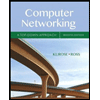
myString. h:
#include <iostream>
using namespace std;
#ifndef _MYSTRING_H_
#define _MYSTRING_H_
class myString
{
private:
char *str;
public:
myString();
myString(const char *s);
void operator = (myString &s);
int length();
friend ostream &operator <<(ostream &out, myString &s);
friend istream &operator >>(istream &out, myString &s);
bool operator <(myString &s);
bool operator <=(myString &s);
bool operator >(myString &s);
bool operator >=(myString &s);
bool operator ==(myString &s);
bool operator !=(myString &s);
};
#endif
myString. cpp:
#include "myString. h"
#include <cstring>
myString::myString()
{
str = new char;
str[0] = '\0';
}
myString::myString(const char *s){
int len = strlen(s);
str = new char[len + 1];
for(int i = 0; i < len; ++i){
str[i] = s[i];
}
str[len] = '\0';
}
void myString::operator = (myString &s){
s.str = new char[length() + 1];
for(int i = 0; i < length(); ++i){
s.str[i] = str[i];
}
s.str[length()] = '\0';
}
int myString::length(){
return strlen(str);
}
ostream &operator <<(ostream &out, myString &s){
out << s.str;
return out;
}
istream &operator >>(istream &in, myString &s)
{
in >> s.str;
return in;
}
bool myString::operator < (myString &s)
{
return strcmp(str, s.str) < 0;
}
bool myString::operator <= (myString &s)
{
return strcmp(str, s.str) <= 0;
}
bool myString::operator > (myString &s)
{
return strcmp(str, s.str) > 0;
}
bool myString::operator >= (myString &s)
{
return strcmp(str, s.str) >= 0;
}
bool myString::operator == (myString &s)
{
return strcmp(str, s.str) == 0;
}
bool myString::operator != (myString &s)
{
return strcmp(str, s.str) != 0;
}
main. cpp:
#include "myString.h"
#include <iostream>
using namespace std;
int main()
{
myString s1, s2;
cout << "Enter first string: ";
cin >> s1;
cout << "Enter second string: ";
cin >> s2;
cout << "you entered: " << s1 << " and " << s2 << endl;
myString s3 = s1;
cout << "Value of s3 is " << s3 << endl;
if(s1 < s2)
{
cout << s1 << " < " << s2 << endl;
}
if(s1 <= s2)
{
cout << s1 << " <= " << s2 << endl;
}
if(s1 > s2)
{
cout << s1 << " > " << s2 << endl;
}
if(s1 >= s2)
{
cout << s1 << " >= " << s2 << endl;
}
if(s1 == s2)
{
cout << s1 << " == " << s2 << endl;
}
if(s1 != s2)
{
cout << s1 << " != " << s2 << endl;
}
return 0;
}
2. A read() function
The read() function will allow the client programmer to specify the delimiting character (the character at which reading will stop). It should work just like the getline() function works for c-strings; that is, it should place everything up to but not including the delimiting character into the calling object, and it should also consume (and discard) the delimiting character. This will be a void function that will take two arguments, a stream and the delimiting character. It should not skip leading spaces. The limit of 127 characters imposed on the >> function above also applies to this function.
Hint: Don't try to read character by character in a loop. Use the in.getline() function to read the input into a non-dynamic array, then use strcpy() to copy it into your data member.

Step by stepSolved in 2 steps

- C++ Find the errors in the code for Date Class and Source.cpp program and fix them. source.cpp include <iostream>#include "Date.h" // include definition of class Date from Date.h using namespace std; // function main begins program executionint main(){Date date(); // create a Date object for May 6, 1981 // display the values of the three Date data members cout << "Month: " << date.getMonth() << endl;cout « "Day: " << date.getDay() << endl;cout << "Year: " << date.getYear(2017) << endl; cout << "\nOriginal date:" << endl;date = displayDate(); // output the Date// modify the Date setMonth(13);setDay(1);setYear(2005); cout "\nNew date:" << endl;date.displayDate(); // output the modified date } // end main Date.h // class Data definition#include <iostream>using namespace std; //Data constructor that initializes the three data members;class Date{publicdate(m, d, y){setMonth();setDay();setYear();};// set monthvoid…arrow_forward#include <iostream> #include <cmath> #include <iomanip> using namespace std; int calc_qty(int qty); void receipt( int qty, int qty2); int calc_qty(int qty); void coffee_size(int qty, int size, int choice, int qty2); void slices(); void beanounces(); int main() { int qty = 0, order = 0, size = 0, qty2 = 0; receipt (qty,qty2); calc_qty (qty); do { //displays the menu cout<<" Please select an item on the menu you would like to buy."<<endl; cout<<"Enter 1 for Coffee\n"<<"Enter 2 for cheese cake\n"<<"Enter 3 for coffee beens\n"<<endl; cin>>choice; } switch (choice) { case 1: coffee_size(qty, size, order, qty2); break; case 2: slices(); break; case 3: beanounces(); break; case 4: cout<<"Enjoy your day"<<endl;…arrow_forwardC++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forward
