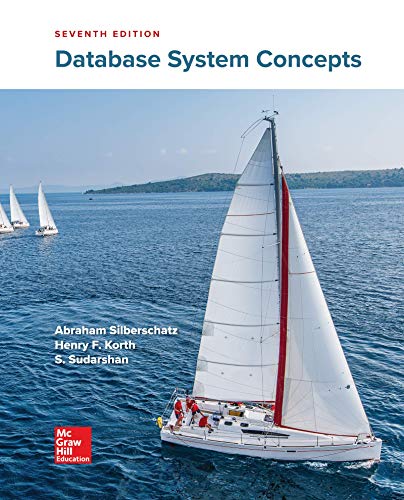
Concept explainers
Given is Parser.java. Methods must accept tokens appropriately and generates OperationNode or returns partial result. I need the methods to be created for the following:
-Post Increment/Decrement
-Exponents
-Factor
-Term
-Expression
-Concatenation
-Boolean Compare
-Match
-Array Membership
-And
-Or
-Ternary
-Assignment
Parser.java

It is essential to use techniques that can effectively handle a broad range of operations when creating a parser for a programming language. Exponentiation, factors, terms, expressions, concatenation, boolean comparisons, matching operations, array membership checks, logical AND and OR operations, ternary conditionals, and assignment operations are some of the operations in this list. The syntax and semantics of the language are significantly influenced by each of these procedures. We will describe the crucial methods that must be added to the Parser.java class in this context in order to successfully parse and produce abstract syntax trees (ASTs) or deliver partial results for these tasks.
Please refer to the following steps for the complete solution to the problem above.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

This is an incomplete code. Where are the implementations for each method you mentioned in the comments? I need the implementation logic (code) for each method you mentioned in the comments. Please include the code implementation logic for each method instead of the comments.
This is an incomplete code. Where are the implementations for each method you mentioned in the comments? I need the implementation logic (code) for each method you mentioned in the comments. Please include the code implementation logic for each method instead of the comments.
- Im trying to figure out a java homework assignment where I have to evaluate postfix expressions using stacks and Stringtokenizer as well as a isNumber method. when I try to run it it gives me an error. this is the code I have so far: static double evaluatePostfixExpression(String expression){ // Write your code Stack aStack = new Stack(); double value1, value2; String token; StringTokenizer tokenizer = new StringTokenizer(expression); while (tokenizer.hasMoreTokens()) { token = tokenizer.nextToken(); if(isNumber(expression)) { value1 = (double)aStack.pop(); value2 = (double)aStack.pop(); if (token.equals("+")) { aStack.push(value2+value1); } else if (token.equals("-")) { aStack.push(value2-value1); } else if (token.equals("*")) { aStack.push(value2*value1); } else if (token.equals("/")) { aStack.push(value2/value1); } } }…arrow_forwardJava Code: Below is Parser.java and there are errors. getType() and getStart() is undefined for the type Optional<Token> and there is an error in addNode(). Make sure to get rid of all the errors in the code. Attached is images of the errors. Parser.java import java.text.ParseException;import java.util.LinkedList;import java.util.List;import java.util.Optional; import javax.swing.ActionMap; public class Parser { private TokenHandler tokenHandler; private LinkedList<Token> tokens; public Parser(LinkedList<Token> tokens) { this.tokenHandler = new TokenHandler(tokens); this.tokens = tokens; } public boolean AcceptSeparators() { boolean foundSeparator = false; while (tokenHandler.MoreTokens()) { Optional<Token> currentToken = tokenHandler.getCurrentToken(); if (currentToken.getType() == Token.TokenType.NEWLINE || currentToken.getType() == Token.TokenType.SEMICOLON) {…arrow_forwardHello, i need help with understanding Big-O. What is the execution time Big-O for each of the methods in the Queue-1.java interface for the ArrayQueue-1.java and CircularArrayQueue-1.java implementations? Short Big-O reminders: Big-O is a measure of the worst case performance (in this case execution time). O(1) is constant time (simple assignment statements) O(n) is a loop that iterates over all of the elements. O(n2) is one loop nested in another loop, each of which iterates over all the elements. If a method calls another method, what happens in the called method must be taken into consideration. Queue.java public interface Queue<E> { /** * The element enters the queue at the rear. */ public void enter(E element); /** * The front element leaves the queue and is returned. * @throws java.util.NoSuchElementException if queue is empty. */ public E leave(); /** * Returns True if the queue is empty. */ public boolean…arrow_forward
- import java.util.Comparator; import java.util.PriorityQueue; class StringLengthComparator implements Comparator public int compare(String o1, String 02) if (01.length()02.length()){ return 1; }else{ } } } } class StringLengthComparator1 implements Comparator { return 0; public int compare(String o1, String o2) { if(01.length()02.length()){ return -1;//means here we swap it }else{ } } return 0; public class StringLength { public static void main(String[] args) { String arr[] = {"this", "at", "a", "their", "queues"}; StringLengthComparator string Comparator = new StringLengthComparator(); StringLengthComparator1 string Comparator1 = new StringLengthComparator1(); PriorityQueue pq = new PriorityQueue(stringComparator); PriorityQueue pq1 = new PriorityQueue(stringComparator1); for(int i=0;iarrow_forwardConsider the following implementation of binarySearch: public static bool binarySearch(int[] a, int item) { int first = 0; int last = a.length - 1; while( first <= last) { int mid = (first + last)/2; if(a[mid] item) return true; else if (a[mid] < item) first = mid + 1; else last = mid - 1; } return false; == } how many times is the line of code int mid = (first + last)/2; executed when it is called with a = {2, 3, 5, 7, 11, 13,17, 19, 23, 29, 31, 37} and item = 2?arrow_forwardYour crazy boss has assigned you to write a linear array-based implementation of the IQueue interface for use in all the company's software. You've tried explaining why that's a bad idea with logic and math, but to no avail. So - suppress your inner turmoil and complete the add() and remove() methods of the AQueue class and identify the runtime order of each of your methods. /** A minimal queue interface */ public interface IQueue { /** Add element to the end of the queue */ public void add(E element); /** Remove and return element from the front of the queue * @returns fırst element * @throws NoSuchElementException if queue is empty */ public E remove(); /** Not an ideal Queue */ public class AQueue implements IQueue{ private El) array: private int rear; public AQueue(){ array = (E[)(new Object[10]): rear = 0: private void expandCapacity) { if (rear == array.length) { array = Arrays.copyOf(array, array.length 2): @Override public void add(E element) { / TODO @Override public E…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
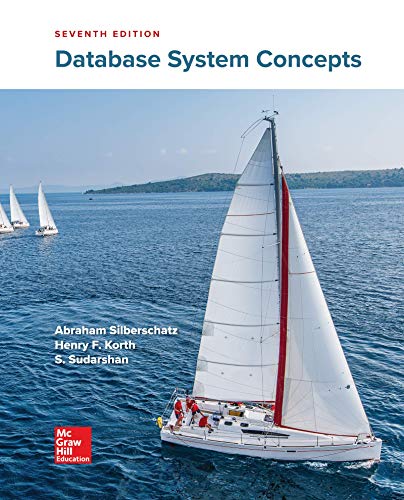
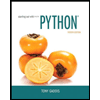
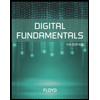
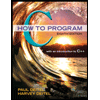
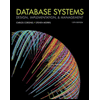
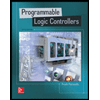