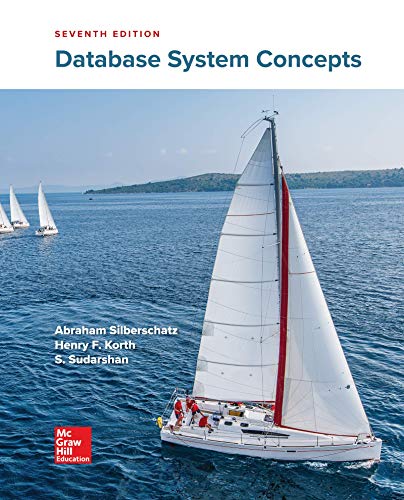
Concept explainers
write a complete member function in class set defined by you in this code to count number of elements in intersection between 2 sets
: the code is ::: using C++ Programming
#include <iostream>
using namespace std;
class Set
{
int n,set[10];
public:
void create ()
{
cout<<"Enter Number Of Elements:";
cin>>n;
int val;
cout<<"Enter Elements in set:"<<endl;
cin>>set[0];
for (int i=1;i<n;i++)
{
cin>>val;
for (int j=0;j<i;j++)
{
if (set[j]==val)
{
i--;
break;
}
else
{
set[i]=val;
}
}
}
cout<<"Set Created"<<endl;
}
void display ()
{
for (int i=0;i<n;i++)
{
cout<<set[i]<<" ";
}
cout<<endl;
}
void setUnion (Set *s2)
{
Set s3;
int x=0,y=0,z=0;
while (x<n && y<s2->n)
{
if (set[x]<(*s2).set[y])
{
s3.set[z]=set[x];
x++;
z++;
}
else if (set[x]==(*s2).set[y])
{
s3.set[z]=set[x];
x++;
y++;
z++;
}
else
{
s3.set[z]=(*s2).set[y];
y++;
z++;
}
}
while (x<n)
{
s3.set[z]=set[x];
x++;
z++;
}
while (y<(*s2).n)
{
s3.set[z]=(*s2).set[y];
y++;
z++;
}
s3.n=z;
s3.display ();
}
void setIntersection (Set *s2)
{
Set s3;
int x=0,y=0,z=0;
while (x<n && y<(*s2).n)
{
if (set[x]<(*s2).set[y])
{
x++;
}
else if (set[x]==(*s2).set[y])
{
s3.set[z]=set[x];
x++;
y++;
z++;
}
else
{
y++;
}
}
s3.n=z;
s3.display ();
}
};
int main ()
{
int Choice;
char answer='y';
do
{
cout<<"-_- This Program Just For Two Sets -_-"<<endl;
cout<<"\n";
cout<<"\t -Menu- \t"<<endl;
cout<<"1.Create"<<endl;
cout<<"2.Print"<<endl;
cout<<"3.Union"<<endl;
cout<<"4.Intersection"<<endl;
cout<<"5.Exit"<<endl;
cout<<"Enter Your Choice:"<<endl;
cin>>Choice;
system("CLS");
switch (Choice)
{
Set s1,s2;
case 1:
cout<<"Set 1:"<<endl;
s1.create ();
cout<<"Set 2:"<<endl;
s2.create ();
break;
case 2:
cout<<"Set 1:"<<endl;
s1.display ();
cout<<"Set 2:"<<endl;
s2.display ();
break;
case 3:
cout<<"Set 1 Union Set 2:"<<endl;
s1.setUnion (&s2);
break;
case 4:
cout<<"Set 1 Intersection Set 2:"<<endl;
s1.setIntersection (&s2);
break;
case 5:
exit (0);
break;
default:
cout<<"Wrong Choice"<<endl;
}
cout<<"Do You Want To Continue (y/n):"<<endl;
cin>>answer;
system("CLS");
}
while (answer=='y');
return 0;
}

Step by stepSolved in 2 steps with 1 images

- C++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forwardWhich of the following statements is NOT correct? Group of answer choices C++ language provides a set of C++ class templates to implement common data structures, which is known as Standard Template Library (STL); In STL, a map is used to store a collection of entries that consists of keys and their values. Keys must be unique, and values need not be unique; In STL, a set is used to store a collection of elements and it does not allow duplicates; Both set and map class templates in STL are implemented with arrays.arrow_forward1. Write a function invert_dict to reverse a dictionary, for example: >>> eng2sp = {'one': 'uno', 'two': 'dos', 'three': 'tres'} >>> sp2eng = invert_dict(eng2sp) >>> sp2eng {'uno':'one', 'dos':'two', 'tres':'three'} 2. Write a function print_dict to print all the key-values in a dictionary, for example: >>> d2 {'a': 'one', 'b': 'two', 'c': 'Three'} >>> print_dict(d2) a:one b:two c:Threearrow_forward
- please help me with codingarrow_forwardComplete the C++ code below#include "std_lib_facilities.h"/*1. Define a function which calculates the outer product of two vectors. The function return is a matrix. */vector<vector<int>> outerProduct(vector<int>& A, vector<int>& B){//implement here}/*2. Define a function which transposes a matrix. */vector<vector<int>> transpose(vector<vector<int>>& A){//implementation}/*3. Define a function which calculates the multiplication of a matrix and it's transpose. */vector<vector<int>> product(vector<vector<int>>& A){// implementation}/*4. Define a print out function that will print out a matrix in the following format:Example: a 3 by 4 matrix should have the print out:1 2 3 42 3 4 53 4 5 6*/void printMatrix(vector<vector<int>>& A){// implementation}int main(){// Define both vector A and vector Bvector<int> A = {1 ,2, 3, 4, 5};vector<int> B = {1, 2, 3};/*Test outerProduct…arrow_forwardC++ Please help!arrow_forward
- Write a value-returning function that receives an array of structs of the type defined below and the length of the array as parameters. It should return as the value of the function the sum of the offspring amounts in the array. Note: you do not need a main function or to create an array. Assume the array already exists from main. #include <iostream>using namespace std; // struct declaration for Animal struct Animal { // a string for animal typestring animal_type;// a string for color string color;// an integer for number of offspring. int number_of_offspring;}; // Main function to declare a structure variable of type Animal. int main(){struct Animal A1;return 0;}arrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
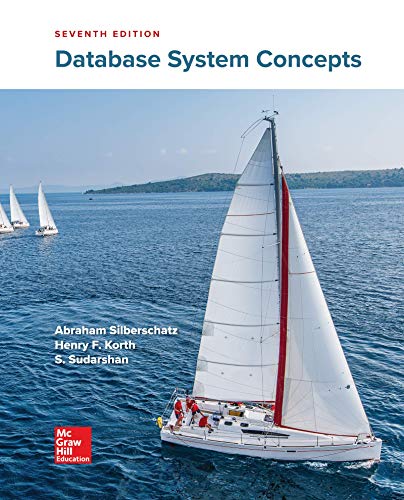
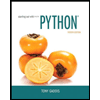
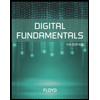
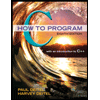
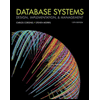
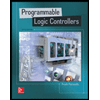