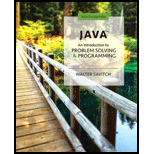
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 11PP
Program Plan Intro
“LinkedQueue” class
Program Plan:
Filename: “LinkedQueue.java”
- Define “LinkedQueue” class.
- Declare variable for reference of first node and number of items.
- Default constructor for “LinkedQueue” class.
- Set front to “null” and count to “0”.
- Define the method “addToQueue()” with argument of “item”.
- If “front” is not equal to “null”, then
- Create a new node using “QueueNode” class.
- Create a reference to front.
- Performs “while” loop. This loop will repeat to obtain last node.
- Compute the value of next node by calling “getNextNode()” method.
- Set the new node at last of the queue by calling “setNextNode()” method.
-
- If the queue is empty, then
- Assign the next node to “null” by using “QueueNode” class.
- Increment the number of items.
- If the queue is empty, then
-
- If “front” is not equal to “null”, then
- Define the method “removeFromQueue()”.
- If the queue is not empty, then get the element at front node by calling “getQueueElement()” method and eliminate the front node.
- Finally returns the resultant element.
-
- If the queue is empty, then returns null.
-
- Finally returns the resultant element.
- If the queue is not empty, then get the element at front node by calling “getQueueElement()” method and eliminate the front node.
- Define the method “isEmpty()”.
- This method returns “true” if the value of “count” is equal to “0”. Otherwise, returns “false”.
- Define the method “displayQueueValues()”.
- Create a reference to front.
- Performs “while” loop. This loop will repeat to display all elements in queue.
- Display the element of the queue.
- Go to the next node by using “getNextNode()” method.
Filename: “QueueNode.java”
- Define “QueueNode” class.
- Declare required variables for queue elements and next node value.
- Create default constructor for “QueueNode” class.
- Assign “null” values for queue elements and next node.
- Create the parameterized constructor for “QueueNode” class.
- Define the method “setNextNode()” which is used to set value for next node.
- Define the method “getNextNode()” which is used to returns the next node value.
- Define the method “setQueueElement()” which is used to assign value for queue elements.
- Define the method “getQueueElement()” which is used to returns queue elements.
Filename: “LinkedQueueTest.java”
- Define “LinkedQueueTest” class.
- Define main function.
- Create an object “nameQueue” for “LinkedQueue” class.
- Add names to object “nameQueue” by using “addToQueue()” method.
- Call the method “displayQueueValues()” to display the elements in queue.
- Remove an element from the queue by calling the method “removeFromQueue()”.
- Display the result of whether the queue is empty or not by calling the method “isEmpty()”.
- Display the elements in queue after removing an element from queue by calling the method “displayQueueValues()”.
- Remove the elements from the queue by calling the method “removeFromQueue()”.
- Display the result of whether the queue is empty or not by calling the method “isEmpty()”.
- Define main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Python For this assignment, you will write a program to allow the user to store the names and phone numbers of their contacts. Create the following classes:
Contact: two attributes. One for the contact's name and the contact's phone number.
Node: two attributes: _data and _next. For use in a linked list.
ContactList: One attribute: _head, a reference to the head of an internal linked list of Node objects.
Note: self._head is an attribute of the class, so any method which updates the internal linked list can simply update the list self._head references. There is no need to return anything like we did in methods such as add_to_end in the lecture. Note also that for the same reason, we do not have to pass a reference to the head of the list to any method, because each method should already have access to the self._head attribute.
ContactList should also have the following methods:
add(name, new_number). Creates a new Node with a Contact object as its _data. The new Node is then added…
You may find a doubly-linked list implementation below. Our first class is Node which we can make a new node with a given element. Its constructor also includes previous node reference prev and next node reference next.
We have another class called DoublyLinkedList which has start_node attribute in its constructor as well as the methods such as:
1. insert_to_empty_list() 2. insert_to_end() 3. insert_at_index()
Hints: Make a node object for the new element. Check if the index >= 0.If index is 0, make new node as head; else, make a temp node and iterate to the node previous to the index.If the previous node is not null, adjust the prev and next references. Print a message when the previous node is null.
4. delete_at_start()
5. delete_at_end() .
