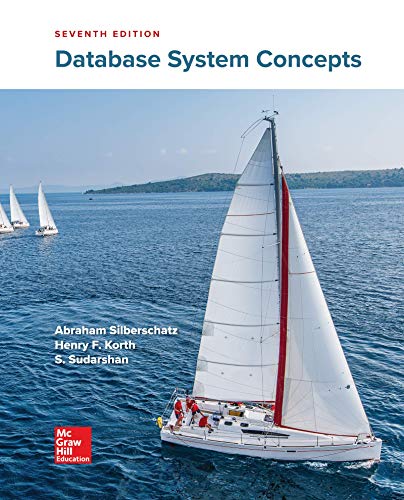
Java Programming: Below is parser.java and attached is circled all the methods that must be implemented in parser.java. Make sure to show a full working code & the screenshot of the output.
Parser.java
package mypack;
import java.util.List;
import java.util.Queue;
public class Parser {
private Queue<Token> tokens;
public Parser(List<Token> tokens) {
this.tokens = (Queue<Token>) tokens;
}
private Token matchAndRemove(Token.TokenType... types) {
if (tokens.isEmpty()) {
return null;
}
Token token = tokens.peek();
for (Token.TokenType type : types) {
if (token.getTokenType() == type) {
tokens.remove();
return token;
}
}
return null;
}
private void expectEndsOfLine() throws SyntaxErrorException {
while (matchAndRemove(Token.TokenType.ENDOFLINE) != null);
if (tokens.isEmpty()) {
throw new SyntaxErrorException();
}
}
private Token peek(int i) {
if (tokens.size() < i) {
return null;
}
return (Token) tokens.toArray()[i-1];
}
public Node parse() throws SyntaxErrorException {
Node result = null;
do {
result = expression();
if (result != null) {
System.out.println(result.toString());
expectEndsOfLine();
}
} while (result != null);
return null;
}
private Node expression() throws SyntaxErrorException {
Node left = term();
if (left == null) {
return null;
}
while (true) {
Token op = matchAndRemove(Token.TokenType.PLUS, Token.TokenType.MINUS);
if (op == null) {
return left;
}
Node right = term();
if (right == null) {
throw new SyntaxErrorException();
}
left = new MathOpNode(op.getTokenType(), left, right);
}
}
private Node term() throws SyntaxErrorException {
Node left = factor();
if (left == null) {
return null;
}
while (true) {
Token op = matchAndRemove(Token.TokenType.TIMES, Token.TokenType.DIVIDE, Token.TokenType.MODULUS);
if (op == null) {
return left;
}
Node right = factor();
if (right == null) {
throw new SyntaxErrorException();
}
left = new MathOpNode(op.getTokenType(), left, right);
}
}
private Node factor() throws SyntaxErrorException {
Token token = matchAndRemove(Token.TokenType.NUMBER, Token.TokenType.MINUS, Token.TokenType.LEFT_PAREN, Token.TokenType.IDENTIFIER);
if (token == null) {
return null;
}
switch (token.getTokenType()) {
case NUMBER:
return new IntegerNode(Integer.parseInt(token.getLexeme()));
case MINUS:
Node inner = factor();
if (inner == null) {
throw new SyntaxErrorException();
}
return new MathOpNode(Token.TokenType.MINUS, new IntegerNode(0), inner);
case LEFT_PAREN:
Node expression = expression();
if (expression == null) {
throw new SyntaxErrorException();
}
if (matchAndRemove(Token.TokenType.RIGHT_PAREN) == null) {
throw new SyntaxErrorException();
}
return expression;
case IDENTIFIER:
VariableReferenceNode varRefNode = new VariableReferenceNode(token.getLexeme());
if (matchAndRemove(Token.TokenType.LEFT_BRACE) != null) {
Node indexExpr = expression();
if (indexExpr == null) {
throw new SyntaxErrorException();
}
varRefNode.setIndex(indexExpr);
if (matchAndRemove(Token.TokenType.RIGHT_BRACE) == null) {
throw new SyntaxErrorException();
}
}
return varRefNode;
default:
return null;
}
}
}
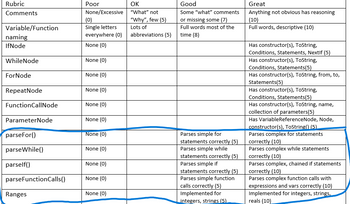

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Im trying to figure out a java homework assignment where I have to evaluate postfix expressions using stacks and Stringtokenizer as well as a isNumber method. when I try to run it it gives me an error. this is the code I have so far: static double evaluatePostfixExpression(String expression){ // Write your code Stack aStack = new Stack(); double value1, value2; String token; StringTokenizer tokenizer = new StringTokenizer(expression); while (tokenizer.hasMoreTokens()) { token = tokenizer.nextToken(); if(isNumber(expression)) { value1 = (double)aStack.pop(); value2 = (double)aStack.pop(); if (token.equals("+")) { aStack.push(value2+value1); } else if (token.equals("-")) { aStack.push(value2-value1); } else if (token.equals("*")) { aStack.push(value2*value1); } else if (token.equals("/")) { aStack.push(value2/value1); } } }…arrow_forwardAdd the three classic operations of Queues in the MagazineList.java to make it become a queue. Add testing code in MagazineRack.java to test your code. (You do not need to modify Magazine.java) Magazine.java : public class Magazine {private String title;//-----------------------------------------------------------------// Sets up the new magazine with its title.//-----------------------------------------------------------------public Magazine(String newTitle){ title = newTitle;}//-----------------------------------------------------------------// Returns this magazine as a string.//-----------------------------------------------------------------public String toString(){return title;}} MagazineList.java : attached the image below MagazineRack.java: public class MagazineRack{//----------------------------------------------------------------// Creates a MagazineList object, adds several magazines to the// list, then prints…arrow_forwardConsider the instance variables and constructors. Given Instance Variables and Constructors: public class SimpleLinkedList<E> implements SimpleList<E>, Iterable<E>, Serializable { // First Node of the List private Node<E> first; // Last Node of the List private Node<E> last; // Number of List Elements private int count; // Total Number of Modifications (Add and Remove Calls) private int modCount; /** * Creates an empty SimpleLinkedList. */ publicSimpleLinkedList(){ first = null; last = null; count = 0; modCount = 0; } ... Assume the class contains the following methods that work correctly: public boolean isEmpty() public int size() public boolean add(E e) public E get(int index) private void validateIndex(int index, int end) Complete the following methods based on the given information from above. /** * Adds an element to the list at the…arrow_forward
- Draw a UML class diagram for the following code: import java.util.*; public class QueueOperationsDemo { public static void main(String[] args) { Queue<String> linkedListQueue = new LinkedList<>(); linkedListQueue.add("Apple"); linkedListQueue.add("Banana"); linkedListQueue.add("Cherry"); System.out.println("Is linkedListQueue empty? " + linkedListQueue.isEmpty()); System.out.println("Front element: " + linkedListQueue.peek()); System.out.println("Removed element: " + linkedListQueue.remove()); System.out.println("Front element after removal: " + linkedListQueue.peek()); Queue<Integer> arrayDequeQueue = new ArrayDeque<>(); arrayDequeQueue.add(10); arrayDequeQueue.add(20); arrayDequeQueue.add(30); System.out.println("Is arrayDequeQueue empty? " + arrayDequeQueue.isEmpty()); System.out.println("Front element: " + arrayDequeQueue.peek());…arrow_forwardChange the __str__ method of the Queue class (provided below) so that it prints each object in the queue along with its order in the queue (see sample output below). class Queue(): def __init__(self): self.queue = [] # implement with Python lists! # start of physical Python list == front of a queue # end of physical Python list == back of a queue def enqueue(self, new_obj): self.queue.append(new_obj); def dequeue(self): return self.queue.pop(0) def peek(self): return self.queue[0] def bad_luck(self): return self.queue[-1] def __str__(self): return str(self.queue) # let's try a more fun waySample output: >>> my_queue = Queue()>>> everyone = ["ESC", "ABC", "YOLO", "HTC"]>>> for initials in everyone:>>> my_queue.enqueue(initials)>>> print(my_queue)Output: 1: ESC2: ABC3: YOLO4: HTCarrow_forwardimport java.util.*;import java.io.*; public class HuffmanCode { private Queue<HuffmanNode> queue; private HuffmanNode overallRoot; public HuffmanCode(int[] frequencies) { queue = new PriorityQueue<HuffmanNode>(); for (int i = 0; i < frequencies.length; i++) { if (frequencies[i] > 0) { HuffmanNode node = new HuffmanNode(frequencies[i]); node.ascii = i; queue.add(node); } } overallRoot = buildTree(); } public HuffmanCode(Scanner input) { overallRoot = new HuffmanNode(-1); while (input.hasNext()) { int asciiValue = Integer.parseInt(input.nextLine()); String code = input.nextLine(); overallRoot =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
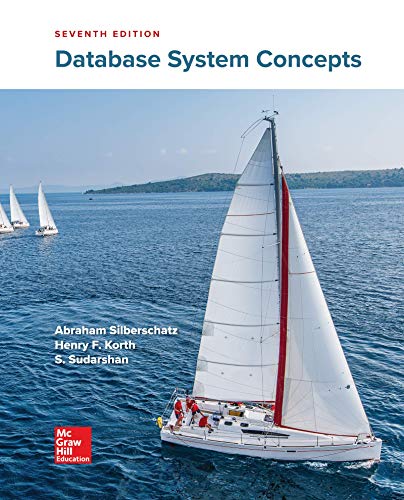
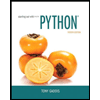
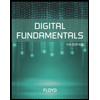
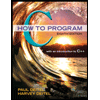
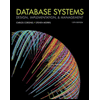
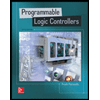