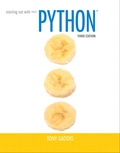
Starting Out with Python (3rd Edition)
3rd Edition
ISBN: 9780133743692
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 8, Problem 9PE
Program Plan Intro
Vowels and Consonants
Program Plan:
- • Declare a function named “vowelsCount()” that accepts a string “my_string” as input. Inside the function,
- ○ Create a counter variable “cnt1” to store the number of vowels in the string.
- ○ Use the “for” loop to process all the characters in the “my_string” to check for vowels.
- ○ Inside the “for” loop,
- ■ Check if the current character “ch” is the alphabet.
- ■ If so, then check if the current character “ch” is the vowel.
- ■ If condition is true, then increment the “cnt1” value by “1”.
- ○ After processing all the characters in the “my_string”, return the counter “cnt1” value.
- • Declare a function named “consonantsCount()” that accepts a string “my_string” as input. Inside the function,
- ○ Create a counter variable “cnt2” to store the number of consonants in the “my_string”.
- ○ Use the “for” loop to process all the characters in the “my_string” to check for consonants.
- ○ Inside the “for” loop,
- ■ Check if the current character “ch” is the alphabet.
- ■ If so, then check if the current character “ch” is not a vowel.
- ■ If the condition is true, then increment the “cnt2” value by “1”.
- ○ After processing all the characters in the “my_string”, return the counter “cnt2” value.
- • Declare a function named “main()”. In the “main()” function,
- ○ Get a string from the user and store it in the “my_string” variable.
- ○ Call the function “vowelsCount()” to count the vowels and pass “my_string” as input to the function and store the return count in “count1”.
- ○ Call the function “consonantsCount()” to count the consonants and pass “my_string” as input to the function and store the return count in “count2”.
- ○ Display “count1” and “count2” using the “print()” function.
- • In the last, call the function “main()” to execute the program.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Exercise 1:
Word Separator
Write a program that accepts as input a sentence in which all of the words are run together, but the first character of each word is uppercase. Convert the sentence to a string in which the words are separated by spaces and only the first word starts with an uppercase letter. For example the string StopAndSmellTheRoses. would be converted to “Stop and smell the roses.”
Exercise 2:
replaceSubstring Function
Write a function named replaceSubstring. The function should accept three string object arguments. Let’s call them string1, string2, and string3. It should search string1 for all occurrences of string2. When it finds an occurrence of string2, it should replace it with string3. For example, suppose the three arguments have the following values:
string1: “the dog jumped over the fence”
string2: “the”
string3: “that”
With these three arguments, the function would return a string object with the value
“that dog jumped over that fence.” Demonstrate the…
String Manipulation
In this question, you will be implementing the following functions
int findChar(char * str, char c);
Searches for the character c in the string str and returns the index of the character in the string. If the character does not exist, returns -1
int replaceChar(char * str, char c1, char c2);
Searches for the character c1 in the string str and if found, replace it with c2.The function returns the number of replacements it has performed. If the character does not exist, returns 0.
int removeChar(char * str1, char * str2, char c);
Creates a copy of str1 into str2 except for the character c that should be replaced with ‘*’
For example, if
str1=”Hello World” and
c=’l’ then the function should make
str2=”He**o Wor*d”
int isPalindrome(char * str)
Checks to see if a string is Palindrome(reversible). If it is, returns 1, otherwise returns 0. A palindrome string reads similarly from left to right and from right to left like madam, level, radar, etc.
int reverseString(char…
10.17: Morse Code Converter C++Morse code is a code where each letter of the English alphabet, each digit, and various punctuation characters are represented by a series of dots and dashes. Table 10-8 from the textbook shows part of the code.
Write a program that asks the user to enter a string, and then converts that string to Morse code. Note that Morse code represents both upper and lower case letters so that both 'A' and 'a' will be converted to ".-".
Input Validation.None.
Instructor Notes:
Note: You can use the Morse table in the book or this site (space isn't listed, but it is just mapped to a space). You will most certainly need to create two parallel arrays to map the characters to the Morse code strings.
Chapter 8 Solutions
Starting Out with Python (3rd Edition)
Ch. 8.1 - Assume the variable name references a string....Ch. 8.1 - What is the index of the first character in a...Ch. 8.1 - If a string has 10 characters, what is the index...Ch. 8.1 - Prob. 4CPCh. 8.1 - Prob. 5CPCh. 8.1 - Prob. 6CPCh. 8.2 - Prob. 7CPCh. 8.2 - Prob. 8CPCh. 8.2 - Prob. 9CPCh. 8.2 - What will the following code display? mystring =...
Ch. 8.3 - Prob. 11CPCh. 8.3 - Prob. 12CPCh. 8.3 - Write an if statement that displays Digit" if the...Ch. 8.3 - What is the output of the following code? ch = 'a'...Ch. 8.3 - Write a loop that asks the user Do you want to...Ch. 8.3 - Prob. 16CPCh. 8.3 - Write a loop that counts the number of uppercase...Ch. 8.3 - Assume the following statement appears in a...Ch. 8.3 - Assume the following statement appears in a...Ch. 8 - This is the first index in a string. a. 1 b. 1 c....Ch. 8 - This is the last index in a string. a. 1 b. 99 c....Ch. 8 - This will happen if you try to use an index that...Ch. 8 - This function returns the length of a string. a....Ch. 8 - This string method returns a copy of the string...Ch. 8 - This string method returns the lowest index in the...Ch. 8 - This operator determines whether one string is...Ch. 8 - This string method returns true if a string...Ch. 8 - This string method returns true if a string...Ch. 8 - This string method returns a copy of the string...Ch. 8 - Once a string is created, it cannot be changed.Ch. 8 - You can use the for loop to iterate over the...Ch. 8 - The isupper method converts a string to all...Ch. 8 - The repetition operator () works with strings as...Ch. 8 - Prob. 5TFCh. 8 - What does the following code display? mystr =...Ch. 8 - What does the following code display? mystr =...Ch. 8 - What will the following code display? mystring =...Ch. 8 - Prob. 4SACh. 8 - What does the following code display? name = 'joe'...Ch. 8 - Assume choice references a string. The following...Ch. 8 - Write a loop that counts the number of space...Ch. 8 - Write a loop that counts the number of digits that...Ch. 8 - Write a loop that counts the number of lowercase...Ch. 8 - Write a function that accepts a string as an...Ch. 8 - Prob. 6AWCh. 8 - Write a function that accepts a string as an...Ch. 8 - Assume mystrinc references a string. Write a...Ch. 8 - Assume mystring references a string. Write a...Ch. 8 - Look at the following statement: mystring =...Ch. 8 - Initials Write a program that gets a string...Ch. 8 - Sum of Digits in a String Write a program that...Ch. 8 - Date Printer Write a program that reads a string...Ch. 8 - Prob. 4PECh. 8 - Alphabetic Telephone Number Translator Many...Ch. 8 - Average Number of Words If you have downloaded the...Ch. 8 - If you have downloaded the source code you will...Ch. 8 - Sentence Capitalizer Write a program with a...Ch. 8 - Prob. 9PECh. 8 - Prob. 10PECh. 8 - Word Separator Write a program that accepts as...Ch. 8 - Pig Latin Write a program that accepts a sentence...
