two_layer_net
.py
keyboard_arrow_up
School
University of Michigan *
*We aren’t endorsed by this school
Course
599
Subject
Computer Science
Date
Apr 3, 2024
Type
py
Pages
8
Uploaded by abigailrafter
"""
Implements a two-layer Neural Network classifier in PyTorch.
WARNING: you SHOULD NOT use ".to()" or ".cuda()" in each implementation block.
"""
import torch
import random
import statistics
from rob599.p2_helpers import sample_batch
from typing import Dict, List, Callable, Optional
def hello_two_layer_net():
"""
This is a sample function that we will try to import and run to ensure that
our environment is correctly set up on Google Colab.
"""
print("Hello from two_layer_net.py!")
# Template class modules that we will use later: Do not edit/modify this class
class TwoLayerNet(object):
def __init__(
self,
input_size: int,
hidden_size: int,
output_size: int,
dtype: torch.dtype = torch.float32,
device: str = "cuda",
std: float = 1e-4,
):
"""
Initialize the model. Weights are initialized to small random values and
biases are initialized to zero. Weights and biases are stored in the
variable self.params, which is a dictionary with the following keys:
W1: First layer weights; has shape (D, H)
b1: First layer biases; has shape (H,)
W2: Second layer weights; has shape (H, C)
b2: Second layer biases; has shape (C,)
Inputs:
- input_size: The dimension D of the input data.
- hidden_size: The number of neurons H in the hidden layer.
- output_size: The number of classes C.
- dtype: Optional, data type of each initial weight params
- device: Optional, whether the weight params is on GPU or CPU
- std: Optional, initial weight scaler.
"""
# reset seed before start
random.seed(0)
torch.manual_seed(0)
self.params = {}
self.params["W1"] = std * torch.randn(
input_size, hidden_size, dtype=dtype, device=device
)
self.params["b1"] = torch.zeros(hidden_size, dtype=dtype, device=device)
self.params["W2"] = std * torch.randn(
hidden_size, output_size, dtype=dtype, device=device
)
self.params["b2"] = torch.zeros(output_size, dtype=dtype, device=device)
def loss(
self,
X: torch.Tensor,
y: Optional[torch.Tensor] = None,
reg: float = 0.0,
):
return nn_forward_backward(self.params, X, y, reg)
def train(
self,
X: torch.Tensor,
y: torch.Tensor,
X_val: torch.Tensor,
y_val: torch.Tensor,
learning_rate: float = 1e-3,
learning_rate_decay: float = 0.95,
reg: float = 5e-6,
num_iters: int = 100,
batch_size: int = 200,
verbose: bool = False,
):
# fmt: off
return nn_train(
self.params, nn_forward_backward, nn_predict, X, y,
X_val, y_val, learning_rate, learning_rate_decay,
reg, num_iters, batch_size, verbose,
)
# fmt: on
def predict(self, X: torch.Tensor):
return nn_predict(self.params, nn_forward_backward, X)
def save(self, path: str):
torch.save(self.params, path)
print("Saved in {}".format(path))
def load(self, path: str):
checkpoint = torch.load(path, map_location="cpu")
self.params = checkpoint
if len(self.params) != 4:
raise Exception("Failed to load your checkpoint")
for param in ["W1", "b1", "W2", "b2"]:
if param not in self.params:
raise Exception("Failed to load your checkpoint")
# print("load checkpoint file: {}".format(path))
def nn_forward_pass(params: Dict[str, torch.Tensor], X: torch.Tensor):
"""
The first stage of our neural network implementation: Run the forward pass
of the network to compute the hidden layer features and classification
scores. The network architecture should be:
FC layer -> ReLU (hidden) -> FC layer (scores)
As a practice, we will NOT allow to use torch.relu and torch.nn ops
just for this time (you can use it from A3).
Inputs:
- params: a dictionary of PyTorch Tensor that store the weights of a model.
It should have following keys with shape
W1: First layer weights; has shape (D, H)
b1: First layer biases; has shape (H,)
W2: Second layer weights; has shape (H, C)
b2: Second layer biases; has shape (C,)
- X: Input data of shape (N, D). Each X[i] is a training sample.
