTail()” returns the “tail” value.
- ○ The method “getBase()” returns the “base” value.
- ○ Define the method “drawHere ()”.
- ■ Call the “drawTop()”, “drawTail()”, and “drawBottom()” methods.
- ○ Define the “drawTop ()” method.
- ■ Declare the variable “linecount” and assign the value of “getBase()” value divided by 2.
- ■ Declare the variable “numberOfSpaces” and calculate it.
- ■ Call the “skipSpaces()” method with a parameter “numberOfSpaces” value.
- ■ Display the “*” character.
- ■ Declare the “count” variable.
- ■ Declare the “insideWidth” with value 1.
- ■ The “for” condition is used to display the “*” character in left arrow shape.
- • Decrement the “numberOfSpaces” value by 2.
- • Call the “skipSpaces()” method with “numberOfSpaces” value.
- • Display the “*” character.
- • Call the “skipSpaces()” method with “insideWidth” value.
- • Display the “*” character.
- • Increment the “insideWidth” value by 2.
- ○ Define the “drawTial()” method.
- ■ Call the “skipSpaces()” method with “getOffset()” value.
- ■ Display the “*” character.
- ■ Declare and calculate the “insideWidth” value.
- ■ Call the “skipSpaces()” method with “insideWidth” value.
- ■ Declare the “count” variable.
- ■ The “for” condition is used to display the “*” character in left arrow shape.
- • Display the “*” character.
- ○ Define the “drawBottom()” method.
- ■ Declare the required variables and calculate them respectively.
- ■ The “for” condition is used to display the “*” character in left arrow shape.
- • Call the “skipSpaces()” method with “startOfLine” value.
- • Display the “*” character.
- • Call the “skipSpaces()” method with “inideWidth” value.
- • Display the “*” character.
- • Increment the “startOfLine” value by 2.
- • Decrement the “insideWidth” value by 2.
- ○ Define “skipSpaces()” method.
- ■ Display the space.
Filename: RightArrow.java
- • Define the class “RightArrow” extends from the “ShapeBase” class.
- ○ Declare the required variables.
- ○ Define the default constructor.
- ■ Call the “super” method.
- ■ Set the values.
- ○ Define the constructor with the arguments “theTail”, “theBase”.
- ■ Call the method “super” with an offset value.
- ■ Set the value.
- ■ Check the “theBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “theBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “theBase”.
- • Assign “base” is equal to 3.
- ○ Define another constructor with the arguments “theTail”, “theBase”.
- ■ Call the method “super”.
- ■ Set the value.
- ■ Check the “theBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “theBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “theBase”.
- ■ Define another constructor with the parameters “theTail”, “theBase”.
- • Assign “base” is equal to 3.
- ○ Define the “set” method with the arguments “newOffset”, newTail”, and “newBase”.
- ■ Call the method “super” with an offset value.
- ■ Set the value.
- ■ Check the “newBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “newBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “newBase”.
- • Assign “base” is equal to 3.
- ○ Define the “setTail” method with an argument “newTail”.
- ■ Set the value.
- ○ Define the method “setBase” with an argument “newBase”.
- ■ Check the “newBase” value is less than 3.
- • Assign “base” is equal to 3.
- ■ Check the “newBase% 2” is equal to 0.
- • Assign “base” value is equal to “theBase + 1”.
- ■ Otherwise, assign the “base” is equal to “newBase”.
- • Assign “base” is equal to 3.
- ■ Check the “newBase” value is less than 3.
- ○ Define the “writeOutput()” method.
- ■ Call the “writeOffset()”, “writeTail ()”, and “writeBase()” methods.
- ○ Define the “writeOffset()” method.
- ■ Display the offset by calling the “getOffset()” method.
- ○ Define the “writeTail()” method.
- ■ Display the offset by calling the “getTail()” method.
- ○ Define the “writeBase()” method.
- ■ Display the offset by calling the “getBase()” method.
- ○ The method “getTail()” returns the “tail” value.
- ○ The method “getBase()” returns the “base” value.
- ○ Define the method “drawHere ()”.
- ■ Call the “drawTop()”, “drawTail()”, and “drawBottom()” methods.
- ○ Define the “drawTop ()” method.
- ■ Declare the variable “startOfLine” and assign the value of “getOffset()” plus “getTail()”.
- ■ Call the “skipSpaces()” method with a parameter “startOfLine” value.
- ■ Display the “*” character.
- ■ Declare the “linecount” and calculate it.
- ■ Declare the “count” variable.
- ■ Declare the “insideWidth” with value 1.
- ■ The “for” condition is used to display the “*” character in right arrow shape.
- • Call the “skipSpaces()” method with “startOfLine” value.
- • Display the “*” character.
- • Call the “skipSpaces()” method with “insideWidth” value.
- • Display the “*” character.
- • Increment the “insideWidth” value by 2.
- ○ Define the “drawTial()” method.
- ■ Call the “skipSpaces()” method with “getOffset()” value.
- ■ Declare the “count” variable.
- ■ The “for” condition is used to display the “*” character in right arrow shape.
- • Display the “*” character.
- • Calculate the “insideWidth”.
- • Call the “skipSpaces()” method with “insideWidth” value.
- • Display the “*” character.
- ○ Define the “drawBottom()” method.
- ■ Declare the required variables and calculate them respectively.
- ■ The “for” condition is used to display the “*” character in right arrow shape.
- • Call the “skipSpaces()” method with “startOfLine” value.
- • Display the “*” character.
- • Call the “skipSpaces()” method with “inideWidth” value.
- • Display the “*” character.
- • Decrement the “insideWidth” value by 2.
- • Call the “skipSpaces()” method with “startOfLine” value.
- • Display the “*” character.
- ○ Define “skipSpaces()” method.
- ■ Display the space.
Filename: ShapeInterface.java
Define the interface “SpaceInterface”.
- • Declare the “setOffset()” method.
- • Declare the “getOffset()” method.
- • Declare the “drawAt()” method.
- • Declare the “drawHere()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
1) Consider the following class Date, which represents a date using three ints for month, day and year:
class Date
{
private:
int month;
int day;
int year;
public:
Date() { month = day = year = 0; }
Date(int m, int d, int y) { month = m; day = d; year = y; }
bool operator==(Date);
bool operator<(Date);
};
It asks to create an implementation for both overloaded operators. == should return true if the Date objects are equivalent and false if not. < should return true if the Date of the calling object (left object) comes before the parameter Date object (right object) and false if not.
Assume that all objects of class Date are valid, i.e. month is between 1 and 12, inclusive; day contains an appropriate day for the given month, and year can be anything. You don't need to demonstrate calling these operators.
Write Java classes to solve the following problem:
A company has two types of employees. These are called Exempt and NonExempt. Exempt means the employee is not eligible for overtime pay and is paid a fixed amount each pay period. NonExempt means the employee is paid by the hour and receives 1.5 times the hourly rate for hours worked over 40.
Each Employee has an id number which is a string. They also have a name.
Employee should be an abstract class with the member variables described in number 2.
Employee should have an abstract method called findPay, a toString method, and it should implement the Comparable interface. Use alphabetical order of id number to determine less than, greater than, and equal to. The toString method should produce a string like the following: Emp: ID: 4590 Emp Name: Fred Jones
Employee should have an equals method. Define two employees to be equal if they have the same name and the same id number.
Provide accessor and mutators for Employee.
NonExempt should…
Consider the definition of the following class:
public class CC
{
private int u;
private int v;
private double w;
public CC( ) //Line 1
{…}
public CC(int a) //Line 2
{…}
public CC(int a, int b) //Line 3
{…}
public CC(int a, int b, double d) //Line 4
{…}
A. Give the line number containing the constructor that is executed in each of the following declarations:
(i) CC one = new CC( ); _________________
(ii) CC two = new CC(5, 6); _________________
(iii) CC three = new CC(2, 8, 3.5); _________________
B. Write the definition of the constructor in Line 1 so that the instance variables are
initialized to 0.
C.Write the definition of the constructor in Line 4 so that the instance variables u, v, and w are initialized according to the values of the parameters a, b, and d,…
Chapter 8 Solutions
Java: Intro. to Prob. Solv... (Looseleaf)
Ch. 8.1 - Prob. 1STQCh. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Suppose the class SportsCar is a derived class of...Ch. 8.1 - Can a derived class directly access by name a...Ch. 8.1 - Can a derived class directly invoke a private...Ch. 8.1 - Prob. 6STQCh. 8.1 - Suppose s is an object of the class Student. Base...Ch. 8.2 - Give a complete definition of a class called...Ch. 8.2 - Add a constructor to the class Student that sets...Ch. 8.2 - Rewrite the definition of the method writeoutput...
Ch. 8.2 - Rewrite the definition of the method reset for the...Ch. 8.2 - Can an object be referenced by variables of...Ch. 8.2 - What is the type or types of the variable(s) that...Ch. 8.2 - Prob. 14STQCh. 8.2 - Prob. 15STQCh. 8.2 - Consider the code below, which was discussed in...Ch. 8.2 - Prob. 17STQCh. 8.3 - Prob. 18STQCh. 8.3 - Prob. 19STQCh. 8.3 - Is overloading a method name an example of...Ch. 8.3 - In the following code, will the two invocations of...Ch. 8.3 - In the following code, which definition of...Ch. 8.4 - Prob. 23STQCh. 8.4 - Prob. 24STQCh. 8.4 - Prob. 25STQCh. 8.4 - Prob. 26STQCh. 8.4 - Prob. 27STQCh. 8.4 - Prob. 28STQCh. 8.4 - Are the two definitions of the constructors given...Ch. 8.4 - The private method skipSpaces appears in the...Ch. 8.4 - Describe the implementation of the method drawHere...Ch. 8.4 - Is the following valid if ShapeBaSe is defined as...Ch. 8.4 - Prob. 33STQCh. 8.5 - Prob. 34STQCh. 8.5 - What is the difference between what you can do in...Ch. 8.5 - Prob. 36STQCh. 8 - Consider a program that will keep track of the...Ch. 8 - Implement your base class for the hierarchy from...Ch. 8 - Draw a hierarchy for the components you might find...Ch. 8 - Suppose we want to implement a drawing program...Ch. 8 - Create a class Square derived from DrawableShape,...Ch. 8 - Create a class SchoolKid that is the base class...Ch. 8 - Derive a class ExaggeratingKid from SchoolKid, as...Ch. 8 - Create an abstract class PayCalculator that has an...Ch. 8 - Derive a class RegularPay from PayCalculator, as...Ch. 8 - Create an abstract class DiscountPolicy. It should...Ch. 8 - Derive a class BulkDiscount from DiscountPolicy,...Ch. 8 - Derive a class BuyNItemsGetOneFree from...Ch. 8 - Prob. 13ECh. 8 - Prob. 14ECh. 8 - Create an interface MessageEncoder that has a...Ch. 8 - Create a class SubstitutionCipher that implements...Ch. 8 - Create a class ShuffleCipher that implements the...Ch. 8 - Define a class named Employee whose objects are...Ch. 8 - Define a class named Doctor whose objects are...Ch. 8 - Create a base class called Vehicle that has the...Ch. 8 - Create a new class called Dog that is derived from...Ch. 8 - Define a class called Diamond that is derived from...Ch. 8 - Prob. 2PPCh. 8 - Prob. 3PPCh. 8 - Prob. 4PPCh. 8 - Create an interface MessageDecoder that has a...Ch. 8 - For this Programming Project, start with...Ch. 8 - Modify the Student class in Listing 8.2 so that it...Ch. 8 - Prob. 8PPCh. 8 - Prob. 9PPCh. 8 - Prob. 10PP
Knowledge Booster
Similar questions
- Java - Polymorphism Create a class named “Shape” that has the abstract methods getArea() and getPerimeter(). Create classes named “Rectangle”, “Square”, and “Circle”. Override the methods getArea() and getPerimeter() with the right equation in getting the area and perimeter. For rectangle, create attribute length and width. For square, side. For circle, radius. Inputs 1. Char input for Shape type (R/S/C) 2. Dimensions of specific type of Shape C 5 Sample Output Shape(R/S/C): R Length: 10 Width: 50 Area: 500.00 Perimeter: 120.00arrow_forwardWrite in Java Given main() and a base Book class, define a derived class called Encyclopedia. Within the derived Encyclopedia class, define a printInfo() method that overrides the Book class' printInfo() method by printing not only the title, author, publisher, and publication date, but also the edition and number of volumes.arrow_forwardWrite a java program to create a class Employee which contains member variables as name (String object)empid (Integer object)salary (Float object)age (Integer object)Define a default as well as a parameterized constructor to initialize member variables with default and specified values respectively. Define and overload a method search as defined below boolean search (Employee e[ ], String name) To search an employee with name in an array of employee objects, if found return true, else false.boolean search (Employee e[ ], Integer empid) To search an employee with the empid in an array of employee objects, if found return true, else false.Also define another method to get the name of employee having highest salary in a set of employees. String getHighestSalary(Employee e[ ]) To return the name of the employee with highest salary in an array of employee objects.Input: At least 3 employee details like name, empid, salary and age.Name of employee to searchId of employee to searchOutput:…arrow_forward
- Use abstract classes and pure virtual functions to design classes to manipulate various types ofaccounts. For simplicity, assume that the bank offers three types of accounts: savings, checking, andcertificate of deposit, as described next.Savings accounts: Suppose that the bank offers two types of savings accounts: one that has no minimumbalance and a lower interest rate and another that requires a minimum balance and has a higherinterest rate.Checking accounts: Suppose that the bank offers three types of checking accounts: one with a monthlyservice charge, limited check writing, no minimum balance, and no interest; another with no monthlyservice charge, a minimum balance requirement, unlimited check writing and lower interest; and a thirdwith no monthly service charge, a higher minimum requirement, a higher interest rate, and unlimitedcheck writing.Certificate of deposit (CD): In an account of this type, money is left for some time, and these accountsdraw higher interest rates than…arrow_forwardUsing JAVA write a code to: Implement a class Person with a name and a year of birth. Add a class Student that inherits from Person and has a major. Add a class Instructor that inherits from Person and has a salary. For each class, write the needed constructors, getters, setters, and a toString method that stringifies the instance variables in a readable format. Supply a test program that instantiates an instance of each class and exercises each method defined for the class.arrow_forwarduse java (ArrayList, inheritance, polymorphism, File I/O and basic Java) Student class: First, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information, and all of these should be defined as Private: A first name (given name) A last name (family name/surname) Student number (ID) – an integer number (of type long) The Student class will have at least the following constructors and methods: (i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables of Student. (ii) only necessary set and get methods for a valid class design. (iii) a reportGrade method, which you have nothing to report here, you can just print to the screen a message “There is no grade here.”. This method will be overridden in the respective child classes. (iv) an equals method which compares two student objects and returns true if they…arrow_forward
- Write in java an abstract class Student that includes the following hidden attributes: id(int), name(String), major(String) and grade(double), then create setter and getter for each of them. Write two classes that inherited from Student: ItStudent and ArtStudent. The grade for ItStudent is calculated as: grade = mid*0.30 + project*0.30 + final*40 and the grade for ArtStudent is calculated as: grade = mid*0.40 + report*0.10 + final*50. Crate main class that achieve the following:a. Create an array of some Student objects from both ItStudent and ArtStudent classes.b. Sort the Student objects by grade in descending order using functions.c. Save the sorted objects into file.arrow_forwardCreate a program that has the following classes: Point - Just like in the lecture, with attributes x and y set to 0 by default. Circle - Contains two attributes, center and radius. center is of type Point defined above (initialize a Point instance for this), while radius is set to 0 by default. The program must have the function point_in_circle(c, p) where c is a Circle and p is a Point. The function returns True if the point is within the circle, and False otherwise. You may use the math module (and nothing else) if necessary. You may also create other functions that can help you code the main function much easier. Example usage # Initialize a circle with center at (0, 0) and radius 1 (unit circle). c = Circle() c.center.x, c.center.y, c.radius = 0, 0, 1 # Initialize a point located at (1, 1) p = Point() p.x, p.y = 1, 1 # This returns False since (1, 1) is outside the circle point_in_circle(c, p)arrow_forwardConsider the following class implementation, which represents a price in dollars and cents: class Price { private: int dollars; int cents; public: Price() { dollars = cents = 0; } Price(int d, int c) { dollars = d; cents = c; } bool operator!=(Price); bool operator<(Price); }; Write an implementation for both overloaded operators. != should return true if the Prices are not equivalent, and should return false if they are equivalent. < should return true if the Price of the calling object (left object) is less than the parameter Price object (right object). You do not need to demonstrate calling these operators.arrow_forward
- a) Implement a class Point with three attributes, x, y, and z.b) Implement an init method with an optional parameter type: 1) Set the default value of x, y, and z to 0.c) Implement a display method to print the values of x, y, and z as the example output below.d) Instantiate two objects of type Point, one with arguments,1, 2, and 3, and the other one without any arguments.e) Call display() to print x, y, and z. Example Output(x, y, z): (1, 2, 3)(x, y, z) : (0, 0, 0)arrow_forwardImplement the following concepts by constructing suitable classes in Java -a. Constructorsb. Constructor Overloadingc. Function Overloadingd. Function Overridinge. InheritanceCreate a class Person - Field - Name, Birthdate, Contact no and Mail idMethods - Constructor, accept and displayClass Employee - Inherits the Person classFields : Emp ID, grade, basic salary, Gross Salary, HRA (House Rent Allowance), TA (TravelingAllowance), DA (Dearness Allowance)Given:Grade Basic TAA 8000 2000B 7000 1500C 6000 1000D 5000 500Calculate Gross Salary :Gross Salary = Basic + HRA (20% of Basic) + DA (50% Basic) + TAe.g if Basic = 8000TA = 2000HRA = 20% of 8000 = 1600DA = 50 % of 8000 = 4000Gross = 8000 + 2000 + 1600 + 4000 = 15600Optional : you can implement increment function in which you can change grade of an employee andrecalculate salary with new gradearrow_forwardCan you implement the Derived Class Parameterized constructor? A solution is placed in the "solution" section to help you, but we would suggest you try to solve it on your own first. Implement the constructor Dell(String name) of the Derived Class Dell which takes a string, name. We have already implemented the Base Class Laptop with the member function getName() and a parameterized constructor. Input# Laptop name is being passed through the parameterized constructor. Output# getName() method is returing Laptop name. Sample Input# Dell dell = new Dell("Dell Inspiron"); Sample Output# "Dell Inspiron"arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
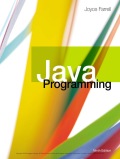
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT