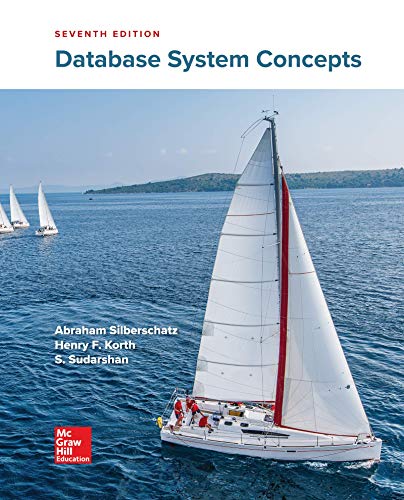
Concept explainers
Does the below program use recursion to create a sierpinski triangle?
Souce code:
package application;
public class Triangle {
publicstaticvoid main(String [] args) {
TriangleFrame tf = new TriangleFrame();
}
}
package application;
import java.awt.*;
import javax.swing.*;
public class SierpinskiGasket extends JPanel {
public SierpinskiGasket() {
}
public void drawTriangle (Graphics g, int x, int y, int width, int height) {
if (height == 1) {
g.drawRect(x, y, width, height);
}
else {
drawTriangle(g, x + (width / 4), y, width / 2, height / 2);
drawTriangle(g, x, y + (height / 2), width / 2, height / 2);
drawTriangle(g, x + (width / 2), y + (height / 2), width/ 2, height / 2);
}
}
public void paintComponent (Graphics g) {
super.paintComponent(g);
drawTriangle(g, 0, 0, this.getWidth(), this.getHeight());
}
}

The Sierpinski triangle is a well-known fractal pattern that can be created by recursively subdividing an equilateral triangle into smaller equilateral triangles. It exhibits self-similar and intricate geometric properties.
Step by stepSolved in 3 steps

- Question 2. package sortsearchassigncodeex1; import java.util.Scanner; import java.io.*; // // public class Sortsearchassigncodeex1 { // public static void fillArray(Scanner inputFile, int[] arrIn){ int indx = 0; //Complete code { arrIn[indx] = inputFile.nextInt(); indx++; } }arrow_forward1 import java.util.Scanner; 3 public class TriangleArea { INM&567 000 2 4 8 9 10 11 12 13 14 15 4567890 16 17 18 19 20 21 } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); } Current file: TriangleArea.java = Triangle triangle1 new Triangle(); Triangle triangle2 = new Triangle(); // TODO: Read and set base and height for triangle1 (use setBase() and setHeight()) // TODO: Read and set base and height for triangle2 (use setBase() and setHeight()) System.out.println("Triangle with smaller area:"); // TODO: Determine smaller triangle (use getArea()) // and output smaller triangle's info (use printInfo())arrow_forwardHow do I remove the space at the end of the result? Code: import java.util.Scanner; public class FinalExamAnswers{ public static void main(String [] args) { manipulateString(); //calls function } //your code here public static void manipulateString() { Scanner sc=new Scanner(System.in); //create Scanner instance System.out.println("Enter a sentence"); String sentence=sc.nextLine(); //input a sentence String[] words=sentence.split(" "); //split the sentence at space and store it in array for(int i=0;i<words.length;i++) //i from 0 to last index { if(i%2==0) //if even index words[i]=words[i].toUpperCase(); //converted to upper case else //if odd index words[i]=words[i].toLowerCase(); //converted to lower case } for(int i=words.length-1;i>=0;i--) //i from last index to 0 {…arrow_forward
- import java.util.Scanner; public class TriangleArea { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Triangle triangle1 = new Triangle(); Triangle triangle2 = new Triangle(); // TODO: Read and set base and height for triangle1 (use setBase() and setHeight()) // TODO: Read and set base and height for triangle2 (use setBase() and setHeight()) System.out.println("Triangle with smaller area:"); // TODO: Determine smaller triangle (use getArea()) // and output smaller triangle's info (use printInfo()) }} public class Triangle { private double base; private double height; public void setBase(double userBase){ base = userBase; } public void setHeight(double userHeight) { height = userHeight; } public double getArea() { double area = 0.5 * base * height; return area; } public void printInfo() { System.out.printf("Base:…arrow_forwardimport java.util.Scanner; public class CharMatch { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userString; char charToFind; int strIndex; userString = scnr.nextLine(); charToFind = scnr.next().charAt(0); strIndex = scnr.nextInt(); /* Your code goes here */ }}arrow_forwardFix the code below so that there is a function that calculate the height of the triangle. package recursion; import javax.swing.