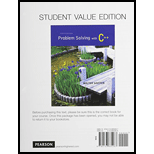
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
9th Edition
ISBN: 9780133862225
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 15, Problem 12PP
Program Plan Intro
Priority Queue
Program Plan:
queue.h:
- Include required header files.
- Create a namespace named “queuesavitch”.
- Create a structure.
- Declare a variable and a pointer.
- Declare a class “Queue”.
- Inside “public” access specifier.
- Declare the constructor and destructor.
- Declare the functions “add ()”, “remove ()”, “empty ()”.
- Inside the “protected” access specifier,
- Create a pointer “front” that points to the head of the linked list.
- Create a pointer “back” that points to the other end of the linked list.
- Inside “public” access specifier.
- Create a structure.
queue.cpp:
- Include required header files.
- Create a namespace named “queuesavitch”.
- Declare the constructor.
- Inside the parameterized constructor,
- Declare the temporary point that moves through the nodes from front to the back.
- Create a pointer “temp_ptr_new” that is used to create new nodes.
- Create new nodes.
- Assign “emp_ptr_old->link” to “temp_ptr_old”.
- Using while condition “temp_ptr_old != NULL”.
- Create a new node.
- Assign the temporary old data to the new pointer.
- Assign NULL to the link of temporary new pointer.
- Assign “temp_ptr_new” to “back->link”.
- Assign “temp_ptr_new” to “back”.
- Assign “temp_ptr_old->link” to “temp_ptr_old”.
- Give definition for the destructor.
- Declare a variable “next”.
- Do till the queue is empty.
- Remove the items using “remove ()” method.
- Give definition for “empty ()” to check if the queue is empty or not.
- Return “back == NULL”.
- Give definition for the method “add ()”.
- Check if the queue is empty.
- Create a new node.
- Assign the item to the data field.
- Make the front node as null.
- Assign front as the back.
- Else,
- Create a new pointer.
- Create a new node.
- Assign the item to “temp_ptr->data”.
- Assign “NULL” to “temp_ptr->link”.
- Assign temporary pointer to the link.
- Assign temporary pointer to the back.
- Check if the queue is empty.
- Give definition for the method “remove ()”.
- Check if the queue is empty.
- Print the message.
- Store the value into the variable “result”.
- Create an object “discard” for the pointer “QueueNodePtr”.
- Assign “front” to “discard”.
- Assign the link of front to “front”.
- Check if the front is null.
- Assign null to the back.
- Delete the object “discard”.
- Return “result”.
- Check if the queue is empty.
PriorityQueue.h:
- Include required header files.
- Create a namespace named “queuesavitch”.
- Declare a class “PriorityQueue”.
- Inside “public” access specifier,
- Declare the constructor and destructor.
- Declare the virtual function.
- Inside “public” access specifier,
- Declare a class “PriorityQueue”.
PriorityQueue.cpp:
- Include required header files.
- Create a namespace named “queuesavitch”.
- Declare the constructor and destructor.
- Give function to remove items.
- Check if the queue is empty.
- Print the messge.
- Assing “front->data” to “smallest”.
- Assign “NULL” to “nodeBeforeSmallest”.
- Assign “front” to “previousPtr”.
- Assign the link of front to “current”.
- Using while condition “current != NULL”,
- Check if the data is smaller.
- Assing “current->data” to “smallest”.
- Assign “previousPtr” to “nodeBeforeSmallest”.
- Assign “current” to “previousPtr”.
- Assign “current->link” to “current”.
- Check if the data is smaller.
- Create an object “discard”.
- Check if the node is null.
- Assign “front” to “discard”.
- Assign the link of front to “front”.
- Check if the link is equal to back.
- Assign “back” to “discard”.
- Assign “nodeBeforeSmallest” to “back”.
- Assign “NULL” to “back->link”.
- Else,
- Assign “nodeBeforeSmallest->link” to “discard”.
- Assign “discard->link” to “nodeBeforeSmallest->link”.
- Check if front is equal to null.
- Assign “NULL” to “back”.
- Delete the object “discard”.
- Return the smallest item.
- Check if the queue is empty.
main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object for “PriorityQueue”.
- Add the integers to the queue using “add ()” method.
- While “(!que.empty())”,
- Call the function “que.remove()”.
- Declare a character variable.
- Get a character.
- Return the statement.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Given the linked list data structure, implement a sub-class TSortedList that makes sure that elements are inserted and maintained in an ascending order in the list. So, given the input sequence {1,7,3,11,5}, when printing the list after inserting the last element it should print like 1, 3, 5, 7, 11. Note that with inheritance, you have to only care about the insertion situation as deletion should still be handled by the parent class.
Write the definition of the function moveNthFront that takes as a parameter a positive integer, n. The function moves the nth element of the queue to the front. The order of the remaining elements remains unchanged. For example, suppose:queue = {5, 11, 34, 67, 43, 55} and n = 3.After a call to the function moveNthFront:queue = {34, 5, 11, 67, 43, 55}Add this function to the class queueType. Also, write a program to test your method.
Assume you have a class called Deck which represents a deck of cards using a singly linked lists. Each node is an object of type Card with a field called next where a reference to the next Card is stored. A field from the Deck class called head stores a reference to the card on the top of the deck, and a field called tail stores a reference to the last card, at the bottom of the deck.
We can cut a deck of cards by performing what is called a triple cut: the deck is partitioned into three parts and the bottom part is positioned between the first two. You would like to write a method that implements such a cut on your deck data structure. The method will take as input two cards, the first one being the last card of the first part of the deck, and the second being the first one of the third part of the deck.
For example, assume a deck contains cards in the following order
AC 2C 3C 4C 5C AD 2D 3D 4D 5D
After calling tripleCut() on the deck the card, with input a reference to 3C and a…
Chapter 15 Solutions
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
Ch. 15.1 - Is the following program legal (assuming...Ch. 15.1 - Prob. 2STECh. 15.1 - Is the following a legal definition of the member...Ch. 15.1 - The class SalariedEmployee inherits both of the...Ch. 15.1 - Give a definition for a class TitledEmployee that...Ch. 15.1 - Give the definitions of the constructors for the...Ch. 15.2 - You know that an overloaded assignment operator...Ch. 15.2 - Suppose Child is a class derived from the class...Ch. 15.2 - Give the definitions for the member function...Ch. 15.2 - Define a class called PartFilledArrayWMax that is...
Ch. 15.3 - Prob. 11STECh. 15.3 - Why cant we assign a base class object to a...Ch. 15.3 - What is the problem with the (legal) assignment of...Ch. 15.3 - Suppose the base class and the derived class each...Ch. 15 - Write a program that uses the class...Ch. 15 - Listed below are definitions of two classes that...Ch. 15 - Solution to Programming Project 15.1 Give the...Ch. 15 - Create a base class called Vehicle that has the...Ch. 15 - Define a Car class that is derived from the...Ch. 15 - Prob. 4PPCh. 15 - Consider a graphics system that has classes for...Ch. 15 - Flesh out Programming Project 5. Give new...Ch. 15 - Banks have many different types of accounts, often...Ch. 15 - Radio Frequency IDentification (RFID) chips are...Ch. 15 - The goal for this Programming Project is to create...Ch. 15 - Solution to Programming Project 15.10 Listed below...Ch. 15 - The computer player in Programming Project 10 does...Ch. 15 - Prob. 12PP
Knowledge Booster
Similar questions
- The implementation for the priority queue in this chapter starts by modifying theHeap class so that the order of the elements can be determined by the programmer.Earlier the heap kept the highest value on the top of the container, but now it willbe altered to allow for either the highest or the lowest. The changes made to thisclass involve specifying another template parameter, which will be used for thecomparison operator. Altering the push() and pop() functions to make use of thecomparison operator instead of assuming that the largest value element will be ontop. use c++ to code.arrow_forwardin java, Two abstract data types are the ordered list and the unordered list. Explain how these two ADTs are similar and how they differ. To answer this question, assume that you do not know how they are implemented (that is, whether they are implemented using an array or a linked list).arrow_forwardProgramming Exercise 8 asks you to redefine the class to implement the nodes of a linked list so that the instance variables are private. Therefore, the class linkedListType and its derived classes unorderedLinkedList and orderedLinkedList can no longer directly access the instance variables of the class nodeType. Rewrite the definitions of these classes so that these classes use the member functions of the class nodeType to access the info and link fields of a node. Also write programs to test various operations of the classes unorderedLinkedList and orderedLinkedList. template <class Type>class nodeType{public:const nodeType<Type>& operator=(const nodeType<Type>&);//Overload the assignment operator.void setInfo(const Type& elem);//Function to set the info of the node.//Postcondition: info = elem;Type getInfo() const;//Function to return the info of the node.//Postcondition: The value of info is returned.void setLink(nodeType<Type>…arrow_forward
- In java complete the class of the skeleton code at the bottom using the instructions bellow. the constructor is passed a threshold and one or more integer nums (called s) that indicate the conditions to use in the work() method (both of which must be stored in the attribute of the same name).a. A s of 1 means an element in the List that is greater than the threshold will not be filtered out by the work() method.b. A s of 0 indicates that an element in the List that is equal to the threshold will not be filtered out by the work() method.c. A s of -1 indicates that an element in the List that is less than the threshold will not be filtered out by the work() method. d. The s values need not be ordered. Bellow a skeleton code to help: public class Thre_shold { private int[] s; private LD hold; public Thre_shold(final double hold, final int... s) { this.hold = new LD("Threshold", hold); this.s = s; } public List<LD> work(List<LD> g) { }arrow_forwardImplement a Doubly linked list to store a set of Integer numbers (no duplicate) Using Java• Instance variable• Constructor• Accessor and Update methods1. Define a Node Class.a. Instance Variables # E element - (generics framework) # Node next (pointer) - refer to the next node (Self-referential) # Node prev (pointer) - refer to the previous node (Self-referential)b. Constructorc. Methods # E getElement() //Return the value of this node. # setElement(E e) // Set value to this node. # Node getNext() //Return the next pointer of this node. # setNext(Node n) //Set the next pointer to this node. # Node getPrev() //Return the pointer of this node. # setPrev(Node n) //Set the previous pointer to this node. # displayNode() //Display the value of this node.arrow_forwardWrite a C++ program that creates and prints a linked list of classes. Base this program on what you know so far about using structures and linked lists, however, create a class. Create a class with private member variables string and next (to point to the next class in the list). You'll need setters and getters. The string will simply be a word you enter. Request a word from the user until the user enters end. As the words are entered, build a linked list of classes, forward. Once the list is built, print the list of classes. Enter at least 4 words for a linked list of 4 class objects. You may call the class list . The program must compile and create at least one node with data.arrow_forward
- CODE IN JAVA You have probably heard about the deque (double-ended queue) data structure, which allows for efficient pushing and popping of elements from both the front and back of the queue. Depending on the implementation, it also allows for efficient random access to any index element of the queue as well. Now, we want you to bring this data structure up to the next level, the teque (triple-ended queue)!arrow_forwardThere’s a somewhat imperfect analogy between a linked list and a railroad train, where individual cars represent links. Imagine how you would carry out various linked list operations, such as those implemented by the member functions insertFirst(), removeFirst(), and remove(int key) from the LinkList class in this hour. Also implement an insertAfter() function. You’ll need some sidings and switches. You can use a model train set if you have one. Otherwise, try drawing tracks on a piece of paper and using business cards for train cars.arrow_forwardDraw an UML class diagram for the following code: import java.util.EmptyStackException; class Node { int data; Node next; public Node(int data) { this.data = data; this.next = null; } } class List { private Node head; private int size; public List() { head = null; size = 0; } public void add(int data) { Node newNode = new Node(data); if (head == null) { head = newNode; } else { Node current = head; while (current.next != null) { current = current.next; } current.next = newNode; } size++; } public int get(int index) { if (index < 0 || index >= size) { throw new IndexOutOfBoundsException("Index out of range"); } Node current = head; for (int i = 0; i < index; i++) { current = current.next; } return current.data; } public void…arrow_forward
- Create a class Circular Queue that implements the functionality of a queue providing all therequired operations (Enqueue(), Dequeue(), Empty(), Full() and getFront()). in C++arrow_forwardDraw a memory map for the code you see on the next page, until the execution reaches the point indicated by the comment /* HERE */.In your diagram:• You must have a stack, heap, and static memory sections • Identify each frame as illustrated by the previous examples.• Draw your variables as they are encountered during program execution. Code: public class Passenger {private String name;private int ticketCost;private StringBuffer luggage;private static final int LUGGAGE_COST = 20;public Passenger(String name, int ticketCost) {this.name = name;this.ticketCost = ticketCost;luggage = new StringBuffer();}public Passenger addLuggage(String desc) {ticketCost += LUGGAGE_COST;luggage.append(desc);return this;}public int getTicketCost() {return ticketCost;}public Passenger reduceCost(int by) {ticketCost -= by;/* HERE */return this;}public String toString() {return "Passenger [name=" + name + ", ticketCost=" + ticketCost + ", luggage=" + luggage + "]";}}public class Driver {public static void…arrow_forwardA) Write a generic Java queue class (a plain queue, not a priority queue). Then, call it GenericQueue, because the JDK already has an interface called Queue. This class must be able to create a queue of objects of any reference type. Consider the GenericStack class shown below for some hints. Like the Stack class below, the GenericQueue should use an underlying ArrayList<E>. Write these methods and any others you find useful: enqueue() adds an E to the queue peek() returns a reference to the object that has been in the queue the longest, without removing it from the queue dequeue() returns the E that has been in the queue the longest, and removes it from the queue contains(T t) returns true if the queue contains at least one object that is equal to t *in the sense that calling .equals() on the object with t the parameter returns true.* Otherwise contains returns false. size() and isEmpty() are obvious.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
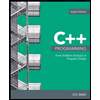
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning