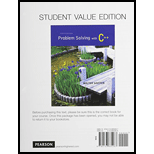
Concept explainers
Create a base class called Vehicle that has the manufacturer’s name (type string), number of cylinders in the engine (type int), and owner (type Person, given below). Then create a class called Truck that is derived from Vehicle and has additional properties: the load capacity in tons (type double since it may contain a fractional part) and towing capacity in pounds (type int). Be sure your classes have a reasonable complement of constructors, accessor, and mutator member functions, an overloaded assignmentoperator, and a copy constructor. Write a driver program that tests all your member functions.
The definition of the class Person follows. The implementation of the classis part of this
class Person { public: Person(); Person(string theName); Person(const Person& theObject); string getName() const; Person& operator = (const Person& rtSide); friend istream& operator >>(istream& inStream, Person& personObject); friend ostream& operator <<(ostream& outStream, const Person& personObject); private: string name; }; |

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
Additional Engineering Textbook Solutions
Database Concepts (7th Edition)
Artificial Intelligence: A Modern Approach
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Computer Systems: A Programmer's Perspective (3rd Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Concepts Of Programming Languages
- All vehicles used for transportation in the U.S. must have identification, which varies according to the type of vehicle. For example, all automobiles have a unique Vehicle Identification Number (VIN) assigned by the manufacturer, plus a license plate number assigend by the state in which the auto is registerd. Your task is to modify the Auto class below to override the equals method of the Vehicle class to test that the VIN and license plate number are identical.arrow_forwardIn the code template below, you are given three classes: Card, CardSet, and Deck. The Card class has the attributes of value and suite, which describe the cards in a standard 52-card deck. The attribute value takes in the digits 1 to 10 as well as the standard face cards, Jack, Queen, and King. The suite attribute takes in the four suites, namely clubs, spades, hearts, and diamonds. Obviously, two cards are equal if they have the same value and suite. The CardSet class is just an ensemble of Card instances. To know which cards are in your set, just iterate through the cards via the view method. This gets a formatted list of your cards in the cards attribute. To add cards to your set, use the add_cards method. The Deck class is a child class of the CardSet class. To initialize a deck, all 52 cards from the standard deck must be added to it. For uniformity, place each suite in ascending value -- 1 to 10, then Jack, then Queen, then King. The suites must be placed in this order: clubs -…arrow_forwardIn the code template below, you are given three classes: Card, CardSet, and Deck. The Card class has the attributes of value and suite, which describe the cards in a standard 52-card deck. The attribute value takes in the digits 1 to 10 as well as the standard face cards, Jack, Queen, and King. The suite attribute takes in the four suites, namely clubs, spades, hearts, and diamonds. Obviously, two cards are equal if they have the same value and suite. The CardSet class is just an ensemble of Card instances. To know which cards are in your set, just iterate through the cards via the view method. This gets a formatted list of your cards in the cards attribute. To add cards to your set, use the add_cards method. The Deck class is a child class of the CardSet class. To initialize a deck, all 52 cards from the standard deck must be added to it. For uniformity, place each suite in ascending value -- 1 to 10, then Jack, then Queen, then King. The suites must be placed in this order: clubs -…arrow_forward
- Consider a class BankAccount that has • Two attributes i.e. accountID and balance and• A function named balanceInquiry() to get information about the current amount in the account Derive two classes from the BankAccount class i.e. CurrentAccount and the SavingsAccount. Both classes (CurrentAccount and SavingsAccount) inherit all attributes/behaviors from the BankAccount class. In addition, followings are required to be the part of both classes• Appropriate constructors to initialize data fields of base class• A function named amountWithdrawn(amount) to withdraw certain amount while taken into account the following conditionso While withdrawing from current account, the minimum balance should not decrease Rs. 5000o While withdrawing from savings account, the minimum balance should not decrease Rs. 10,000• amountDeposit(amount) to deposit amount in the accountIn the main() function, create instances of derived classes (i.e. CurrentAccount and SavingsAccount) and invoke their respective…arrow_forwardFirst, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information, and all of these should be defined as Private: A first name (given name) A last name (family name/surname) Student number (ID) – an integer number (of type long) The Student class will have at least the following constructors and methods: (i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables of Student. (ii) only necessary set and get methods for a valid class design. (iii) a reportGrade method, which you have nothing to report here, you can just print to the screen a message “There is no grade here.”. This method will be overridden in the respective child classes. (iv) an equals method which compares two student objects and returns true if they have the same student number (ID), otherwise it returns false. You may add other…arrow_forwardConsider the case of a shop that sells CDs in cash. The CDs are of three types: Movie CDs, Software CDs and Music CDs. For each of the following CD types, the required attributes are:Movie CD: Movie Title, Year of Release, Lead Actor Name, CD price and CD quantitySoftware CD: Software Name, Edition, Year of Release, CD price and CD quantityMusic CD: Album Title, Year of Release, Number of Songs, Format, CD price and CD quantity All objects of the classes cannot change their state once they are instantiated. How will you implement this?arrow_forward
- In this exercise, you are going to be working with 4 classes, a Book superclass with TextBook and Novel subclasses, and a BookTester class to run your program. For the Book, TextBook, and Novel class, you will create a constructor and all getters and setters. Be sure to follow standard naming conventions for your getters and setters! Additional information for each class is below. Book Class The Book class will have a title and author as instance variables and the constructor should follow this format: public Book(String title, String author) TextBook Class The TextBook class will have a subject and edition as instance variables and the constructor should follow this format: public TextBook(String title, String author, String subject, String edition) Novel Class The Novel class will have a genre and pages as instance variables and the constructor should follow this format: public Novel(String title, String author, String genre, int pages) BookTester In the tester class, you should…arrow_forwardCreate two classes of your choice (a parent and a child) and access their properties as shownabovearrow_forwardWrite the source code of the class Passenger. A passenger can travel with an infant (i.e. his/her child). Code the class Infant as an inner class of the class Passenger. If a passenger is not travelling with an infant, the getInfantMonths method must return –1.arrow_forward
- In this challenge, we'll implement an Account class along with two derived classes, Savings and Current. Problem Statement# Write a code that has: ● A parent class named Account. ○ Inside it define: ■ a protected double member balance ■ public void Withdraw(double amount) ■ public void Deposit(double amount) ■ public void printBalance() ● Then, there are two derived classes ○ Savings class ■ has a private member interestRate set to 0.8 ■ Withdraw(double amount) deducts amount from balance with interestRate ■ Deposit(double amount) adds amount in balance with interestRate ■ printBalance() displays the balance in the account ○ Current class ■ Withdraw(double amount) deducts amount from balance ■ Deposit(double amount) adds amount in balance ■ printBalance() displays the balance in the account Input# ● In the Savings class, balance is set to 50000 in the parametrized constructor ● In the Current class, balance is set to 50000 in the parametrized constructor Output# Balance before…arrow_forwardYou are asked to write a discount system for a beauty saloon, which provides services and sells beauty products. It offers 3 types of memberships: Premium, Gold, and Silver. Premium, gold and silver members receive a discount of 20%, 15%, and 10%, respectively, for all services provided. Customers without membership receive no discount. All members receives a flat 10% discount on products purchased (this might change in the future). Your system shall consist of three classes: Customer, DiscountRate and Visit, as shown in the class diagram. It shall compute the total bill if a customer purchases $x of products and $y of services, for a visit. Also, write a test program VisitDriver (are not to be graded and optional) to exercise all the classes. Important: "Visit Class diagram - ERROR" Constructor method of Visit class must recieve Customer object instead of String name. Visit(Customer customer, Date date) The class DiscountRate contains only static variables and methods (underlined in…arrow_forwardYou are asked to write a discount system for a beauty saloon, which provides services and sells beauty products. It offers 3 types of memberships: Premium, Gold, and Silver. Premium, gold and silver members receive a discount of 20%, 15%, and 10%, respectively, for all services provided. Customers without membership receive no discount. All members receives a flat 10% discount on products purchased (this might change in the future). Your system shall consist of three classes: Customer, DiscountRate and Visit, as shown in the class diagram. It shall compute the total bill if a customer purchases $x of products and $y of services, for a visit. Also, write a test program VisitDriver (are not to be graded and optional) to exercise all the classes. Important: "Visit Class diagram - ERROR" Constructor method of Visit class must recieve Customer object instead of String name. Visit(Customer customer, Date date) The class DiscountRate contains only static variables and methods (underlined in…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
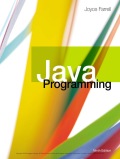
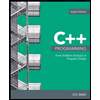
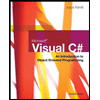