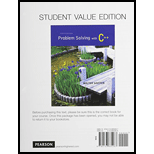
Banks have many different types of accounts, often with different rules for fees associated with transactions such as withdrawals. Customers are allowed to transfer funds between accounts incurring the appropriate fees associated with withdrawal of funds from one account.
Write a
For the classes, create a base class called BankAccount that has the name of the owner of the account (a string) and the balance in the account (double) as data members. Include member functions deposit and withdraw (each with a double for the amount as an argument) and accessor functions getName and getBalance. Deposit will add the amount to the balance (assuming the amount is nonnegative) and withdraw will subtract the amount from the balance (assuming the amount is nonnegative and less than or equal to the balance). Also create a class called MoneyMarketAccount that is derived from BankAccount. In a MoneyMarketAccount the user gets two free withdrawals in a given period of time (don’t worry about the time for this problem). After the free withdrawals have been used, a withdrawal fee of $1.50 is deducted from the balance per withdrawal. Hence, the class must have a data member to keep track of the number of withdrawals. It also must override the withdraw definition. Finally, create a CDAccount class (to model a Certificate of Deposit) derived from BankAccount that in addition to having the name and balance also has an interest rate. CDs incur penalties for early withdrawal of funds. Assume that a withdrawal of funds (any amount) incurs a penalty of 25% of the annual interest earned on the account. Assume the amount withdrawn plus the penalty are deducted from the account balance. Again, the withdraw function must override the one in the base class. For all three classes, the withdraw function should return an integer indicating the status (either ok or insufficient funds for the withdrawal to take place). For the purposes of this exercise, do not worry about other functions and properties of these accounts (such as when and how interest is paid).

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
Additional Engineering Textbook Solutions
Starting out with Visual C# (4th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Starting Out With Visual Basic (8th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
- Write a program having a base class Student with data member rollno and member functions getnum() to input rollno and putnum() to display rollno. A class Test is derived from class Student with data member marks and member functions getmarks() to input marks and putmarks() to display marks. Class Sports is also derived from class Student with data member score and member functions getscore() to input score and putscore() to display score. The class Result is inherited from two base classes, class Test and class Sports with data member total and a member function display() to display rollno, marks, score and the total(marks + score). SUBJECT: Object Oriented Programming Write Code in C++arrow_forwardWe discussed in the class that during the design, you may need a class that serves as an interface for its derived classes, but you would not create any instance of that class. Such a class is called an abstract class. The UML class diagram shown below shows a class hierarchy starting from the Vehicle class which is an abstract class. Write a program to implement this class hierarchy such that the given code in the main function runs without error. Vehicle Bus Car Truck IntraCityBus InterCityBus int main() { Vehicle* V[] = { new Bus, new Car, new Truck, new IntraCityBus, new InterCityBus }; for (int i = 0; i < 5; i++) { cout<< V[i]->move() <<endl; } return 0; } Your program should produce an output as the following: Bus: Moves to carry a large number of people Car: Moves to carry a small number of people Truck: Moves to transport goods Intra-City Bus: Intra-City Bus: Moves to carry a large number of people between two areas of a city Inter-City Bus:…arrow_forwardWrite a program In C++ to define a class “Bank” that has a member “Bank_Balance” . A person opens two accounts in the first month of the year : saving account and salary account .The initial balance in both of these accounts is 500. For account safety, the balance shouldn’t be accessible to anyone other than the person who opened the account The salary he receives each month is 40000. He doesn’t withdraw any amount for five months. Display using member functions, the balance of the salary account for five months . The person deposits 5000 in his savings account. The savings account adds an interest of 20 percent every month. Display the data for next five months of the savings account.arrow_forward
- Write the definitions of the member functions of the class calendarType (designed in Programming Exercise 9) to implement the operations of the class calendarType. Write a test program that accepts the number of a month (1 - 12) and the year from the user as input and prints the calendar for that particular month and year.arrow_forwardWrite a java program that contains variables to hold employee data like; employeeCode, employeeName and date Of Joining. Write a function that assigns the user defined values to these variables. Write another function that asks the user to enter current date and then checks if the employee tenure is more than three years or not. Call the functions in main. Now write a runner class that declares two employee objects and check their tenure periods.arrow_forwardWrite a C++ class to represent an Employee. Each employee is given a name, an age, a NIC number, anaddress, and a salary. Furthermore: The Employee class should keep track of how many employees (objects) have been created inthe system. Your Employee class should initialize all of its fields via a constructor. Create function prototypes to set and get an employee's zip code. Declare a print_employee() function that prints all of an employee object's details.(Whenever possible, use constant functions.)arrow_forward
- Create a class: Doctor, with private data members: id, name, specialization and salary with public member function: get_data () to take input for all data members, show_data() to display the values of all data members and get specialization () to return specialization. Write a program to write for detail of n doctors by allocating the memory at run time only.c++arrow_forwardWrite a program that does the following: 1-Define two classes Teacher and Department. Each Teacher has a department object as follow: [3 points] class Department{ int dno; char *name; //add data member to count number of departments automatically public: //add required methods here //define print function that prints all details of department. Note that print function is NOT function member of class Department }; class Teacher{ const int SSN; char *name; Department d; public: //add all required methods //define print function that prints all details of Teacher. Note that print function is NOT function member of class Teacher } 2-inside main do the following: a) create array of 5 Teachers. add all required details for each object inside a loop.(do NOT use cin>>) b)use print function for department and use print function for student. c)define and use a function FindTeachers that takes the array of Teachers and name of department. Then the function returns a list of all Teachers…arrow_forwardWrite the following program in C++. 1. Create a class called Employee that includes attributes: empid, name, points,group, and avg with data types: “int”, “String”, “double”, “String”, and “double”respectively.2. Include a constructor with parameters: empid and name.3. Include another constructor to assign default values to the attributes.4. Include a function called addPoints that is used to add a given amount to the valueof the attribute points.5. Include a function called upgradePoints that is used to increase the value of theattribute points by a given percentage.6. Include a function called removePoints that is used to reduce a given amount fromthe value of the attribute points. If the resultant value is negative then the value ofthe attribute should be set into zero.7. Include a function called computeGroup that assigns a value to the attribute groupbased on the value of the attribute points as given in the following table. Points Group points < 100 Silver 100 ≤…arrow_forward
- Write the definitions of the member functions of the class calendarType (designed in Programming Exercise 9) to implement the operations of the class calendarType. Write a test program that accepts the number of a month (1 - 12) and the year from the user as input and prints the calendar for that particular month and year. For example, the calendar for September 2019 is:Enter 1 - 12 to indicate the calendar month to display: 2 Enter the year: 2019 February 2019 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27. C++ only. I need calendarTypeImp.cpp & main.cpp!!! the rest classes given: calendatType.h, dateType.h, dateTypeImp.cpp, dayType.h, dayTypeImp.cppt, DateType.h, extTypeDateImp.cpparrow_forwardSuppose, we have two related (child) classes named Doctor and Patient. Data membersrequired by each class are given below. data members of doctors name type remarks name sring full name of the person age int age of the person spec string specialization of the doctor data members of patient. name type remarks name string full name of the person age int age of the person diag string diagnosed problem Using the information given above, create the following three classes. a. A parent class named Person containing common data members and functionality ofthe child classes; Doctor and Patientb. The class Doctor which is publicly inherited from the class Personc. The class Patient which is publicly inherited from the class Person Each class must contain at least two constructors in addition to the appropriate set() and show() functions. Functions of child classes should call appropriate functions of the parent class to perform the common functionality. All the…arrow_forwardWrite an OOP program in C++, which contains three, classes i.e. Parent Class, Child1 Class & Child2 Class. Parent Class inherited within Child1 Class & Child2 Class. Parent Class has one member function that is Public: void Show( );. Child1 & Child2 Class will follow the signature of the Parent Class member function; each class will have different function definitions. And during running time a message will be displayed that which class object you want to create to call their member function as shown in the output below. For Example: if enter 1 then it will call parent class function If enter 2 then it will call child1 class function If enter 3 then it will call child2 class function. Hint: Solve this Program using Run Time Polymorphism.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
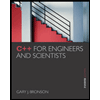
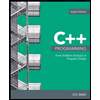