Returns a tuple of:
- scores: Tensor of shape (N, C) giving the classification scores for X
- hidden: Tensor of shape (N, H) giving the hidden layer representation
for each input value (after the ReLU).
"""
# Unpack variables from the params dictionary
W1, b1 = params["W1"], params["b1"]
W2, b2 = params["W2"], params["b2"]
N, D = X.shape
# Compute the forward pass
hidden = None
scores = None
############################################################################
# TODO: Perform the forward pass, computing the class scores for the input.#
# Store the result in the scores variable, which should be an tensor of #
# shape (N, C). #
############################################################################
# Replace "pass" statement with your code
pass
###########################################################################
# END OF YOUR CODE #
###########################################################################
return scores, hidden
def nn_forward_backward(
params: Dict[str, torch.Tensor],
X: torch.Tensor,
y: Optional[torch.Tensor] = None,
reg: float = 0.0
):
"""
Compute the loss and gradients for a two layer fully connected neural
network. When you implement loss and gradient, please don't forget to
scale the losses/gradients by the batch size.
Inputs: First two parameters (params, X) are same as nn_forward_pass
- params: a dictionary of PyTorch Tensor that store the weights of a model.
It should have following keys with shape
W1: First layer weights; has shape (D, H)
b1: First layer biases; has shape (H,)
W2: Second layer weights; has shape (H, C)
b2: Second layer biases; has shape (C,)
- X: Input data of shape (N, D). Each X[i] is a training sample.
- y: Vector of training labels. y[i] is the label for X[i], and each y[i] is
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
in python please
arrow_forward
Develop a class ResizingArrayQueueOfStrings that implements the queueabstraction with a fixed-size array, and then extend your implementation to use arrayresizing to remove the size restriction.Develop a class ResizingArrayQueueOfStrings that implements the queueabstraction with a fixed-size array, and then extend your implementation to use arrayresizing to remove the size restriction.
arrow_forward
from typing import List, Tuple, Dict
from poetry_constants import (POEM_LINE, POEM, PHONEMES, PRONUNCIATION_DICT, POETRY_FORM_DESCRIPTION)
# ===================== Provided Helper Functions =====================
def transform_string(s: str) -> str: """Return a new string based on s in which all letters have been converted to uppercase and punctuation characters have been stripped from both ends. Inner punctuation is left untouched.
>>> transform_string('Birthday!!!') 'BIRTHDAY' >>> transform_string('"Quoted?"') 'QUOTED' >>> transform_string('To be? Or not to be?') 'TO BE? OR NOT TO BE' """
punctuation = """!"'`@$%^&_-+={}|\\/,;:.-?)([]<>*#\n\t\r""" result = s.upper().strip(punctuation) return result
def is_vowel_phoneme(phoneme: str) -> bool: """Return True if and only if phoneme is a vowel phoneme. That is, whether phoneme ends in a 0, 1, or 2.
Precondition:…
arrow_forward
The function interleave_lists in python takes two parameters, L1 and L2, both lists. Notice that the lists may have different lengths.
The function accumulates a new list by appending alternating items from L1 and L2 until one list has been exhausted. The remaining items from the other list are then appended to the end of the new list, and the new list is returned.
For example, if L1 = ["hop", "skip", "jump", "rest"] and L2 = ["up", "down"], then the function would return the list: ["hop", "up", "skip", "down", "jump", "rest"].
HINT: Python has a built-in function min() which is helpful here.
Initialize accumulator variable newlist to be an empty list
Set min_length = min(len(L1), len(L2)), the smaller of the two list lengths
Use a for loop to iterate k over range(min_length) to do the first part of this function's work. On each iteration, append to newlist the item from index k in L1, and then append the item from index k in L2 (two appends on each iteration).
AFTER the loop…
arrow_forward
//import java.util.List;
import javax.swing.*;
import javax.swing.event.*;
import java.awt.*;
/** * JList basic tutorial and example * * @author wwww.codejava.net */
public class JListTask1_Student_Version extends JFrame {
//define a Jlist Component
// Define a String array that use to create the list
private JPanel ContryPanel;
// To hold components private JPanel selectedcontryPanel; // To hold components
// define a TextFiles to show the result
private JLabel label; // A message
/** Constructor */
public JListTask1_Student_Version(){
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("JList Task1");
setSize(200, 200);
setLayout(new BorderLayout());
// Build the month and selectedMonth panels. // Add the panels to the content pane. //
add(new JScrollPane(countryList)); setLocationRelativeTo(null);
setVisible(true); }
public void buildcontryPanel() {
// Create a panel to hold the list.
ContryPanel = new JPanel();
//create the list // Set the selection mode to single…
arrow_forward
Need help making a java file that combines both linearSearch and binarySearch
•Both search methods must use the Comparable<T> interface and the compareTo() method.•Your program must be able to handle different data types, i.e., use generics.•For binarySearch, if you decide to use a midpoint computation formula that is different fromthe textbook, explain that formula briefly as a comment within your code.
//code from textbook
//linearSearch
public static <T> boolean linearSearch(T[] data, int min, int max, T target) { int index = min; boolean found = false; while (!found && index <= max) { found = data [index].equals(target); index++; } return found; }
//binarySearch
public static <T extends Comparable<T>> boolean binarySearch(T[] data, int min, int max, T target) { boolean found = false; int midpoint = (min + max)/2; if (data[midpoint].compareTo(target)==0)…
arrow_forward
Make sure the program run smooth and perfectly, make sure no error encounter. compatible for python software.
Complete the sample code template to implement a student list as a binary search tree (BST).Specification1. Each Studentrecord should have the following fields: =studentNumber =lastname =firstName =course =gpa2. Run the program from an appropriate menu allowing for the following operations:* Adding a Student record
* Deleting a Student record
* Listing students by: • All students • A given course • GPA above a certain value • GPA below a certain value
class Record:def __init__(self, studentNumber = None, lastname = None, firstName = None, course = None, gpa =None,):self.studentNumber = studentNumberself.lastname = lastnameself.firstName = firstNameself.course = courseself.gpa = gpadef __str__(self):record = "\n\nStudent Number : {}\n".format(self.studentNumber)record += "Lastname : {}\n".format(self.lastname)record += "FirstName : {}\n".format(self.firstName)record…
arrow_forward
python:
def typehelper(poke_name): """ Question 5 - API
Now that you've acquired a new helper, you want to take care of them! Use the provided API to find the type(s) of the Pokemon whose name is given. Then, for each type of the Pokemon, map the name of the type to a list of all types that do double damage to that type. Note: Each type should be considered individually.
Base URL: https://pokeapi.co/
Endpoint: api/v2/pokemon/{poke_name}
You will also need to use a link provided in the API response.
Args: Pokemon name (str)
Returns: Dictionary of types
Hint: You will have to run requests.get() multiple times!
>>> typehelper("bulbasaur") {'grass': ['flying', 'poison', 'bug', 'fire', 'ice'], 'poison': ['ground', 'psychic']}
>>> typehelper("corviknight") {'flying': ['rock', 'electric', 'ice'], 'steel': ['fighting', 'ground', 'fire']}
"""
# pprint(typehelper("bulbasaur"))#…
arrow_forward
Implement a function findMixedCase in Python that:
accepts a single argument, a list of words, and
returns the index of the first mixed case word in the list, if one exists, (a word is mixed case if it contains both upper and lower case letters), and
returns -1 if the all words are either upper or lower case.
sample:
>> findMixedCase( ['Hello','how','are','you'] ) # 'Hello' is mixed case0
arrow_forward
Definitely upvote you ... but please answer within 30 minutes...and typed answer....no plagiarism... please help me ??
arrow_forward
Computer Science
Write a program in the Java language that includes: 1. A class for books, taking into account the encapsulation and getter, setter. 2. Entering books by the user using Scanner 3. LinkedList class for: (Adding a new book - Inserting a book- Searching for a book using the ISBN Number - Viewing all books,delete book). 4. Serial number increases automatically with each book that is entered. 5. Printing the names of books is as follows: Serial - ISBN - Name - PubYear - Price - Notes. 6-Design a main menu that includes: • Add Book • Insert Book • Delete Book • Search . Display . Exit
arrow_forward
Design and implement a service that simulates PHP loops. Each of the three loop variants should be encapsulated in an object. The service can be controlled via a parameter and execute three different simulations. The result is returned as JSON.
The input is an array consisting of the letters $characters = [A-Z].
-The For loop should store all even letters in an array.-The Foreach loop simulation is to create a backward sorted array by For loop, i.e. [Z-A] .-The While loop should write all characters into an array until the desired character is found.
Interface:-GET Parameter String: loopType ( possible values: REVERSE, EVEN, UNTIL )-GET parameter String: until (up to which character)
Output:JSON Object: {loopName: <string>, result: <array> }
arrow_forward
storage of strings through pointer saves memory space. justify your answer with an example.
arrow_forward
Help with c++... please paste indented code plzz and keep output same as given
arrow_forward
Implement given operations in java :
* Removes the first instance of the specified element from this * list and returns it. Throws an EmptyCollectionException * if the list is empty. Throws a ElementNotFoundException if the * specified element is not found in the list. * param targetElement the element to be removed from the list * return a reference to the removed element * throws EmptyCollectionException if the list is empty * throws ElementNotFoundException if the target element is not found
arrow_forward
Which best describes this axiom:
( aList.insert ( i, item ) ) . isEmpty ( ) = false
None of these
Adding an item to a list will always result in a list that is empty
Adding an item to a list will always result in a list that is not empty
Adding an item to an existing list will always result in false
arrow_forward
Write a Python code using the given function and conditions. Do not use Numpy. Use LinkedList Manipulation.
Given function: def insert(self, newElement, index)
Pre-condition: The list is not empty.
Post-condition: This method inserts newElement at the given index of the list. If an element with the same key as newElement value already exists in the list, then it concludes the key already exists and does not insert the key. [You must also check the validity of the index].
arrow_forward
Write a Python Code for the given function and conditions:
Given function: def insert(self, newElement)
Pre-condition: None.
Post-condition: This method inserts newElement at the tail of the list. If an element with the same key as newElement already exists in the list, then it concludes the key already exists and does not insert the key.
arrow_forward
Please do this in JAVA PROGRAMMING
Note: The attached images are the instructions and the target output
relatives.dat file in text:
14
Jim Sally
Fred Alice
Jim Tom
Jim Tammy
Bob John
Dot Fred
Dot Tom
Dot Chuck
Bob Tom
Fred James
Timmy Amanda
Almas Brian
Elton Linh
Dot Jason
Dot
Relatives.java reference file:
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.TreeSet;
import java.util.Scanner;
import static java.lang.System.*;
public class Relatives
{
private Map<String,Set<String>> map;
public Relatives()
{
}
public void setPersonRelative(String line)
{
String[] personRelative = line.split(" ");
}
public String getRelatives(String person)
{
return "";
}
public String toString()
{
String output="";…
arrow_forward
In Python
arrow_forward
2) Suppose you have a linked list class that provides the following
methods:
// constructor.
// returns size of the list.
LinkList ()
int size ();
void insertHead (Object data) // insert new data at the head of
// the list.
// remove and return the Object at
// the head of the list.
Object removeHead ()
void insertTail (Object data) // insert new data at the tail of
// the list.
The class below is an implementation of a stack using the linked
list class. Fill in the method implementations:
class Stack
{
private Stack s;
public Stack ()
{
public void push (Object x)
{
}
public Object pop () // assume stack is not empty
{
}
public boolean isEmpty()
{
}
arrow_forward
Implement a list of employees using a dynamic array of strings.Your solution should include functions for printing list, inserting and removingemployees, and sorting lists (use Bubble sort in C++ language).Keep list of employees sorted within the list after insertion.See the following example:How many employees do you expect to have?5 (Carriage Return)Menu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exit2 (Carriage Return)Input name of new employee : MikeMenu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exit1(Carriage Return)1 . MaryMenu :1 . Print full list of employees2 . Insert new employee3 . Remove employee from list0 . Exit
arrow_forward
Problem Y:
Implement the reader writer problem using pthreads and semaphores. You
should allow multiple readers to read at the same time and only one single
writer to write to the data set. You are required to use the following:
1. A semaphore rw_muter initialized to 1.
2. A semaphore muter initialized to 1.
3. An integer reader_count initialized to 0.
arrow_forward
Write a Program in C Language.
arrow_forward
make this code an object oriented programming
from playsound import playsoundfrom tkinter import *
root = Tk()topframe=Frame(root)topframe.pack(side=TOP)
def play():playsound("Tiger Roar Sound Effect.mp3")
redbtn = Button(topframe,text="Lion",fg="Red", command=play)redbtn.pack(pady=20)
def play():playsound("Horse Sounds for children.mp3")
brownbtn=Button(topframe,text="Horse",fg="brown", command=play)brownbtn.pack(pady=20)
def play():playsound("Angry Cat - Ringtone.mp3")greybtn=Button(topframe,text="Cat",fg="Grey", command=play)greybtn.pack(pady=20)
root.mainloop()
arrow_forward
You are tasked to implement an abstract data type class which takes in a given input file called input.txt,
and processes, identifies and sorts all unique word instances found in the file.
Sample Input
Hi hello hi good bye goodbye Bye bye good say
Sample Output
Bye Hi bye hello hi say
The input.txt file is found along with the zip folder where this instructions file also came along with.
Note: you are not allowed to use pythonic lists, and its methods and functions, and string methods.
However the use of the file handling methods (read readlines and readline) are allowed for this project.
arrow_forward
Java Program ASAP
Please modify Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); in the program so it reads the two text files and passes the test cases. Down below is a working code. Also dont add any import libraries in the program just modify the rest of the code so it passes the test cases.
import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.HashMap;import java.util.Map;import java.util.Scanner;public class MorseCodeConverter { public static void main(String[] args) { Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt"); Scanner scanner = new Scanner(System.in); System.out.print("Please enter the file name or type QUIT to exit:\n"); do { String fileName = scanner.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { String text =…
arrow_forward
Answer the given question with a proper explanation and step-by-step solution.
Write in Java:
Write a menu-driven program to illustrate the use of a linked list. The entries will only consist of integer number keys (values). The program should implement the following options in order:
• Insert- insert a key into a list- not allowing duplicate integers.
• Delete- delete a key from the list.
• Search- finds or does not find a particular key in the list.
• Print- prints the list graphically in horizontal form. If the list is empty output- "Nothing to print".
• Size- count of all the keys in the list.
• Sort- sorts the keys in the list in ascending order.
• Reverse- reverses the order of the keys in the list
• Rotate- moves the key at the front of the list to the end of the list. If the list has 0 or 1 elements it should have no effect on the list.
• Shift- rearranges the keys of a list by moving to the end of the list all values that are in odd number positions (indexes) and otherwise…
arrow_forward
PYTHON!!!
Implement a function insert_sorted, which will insert a string into its appropriate location in a list of sorted strings.
This function is called with two parameters:
- A list containing words (each of type str) appearing in an ascending lexicographical (alphabetical) order.
- A word (of type str) that is to be inserted into the list provided by the first parameter.
When the function is called, it should add the second parameter's value into its sorted place in first parameter (the list). That is, it mutates the list, so that after the execution, it would also include the newly added word (the second parameter), and remain sorted.
arrow_forward
main.py
Load default template.
1 def all_permutations (permList, namelist):
2
# TODO: Implement method to create and output all permutations of the List of names.
3
4 if
name
== "
main ":
namelist = input ().split('')
permlist = []
all_permutations(permList, namelist)
5
6
7
arrow_forward
void doo(list &L){
int item,a,p=L.size()-1; L.retrieve(p,a);
for(int i=0;i
arrow_forward
Do not add import statements
arrow_forward
def hike(lists: list[list[int]], start: tuple[int, int],end: tuple[int, int]) -> int:"""Given an elevation map <lists> and a start and end calculate the cost itwould take to hike from <start> to <end>. If the start and end are the same, then return 0.Some definitions and rules:1. You can only hike to either a vertically or horizontally adjacentlocation (you cannot travel diagonally).2. You must only travel in the direction of the <end_point>. Moreexplicitly, this means that any move you make MUST take you closerto the end point, so you cannot travel in the other direction.3. We define the cost to travel between two adjacent blocks as theabsolute difference in elevation between those blocks.4. You will calculate the naive route here, so at every position, youwill have two choices, to either move closer to the end point alonga row, or along a column, and you will chose the position that coststhe least at that point (you do not need to look ahead to see if…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
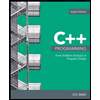
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Related Questions
- in python pleasearrow_forwardDevelop a class ResizingArrayQueueOfStrings that implements the queueabstraction with a fixed-size array, and then extend your implementation to use arrayresizing to remove the size restriction.Develop a class ResizingArrayQueueOfStrings that implements the queueabstraction with a fixed-size array, and then extend your implementation to use arrayresizing to remove the size restriction.arrow_forwardfrom typing import List, Tuple, Dict from poetry_constants import (POEM_LINE, POEM, PHONEMES, PRONUNCIATION_DICT, POETRY_FORM_DESCRIPTION) # ===================== Provided Helper Functions ===================== def transform_string(s: str) -> str: """Return a new string based on s in which all letters have been converted to uppercase and punctuation characters have been stripped from both ends. Inner punctuation is left untouched. >>> transform_string('Birthday!!!') 'BIRTHDAY' >>> transform_string('"Quoted?"') 'QUOTED' >>> transform_string('To be? Or not to be?') 'TO BE? OR NOT TO BE' """ punctuation = """!"'`@$%^&_-+={}|\\/,;:.-?)([]<>*#\n\t\r""" result = s.upper().strip(punctuation) return result def is_vowel_phoneme(phoneme: str) -> bool: """Return True if and only if phoneme is a vowel phoneme. That is, whether phoneme ends in a 0, 1, or 2. Precondition:…arrow_forward
- The function interleave_lists in python takes two parameters, L1 and L2, both lists. Notice that the lists may have different lengths. The function accumulates a new list by appending alternating items from L1 and L2 until one list has been exhausted. The remaining items from the other list are then appended to the end of the new list, and the new list is returned. For example, if L1 = ["hop", "skip", "jump", "rest"] and L2 = ["up", "down"], then the function would return the list: ["hop", "up", "skip", "down", "jump", "rest"]. HINT: Python has a built-in function min() which is helpful here. Initialize accumulator variable newlist to be an empty list Set min_length = min(len(L1), len(L2)), the smaller of the two list lengths Use a for loop to iterate k over range(min_length) to do the first part of this function's work. On each iteration, append to newlist the item from index k in L1, and then append the item from index k in L2 (two appends on each iteration). AFTER the loop…arrow_forward//import java.util.List; import javax.swing.*; import javax.swing.event.*; import java.awt.*; /** * JList basic tutorial and example * * @author wwww.codejava.net */ public class JListTask1_Student_Version extends JFrame { //define a Jlist Component // Define a String array that use to create the list private JPanel ContryPanel; // To hold components private JPanel selectedcontryPanel; // To hold components // define a TextFiles to show the result private JLabel label; // A message /** Constructor */ public JListTask1_Student_Version(){ setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setTitle("JList Task1"); setSize(200, 200); setLayout(new BorderLayout()); // Build the month and selectedMonth panels. // Add the panels to the content pane. // add(new JScrollPane(countryList)); setLocationRelativeTo(null); setVisible(true); } public void buildcontryPanel() { // Create a panel to hold the list. ContryPanel = new JPanel(); //create the list // Set the selection mode to single…arrow_forwardNeed help making a java file that combines both linearSearch and binarySearch •Both search methods must use the Comparable<T> interface and the compareTo() method.