Knowledge Booster
Similar questions
- IN C Programming Language...THANKS Write a function that replaces a given string with ‘*’ within a given text if that string is a full word, or the beginning or end of a word. Test your function with a suitable main.arrow_forward(Count the letters in a string) Write a function that counts the number of letters in a string using the following header: def countLetters(s) : Write a test program that prompts the user to enter a string and displays the number of letters in the string. the answer should be in python.arrow_forward// HouseSign.cpp - This program calculates prices for custom made signs. #include <iostream> #include <string> using namespace std; int main() { // This is the work done in the housekeeping() function // Declare and initialize variables here // Charge for this sign // Color of characters in sign // Number of characters in sign // Type of wood // This is the work done in the detailLoop() function // Write assignment and if statements here // This is the work done in the endOfJob() function // Output charge for this sign cout << "The charge for this sign is $" << charge << endl; return(0); }arrow_forward
- 4. Word Separator:Write a program that accepts as input a sentence in which all of thewords are run together but the first character of each word is uppercase. Convert the sentence to a string in which the words are separated by spaces and only the first word starts with an uppercase letter. For example the string “StopAndSmellTheRoses.” would be converted to “Stop and smell the roses. *python codingarrow_forwardthe function returns the result as a string if the expression is legal. the expression returns an empty string and then put the function public class Ez1MathEval {public String heavywtEval (String exprn) {return ""}}arrow_forward// Airline.cpp - This program determines if an airline passenger is // eligible for a 25% discount. // Harvey Mark, ITSE 1329#include <iostream> #include <string> using namespace std; int main() { string passengerFirstName = ""; // Passenger's first name string passengerLastName = ""; // Passenger's last name int passengerAge = 0; // Passenger's age // This is the work done in the housekeeping() function cout << "Enter passenger's first name: "; cin >> passengerFirstName; cout << "Enter passenger's last name: "; cin >> passengerLastName; cout << "Enter passenger's age: "; cin >> passengerAge; // This is the work done in the detailLoop() function // Test to see if this customer is eligible for a 25% discount if(passengerAge <= 6) { cout << "You are eligable for a discount!\n"; } if(passengerAge >= 65) { cout << "You are eligable for a…arrow_forward
- Function Name: compliments Parameters: answer1 - a boolean (True or False) representing whether the user is "smart" answer2 - a boolean (True or False) representing whether the user is "awesome" answer3 - a boolean (True or False) representing whether the user is "fun" Description: Write a function that outputs a string of compliments based on the adjectives selected by the inputs. Use the inputs True and False. The function should return the string “You are” concatenated with the compliments that are true. The three compliments should be: "smart" "awesome" and "fun". If none of the compliments are true, print the string “Goodbye.” instead. Test Cases: >>>compliments(True, True, True) You are smart awesome fun. >>>compliments(False, True, False) You are awesome. >>>compliments(False, False, False) Goodbye.arrow_forwardC program Write 2 functions that can convert an input string to all lowercase and to all uppercase. Write a program to illustrate the use of these 2 functions. Test Data: Input the string: ComputerEngineering Output: Lowercase: computerengineering Uppercase: COMPUTERENGINEERINGarrow_forward(String Matching): Write a program to use Horspool’s Algorithm to find the pattern in the string. You can define two variables called Text and Pattern. Please display shift table for that pattern and display the shift value for each step. If not match, display a message “Unsuccessful Search”. If match, display the index. For example, If Text =“BARD LOVED BANANAS” and Pattern=”BAOBAB”. The result will be: Shift Table: A=1, B=2, O=3, other=6 Shift 6, shift 2, shift 6, pattern not found If Text=”BARD LOVED BABAOBABANAS” and Pattern=”BAOBAB”. The result will be: Shift Table: A=1, B=2, O=3, other=6 Shift 6, shift 2, shift 2, shift 3, pattern found at position 13 Please let me know the solution in Java which gives exact mentioned outputs in questionarrow_forward
- (String Matching): Write a program to use Horspool’s Algorithm to find the pattern in the string. You can define two variables called Text and Pattern. Please display shift table for that pattern and display the shift value for each step. If not match, display a message “Unsuccessful Search”. If match, display the index. For example, If Text =“BARD LOVED BANANAS” and Pattern=”BAOBAB”. The result will be: Shift Table: A=1, B=2, O=3, other=6 Shift 6, shift 2, shift 6, pattern not found If Text=”BARD LOVED BABAOBABANAS” and Pattern=”BAOBAB”. The result will be: Shift Table: A=1, B=2, O=3, other=6 Shift 6, shift 2, shift 2, shift 3, pattern found at position 13 Please let me know the solution which gives exact mentioned outputs in questionarrow_forwardWord Separator Write a program that accepts as input a sentence in which all of the words are run together but the first character of each word is uppercase. Convert the sentence to a string in which the words are separated by spaces and only the first word starts with an uppercase letter. For example the string “StopAndSmellTheRoses.” would be converted to “Stop and smell the roses.” LINUX !#/bin/basharrow_forwardCoin Toss Write a function named coinToss that simulates the tossing of a coin. When you call the function, it should generate a random number in the range of 1 through 2. If the random number is 1, the function should display "heads". If the random number is 2, the function should display "tails". Demonstrate the function in a program that sks the user how many times the coin should be tossed and the coin then simulates the tossing of the coin that number of times.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
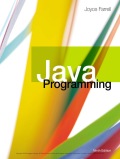
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT