import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> girlsNames = loadNames("GirlNames.txt");
List<String> boysNames = loadNames("BoyNames.txt");
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Enter a name (or 'QUIT' to exit): ");
String input = scanner.nextLine();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
searchAndDisplay(input, girlsNames, boysNames);
}
scanner.close();
}
private static List<String> loadNames(String filename) {
List<String> names = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = reader.readLine()) != null) {
names.add(line.trim());
}
} catch (IOException e) {
System.out.println(filename + " is missing. Exiting...");
System.exit(1); // Exit the program if a file is missing
}
return names;
}
private static void searchAndDisplay(String name, List<String> girlsNames, List<String> boysNames) {
int girlIndex = searchName(name, girlsNames);
int boyIndex = searchName(name, boysNames);
String capitalizedName = name.substring(0, 1).toUpperCase() + name.substring(1).toLowerCase();
if (girlIndex == -1 && boyIndex == -1) {
System.out.println("The name '" + capitalizedName + "' was not found in either list.");
} else if (girlIndex != -1 && boyIndex == -1) {
System.out.println("The name '" + capitalizedName + "' was found in popular girl names list (line " + (girlIndex + 1) + ").");
} else if (girlIndex == -1 && boyIndex != -1) {
System.out.println("The name '" + capitalizedName + "' was found in popular boy names list (line " + (boyIndex + 1) + ").");
} else {
System.out.println("The name '" + capitalizedName + "' was found in both lists: boy names (line " + (boyIndex + 1) + ") and girl names (line " + (girlIndex + 1) + ").");
}
}
private static int searchName(String name, List<String> namesList) {
for (int i = 0; i < namesList.size(); i++) {
if (namesList.get(i).equalsIgnoreCase(name)) {
return i;
}
}
return -1;
}
}
System.out.print("Enter a name (or 'QUIT' to exit): "); to this: Enter a name to search or type QUIT to exit and remove this from the program: System.out.println(filename + " is missing. Exiting...");
THE TEXT FILES ARE LOCATED IN HYPERGRADE I provided them and the failed test case as a screenshot. Thank you.
Test Case 1
Enter a name to search or type QUIT to exit:\n
AnnabelleENTER
The name 'Annabelle' was not found in either list.\n
Enter a name to search or type QUIT to exit:\n
xavierENTER
The name 'Xavier' was found in popular boy names list (line 81).\n
Enter a name to search or type QUIT to exit:\n
AMANDAENTER
The name 'Amanda' was found in popular girl names list (line 63).\n
Enter a name to search or type QUIT to exit:\n
jOrdAnENTER
The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n
Enter a name to search or type QUIT to exit:\n
quitENTER
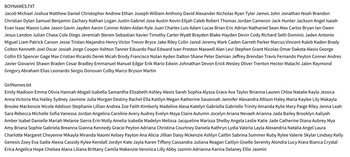
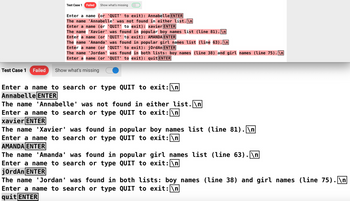

Step by stepSolved in 4 steps with 2 images

- JAVA PROGRAM MODIFY THIS PROGRAM SO IT READS THE TEXT FILES IN HYPERGRADE. I HAVE PROVIDED THE INPUTS AND THE FAILED TEST CASE AS A SCREENSHOT. HERE IS THE WORKING CODE TO MODIFY: import java.io.*;import java.util.*;public class NameSearcher { private static List<String> loadFileToList(String filename) throws FileNotFoundException { List<String> namesList = new ArrayList<>(); File file = new File(filename); if (!file.exists()) { throw new FileNotFoundException(filename); } try (Scanner scanner = new Scanner(file)) { while (scanner.hasNextLine()) { String line = scanner.nextLine().trim(); String[] names = line.split("\\s+"); for (String name : names) { namesList.add(name.toLowerCase()); } } } return namesList; } private static Integer searchNameInList(String name, List<String> namesList) {…arrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardWrite all the code within the main method in the Test Truck class below. Implement the following functionality. a) Constructs two truck objects: one with any make and model you choose and the second object with gas tank capacity 10. b) If an exception occurs, print the stack trace. c) Prints both truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main ( String [] args ) { heres the truck class information A Truck can be described as having a make (string), model (string), gas tank capacity (double), and whether it has a manual transmission (or not). Include the following methods in your class definition. . An overloaded constructor which takes the make and model. This method throws an IllegalArgumentException if the make is "Jeep". An overloaded constructor which takes the gas tank capacity. This method throws an IllegalArgumentException if the capacity of the gas…arrow_forward
- DO NOT COPY FROM OTHER WEBSITES COrrect and detailed answer will be Upvoted else downvoted. Thank you!arrow_forwardIN JAVA PLEASE, need help finding an element in the list /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ public int indexOf(int value) { //Implement this method return -1; }arrow_forwardStarter code for ShoppingList.java import java.util.*;import java.util.LinkedList; public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }} class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}arrow_forward
- Run the program to tell me there is an error, BSTChecker.java:3: error: duplicate class: Node class Node { ^ BSTChecker.java:63: error: class LabProgram is public, should be declared in a file named LabProgram.java public class LabProgram { ^ 2 errors This is my program, please fix it. import java.util.*; class Node { int key; Node left, right; public Node(int key) { this.key = key; left = right = null; } } class BSTChecker { public static Node checkBSTValidity(Node root) { return checkBSTValidityHelper(root, null, null); } private static Node checkBSTValidityHelper(Node node, Integer min, Integer max) { if (node == null) { return null; } if ((min != null && node.key <= min) || (max != null && node.key >= max)) { return node; } Node leftResult = checkBSTValidityHelper(node.left, min, node.key); if (leftResult != null) { return leftResult; } Node rightResult = checkBSTValidityHelper(node.right, node.key, max); if (rightResult != null) { return rightResult; }…arrow_forwardimport java.util.Scanner; public class Playlist { // TODO: Write method to ouptut list of songs public static void main (String[] args) { Scanner scnr = new Scanner(System.in); SongNode headNode; SongNode currNode; SongNode lastNode; String songTitle; int songLength; String songArtist; // Front of nodes list headNode = new SongNode(); lastNode = headNode; // Read user input until -1 entered songTitle = scnr.nextLine(); while (!songTitle.equals("-1")) { songLength = scnr.nextInt(); scnr.nextLine(); songArtist = scnr.nextLine(); currNode = new SongNode(songTitle, songLength, songArtist); lastNode.insertAfter(currNode); lastNode = currNode; songTitle = scnr.nextLine(); } // Print linked list…arrow_forward