•Your program must be able to handle different data types, i.e., use generics.•For binarySearch, if you decide to use a midpoint computation formula that is different fromthe textbook, explain that formula briefly as a comment within your code. //code from textbook //linearSearch public static <T> boolean linearSearch(T[] data, int min, int max, T target) { int index = min; boolean found = false; while (!found && index <= max) { found = data [index].equals(target); index++; } return found; } //binarySearch public static <T extends Comparable<T>> boolean binarySearch(T[] data, int min, int max, T target) { boolean found = false; int midpoint = (min + max)/2; if (data[midpoint].compareTo(target)==0)…arrow_forward
- Make sure the program run smooth and perfectly, make sure no error encounter. compatible for python software. Complete the sample code template to implement a student list as a binary search tree (BST).Specification1. Each Studentrecord should have the following fields: =studentNumber =lastname =firstName =course =gpa2. Run the program from an appropriate menu allowing for the following operations:* Adding a Student record * Deleting a Student record * Listing students by: • All students • A given course • GPA above a certain value • GPA below a certain value class Record:def __init__(self, studentNumber = None, lastname = None, firstName = None, course = None, gpa =None,):self.studentNumber = studentNumberself.lastname = lastnameself.firstName = firstNameself.course = courseself.gpa = gpadef __str__(self):record = "\n\nStudent Number : {}\n".format(self.studentNumber)record += "Lastname : {}\n".format(self.lastname)record += "FirstName : {}\n".format(self.firstName)record…arrow_forwardpython: def typehelper(poke_name): """ Question 5 - API Now that you've acquired a new helper, you want to take care of them! Use the provided API to find the type(s) of the Pokemon whose name is given. Then, for each type of the Pokemon, map the name of the type to a list of all types that do double damage to that type. Note: Each type should be considered individually. Base URL: https://pokeapi.co/ Endpoint: api/v2/pokemon/{poke_name} You will also need to use a link provided in the API response. Args: Pokemon name (str) Returns: Dictionary of types Hint: You will have to run requests.get() multiple times! >>> typehelper("bulbasaur") {'grass': ['flying', 'poison', 'bug', 'fire', 'ice'], 'poison': ['ground', 'psychic']} >>> typehelper("corviknight") {'flying': ['rock', 'electric', 'ice'], 'steel': ['fighting', 'ground', 'fire']} """ # pprint(typehelper("bulbasaur"))#…arrow_forwardImplement a function findMixedCase in Python that: accepts a single argument, a list of words, and returns the index of the first mixed case word in the list, if one exists, (a word is mixed case if it contains both upper and lower case letters), and returns -1 if the all words are either upper or lower case. sample: >> findMixedCase( ['Hello','how','are','you'] ) # 'Hello' is mixed case0arrow_forward
- Definitely upvote you ... but please answer within 30 minutes...and typed answer....no plagiarism... please help me ??arrow_forwardComputer Science Write a program in the Java language that includes: 1. A class for books, taking into account the encapsulation and getter, setter. 2. Entering books by the user using Scanner 3. LinkedList class for: (Adding a new book - Inserting a book- Searching for a book using the ISBN Number - Viewing all books,delete book). 4. Serial number increases automatically with each book that is entered. 5. Printing the names of books is as follows: Serial - ISBN - Name - PubYear - Price - Notes. 6-Design a main menu that includes: • Add Book • Insert Book • Delete Book • Search . Display . Exitarrow_forwardDesign and implement a service that simulates PHP loops. Each of the three loop variants should be encapsulated in an object. The service can be controlled via a parameter and execute three different simulations. The result is returned as JSON. The input is an array consisting of the letters $characters = [A-Z]. -The For loop should store all even letters in an array.-The Foreach loop simulation is to create a backward sorted array by For loop, i.e. [Z-A] .-The While loop should write all characters into an array until the desired character is found. Interface:-GET Parameter String: loopType ( possible values: REVERSE, EVEN, UNTIL )-GET parameter String: until (up to which character) Output:JSON Object: {loopName: <string>, result: <array> }arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
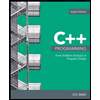
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning