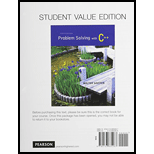
Consider a graphics system that has classes for various figures—rectangles, squares, triangles, circles, and so on. For example, a rectangle might have data members for height, width, and center point, while a square and circle might have only a center point and an edge length orradius, respectively. In a well-designed system, these would be derived from a common class, Figure. You are to implement such a system. The class Figure is the base class. You should add only Rectangle and Triangle classes derived from Figure. Each class has stubs for member functions erase and draw. Each of these member functions outputs amessage telling what function has been called and what the class of the calling object is. Since these are just stubs, they do nothing more than output this message. The member function center calls the erase and draw functions to erase and redraw the figure at the center. Since you have only stubs for erase and draw, center will not do any “cantering” but will call the member functions erase and draw. Also add an output message in the member function center that announces that center is being called. The member functions should take no arguments.
There are three parts to this project:
a. Write the class definitions using no virtual functions. Compile andtest.
b. Make the base class member functions virtual. Compile and test.
c. Explain the difference in results.
For a real example, you would have to replace the definition of each of these member functions with code to do the actual drawing. You will be asked to do this in
Use the following main function for all testing:
//This program tests Programming Project 5. #include <iostream> #include "figure.h" #include "rectangle.h" #include "triangle.h" using std::cout; int main( ) { Triangle tri; tri.draw( ); cout<< "\nDerived class Triangle object calling center( ).\n"; tri.center( ); //Calls draw and center Rectangle rect; rect.draw( ); cout<< "\nDerived class Rectangle object calling center().\n"; rect.center( ); //Calls draw and center return 0; } |

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Concepts Of Programming Languages
Introduction to Programming Using Visual Basic (10th Edition)
Starting Out with Python (4th Edition)
Starting out with Visual C# (4th Edition)
- Write a java program Create an Abstract class Student that contains a method take exam, implement the method in the child classes PhdStudent and GradStudent in which PhdStudent takes exam by giving his final defense presentation while the graduate student gives a written paper.arrow_forwardBelow we have class diagrams with three classes for a simple java based parking management class. Use the classes below to write java code for classes :a parking observer class (that implements an observer pattern) , a transaction manager class and a parking lot class(subject to the observer). Use the class diagram and java class to implement it. You should use an observer pattern to implement your code.The main goal is to implement the following - Once a car enters (in an entry-scan only lot) or leaves (in an entry-scan and exit-scan lot), then the ParkingObserver will be updated, and then can register the charge with the parking system via the TransactionManager’s park() methodarrow_forwardWe have a parking office class for an object-oriented parking management system using java Add (implement) a method to the Parking Office class to return a collection of permit ids (getPermitIds) I have attached two class diagrams with definitions of all related classes in our system (i.e car, customer, .....). N.B. Parking office methods in the class definition like register, getcustomer and addcharge have already been implemented, we just need an additional getPermitIds method as mentioned abovearrow_forward
- An arithmetic progression is a sequence of numbers such that the difference between the consecutive terms is constant. For instance, the sequence 5, 7, 9, 11, 13, 15 is an arithmetic progression with a common difference of 2. Given the class IntNumber: 1. public class IntNumber 2. { 3. public final int value; 4. 5. public IntNumber(int number) { 6. this.value = number; 7. } 8. } where value is an immutable field, that can be directly accessed (public), implement a class called ArithmeticProgression that implements the following methods: •1 public static IntNumber getArithmeticProgressionDifference(ArrayList< IntNumber> list) which returns the difference between terms of the arithmetic progression (as an object of type IntNumber) if the values of the IntNumber objects are an arithmetic progression. The method returns null in any other case. • public static void printIsArithmeticProgression(ArrayList<IntNumber> list ) which prints on the console: The list is an arithmetic…arrow_forwarda) Implement a class Point with three attributes, x, y, and z.b) Implement an init method with an optional parameter type: 1) Set the default value of x, y, and z to 0.c) Implement a display method to print the values of x, y, and z as the example output below.d) Instantiate two objects of type Point, one with arguments,1, 2, and 3, and the other one without any arguments.e) Call display() to print x, y, and z. Example Output(x, y, z): (1, 2, 3)(x, y, z) : (0, 0, 0)arrow_forwardDraw an inheritance hierarchy representing parts of a -computer system (processor, memory, disk drive, DVD drive, printer, scanner, keyboard, mouse, etc.).arrow_forward
- We have a parking office class for an object-oriented parking management system using java Add (implement) a method to the Parking Office to return a collection of permit ids (getPermitIds) I have attached two class diagrams with definitions of all related classes in our system (i.e car, customer, .....). N.B. Parking office methods in the class definition like register, getcustomer and addcharge have already been implemented, we just need an additional getPermitIds method as mentioned above Explain your code in a few wordsarrow_forwardImagine you have two classes: Employee (which represents being an employee) and Ninja (which represents being a Ninja). An Employee has both state and behaviour; a Ninja has only behaviour. You need to represent an employee who is also a ninja (a common problem in the real world). By creating only one interface and only one class (NinjaEmployee), show how you can do this without having to copy method implementation code from either of the original classes.arrow_forwardThe Doctor program described in Chapter 5 combines the data model of a doctor and the operations for handling user interaction. Restructure this program according to the model/view pattern so that these areas of responsibility are assigned to separate sets of classes. The program should include a Doctor class with an interface that allows one to obtain a greeting, a signoff message, and a reply to a patient’s string. To implement the greeting, define a method named greeting for the Doctor class. To implement the signoff message, define a method named farewell for the Doctor class. Both greeting and farewell should return a string with a greeting or farewell message respectively. The reply function is defined for you, it should be added as a method for the Doctor class. The rest of the program, in a separate main program module, handles the user’s interactions with the Doctor object. Develop this program with a terminal-based user interface. Note: The program should output in the…arrow_forward
- Draw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a…arrow_forwardDraw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a…arrow_forwardDraw the UML class diagrams for the following classes: An abstract Java class called Person that has the following attributes: a String called idNumber a Date called dateOfBirth representing the date of birth. a String for name A class called VaccineRecord with the following attributes: an enum called type of VaccineType a Date called date a String called batchCode A class called Patient that extends the Person class and has the following attributes and behaviours: an ArrayList that contains VaccineRecord objects called vaccinationRecord a public method called vaccinate that takes a VaccineRecord with the following signature: public void vaccinate(VaccineRecord vaccineRecord); a public boolean method called isVaccinated that returns whether or not the Patient has had a vaccine. A MedicalPractitioner class that extends the Person class and has the following attributes and behaviours: a String called licenseCode a public method called vaccinatePatient that takes as a…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
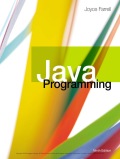