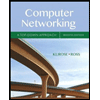
please help with this code.
Code so far:
import java.util.Scanner;
import java.io.File;
Class Authenicator {
private final int SIZE = 100;
private User() users = new User[SIZE];
public Authenticator (String fileName) throws Exception;
Scanner sc = new Scanner(new File(fileName));
int i = 0;
While(sc.hasNext() && i < SIZE) {
users[i] = Users.read(sc);
i++
}
}
public void authenticate(String username, String password) throws Exception{
try {
User u = null;
for(User X : users) {
if(x.getUsername().equals(username) && x.verifyPassword(password){
return ;
TASK
Implement the following class name Authenticator:
State
An array of type User (use a capacity of 100 — I would recommend using a class constant the way I did in the 06-Array class of Lecture 2).
An integer size
Behavior
A constructor accepting a file name, that opens a Scanner on the file and reads in User objects
A method named authenticate that accepts a username and password and attempts to authenticate them against the User array (by doing a search).
Not finding the username in the array causes an exception to be thrown
finding the username, but not matching the password (via verifyPassword) causes an exception with a different message to be thrown (this one with the password hint included).
See below for the exact exception messages expected
The return type of the method is void, i.e., the method returns nothing if the username and password are matched; otherwise an exception is thrown, as described above. (This is a common pattern for authentication methods — if everything is fine, the method simply returns, otherwise it throws an exception.)
The name of your class should be Authenticator. Please remove the public attribute from the class header.
Your class is tested by a AuthenticatorApp class that reads in Users from a file using your read method, loads them into an array and prompts the keyboard for a login sequence (username/password).
For example, if the file users.data contains:
weiss puppy2 woof-woof
arnow java cuppa
sokol brooklyn college
here are some sample excutions of the program:
username? arnow
password? java
Welcome to the system
Sample Test Run #2
Given the same users.data file as above, execution of the program should look like:
username? weiss
password? dontremember
*** Invalid password - hint: woof-woof
username? weiss
password? puppy2
Welcome to the system
Sample Test Run #3
Given the same users.data file as above, execution of the program should look like:
username? sokol
password? CUNY
*** Invalid password - hint: college
username? sokol
password? SUNY
*** Invalid password - hint: college
username? sokol
password? BC
*** Invalid password - hint: college
Too many failed attempts... please try again later

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- edit and finish class authenticate below do not give a solution (example copying from another source and giving it as a solution) that is not part of my code below. Also provided is user class. This is what I have so far please help me finish it. The task is listed below //Authenticate.java import java.util.Scanner;import java.io.File; Class Authenticate {private final int SIZE = 100;private User() users = new User[SIZE];public Authenticator (String fileName) throws Exception;Scanner sc = new Scanner(new File(fileName));int i = 0;While(sc.hasNext() && i < SIZE) {users[i] = Users.read(sc);i++}} public void authenticate(String username, String password) throws Exception{try {User u = null;for(User X : users) {if(x.getUsername().equals(username) && x.verifyPassword(password){ return ; _________________________________________________________________ //User.java import java.io.File;import java.io.FileNotFoundException;import java.util.Scanner; public class User{private…arrow_forwardCode completion and explain. public class X { public static void main(String [] args) { try { badMethod(); System.out.print("A"); } catch (RuntimeException ex) /* Line 10 */ { System.out.print("B"); } catch (Exception ex1) { System.out.print("C"); } finally { System.out.print("D"); } System.out.print("E"); } public static void badMethod() { throw new RuntimeException(); } }.arrow_forwardJAVA CODE: check outputarrow_forward
- java programming I have a Java program with a restaurant/store menu. Can you please edit my program when displaying the physical receipt? I would like the physical receipt to export as text file, not print the console's order receipt. import java.util.*; public class Restaurant2 { publicstaticvoidmain(String[] args) { // Define menu items and pricesString[] menuItems= {"Apple", "Orange", "Pear", "Banana", "Kiwi", "Strawberry", "Grape", "Watermelon", "Cantaloupe", "Mango"};double[] menuPrices= {1.99, 2.99, 3.99, 4.99, 5.99, 6.99, 7.99, 8.99, 9.99, 10.99};StringusersName; // The user's name, as entered by the user.ArrayList<String> arr = new ArrayList<>(); // Define scanner objectScanner input=new Scanner(System.in); // Welcome messageSystem.out.println("Welcome to AppleStoreRecreation, what is your name:");usersName = input.nextLine(); System.out.println("Hello, "+ usersName +", my name is Patrick nice to meet you!, May I Take Your Order?"); // Display menu items and…arrow_forwardI have the following code: import java.util.*; import java.io.*; public class GradeBook { publicstaticvoidmain(String[] args)throwsIOException{ // TODO Auto-generated method stub File infile =newFile("students.dat"); Scanner in =newScanner(infile); while(in.hasNext()){ // Read information form file and create a student object and print String name = in.nextLine(); Student student =newStudent(name, in.nextLine()); for(int i =1; i <=4;++i){ student.setQuiz(i, in.nextInt()); } student.setMidtrmExm(in.nextInt()); student.setFinalExm(in.nextInt()); in.nextLine(); // Calculate grade and letter grade; double overallQuizScore=0.0,score=0.0; for(int i=1;i<=student.NUM_QUIZZES;i++) { overallQuizScore+=(student.getQuiz(i)/student.QUIZ_MAX_POINTS)*100; } overallQuizScore = (overallQuizScore/4)*0.30; score =…arrow_forwardPlease help me using java. I am not understanding why I am getting errorsarrow_forward
- Solution question number 2arrow_forwardusing System; class main { publicstaticvoid Main(string[] args) { Random r = new Random(); int randNo= r.Next(1,11); Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? "); int guess = Convert.ToInt32(Console.ReadLine()); if (guess == randNo) { Console.WriteLine("You guessed correctly!"); } else { Console.WriteLine("I was thinking of the number "+randNo+"\nSorry! Play again."); } } } this is a guessing game program for a random number between 1-10 in C#. how can code be added to tell you if your guess is too high or too low from the chosen random number?arrow_forwardIntegers numberOfParts, required Groups, and invalid Groups are read from input. If numberOfParts is 21 or more, then add 3 to requiredGroups. Otherwise, add 6 to invalidGroups. ► Click here for examples 3 public class GroupSurvey { 4 public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int numberOfParts; int requiredGroups; I int invalidGroups; 345679 8 9 10 11 12 13 14 15 16 17 18 19 20 } numberOfParts = scnr.nextInt (); requiredGroups = scnr.nextInt (); invalidGroups = scnr.nextInt (); /* Your code goes here */ if(numberOfParts>21){ requiredGroups= System.out.println(requiredGroups); System.out.println(invalidGroups);arrow_forward
- Consider the following code: Will the dataMembers class be utilized during the following creation of a file and its records? public class ExampleArea { public static void main(String cvhfg[]) file f = new file(); f. setRecord(4, new record("Bob's Story", "Bob...")); f.setRecord(2, new record("Reita", "16 years and...")); class dataMember String title; public dataMember (String s) title = s; A class file record[] records= new record[10]; public void setRecord(int index,record r) records[index]=r; class record extends dataMember private String hold; public record(String s,String hold) super (s); this.hold=hold; O No, since records are stored instead of dataMembers O t is optional, but recommended since there is an association between record and dataMember O Only if title needs to be set for the individual records O Yes, since dataMember is the base class of recordarrow_forwardAssign the size of userlnput to stringSize. Ex: if userlnput is "Hello", output is: Size of userInput: 1 import java.util.Scanner; 2 3 public class StringSize { public static void main (String [] args) { Scanner scnr = new Scanner(System.in); String userInput; int stringSize; 4 5 8 9. userInput scnr.nextLine(); %3D 10 11 /* Your solution goes here */ 12 13 System.out.println("Size of userInput: + stringSize); 14 15 return; } 16 17 }arrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
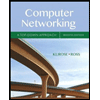
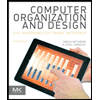
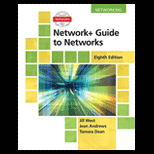
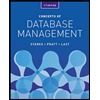
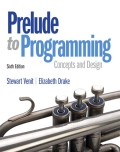
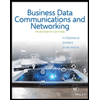