write a program to implement the above interface using Lambda Expression to accept “ Hello World” and print it 10 times. write a program to implement the above interface using Inner Class to accept “ Hello World” and print it 10 times
write a
write a program to implement the above interface using Inner Class to accept “ Hello World” and print it 10 times
This is my code
interface interf1 {
publicvoidrepeat(Strings1);
}
public class Main {
public static void main(String[] args) {
interf1 obj = (s1)-> { //using lambda expression
for(int i=0;i<10;i++) {
System.out.println(s1);
}
};
obj.repeat("Hello World");
}
}
public class Assignment {
public static void main(String[] args) {
interf1 obj = new interf1() { //inner class
public void repeat(String s1){
for(int i=0;i<10;i++){
System.out.println(s1);
}
}
};
obj.repeat("Hello World"); //function call
}
}
But i keep getting this error
Exception in thread "main" java.lang.NoClassDefFoundError: interf1at Main.main(Main.java:17)Caused by: java.lang.ClassNotFoundException: interf1at java.base/jdk.internal.loader.BuiltinClassLoader.loadClass(BuiltinClassLoader.java:641)at java.base/jdk.internal.loader.ClassLoaders$AppClassLoader.loadClass(ClassLoaders.java:188)at java.base/java.lang.ClassLoader.loadClass(ClassLoader.java:521)... 1 more

Step by step
Solved in 3 steps with 1 images

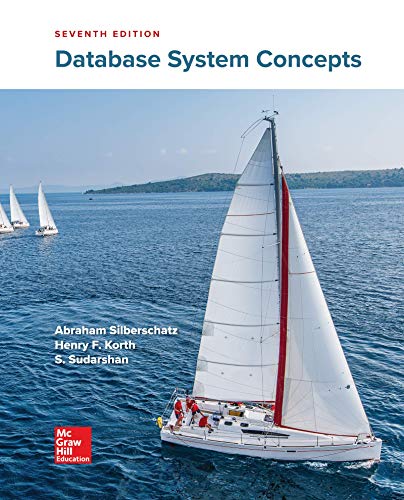
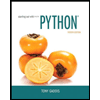
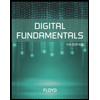
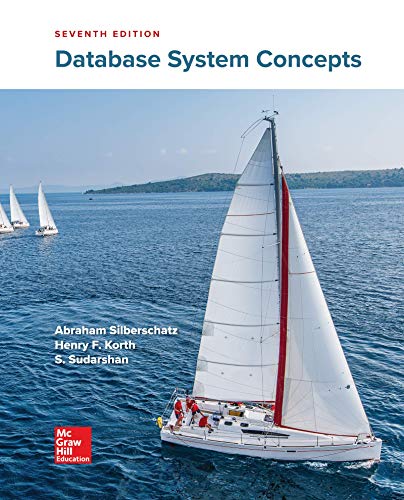
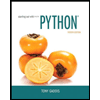
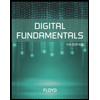
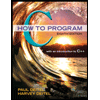
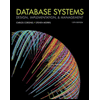
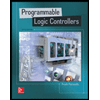