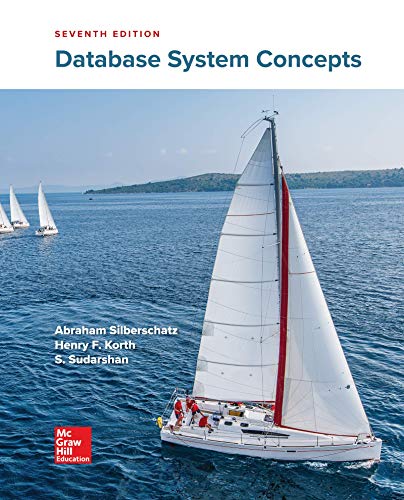
Concept explainers
#include <iostream>
#include <string>
#include <ctime>
using namespace std;
void displayArray(double * items, int start, int end)
{
for (int i = start; i <= end; i++)
cout << items[i] << " ";
cout << endl;
}
//The legendary "Blaze Sort"
//Sorts the specified portion of the array between index start and end (inclusive)
//Hmmm... how fast is it? /*
void blazeSort(double * items, int start, int end)
{
if (end - start > 0)
{
int p = filter(items, start, end);
blazeSort(items, start, p - 1);
blazeSort(items, p + 1, end);
}
}
*/
int main()
{
//////////////////////////////////////////////////// //Part 1: Implement a method called filter.
//////////////////////////////////////////////////// //Filter is a function that takes in an array and a range (start and end).
//
//Call the first item in the range the 'pivot'.
//
//Filter's job is to simply separate items within the range based on whether they are bigger or smaller than the pivot.
//In the example array below, 13 is the pivot, so all items smaller than 13 are placed in indices 0-3. The pivot is then placed at index 4, and all
//remaining items, which are larger than the pivot, are placed at positions 5-10. Note that the array is NOT sorted, just "partitioned" around
//the pivot value. After doing this, the function must return the new index of the pivot value.
double testNumsA[] = { 13, 34.1, 43, 189, 4, 4.5, 18.35, 85, 3, 37.2, 5 };
//The filter will place all items <= 13 to the left of value 13, and all items large than 13 to the right of 13 in the array.
int p = filter(testNumsA, 0, 10);
cout << p << endl;
//should be 4, the new index of 13.
displayArray(testNumsA, 0, 10);
//should display something like this: 5 3 4.5 4 13 18.35 85 189 37.2 43 34.1 //One more example:
double testNumsB[] = { 13, 34.1, 43, 189, 4, 4.5, 18.35, 85, 3, 37.2, 5 };
p = filter(testNumsB, 2, 6);
//Here we are only interested in items from indices 2-6, ie, 43, 189, 4, 4.5, 18.35
cout << p << endl;
//should be 5
displayArray(testNumsB, 0, 10);
//Notice only indices 2-6 have been partioned: 13 34.1 18.35 4 4.5 43 189 85 3 37.2 5
///////////////////////////////////////////////////////////////////////////////// //Part 2: Uncomment "Blaze Sort". //Blaze Sort uses/needs your filter to work properly.
///////////////////////////////////////////////////////////////////////////////// //Test if Blaze Sort correctly sorts the following array.
double testNums[] = { 13, 34.1, 43, 189, 4, 4.5, 18.35, 85, 3, 37.2, 5 }; blazeSort(testNums, 0, 10); displayArray(testNums, 0, 10);
///////////////////////////////////////////////////////////////////// //Part 3: Test how fast Blaze Sort is for large arrays.
//What do you think the run-time (big-Oh) of blaze sort is? ///////////////////////////////////////////////////////////////////// //Stress test:
int size = 100;
//test with: 1000, 10000, 100000,1000000, 10000000
double * numbers = new double[size];
for (int i = 0; i < size; i++)
{
numbers[i] = rand();
}
clock_t startTime, endTime;
startTime = clock();
blazeSort(numbers, 0, size - 1);
endTime = clock();
displayArray(numbers, 0, size - 1);
cout << "Blaze sort took: " << endTime - startTime << " milliseconds to sort " << size << " doubles." << endl;
//////////////////////////////////////////////////////////////////// //Part 4: Sort Moby Dick, but this time with Blaze Sort /////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////
//1) Create a second version of Blaze Sort that sorts arrays of strings //(instead of arrays of doubles).
//2) Download whale.txt from the previous lab. Read the words from the file into //an array, sort the array with Blaze Sort, and then write the sorted words to an output file. // //This time, it has to be fast! Must finish in under 10 seconds. /////////////////////////////////////////////////////////////////
return 0;
}
![```cpp
////////////////////////////////////////////////////////////////////
// Part 2: Uncomment "Blaze Sort".
// Blaze Sort uses/needs your filter to work properly.
////////////////////////////////////////////////////////////////////
// Test if Blaze Sort correctly sorts the following array.
double testNums[] = { 13, 34.1, 43, 189, 4, 4.5, 18.35, 85, 3, 37.2, 5 };
blazeSort(testNums, 0, 10);
displayArray(testNums, 0, 10);
////////////////////////////////////////////////////////////////////
// Part 3: Test how fast Blaze Sort is for large arrays.
// What do you think the run-time (big-Oh) of blaze sort is?
////////////////////////////////////////////////////////////////////
// Stress test:
int size = 100; // test with: 1000, 10000, 100000, 1000000, 10000000
double * numbers = new double[size];
for (int i = 0; i < size; i++)
{
numbers[i] = rand();
}
clock_t startTime, endTime;
startTime = clock();
blazeSort(numbers, 0, size - 1);
endTime = clock();
displayArray(numbers, 0, size - 1);
cout << "Blaze sort took: " << endTime - startTime << " milliseconds to sort " << size << " doubles." << endl;
////////////////////////////////////////////////////////////////////
// Part 4: Sort Moby Dick, but this time with Blaze Sort
////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////
// 1) Create a second version of Blaze Sort that sorts arrays of strings
// (instead of arrays of doubles).
// 2) Download whale.txt from the previous lab. Read the words from the file into
// an array, sort the array with Blaze Sort, and then write the sorted words to an output file.
// This time, it has to be fast! Must finish in under 10 seconds.
////////////////////////////////////////////////////////////////////
return 0;
```
This code snippet is from a sorting algorithm implementation designed to evaluate the performance and correctness of "Blaze Sort." The code is divided into different parts for thorough testing and analysis:
- **Part 2:** The code tests if Blaze Sort can correctly sort a predefined array of doubles.
- **Part 3:** This section is a stress test to measure the speed of Blaze Sort with larger arrays. It hints at using different array sizes and involves clocking the sorting operation to determine its efficiency in milliseconds.
- **](https://content.bartleby.com/qna-images/question/01a138b1-4237-4983-ac1d-3a78af50f30a/4de77f65-105c-412f-80a6-aa9c37a85f4b/6yepzw_thumbnail.png)
![```cpp
#include <iostream>
#include <string>
#include <ctime>
using namespace std;
void displayArray(double * items, int start, int end)
{
for (int i = start; i <= end; i++)
cout << items[i] << " ";
cout << endl;
}
//The legendary "Blaze Sort" algorithm.
//Sorts the specified portion of the array between index start and end (inclusive)
//Hmm... how fast is it?
/*
void blazeSort(double * items, int start, int end)
{
if (end - start > 0)
{
int p = filter(items, start, end);
blazeSort(items, start, p - 1);
blazeSort(items, p + 1, end);
}
}
*/
int main()
{
//////////////////////////////////////////////////////
// Part 1: Implement a method called filter.
//////////////////////////////////////////////////////
// Filter is a function that takes in an array and a range (start and end).
//
// Call the first item in the range the 'pivot'.
//
// Filter's job is to simply separate items within the range based on whether they are bigger or smaller than the pivot.
