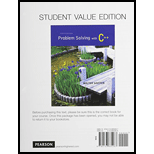
Concept explainers
Give the definitions for the member function addValue, the copy constructor, the overloaded assignment operator, and the destructor for the following class. This class is intended to be a class for a partially filled array. The member variable numberUsed contains the number of array positions currently filled. The other constructor definition is given to help you get started.
#include <iostream> #include <cstdlib> using namespace std; class PartFilledArray { public: PartFilledArray(int arraySize); PartFilledArray(const PartFilledArray& object); ~PartFilledArray(); void operator =(const PartFilledArray& rightSide); void addValue(double newEntry); //There would probably be more member functions //but they are irrelevant to this exercise. protected: double *a; int maxNumber; int numberUsed; }; PartFilledArray::PartFilledArray(int arraySize) : maxNumber(arraySize), numberUsed(0) { a = new double[maxNumber]; } |
(Many authorities would say that the member variables should be private rather than protected. We tend to agree. However, using protected makes for a better practice assignment, and you should have some experience with protected variables because some programmers do use them.)

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++, Student Value Edition plus MyProgrammingLab with Pearson eText -- Access Card Package (9th Edition)
Additional Engineering Textbook Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Starting out with Visual C# (4th Edition)
Absolute Java (6th Edition)
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Artificial Intelligence: A Modern Approach
- Based on the following Diagram answer the below questions. a. What is the Definition of Polymorphism? b. Extract from the above UML Diagram an example of Overridden method? Explain the meaning of Overridden? c. Extract from the above UML Diagram above an example of Overloading method? Explain the meaning of overloading? d. Write an array declaration that is expected to hold up to 10 objects of type Student and Employee?arrow_forwardTest the Baby class below by writing a client program which uses an array to storeinformation about 4 babies. That is, each of the four elements of the arraymust store a Baby object.If you have an array for baby names and another array for baby ages,then you have missed the point of the exercise and therefore not metthe requirement of this exercise.A Baby class object stores the required information about a Baby. Soeach Baby object will have its own relevant information, and thus eachobject must be stored in one element of the array.The client program should:a. Enter details for each baby (name and age) and thus populate theBaby arrayb. Output the details of each baby from the array (name and age)c. Calculate and display the average age of all babies in the arrayd. Determine whether any two babies in the array are the sameAs the required information for these tasks is stored in the Baby array, youwill need to use a loop to access each array element (and use the dot notationto access…arrow_forwardWrite a program that will sort a prmiitive array of data using the following guidelines - DO NOT USE VECTORS, COLLECTIONS, SETS or any other data structures from your programming language. This will also use your 'Currency' and 'Money' classes from the previous lab, so everything for this lab will be in a new file for 'main'. (Programming language Java) Create a helper function called 'RecurInsSort' such that: It is a standalone function not part of any class from the prior lab or any new class you feel like creating here, Takes in the same type of parameters as any standard Insertion Sort with recursion behavior, i.e. void RecurInsSort(Currency arr[], int size) Prints out how the array looks every time a recursive step returns back to its caller The objects in the array should be Money objects added or manipulated using Currency references/pointers. It is OK to print out the array partially when returning from a particular step as long as the process of sorting is clearly…arrow_forward
- I have written a class that has the characteristics of the data structure Set and within it house an array that stores 10 integers. I would like a function written in C++ that will perform a set union comparison between two class set instances and only print out one of each element from both of them, (no duplicates). Thanks!arrow_forwarda. Extend the definition of the class complexType so that it performs the subtraction and division operations. Overload the operators subtraction and division for this class as member functions. If (a, b) and (c, d) are complex numbers, (a, b) - (c, d) = (a - c, b - d), If (c, d) is nonzero, (a, b)/(c, d) = ((ac + bd)/(c ^2+ d ^2 ), (-ad + bc)/(c ^2 + d^ 2 )) b. Write the definitions of the functions to overload the operators – and / as defined in part a. c. Write a test program that tests the various operations on the class complexType. Format your answer with two decimal placesarrow_forwardDefine a “Invalidanalyze” function that accepts an array of “Course” objects. It will return the following information to the caller: - The number of courses with empty or blank description - The number of courses with invalid negative units - The number of courses with invalid day number of the week - The total number of units for all invalid courses in the array Show how this method is being called and return proper information.arrow_forward
- Computer Science Write the definition of the class template ArrayListType, and the derived class unordered ArrayListType. Provide implementations for the non abstract functions in the base template class, and implementations of the template functions in the derived template class. Write a test program to test the functions in the derived template class. Instantiate objects of type derived class in your test program using template parameters of type float, int.arrow_forwardCreate a Vector2D class with properties x and y and a proper constructor method. Plus, minus and multiplication with a scalar operators should be overloaded. Create a Body class with properties of pos, vel, mass and constructor(float Mass, Vector2D Pos, Vector2D Vel), addForce(Vector2D F) and Move(float dt) methods. Your program should create an array or collection of Bodies, at each time step (dt), calculate forces that bodies exert each other and apply accordingly. Finally, in a 2D space, bodies should move freely based on their properties and applied forces. You can use option buttons or another element to represent bodies and move them inside the form.arrow_forwardCreate a simple “shape” hierarchy: a base class called Shape and derived classes called Circle, Square, and Triangle. In the base class, make a virtual function called draw(), and override this in the derived classes. Make an array of pointers to Shape objects that you create on the heap (and thus perform up casting of the pointers), and calldraw() through the base-class pointers, to verify the behavior of the virtual function if your debugger supports it, single-step through the code. The subject is OOP(Object oriented programming) The code should be in C++arrow_forward
- Create a class named Collection for which each object can hold integers. The class should have following two private data members1. An integer pointer named data that holds a reference of an array created dynamically according to the specified size. 2. A constant integer named size to hold the maximum size of the array. Provide the implementation of following constructors and a destructor1. A constructor which accepts an integer that represents the size of an array and initializes it to the "empty collection," i.e., a collection whose array representation contains all 0. 2. An additional constructor that receives an array of integers and the size of that array and uses the array to initialize a collection object. 3. A copy constructor to initialize a collection object with already existing object. 4. A destructor to free any memory resources occupied by the collection object. Provide following member functions for the common set operations 1. getSize returns the size of collection.2.…arrow_forwardOne use of the this pointer is to access a data member when there is a local variable with the same name. For example, if a member function has a local variable x and a data member x, then you can refer to the data member as this->x. Use this fact to complete the constructor implementation of this Point class: class Point { public: Point(int x, int y); int get_x() const; int get_y() const; private: int x; int y; }; Not all lines are useful.arrow_forwardWrite a program to implement binary search algorithm on a dynamic array of objects of “patient” class.“patient” class contains attributes (privately defined) patient_id (int), pat_name (string) andpat_app_date (string). “patient” class should also contain member functions (publicly defined):constructor, input and output function. User will provide values for attributes for objects of “patient”class as input. Objects are required to be stored in array in ascending order on basis of patient_id. Binarysearch function should check for an object of “patient” class which will be input by user as a key valueon basis of patient_id attribute. If object is found its index location in array should display, otherwiseprogram should display a message that value is not found.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
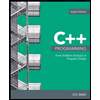