*;import java.awt.*; /** * Draw a Sierpinski Triangle of a given order on a JPanel. * * */public class SierpinskiPanel extends JPanel { private static final int WIDTH = 810; private static final int HEIGHT = 830; private int order; /** * Construct a new SierpinskiPanel. */ public SierpinskiPanel(int order) { this.order = order; this.setMinimumSize(new Dimension(WIDTH, HEIGHT)); this.setMaximumSize(new Dimension(WIDTH, HEIGHT)); this.setPreferredSize(new Dimension(WIDTH, HEIGHT)); } public static double height(double size) { double h = (size * Math.sqrt(3)) / 2.0; return h; } /** * Draw an inverted triangle at the specified location on this JPanel. * * @param x the x coordinate of the upper left corner of the triangle * @param y the y…arrow_forward
- Please help fastarrow_forwardimport java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forwardI have this code: import java.util.Scanner; public class CalcTax { public static void main(String[] args) { Scanner sc = new Scanner(System.in); // Welcome message System.out.println("Welcome to my tax calculation program."); // Declare the variables int i, pinCode, maxRetries = 3; String choice; // Get the PIN code for (i = 0; i < maxRetries; i++) { System.out.print("Please enter the PIN code: "); pinCode = sc.nextInt(); if (pinCode == 5678) { break; } System.out.println("Invalid pin code. Please try again."); } if (i == maxRetries) { System.out.println("Invalid pin code. You have reached the maximum number of retries."); System.exit(0); } // Get the income, interest, deduction, and paid tax amount double income, interest, deduction, paidTax; do { System.out.print("Please…arrow_forward
- import javax.swing.JOptionPane; public class Addition{public static void main( String args[] ){for (int i = 0; i < 10; i++) {String Number = JOptionPane.showInputDialog( "Enter a number" );int number = Integer.parseInt( Number );int sum = number;JOptionPane.showMessageDialog( null, "The sum is " + sum,"Sum of 10 numbers", JOptionPane.PLAIN_MESSAGE );}}} I want to add the number that was entered but I don't know how to do that. Do you know how to add the entered number?arrow_forwardimport java.awt.*; public class TestRectangle {public static void main(String[] args) {Rectangle r1 = new Rectangle(5, 4, 10, 17);Rectangle r2 = new Rectangle(10, 10, 20, 3);Rectangle r3 = new Rectangle(0, 1, 12, 15);Rectangle r4 = new Rectangle(10, 10, 20, 3);System.out.println("r1 = " + r1);System.out.println("r2 = " + r2);System.out.println("r3 = " + r3);System.out.println("r2 equals r1? " + r2.equals(r1));System.out.println("r2 equals r2? " + r2.equals(r2));System.out.println("r2 equals r3? " + r2.equals(r3));System.out.println("r2 equals r4? " + r2.equals(r4)); System.out.println("r1 contains (6, 8)? = " + r1.contains(6, 8));System.out.println("r2 contains (6, 8)? = " + r2.contains(6, 8));System.out.println("r3 contains (6, 8)? = " + r3.contains(6, 8));System.out.println("r2 contains (30, 13)? = " + r2.contains(30, 13)); r1.intersect(r3);r2.intersect(r4);System.out.println("r1 intersect r3 = " + r1);System.out.println("r2 intersect r4 = " + r2);…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
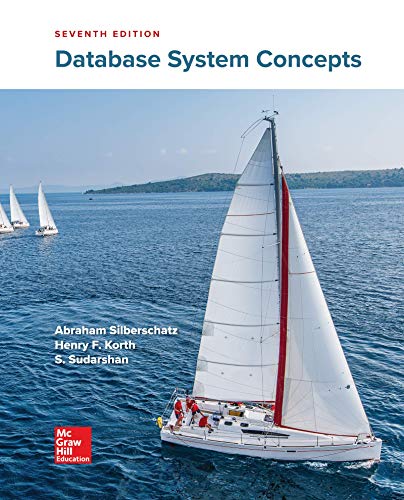
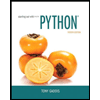
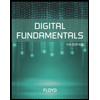
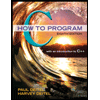
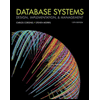
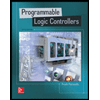