6. display()
the task is to implement these 3 methods: insert_at_index(), delete_at_end(), display().
hint on how to start thee code
# Initialize the Node
class Node:
def __init__(self, data):
self.item = data…
Programming in Java.
What would the difference be in the node classes for a singly linked list, doubly linked list, and a circular linked list? I attached the node classes I have for single and double, but I feel like I do not change enough? Also, I use identical classes for singular and circular node which does not feel right. Any help would be appreciated.
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12.5 - What is the purpose of the FXML file?Ch. 12.5 - Prob. 32STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- Given the following definitions that we have used in class to represent a doubly-linked, circular List with a dummy-header node that contains data of type "struct Student". typedef struct { char *name; char *major; } Student;typedef struct node { Student *data; struct node *next; struct node *prev; } Node;typedef struct { Node *header; int size; } LinkedList;LinkedList *roster; Here is a picture of what the Linked List might look like, although you should answer the following question for a general list (ie, that does not necessarily contain 3 data items). What TYPE of data is the following: *roster Group of answer choices int int * Node Node * Student Student * char char * This is legal code, but it does not match any of the types listed above. This is illegal code, and would produce a syntax error No hand written solution and no imagearrow_forwardGiven the following definitions that we have used in class to represent a doubly-linked, circular List with a dummy-header node that contains data of type "struct Student". typedef struct { char *name; char *major; } Student; typedef struct node { Student *data; struct node *next; struct node *prev; } Node; typedef struct { Node *header; int size; } LinkedList; LinkedList *roster; Here is a picture of what the Linked List might look like, although you should answer the following question for a general list (ie, that does not necessarily contain 3 data items). What TYPE of data is the following: roster->header->data->next->next Group of answer choices int int * Node Node * Student Student * char char * This is legal code, but it does not match any of the types listed above. This is illegal code, and would produce a syntax error.arrow_forwardThere’s a somewhat imperfect analogy between a linked list and a railroad train, where individual cars represent links. Imagine how you would carry out various linked list operations, such as those implemented by the member functions insertFirst(), removeFirst(), and remove(int key) from the LinkList class in this hour. Also implement an insertAfter() function. You’ll need some sidings and switches. You can use a model train set if you have one. Otherwise, try drawing tracks on a piece of paper and using business cards for train cars.arrow_forward
- In Java, Question 15: Answer the following questions You are asked to Implement an ADT for MyQueue. The following is a class definition of a linked list Node: class Node { String content; Node next; } The following is a class definition of a linked list MyQueue: class MyQueue { Node head; } Implement the following methods for your class MyQueue. a) Constructor that does not requre any parameters b) Constructor that accepts a parameter of type MyQueue and creates a new instance of MyQueue that is a clone of the one passed as parameter c) public int AppendCopy method that accepts a single parameter of type MyQueue and clones all elements from the MyQueue instance passed as a parameter and appends them to the instance on which we called the method. Return the number of elements that are cloned and appended d) public int FindElements(String filter) - finds all elements that match the filter and returns their count e) overload the FindElementsmethod to include a…arrow_forwardThis assignment will require you to implement a generic interface (Queue<T>) with an array based queue implementation class QueueArray<T>. floating front design. Once you have implemented the class, the test program will run a client test program on the enqueue, dequeue, display and full methods. The test program will create queues of Integers as well as String objects. Enjoying the power of your generic implementation both will work with no additional changes. There are TODO based comments in the implementation file. Create a project and demonstrate that you can successfully create the required output file, shown below. Thr file includes: Queue.java package queueHw; public interface Queue <T> { public boolean empty(); public boolean full(); public boolean enqueue(T data); public T dequeue(); public void display(); } ... TestQueue.java package queueHw; public class TestQueue { public static void main(String[] args) { //TEST QueueArray with…arrow_forwardFor this assignment, you will be programming an airport simulator. The airport has one runway, and a plane can either be taking off or landing on it at any given unit of time. There will be a queue of planes waiting to take off and a priority queue of planes waiting to land. Your simulator will share some similarities to the movie theater simulator from the lecture notes (and the code is posted on Canvas). To make this project much simpler, I recommend studying that code and basing your code off of it. When the user starts the simulation, let them decide the % chance that a plane will show up to land and the % chance that a plane will show up to take off each clock tick. Let them also decide how many clock ticks to run the simulation for. Recall that the simulation should run inside a for loop. Each loop iteration represents one unit of time (or clock tick). In each clock tick, you need to: Randomly decide if a plane should join the take-off queue, based on the percentage provided by…arrow_forward
- Create a class Queue and a Main class. This queue will be implemented using the LinkedList class that has been provided. This queue will hold values of a generic type (<T>). Your Queue should have the following public methods: public void enqueue(T data) public T dequeue() public T peek() public int size() public boolean isEmpty() public class LinkedList<T> { private Node<T> head; private Node<T> tail; private int size; @Override public boolean isEmpty() { return size == 0; } @Override public void append(T data) { Node<T> node = new Node<>(data); if (tail == null) { head = tail = node; } else { tail.next = node; node.prev = tail; tail = node; } size++; } @Override public void prepend(T data) { Node<T> node = new Node<>(data); if (head == null) { head = tail = node; } else {…arrow_forwardWrite a BlueJ project consisting in a class named Lecture whose goal is to store a list of the student names (of type String) attending it. In addition to declaring and initializing the necessary fields, the class has to implement: • A method boolean addStudent(String name) that adds a name to the (internal) list and returns an appropriate value (see Hint): the returned boolean value must be the same returned by the method add of the “internal list”. • A method int getHomonymNumber(String name) that returns the number of students that have the indicated name. • A method void printCSList() that prints the list of student names, in one single line and separated by a comma. For example: Goofy, Donald, Mickey Notice that you may not print a comma after the last name! • A method boolean swap(int index1, int index2) that swaps 2 students names in the list and returns true if the operation is successful. • A method void testIt() that adds at least 4 students (at least two with the same name),…arrow_forwardImplement PokerValue as specified in textbook page 417, problem 33(a). Your implementation must include following: 1. At least one ADT type selected from textbook files such as Stack, Queue, Collection and List for storing the poker hand. 2. Use only CardDeck.java to hold deck of cards, and Card.java to represent each card. GUI is not required. 3. Default constructor to create a five-card poker hand from CardDeck.java. Make sure the poker hand is implemented with one of the textbook ADT as set forth above. 4. Overloaded constructor accepts an array of five cards to initialize one poker hand. 5. toString() method outputs in the same format as shown in SampleActualOutput.txt 6. You may add other methods as necessary. 7. Test your PokerValue.java with supplied PokerGame.java. Submit PokerValue.javaarrow_forward
- The two classes you will create will implement the operations defined in the interface as shown in the UML class diagram above. In addition, BinarySearchArray will implement a static method testBinarySearchArray() that populates the lists, and lets the user interactively test the two classes by adding and removing elements. The parameter BinarySearch can represent either class and tells testBinarySearchArray which class to test. Steps to Implement: 1) To get started create a new project in IntelliJ called BinarySearch. Add a class to your project called BinarySearchArray. Then add another class BinarySearchArrayList and an interface called BinarySearch. The interface BinarySearch includes public method stubs as shown in the diagram. You are allowed to add BinarySearchArrayList to the same file as BinarySearch but don't add an access modifier to this class, or for easier reading, you can declare the classes in separate files with public access modifiers. Only the class…arrow_forwardImplement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this. Use C++! The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to create an empty unsorted list add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. putItem () the numbers in the third line of the data file to the corresponding location in The list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one line…arrow_forwardAssume you have a class called Deck which represents a deck of cards using a singly linked lists. Each node is an object of type Card with a field called next where a reference to the next Card is stored. A field from the Deck class called head stores a reference to the card on the top of the deck, and a field called tail stores a reference to the last card, at the bottom of the deck. We can cut a deck of cards by performing what is called a triple cut: the deck is partitioned into three parts and the bottom part is positioned between the first two. You would like to write a method that implements such a cut on your deck data structure. The method will take as input two cards, the first one being the last card of the first part of the deck, and the second being the first one of the third part of the deck. For example, assume a deck contains cards in the following order AC 2C 3C 4C 5C AD 2D 3D 4D 5D After calling tripleCut() on the deck the card, with input a reference to 3C and a…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
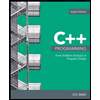
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning