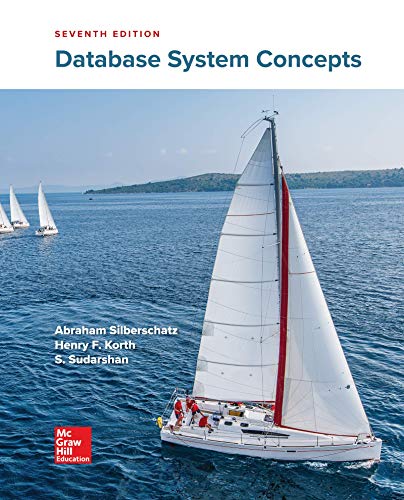
Concept explainers
how do i create a method that adds instances from a class into a singly linked list(java)

Answer :
Inserting a new element into a simply linked list at the beginning is fairly simple. We just need to make a few adjustments to the linking of the nodes. To insert a new node in the list at the beginning, the following steps must be performed.
Allocate space for the new node and store the data in the data part of the node. This will be done using the following statements.
ptr = (struct node *) malloc(sizeof(struct node *));
ptr → data = item
Make the link as part of the new node point to the existing first node of the list. This is done using the following command.
ptr->next = head;
Finally, we need to create a new node as the first node of the list, which we do with the following command.
head = ptr;
Step by stepSolved in 2 steps

- Looking at all four list implementations, which actions/methods tend to be less efficient in the Linked List implementation compared to the Array Implementation? Explain why for each action/method you specify.arrow_forwardA consecutive sequence a list of numbers that are organized in increasing order with the next eleme. one bigger than the current. Write a non-recursive method "lengthConsec", which takes an IntNode myList as the parameter and returns the length of the consecutive sequence in myList. To simplify the implementation, you can assume that there is no more than one consecutive sequence in the list. For example, in the following linked list, the consecutive sequence begins at node "5" and ends at node "7", so lengthConsec (myList) should return 3 in this case. myList 8 13 4 public class IntNode { 12 private int m_data; private IntNode m_link; Consecutive sequence 6 7 28arrow_forwardCreate a Linked list program in java using scanner in one program Add element to LinkedList using Scanner Remove element to LinkedList using Scanner Changing element to LinkedList using Scanner Searching element to LinkedList using Scannerarrow_forward
- Instruction: To test the Linked List class, create a new Java class with the main method, generate Linked List using Integer and check whether all methods do what they’re supposed to do. A sample Java class with main method is provided below including output generated. If you encounter errors, note them and try to correct the codes. Post the changes in your code, if any. Additional Instruction: Linked List is a part of the Collection framework present in java.util package, however, to be able to check the complexity of Linked List operations, we can recode the data structure based on Java Documentation https://docs.oracle.com/javase/8/docs/api/java/util/LinkedList.html package com.linkedlist; public class linkedListTester { public static void main(String[] args) { ListI<Integer> list = new LinkedList<Integer>(); int n=10; for(int i=0;i<n;i++) { list.addFirst(i); } for(int…arrow_forwardJava Programming language Help please.arrow_forwardJAVA plese Implement the indexOf method in the LinkedIntegerList class public int indexOf(int value); /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ } public static void main(String[] args) { // TODO Auto-generated method stub SimpleIntegerListADT myList = null; System.out.println(myList); for(int i=2; i<8; i+=2) { myList.add(i); } System.out.println(myList.indexOf(44));arrow_forward
- Starter code for ShoppingList.java import java.util.*;import java.util.LinkedList; public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }} class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}arrow_forwardPlease fill in all the code gaps if possible: (java) public class LinkedList { privateLinkedListNode head; **Creates a new instance of LinkedList** public LinkedList () { } public LinkedListNode getHead() public void setHead (LinkedListNode head) **Add item at the front of the linked list** public void insertFront (Object item) **Remove item at the front of linked list and return the object variable** public Object removeFront() }arrow_forwardWhich best describes this axiom: aList.getLength ( ) = ( aList.remove ( i ) ) . getLength ( ) + 1 You cannot remove an item from from existing list that is empty Removing an item from an existing list decreases its length by one Removing an item from an existing list increases the list size by one None of thesearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
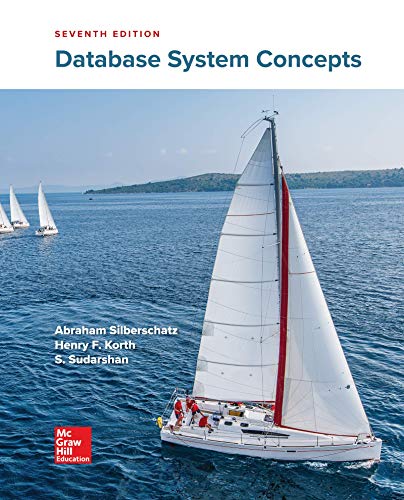
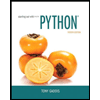
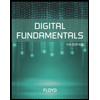
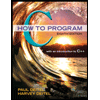
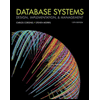
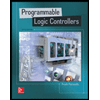