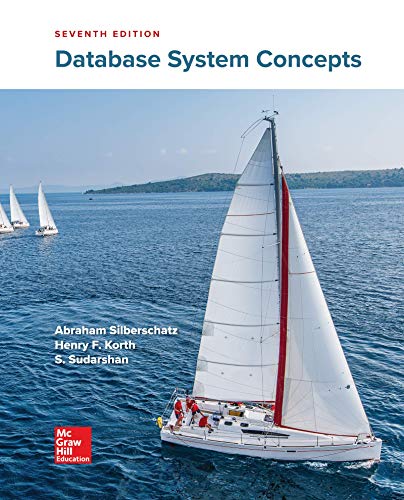
Concept explainers
//In Java Language please
package layoutDemos;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.Pane;
import javafx.scene.layout.StackPane;
import javafx.scene.layout.VBox;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class ButtonsOnTop extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Two Button VBox");
// --------------------------------------------------------------------
VBox vbox1 = new VBox(10); // the attribute sets the space between nodes
vbox1.setPadding(new Insets(15, 15, 15, 15)); // sets space around the edges
GridPane gridBox = new GridPane();
gridBox.setAlignment(Pos.CENTER);
gridBox.setHgap(20);
gridBox.setPadding(new Insets(10, 10, 15, 15));
gridBox.setStyle("-fx-background-color: CYAN");
// --------------------------------------------------------------------
Text txt1 = new Text("Text at the top of the VBox stack");
Text txt2 = new Text("Text at the bottom of the VBox stack");
Button btn1 = new Button();
Button btn2 = new Button();
// --------------------------------------------------------------------
btn1.setText("This is Button ONE");
btn1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
System.out.println("Button One pushed");
txt1.setText("Button ONE pushed");
}
});
btn2.setText("This is Button TWO");
btn2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
System.out.println("Button Two pushed");
txt1.setText("Button TWO pushed");
}
});
// --------------------------------------------------------------------
gridBox.add(btn1, 0, 0);
gridBox.add(btn2, 1, 0);
vbox1.getChildren().add(gridBox);
vbox1.getChildren().add(txt1);
vbox1.getChildren().add(txt2);
Scene sc = new Scene(vbox1, 400, 250);
primaryStage.setScene(sc);
primaryStage.show();
}
public static void main(String[] args) {
Application.launch(args);
}
}
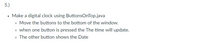

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- JAVA PROGRAMMINGOBJECT-ORIENTED PROGRAMMING Add functionalities to the buttons in the project The_Swing_API_example_2. In other words, make thebuttons react to the user's input.arrow_forwardbelow is my code, need help with this javafx code package com.example.demo1;import javafx.application.Application;import javafx.application.Platform;import javafx.event.ActionEvent;import javafx.event.EventHandler;import javafx.fxml.FXMLLoader;import javafx.geometry.HPos;import javafx.geometry.Insets;import javafx.geometry.Pos;import javafx.scene.Scene;import javafx.scene.control.*;import javafx.scene.layout.GridPane;import javafx.scene.layout.HBox;import javafx.scene.layout.VBox;import javafx.scene.paint.Color;import javafx.scene.text.Font;import javafx.scene.text.FontWeight;import javafx.stage.Stage;import java.io.IOException;import java.lang.reflect.Field;import java.util.Objects;import java.util.Optional;public class HelloApplication extends Application {public boolean inputValidation(String s){try {int a = Integer.parseInt(s);return true;}catch (Exception e ){return false;}}@Overridepublic void start(Stage stage) throws IOException {GridPane grid= new…arrow_forwardQuestion & instructions can be found in images please see images first Starter code import javax.swing.*;import java.awt.*;import java.awt.event.*;import java.text.DecimalFormat; public class GPACalculator extends JFrame{private JLabel gradeL, unitsL, emptyL; private JTextField gradeTF, unitsTF; private JButton addB, gpaB, resetB, exitB; private AddButtonHandler abHandler;private GPAButtonHandler cbHandler;private ResetButtonHandler rbHandler;private ExitButtonHandler ebHandler; private static final int WIDTH = 400;private static final int HEIGHT = 150; private static double totalUnits = 0.0; // total units takenprivate static double gradePoints = 0.0; // total grade points from those unitsprivate static double totalGPA = 0.0; // total GPA (gradePoints / totalUnits) //Constructorpublic GPACalculator(){//Create labelsgradeL = new JLabel("Enter your grade: ", SwingConstants.RIGHT);unitsL = new JLabel("Enter number of units: ", SwingConstants.RIGHT);emptyL = new…arrow_forward
- What is interesting and difficult about GUI concepts in Java? For instance event handlers, layout managers, frames, panels. polymorphism, JavaFX.arrow_forwardPlease check if there is any issue with my code and please upload a screenshot of result when it is working. Please add proper comments as well. // need for using JavaFx import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.geometry.*; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.geometry.Insets; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.layout.GridPane; import javafx.stage.Stage; public class Main extends Application { atOverride public void start(Stage primaryStage) throws Exception { primaryStage.setTitle("Tip Calculator"); //setting the title GridPane rootNode = new GridPane(); rootNode.setPadding(new Insets(15)); Scene myScene = new…arrow_forwardWrite a JavaFX application that displays the side view of a spaceship that follows the movement of the mouse. When the mousebutton is pressed down, have a laser beam shoot out of the frontof the ship (one continuous beam, not a moving projectile) untilthe mouse button is released. Define the spaceship using a separate classarrow_forward
- Create a class RectangleExample in eclipse. Copy the following code into the class. RectangleExample.java package mypackage; import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.stage.Stage; import javafx.scene.shape.Rectangle; public class RectangleExample extends Application { Rectangle rect1, rect2; @Override public void start(Stage stage) { Group root = new Group(); //Drawing a Rectangle rect1 = new Rectangle(); //Setting the properties of the rectangle rect1.setX(20.0f); rect1.setY(20.0f); rect1.setWidth(300.0f); rect1.setHeight(10.0f); rect1.setFill(javafx.scene.paint.Color.RED); //Add to a Group object root.getChildren().add(rect1); //Drawing a Rectangle rect2 = new Rectangle(); //Setting the properties of the rectangle rect2.setX(20.0f); rect2.setY(40.0f); rect2.setWidth(200.0f); rect2.setHeight(10.0f); rect2.setFill(javafx.scene.paint.Color.GREEN); //Add to a Group object root.getChildren().add(rect2); //Creating a scene…arrow_forwardits a JavaFX project Create a working order form for Orinoco as shown. Sales tax is 7%.To display the Total Due (a Label) as currency, research how to use class NumberFormat. package application;import javafx.application.Application;import javafx.event.ActionEvent;import javafx.event.EventHandler;import javafx.geometry.Insets;import javafx.stage.Stage;import javafx.scene.Scene;import javafx.scene.control.Button;import javafx.scene.control.CheckBox;import javafx.scene.control.Label;import javafx.scene.control.RadioButton;import javafx.scene.control.TextField;import javafx.scene.control.ToggleGroup;import javafx.scene.layout.GridPane; public class Main extends Application {TextField itemField,quantityField,priceField;Label totalValue;CheckBox isTaxable;ToggleGroup shipping;@Overridepublic void start(Stage primaryStage) {primaryStage.setTitle("orinoco.com");GridPane rootNode = new GridPane();rootNode.setPadding(new Insets(25));rootNode.setHgap(10);rootNode.setVgap(10);Scene scene = new…arrow_forwardCan you show me where and how to add an image folder (Create a pane to hold the images views) with gif images on this code, to hold image views. code is below import javafx.application.Application;import javafx.scene.Scene;import javafx.scene.image.Image;import javafx.scene.image.ImageView;import javafx.scene.layout.GridPane;import javafx.stage.Stage; public class ex1401 extends Application {@Override public void start(Stage primaryStage) { /** pane to hold four images */GridPane p= new GridPane();p.add(new ImageView(new Image("image/uk.gif")), 0, 0);p.add(new ImageView(new Image("image/ca.gif")), 1, 0);p.add(new ImageView(new Image("image/china.gif")), 0, 1);p.add(new ImageView(new Image("image/us.gif")), 1, 1); Scene s= new Scene(p);primaryStage.setTitle("Exercise_14_01");primaryStage.setScene(s);primaryStage.show(); } }arrow_forward
- Please help me fix the errors in the java program below. There are two class AnimatedBall and BouncingBall import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class AnimatedBall extends JFrame implements ActionListener{private Button btnBounce; // declare a button to playprivate TextField txtSpeed; // declare a text field to enterprivate Label lblDelay;private Panel controls; // generic panel for controlsprivate BouncingBall display; // drawing panel for ballContainer frame;public AnimatedBall (){// Set up the controls on the appletframe = getContentPane();btnBounce = new Button ("Bounce Ball");//create the objectstxtSpeed = new TextField("10000", 10);lblDelay = new Label("Enter Delay");display = new BouncingBall();//create the panel objectscontrols = new Panel();setLayout(new BorderLayout()); // set the frame layoutcontrols.add (btnBounce); // add controls to panelcontrols.add (lblDelay);controls.add…arrow_forwardimport javafx.application.Application; import javafx.geometry.*; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.layout.*; import javafx.stage.Stage; import javafx.event.*; import javafx.scene.text.*; public class SweepstakesJavaFX extends Application { private TextField first = new TextField(); private TextField last = new TextField(); private TextField phone = new TextField(); private TextField email = new TextField(); private TextField luckyNum = new TextField(); private TextField dob = new TextField(); public Label error = new Label(); public Label title = new Label("Sweepstakes Entry Form\nPlease complete the fields below"); public static void main(String[] args) { Application.launch(args); } @Override public void start(Stage primaryStage) { VBox labels = new VBox(); GridPane pane = new GridPane(); pane.setAlignment(Pos.CENTER); labels.setAlignment(Pos.CENTER);…arrow_forwardJavaFX ProgrammingUsing scenebuilder with eclipse or another ide code this IN JAVA 03 - Calculator GUI: Create the GUI for a four function calculator. No functionality is required.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
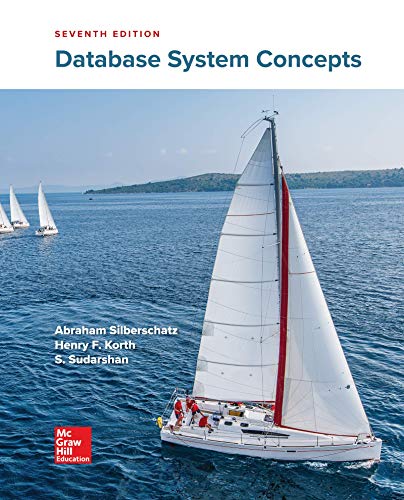
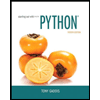
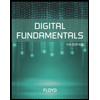
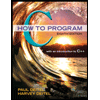
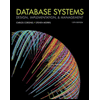
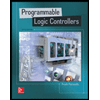