- #include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forwardHello, I was making a hangman simulation in C++. The code runs, but not fully. Could you identify the error and fix it? #include<iostream>#include<cstring> // for string class functions#include<fstream>#include <cctype>using namespace std; int main(){// define variable to get the response from user "Yes" or "No"string response;// Define index variableint w = 0;// define number of words that need to be guessed by the user assume 4const int WORDS = 4;// loopdo{// we will define the hangman bodyconst char body[] = "o/|\\|\\";// here we define the wordsstring words[WORDS] = {"MACAW", "SADDLE", "TOASTER", "XENOICIDE"};// fetch size or lengthstring xword(words[w].length(),'*');// define iterator to fetch the wordsstring::iterator i, ix = xword.begin();// define number of words to prompt for the userchar letters[26]={"\0"};// Now we define the variables which will be used in the simulationint n =0, xcount = xword.length();bool found = false, solved = false, hung =…arrow_forwardC++ #include <iostream>using namespace std; //creating struct to hold //student name,age and letter gradestruct student{ //data members string name; int age; char grade;};int main(){ //declaring object for the struct student *k = new student; //filling it with data k->name = "Kate"; k->age=24; k->grade='B'; ///then printing student data cout<<"Name:"<<k->name<<endl; cout<<"Age:"<<k->age<<endl; cout<<"Grade:"<<k->grade<<endl; return 0;}arrow_forward
- C:/Users/r1821655/CLionProjects/untitled/sequence.cpp:48:5: error: return type specification for constructor invalidtemplate <class Item>class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const;private:value_type data[CAPACITY];size_type used;size_type current_index;};} 48 | void sequence<Item>::sequence() : used(0), current_index(0) { } | ^~~~ line 47 template<class Item> line 48 void sequence<Item>::sequence() : used(0), current_index(0) { }arrow_forward//client.c#include "csapp.h" int main(int argc, char **argv){ int connfd; rio_t rio; connfd = Open_clientfd(argv[1], argv[2]); Rio_readinitb(&rio, connfd); char buffer[MAXLINE]; printf("Your balance is %s\n", buffer); Close(connfd); exit(0); int choice; float amount; int acc2; char buffer[MAXLINE]; rio_t rio; Rio_readinitb(&rio, connfd); while (1) { printf("\nHello welcome to the Bank Management System\n"); printf("----------------------\n"); printf("1. Check Balance\n"); printf("2. Deposit\n"); printf("3. Withdraw\n"); printf("4. Transfer\n"); printf("5. Quit\n"); printf("Enter your choice: "); Rio_writen(connfd, &choice, sizeof(int)); if (choice == 1) { printf("Your balance is %s\n", buffer); } else if (choice == 2) { printf("Enter amount to deposit: "); Rio_writen(connfd, &amount,…arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forward
- Write in c language please: write a statement that calls the function IncreaseItemQty with parameters computerInfo and addStock. Assign computerInfo with the returned value. #include <stdio.h> typedef struct ProductInfo_struct { char itemName[50]; int itemQty;} ProductInfo; ProductInfo IncreaseItemQty(ProductInfo productToStock, int increaseValue) { productToStock.itemQty = productToStock.itemQty + increaseValue; return productToStock;} int main(void) { ProductInfo computerInfo; int addStock; scanf("%s", computerInfo.itemName); scanf("%d", &computerInfo.itemQty); scanf("%d", &addStock); /* Your code goes here */ printf("Name: %s, stock: %d\n", computerInfo.itemName, computerInfo.itemQty); return 0;} thank you in advancearrow_forward/* Interface File: Movie.h Declaration of Movie Class (Variables - "Data Members" or "Attributes" AND Functions - "Member Functions" or "Methods") */ #ifndef MOVIE_H // Include Guard or Header Guard -If already defined ignore rest of code #define MOVIE_H // Otherwise, define MOVIE_H #include<string> // Note: Not "using namespace std;" or even "using std::string" class Movie { private: std::string title = ""; // Explict scope used --> std::string int year = 0; public: Movie(std::string title = "", int year = 1888); // Declaring a Default Constructor ~Movie(); // A Destructor used for freeing up resources void set_title(std::string title_param); std::string get_title() const; // "const" safeguards class variable changes within function std::string get_title_upper() const; void set_year(int year_param); int get_year() const; }; //…arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> T func(T a) { cout<<a; return a; } template<class U> void func(U a) { cout<<a; } int main(int argc, char const *argv[]) { int a = 5; int b = func(a); return 0; } Give output for this code.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
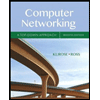
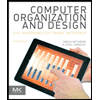
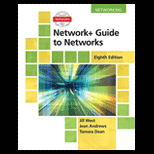
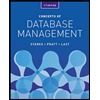
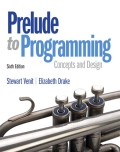
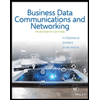