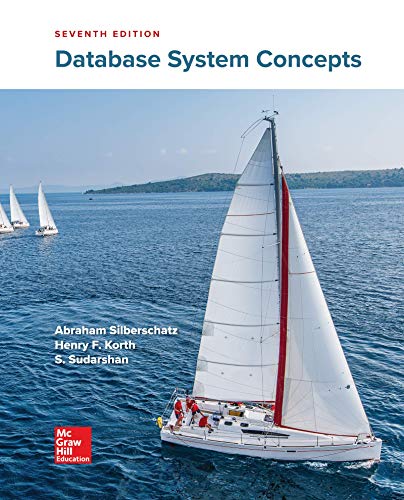
Concept explainers
Java Code: For Lexer.java
Make a HashMap of <String, TokenType> in your Lex class. Below is a list of the keywords that you need. Make token types and populate the hash map in your constructor (I would make a helper method that the constructor calls).
while, if, do, for, break, continue, else, return, BEGIN, END, print, printf, next, in, delete, getline, exit, nextfile, function
Modify “ProcessWord” so that it checks the hash map for known words and makes a token specific to the word with no value if the word is in the hash map, but WORD otherwise.
For example,
Input: for while hello do
Output: FOR WHILE WORD(hello) DO
Make a token type for string literals. In Lex, when you encounter a “, call a new method (I called it HandleStringLiteral() ) that reads up through the matching “ and creates a string literal token ( STRINGLITERAL(hello world) ). Be careful of two things: make sure that an empty string literal ( “” ) works and make sure to deal with escaped “ (String quote = “She said, \”hello there\” and then she left.”;)
Make a new token type, a new method (HandlePattern) and call it from Lex when you encounter a backtick.
The last thing that we need to deal with in our lexer is symbols. Most of these will be familiar from Java, but a few I will detail a bit more. We will be using two different hash maps – one for two-character symbols (like ==, &&, ++) and one for one character symbols (like +, -, $). Why? Well, some two-character symbols start with characters that are also symbols (for example, + and +=). We need to prioritize the += and only match + if it is not a +=.
Create a method called “ProcessSymbol” – it should use PeekString to get 2 characters and look them up in the two-character hash map. If it exists, make the appropriate token and return it. Otherwise, use PeekString to get a 1 character string. Look that up in the one-character hash map. If it exists, create the appropriate token and return it. Don’t forget to update the position in the line. If no symbol is found, return null. Call ProcessSymbol in your lex() method. If it returns a value, add the token to the token list.
Make sure all the functionality of the unit test are tested. Below are lexer.java and token.java. Make sure to show full code with screenshot of the output. Attached is checklist.
Lexer.java

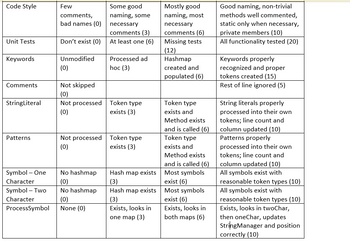

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 1 images

Where are the unit test? I need to see various junit test cases being tested.
Where are the unit test? I need to see various junit test cases being tested.
- Java Code: Create a Parser class. Much like the Lexer, it has a constructor that accepts a LinkedList of Token and creates a TokenManager that is a private member. The next thing that we will build is a helper method – boolean AcceptSeperators(). One thing that is always tricky in parsing languages is that people can put empty lines anywhere they want in their code. Since the parser expects specific tokens in specific places, it happens frequently that we want to say, “there HAS to be a “;” or a new line, but there can be more than one”. That’s what this function does – it accepts any number of separators (newline or semi-colon) and returns true if it finds at least one. Create a Parse method that returns a ProgramNode. While there are more tokens in the TokenManager, it should loop calling two other methods – ParseFunction() and ParseAction(). If neither one is true, it should throw an exception. bool ParseFunction(ProgramNode) bool ParseAction(ProgramNode) -Creates ProgramNode,…arrow_forwardHelp with this java question: Map<KeyType, ValueType> mapName = new HashMap<>();arrow_forwardJava Code: Build the two variable classes that we need: InterpreterDataType and a subclass InterpreterArrayDataType. I will abbreviate these as IDT and IADT. IDT holds a string and has two constructors - one with and one without an initial value supplied. IADT holds a HashMap<String,IDT>. Its constructor should create that hash map. We will use these as our variable storage classes as the interpreter runs. Let's start the interpreter itself. Create a new class (Interpreter). Inside of it, we will have an inner class - LineManager. We will also need a HashMap<String,IDT> that will hold our global variables. We will also have a member HashMap<String, FunctionDefinitionNode> - this will be our source when someone calls a function. The LineManager should take a List<String> as a parameter to the constructor and store it in a member. This will be our read-in input file. It will need a method : SplitAndAssign. This method will get the next line and split it by looking…arrow_forward
- Write a generic class Students.java which has a constructor that takes three parameters – id, name, and type. Type will represent if the student is ‘remote’ or ‘in-person’. A toString() method in this class will display these details for any student. A generic method score() will be part of this class and it will be implemented by inherited classes. Write accessors and mutators for all data points. Write two classes RemoteStudents.java and InPersonStudents.java that inherits from Student class. Show the use of constructor from parent class (mind you, RemoteStudents have one additional parameter – discussion). Implement the abstract method score() of the parent class to calculate the weighted score for both types of students. Write a driver class JavaProgramming.java which has the main method. Create one remote student object and one in-person student object. The output should show prompts to enter individual scores – midterm, finals, ...... etc. and the program will…arrow_forwardBuiltInFunctionDefinitionNode.java has an error so make sure to fix it. BuiltInFunctionDefinitionNode.java import java.util.HashMap; import java.util.function.Function; public class BuiltInFunctionDefinitionNode extends FunctionDefinitionNode { private Function<HashMap<String, InterpreterDataType>, String> execute; private boolean isVariadic; public BuiltInFunctionDefinitionNode(Function<HashMap<String, InterpreterDataType>, String> execute, boolean isVariadic) { this.execute = execute; this.isVariadic = isVariadic; } public String execute(HashMap<String, InterpreterDataType> parameters) { return this.execute.apply(parameters); } }arrow_forwardfor (String name : likedBy) { String likedUser= name.trim(); Set<String> likes = likesMap.getOrDefault(likedUser, new HashSet<>()); Here you create a new Set for likes. This is in the iteration over the likers though so it results in many missing entries. [ How do i Fix this ] import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.HashMap; import java.util.HashSet; import java.util.List; import java.util.Map; import java.util.Set; public class FacebookLikeManager { private Map<String, Set<String>> likesMap; public FacebookLikeManager() { likesMap = new HashMap<>(); } public void buildMap(String filePath) { try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) { String line = reader.readLine(); while (line != null) { String[]…arrow_forward
- Please do not give solution in image format thanku USING JAVA Modify the code to use Arraylist Instead of HashmapCODE:import java.util.ArrayList;import java.util.HashMap;import java.util.List;import java.util.Map;class Student {private String name;private List<String> certificates;public Student(String name) {this.name = name;this.certificates = new ArrayList<>();}public void addCertificate(String certificate) { certificates.add(certificate);}public boolean hasCertificate(String certificate) {return certificates.contains(certificate);}public String getName() {return name;}}public class CertificateManagement {public static void main(String[] args) { Map<String, Student> students = new HashMap<>();Student student1 = new Student("John");student1.addCertificate("Java");student1.addCertificate("Python");students.put("John", student1);Student student2 = new Student("Alice");student2.addCertificate("C++");students.put("Alice", student2);Student student3 = new…arrow_forwardDon't send AI generated answer or plagiarised answer. If I see these things I'll give you multiple downvotes and will report immediately.arrow_forwardIn Java, Create Movie class, which has private fields for title, director, rating. It has a constructor to initialize these fields and a toString method for printing movie details. There is also a getRating method to retrieve the movie rating. Create Rating Comparator class which implements the Comparator interface for comparing movies based on their ratings. Create DemoMovies Class. Initialize necessary data structures like LinkedList, TreeMap for rating as key and Movie from Movie class as value, TreeSet, and ProtiyQueue. Use a loop to take user input for movie detailsuntil the user enters 'WWW' as the movie title.Inside the loop, for each movie, create a Movie object, add it to various data structures that is title, director and rating based on the constructor. Add the Movie object to the LinkedList. Add the rating and the object to the TreeMap.Add the rating to the TreeSet.Add the Movie object to the Priority Queue.Display the entered movies. Sort and display Movies by rate by…arrow_forward
- assume you have a HashMap class that uses a threshold of 0.75 (75%),regardless of the collision resolution mechanism used, and has an initial array size of 13. You may assume the array is resized when the current item to be added will make the load factor greater than or equal to the threshold. Recall that the load factor is the fraction of a hash map that is full. If the array is to be resized, assume the array doubles in size and adds one (2 * size + 1).Table 1 contains a list of items and their associated hash codes that were computed with somehypothetical hash function. Assume the items are added to a newly created instance of the HashMap class in the same order in which they are listed in the table. Based on this information, show what the array of the HashMap would look like after all the items have been added using both of the followinghash collision resolution techniques.1. Separate chaining2. linear probingProvide 2 arrays(one for each problem). One that uses separate…arrow_forwardWrite a method void removeShortStrings(HashSet<String> set). The method should remove from the set any string that is shorter than 100.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
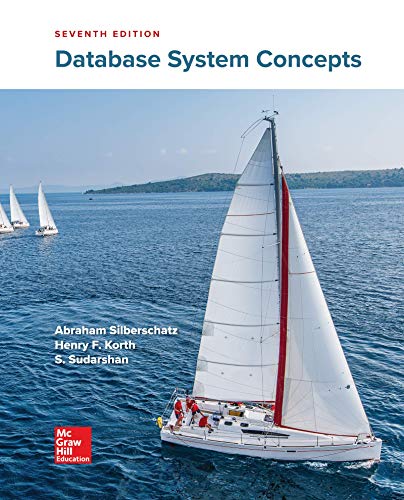
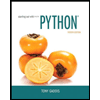
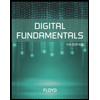
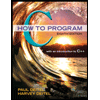
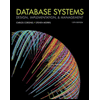
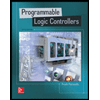