// The example array below, 13 is the pivot, so all items smaller than 13 are placed in indices 0-3. The pivot is then placed at index 4, and all
// remaining items, which are larger than the pivot, are placed at positions 5-10. Note that the array is NOT sorted, just "partitioned" around
// the pivot value. After doing this, the function must return the new index of the pivot value.
double testNumsA[] = { 13, 34.1, 43, 189, 4, 4.5, 18.35, 85, 3, 37.2, 5 };
// The filter will place all items <= 13 to the left of value 13, and all items large than 13 to the right of 13 in the array.
int p = filter(testNumsA, 0, 10);
cout << p << endl; // should be 4, the new index of 13.
displayArray(testNumsA, 0, 10); // should display something like this: 5 3 4.5 4 13 18.](https://content.bartleby.com/qna-images/question/01a138b1-4237-4983-ac1d-3a78af50f30a/4de77f65-105c-412f-80a6-aa9c37a85f4b/tpxib6b_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- C++arrow_forwardRemove Char This function will be given a list of strings and a character. You must remove all occurrences of the character from each string in the list. The function should return the list of strings with the character removed. Signature: public static ArrayList<String> removeChar(String pattern, ArrayList<String> list) Example:list: ['adndj', 'adjdlaa', 'aa', 'djoe']pattern: a Output: ['dndj', 'djdl', '', 'djoe']arrow_forwardCheck 1 ALLLEURE #include #include using namespace std; void PrintSize(vector numsList) { cout intList (2); PrintSize(intList); cin >> currval; while (currVal >= 0) { } Type the program's output intList.push_back(currval); cin >> currval; PrintSize(intList); intList.clear(); PrintSize(intList); return 0; CS Scanned with Calin canner Janviantars Input 12345-1 Output Feedback? 口口。arrow_forward
- Python function getData(fname:str)->ndarray that inputs data from a file into a list, then converts the list to a NumPy array of integer values and returns the array. The text file (fname) contains: integer numbers, one per line numbers are in the range 0 <= n <= 99 r * c (rows * columns) quantity of numbers, where c = r + 1 Example: 15 22 65 4 78 91 7 35 60 16 99 12 == [[15 22 65 4] [78 91 7 35] [60 16 99 12]]arrow_forward1. Stack Implementation Write a method called insert for the class Stack. The method shall be implemented using an array-based structure. You can assume that String[] stack is defined in the class, which is responsible to store the elements and responsible to enforce the order of first-in last-out, a.k.a., FIFO. Additionally, you can assume there is a pointer called top, that indicates the position of the top of the stack, pointing to the next available position to insert. The method shall: • take a String s as a parameter, and shall add it at the top of the stack. shall return true if the element s was added successfully at the top of the stack, false otherwise. . . The method must check boundaries of capacity and limitation of the Stack. In case the method is invoked to insert an element of the top of the stack that exceeds its current capacity, the method shall handle the situation properly. Do not provide the entire Stack implementation, only the code solution of the method.arrow_forward//main.cpp file #include <iostream> #include "helper.h" #include "sorting.h" using namespace std; int main() { unsigned int size; cout << "Enter the size of the array: "; cin >> size; int* Array = new int[size]; unsigned int choice = 0; do { fillRandom(Array, size); cout << "Choose your sorting algorithm:" << endl; cout << "1. Bubble Sort" << endl; cout << "2. Selection Sort" << endl; cout << "3. Insertion Sort" << endl; cout << "4. Merge Sort" << endl; cout << "5. Quick Sort" << endl; cout << "6. Shell Sort" << endl; //Optional cout << "Enter 0 to exit" << endl; cout << "Your choice: "; cin >> choice; cout << "Unsorted Array: "; print(Array, size); /***************************** TODO: Implement what you will do with the choice*****************************/ cout << "Sorted Array: "; print(Array, size); } while(choice!=0); delete [] Array; return 0; }…arrow_forward
- #include <iostream>using namespace std;double median(int *, int);int get_mode(int *, int);int *create_array(int);void getinfo(int *, int);void sort(int [], int);double average(int *, int);int getrange(int *,int);int main(){ int *dyn_array; int students; int mode,i,range;float avrg;do{cout << "How many students will you enter? ";cin >> students;}while ( students <= 0 );dyn_array = create_array( students );getinfo(dyn_array, students);cout<<"\nThe array is:\n";for(i=0;i<students;i++)cout<<"student "<<i+1<<" saw "<<*(dyn_array+i)<<" movies.\n";sort(dyn_array, students);cout << "\nthe median is "<<median(dyn_array, students) << endl;cout << "the average is "<<average(dyn_array, students) << endl;mode = get_mode(dyn_array, students);if (mode == -1)cout << "no mode.\n";elsecout << "The mode is " << mode << endl;cout<<"The range of movies seen is…arrow_forwardArrays write a C++ program that will declare two static arrays where each will hold the contents of a file (random.txt) of 200 integer values. Sorting Using the two arrays, call a private member function that uses the bubble sort algorithm to sort one of the arrays in ascending order. The function should count the number of exchanges it makes. Display this value. The program should then call a private member function that uses the selection sort algorithm to sort the other array. It should also count the number of exchanges it makes. Display this value. Searching Next, call a private member function that uses the linear search program to locate the value 869. The function should keep a count of the number of comparisons it makes until it finds the value. Display this value The program then should call a private member function that uses the binary search algorithm to locate the same value. It should also keep count of the numbers of comparisons it makes. Display this…arrow_forward#include <iostream> using namespace std; void insertElement(int* LA, int ITEM, int N, int K){ int J=N; //initialize j to n while(J>=K) //enters loop only if j less than or equal to k { LA[J+1]=LA[J]; //stores current value in next index J=J-1; //decrement j } LA[K]=ITEM; //insert element at index k N=N+1; //increment n by 1 //this is just used for printing and you can ignore if not required for(int i=0;i<N;i++) //i from 0 to n { cout << LA[i] << " "; //print element at index i }} int main(){ int LA[6]={1,2,3,4,5}; //declare an array and initialize values to it insertElement(LA,6,5,3); //call function to insert 6 at index 3 return 0;}note: Remove Funcationarrow_forward
- struct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forward#include <iostream>using namespace std;double median(int *, int);int get_mode(int *, int);int *create_array(int);void getinfo(int *, int);void sort(int [], int);double average(int *, int);int getrange(int *,int);int main(){ int *dyn_array; int students; int mode,i,range;float avrg;do{cout << "How many students will you enter? ";cin >> students;}while ( students <= 0 );dyn_array = create_array( students );getinfo(dyn_array, students);cout<<"\nThe array is:\n";for(i=0;i<students;i++)cout<<"student "<<i+1<<" saw "<<*(dyn_array+i)<<" movies.\n";sort(dyn_array, students);cout << "\nthe median is "<<median(dyn_array, students) << endl;cout << "the average is "<<average(dyn_array, students) << endl;mode = get_mode(dyn_array, students);if (mode == -1)cout << "no mode.\n";elsecout << "The mode is " << mode << endl;cout<<"The range of movies seen is…arrow_forward#include <iostream>#include <cstdlib>#include <time.h>#include <chrono> using namespace std::chrono;using namespace std; void randomVector(int vector[], int size){ for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; }} int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast<microseconds>(stop - start); cout << "Time taken by function: " << duration.count()…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
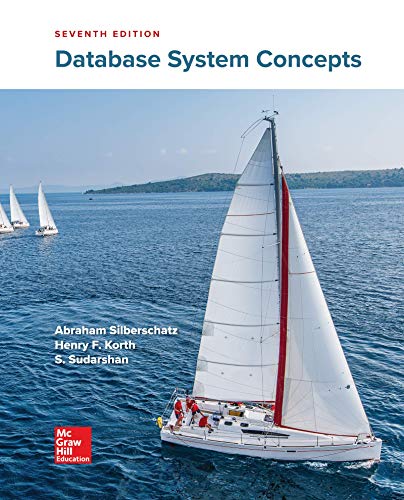
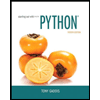
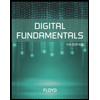
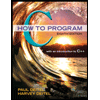
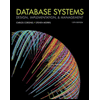
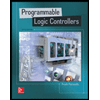