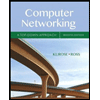
1. Create a project with a common package and three files, separating interface from implementation and use. One of your files will be hosting the Main class which you can use to test and debug your project.
2. Implement the given BagInterface in a class VectorBag. This class will not have a static size (n), but you will allow it to grow dynamically. Recall that we collect arbitrary objects, so you need to use
3. Test and debug your project.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 7 images

- Say we are about to build an ArrayList. Your ArrayList should guarantee that the array capacity is at most four times the number of elements. What would you like to do to maintain such a limit on the capacity? What is the benefit of using iterators? Can you describe your first experience of GUIs? And could you describe what is the advantage of using GUIs over Command-Line Interface (CLI) operations?arrow_forwardThe program first reads integer cityCount from input, representing the number of pairs of inputs to be read. Each pair has a string and a character. One City object is created for each pair and added to vector cityList. Write the PrintSelectedCities() function in the SmallTowns class using Print() to output all the City objects with tour status equal to 'N'. Ex: If the input is: 4 Sundance N Adomstown N Hum Y Hanna Y then the output is: City: Sundance, Tour: N City: Adomstown, Tour: N Note: The vector has at least one element. #include <iostream>#include <vector>using namespace std; class City {public:void SetNameAndTour(string newName, char newTour); char GetTour() const; void Print() const;private:string name;char tour;}; void City::SetNameAndTour(string newName, char newTour) {name = newName;tour = newTour;} char City::GetTour() const { return tour;} void City::Print() const { cout << "City: " << name << ", Tour: " << tour <<…arrow_forwardUsing Java. Implement a deque, except base your implementation on an array. The constructor for the class should accept an integer parameter for the capacity of the deque and create an array of that size. Use a graphical user interface based on an array of text fields similar to what is shown in the image attached. Test your deque class by constructing a deque with capacity 10. Below also is an unfinished code for the challenge. #################################Array Based Deque################################# public class ArrayBaseDeque{private String [ ] q; // Holds queue elementsprivate int front; // Next item to be removedprivate int rear; // Next slot to fillprivate int size; // Number of items in queue private int count = 0;/**Constructor.@param capacity The capacity of the queue.*/ArrayBaseDeque(int capacity){q = new String[capacity];front = 0; rear = 0;size = 0;}/**The capacity method returns the length of the array used to implement the queue.@return The capacity of the…arrow_forward
- implement a database about people, they could be made up, but you want to include a sizable number, maybe 10-20. The data that you store for each person must include a name and at least one additional piece of information, such as a birthday. You can assume that the names are unique. The database should enable you to enter, remove, modify, or search this data. You also should be able to save the data in a file for use later. Define a class Person to represent a person and another one to represent the database. A dictionary should be a data member of the database class, with Person objects as search keys for this dictionary. Write a program that tests and demonstrates your database.arrow_forwardCreate a new project named lab10. You will be implementing two classes: A SolarSystem class, and a Planet class. The SolarSystem contains Planet objects stored in a vector. A UML diagram for the Solar System and Planet classes The first part you should implement is the Planet class. Don't add the SolarSystem files to the project at the beginning, just work on the Planet class. Test the different overloaded operators as we did during lecture for your Planet class, and then move on to the SolarSystem class. The SolarSystem default constructor should resize the vector to zero. The default constructor values for Planet can be whatever you’d like, but make the mass a very small number, so later on when you're looking for the largest planet, it doesn't use the default Planet value. The addPlanets() method will take a number as an argument for the number of Planets that will be in your SolarSystem. So if you call addPlanets with 5 as an argument, there will be 5 Planets to add to the…arrow_forwardFor the Assignment 5 (Part 2), Routes v.2 at the end of this module you will create a Route class that has a member a "bag" of Leg objects. The bag is to use a vector of pointers, but since all the objects in the bag are to be Leg s, it's okay to use Leg* instead of void* in the bag's declaration. You wrote its first constructor in a previous exercise. Now write a second one. (You may refer to the Assignment page to refer to the completed class declarations of Leg and Route classes). Assume that class Leg has data member C strings const char* const beginCity; and const char* const endCity; that are available to Route by a friend relationship. Write a constructor function for a Route class that takes exactly two parameters: a constant Route object reference to an already-existing Route , and a constant Leg object reference to be appended to the end of that Route to form the new Route . Here's the algorithm: Copy the first parameter's bag into the host object's bag. If the endCity of…arrow_forward
- in Javaarrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forwardTest your Baby class by writing a client program which uses an array to storeinformation about 4 babies. That is, each of the four elements of the arraymust store a Baby object If you have an array for baby names and another array for baby ages,then you have missed the point of the exercise and therefore not metthe requirement of this exercise. A Baby class object stores the required information about a Baby. Soeach Baby object will have its own relevant information, and thus eachobject must be stored in one element of the array.The client program should:a. Enter details for each baby (name and age) and thus populate theBaby arrayb. Output the details of each baby from the array (name and age)c. Calculate and display the average age of all babies in the arrayd. Determine whether any two babies in the array are the sameAs the required information for these tasks is stored in the Baby array, youwill need to use a loop to access each array element (and use the dot notationto access the…arrow_forward
- You have to use comment function to describe what each line does import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class PreferenceData { private final List<Student> students; private final List<Project> projects; private int[][] preferences; private static enum ReadState { STUDENT_MODE, PROJECT_MODE, PREFERENCE_MODE, UNKNOWN; }; public PreferenceData() { super(); this.students = new ArrayList<Student>(); this.projects = new ArrayList<Project>(); } public void addStudent(Student s) { this.students.add(s); } public void addStudent(String s) { this.addStudent(Student.createStudent(s)); } public void addProject(Project p) { this.projects.add(p); } public void addProject(String p) { this.addProject(Project.createProject(p)); } public void createPreferenceMatrix() { this.preferences = new…arrow_forwardBuild two classes (Fraction and FractionCounter) and a Driver for use in counting the number of unique fractions read from a text file. We’ll also reuse the ObjectList class we built in lab to store our list of unique FractionCounters, instead of directly using arrays or the ArrayList. Remember NO DECIMALS! Handle input of any length Introduction Your project is to read in a series of fractions from a text file, which will have each line formatted as follows: “A/B”. A sample text file is listed below, and the purpose of your program is to read in one line at a time and build a Fraction object from A and B. For each unique Fraction seen, your program will create a FractionCounter object used to track the number of occurrences of that specific fraction. When all the input is consumed, your program will print out its ObjectList of unique FractionCounters, which should report the fraction and its count – see output below. You can assume no blank lines or misleading characters; see…arrow_forwardWrite a program that uses two classes. The first class is called “dAta” and holds x and y coordinates of a point in 2-d space called p1. The second class is called “cOmpute” and holds an array of two pointers to the “dAta” class. The “cOmpute” class has a function, lOad(float x, float y, int n), which loads x and y coordinate data into the array at index n. It also has a function, sLope(), which computes the slope of the line connecting the two array data coordiantes. It also has a function, pRint(), which prints the slope result to the screen. Implement a divide by zero exception using throw in the sLope() nd/or pRint() function as appropriate. The exception should, when caught, print “Slope calculation error…” to the screen and exit the function. The program should load the array with some example points and print the slope to the screen using the class functions.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
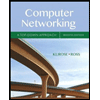
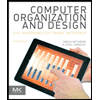
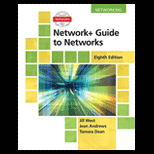
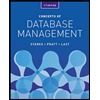
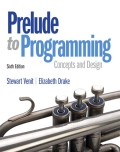
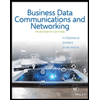